Intro
Unlock the power of VBA checkboxes! Learn how to access and manipulate checkbox values in Excel VBA with these 5 simple methods. Discover how to retrieve checkbox states, loop through checkboxes, and use checkbox values in your VBA code. Master VBA checkbox control and boost your Excel automation skills.
Excel's Visual Basic for Applications (VBA) is a powerful tool that allows users to create and automate various tasks, including working with checkboxes. Checkboxes are a common UI element in Excel, allowing users to select or deselect options. However, accessing the values of these checkboxes can be tricky. In this article, we will explore five ways to access VBA checkbox values.
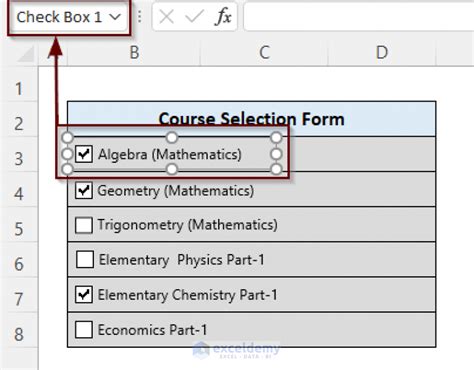
Understanding VBA Checkboxes
Before we dive into accessing checkbox values, it's essential to understand how VBA checkboxes work. VBA checkboxes are part of the Excel UserForm, which is a graphical user interface (GUI) that allows users to interact with Excel. Checkboxes are added to the UserForm using the Toolbox, and they can be linked to a cell or a variable.
Method 1: Using the CheckBox Object
The most straightforward way to access a checkbox value is by using the CheckBox object. This object has a Value
property that returns the current state of the checkbox. The Value
property can be either True
(checked) or False
(unchecked).
Sub GetCheckBoxValue()
If CheckBox1.Value = True Then
MsgBox "CheckBox1 is checked"
Else
MsgBox "CheckBox1 is not checked"
End If
End Sub
Method 2: Using the Cell Link
If a checkbox is linked to a cell, you can access its value by referencing the cell. The checkbox value is stored in the cell as a boolean value (True
or False
).
Sub GetCheckBoxValueFromCell()
If Range("A1").Value = True Then
MsgBox "CheckBox1 is checked"
Else
MsgBox "CheckBox1 is not checked"
End If
End Sub
Method 3: Using the ControlArray
If you have multiple checkboxes with the same name, you can access their values using a ControlArray. A ControlArray is an array of controls with the same name.
Sub GetCheckBoxValuesFromControlArray()
Dim i As Integer
For i = 0 To CheckBox1.Controls.Count - 1
If CheckBox1(i).Value = True Then
MsgBox "CheckBox" & i + 1 & " is checked"
Else
MsgBox "CheckBox" & i + 1 & " is not checked"
End If
Next i
End Sub
Method 4: Using the UserForm
You can also access checkbox values by referencing the UserForm. This method is useful when you have multiple checkboxes on the same UserForm.
Sub GetCheckBoxValuesFromUserForm()
Dim ctl As Control
For Each ctl In UserForm1.Controls
If TypeName(ctl) = "CheckBox" Then
If ctl.Value = True Then
MsgBox ctl.Name & " is checked"
Else
MsgBox ctl.Name & " is not checked"
End If
End If
Next ctl
End Sub
Method 5: Using the Properties Window
Finally, you can access checkbox values using the Properties window. This method is useful when you need to access a checkbox value at design time.
- Open the Visual Basic Editor (VBE).
- In the Project Explorer, select the UserForm that contains the checkbox.
- In the Properties window, select the checkbox.
- In the Properties window, find the
Value
property. - The
Value
property will display the current state of the checkbox (True
orFalse
).
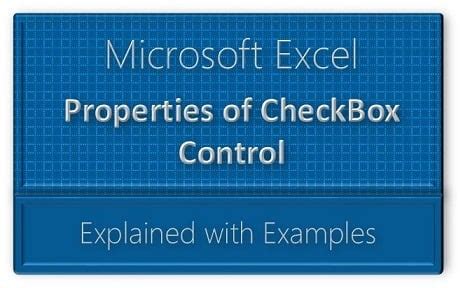
Conclusion
In this article, we explored five ways to access VBA checkbox values. Each method has its own strengths and weaknesses, and the choice of method depends on the specific requirements of your project. By mastering these methods, you can unlock the full potential of VBA checkboxes and create more powerful and interactive Excel applications.
Gallery of VBA Checkbox Images
VBA CheckBox Image Gallery
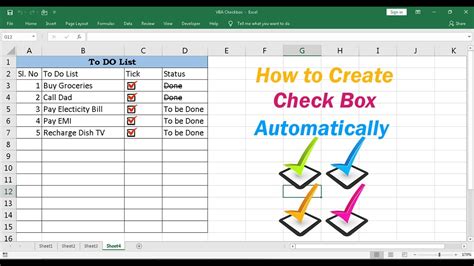
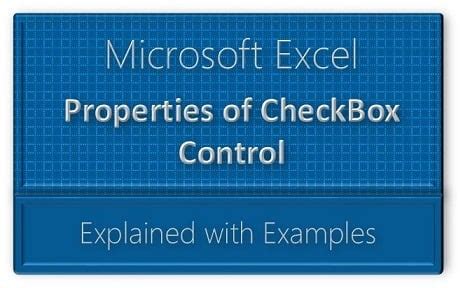
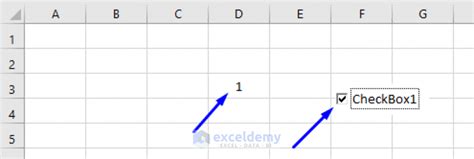
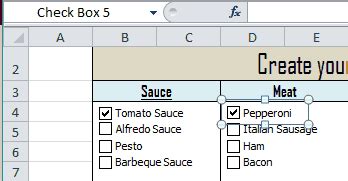
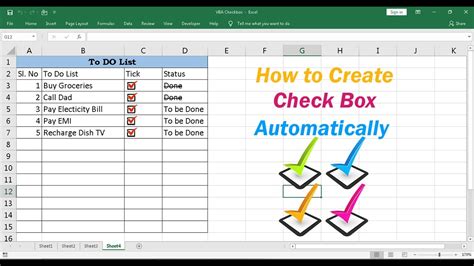
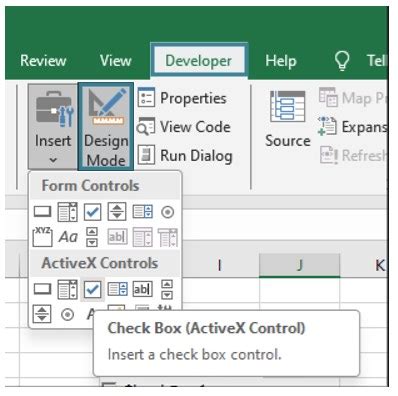