Intro
Master deleting tables in Access using VBA with ease! Learn how to use Visual Basic for Applications to permanently remove tables from your database. Discover the simple steps and code snippets to delete tables programmatically, including handling errors and table dependencies, making database management a breeze with Access VBA.
Microsoft Access is a powerful database management system that allows users to store, organize, and analyze data. While working with Access, you may need to delete tables that are no longer needed or redundant. Deleting tables can be a bit tricky, but with the help of VBA (Visual Basic for Applications), it can be done easily and efficiently.
Why Delete Tables in Access?
Before we dive into the process of deleting tables using VBA, let's quickly discuss why you might need to do so. Here are a few reasons:
- Removing redundant data: If you have duplicate tables or tables that contain redundant data, deleting them can help declutter your database and improve performance.
- Updating database structure: As your database evolves, you may need to delete tables that are no longer relevant or that have been replaced by new tables.
- Freeing up storage space: Deleting tables can help free up storage space, especially if you have large tables that are no longer needed.
Understanding VBA in Access
VBA is a programming language that allows you to automate tasks in Access. With VBA, you can create macros that perform specific actions, such as deleting tables.
To delete a table using VBA, you'll need to use the DoCmd
object, which provides a way to execute Access actions from VBA code.
Deleting a Table using VBA
Here's an example of how you can delete a table using VBA:
Sub DeleteTable()
Dim db As DAO.Database
Dim td As DAO.TableDef
Set db = CurrentDb()
Set td = db.TableDefs("TableName")
DoCmd.DeleteObject acTable, "TableName"
Set td = Nothing
Set db = Nothing
End Sub
In this example, we're using the DoCmd
object to delete a table named "TableName". The acTable
constant specifies that we're deleting a table, and the "TableName"
argument specifies the name of the table to delete.
Deleting Multiple Tables using VBA
If you need to delete multiple tables, you can modify the code to loop through a list of table names. Here's an example:
Sub DeleteMultipleTables()
Dim db As DAO.Database
Dim td As DAO.TableDef
Dim tableNames As Variant
tableNames = Array("Table1", "Table2", "Table3")
Set db = CurrentDb()
For Each tableName In tableNames
Set td = db.TableDefs(tableName)
DoCmd.DeleteObject acTable, tableName
Next tableName
Set td = Nothing
Set db = Nothing
End Sub
In this example, we're using an array to store the names of the tables to delete. We then loop through the array, deleting each table in turn.
Tips and Precautions
Before you start deleting tables using VBA, here are a few tips and precautions to keep in mind:
- Backup your database: Before deleting any tables, make sure to backup your database to prevent losing any important data.
- Verify table names: Make sure to verify the names of the tables you're deleting to avoid accidentally deleting the wrong tables.
- Use error handling: Consider adding error handling to your code to handle any errors that may occur during the deletion process.
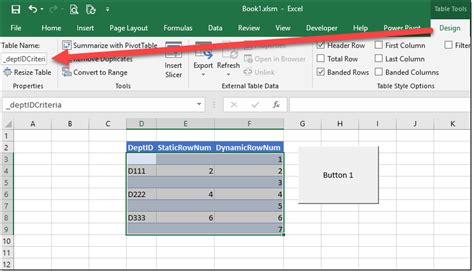
Conclusion
Deleting tables in Access using VBA can be a powerful way to automate tasks and improve database performance. By following the examples and tips provided in this article, you should be able to delete tables with ease. Remember to always backup your database and verify table names before deleting any tables.
FAQ
Q: What is VBA in Access? A: VBA (Visual Basic for Applications) is a programming language that allows you to automate tasks in Access.
Q: How do I delete a table in Access using VBA?
A: You can delete a table in Access using VBA by using the DoCmd
object and specifying the name of the table to delete.
Q: Can I delete multiple tables using VBA? A: Yes, you can delete multiple tables using VBA by looping through a list of table names.
Gallery of Delete Table Access VBA
Delete Table Access VBA Image Gallery
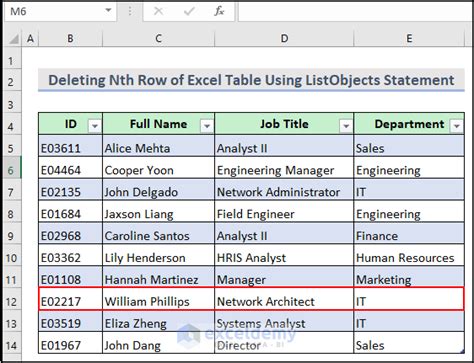
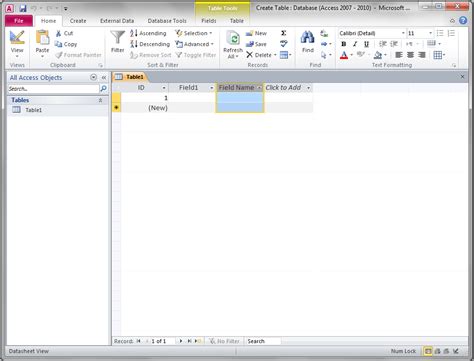

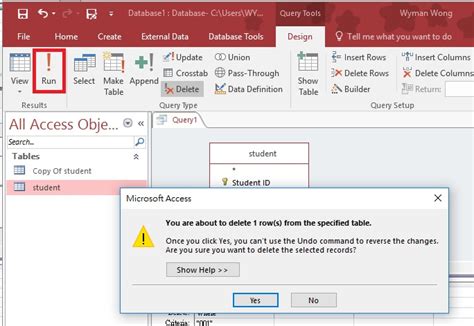
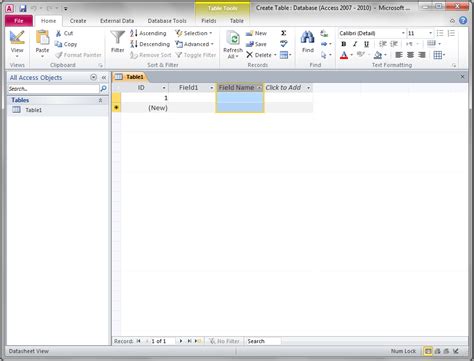
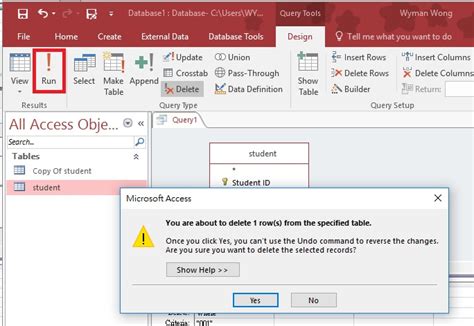
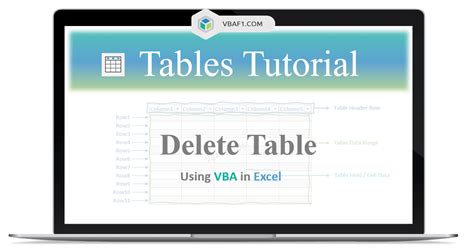
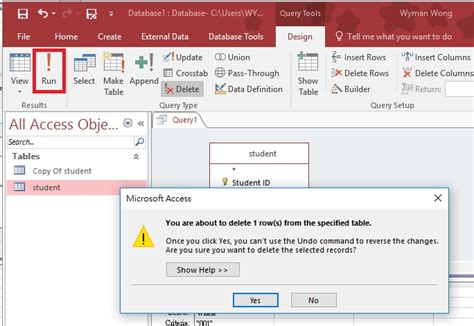
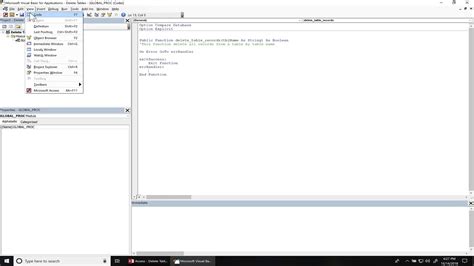