Intro
Master VBA worksheet management with our expert guide. Discover 5 efficient ways to add new worksheets in VBA, including using the Worksheets.Add method, copying existing sheets, and more. Boost productivity with these actionable VBA worksheet techniques and optimize your Excel workflow. Learn how to automate and simplify worksheet creation in VBA.
Adding new worksheets in VBA is a common task that can be achieved through various methods. In this article, we will explore five different ways to add new worksheets in VBA, including using the Worksheets.Add
method, Worksheets.Insert
method, copying an existing worksheet, using a template, and using a loop to add multiple worksheets.
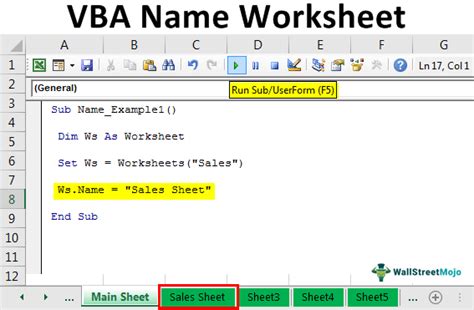
Method 1: Using the Worksheets.Add
Method
The Worksheets.Add
method is the most common way to add a new worksheet in VBA. This method allows you to specify the position of the new worksheet and the type of worksheet to add.
Sub AddNewWorksheet()
Worksheets.Add(After:=Worksheets(Worksheets.Count)).Name = "New Worksheet"
End Sub
In this example, a new worksheet is added after the last existing worksheet, and its name is set to "New Worksheet".
Method 2: Using the Worksheets.Insert
Method
The Worksheets.Insert
method allows you to insert a new worksheet at a specific position in the workbook.
Sub InsertNewWorksheet()
Worksheets.Insert(After:=Worksheets(1)).Name = "New Worksheet"
End Sub
In this example, a new worksheet is inserted after the first existing worksheet, and its name is set to "New Worksheet".
Method 3: Copying an Existing Worksheet
You can also add a new worksheet by copying an existing worksheet. This method is useful when you want to create a new worksheet with the same layout and formatting as an existing worksheet.
Sub CopyExistingWorksheet()
Worksheets("Existing Worksheet").Copy After:=Worksheets(Worksheets.Count)
ActiveSheet.Name = "New Worksheet"
End Sub
In this example, the worksheet "Existing Worksheet" is copied and added after the last existing worksheet, and its name is set to "New Worksheet".
Method 4: Using a Template
You can use a template to add a new worksheet with pre-defined layout and formatting.
Sub AddNewWorksheetFromTemplate()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Add(Template:="C:\Template\template.xlsx")
ws.Name = "New Worksheet"
End Sub
In this example, a new worksheet is added using the template file "template.xlsx" located in the "C:\Template" folder, and its name is set to "New Worksheet".
Method 5: Using a Loop to Add Multiple Worksheets
You can use a loop to add multiple worksheets at once.
Sub AddMultipleWorksheets()
Dim i As Integer
For i = 1 To 5
Worksheets.Add(After:=Worksheets(Worksheets.Count)).Name = "New Worksheet " & i
Next i
End Sub
In this example, a loop is used to add 5 new worksheets, each with a name that includes a incrementing number.
Benefits of Adding New Worksheets in VBA
Adding new worksheets in VBA can be beneficial in various ways:
- Automation: VBA allows you to automate the process of adding new worksheets, saving you time and effort.
- Customization: You can customize the new worksheet with specific layout, formatting, and data.
- Efficiency: Adding new worksheets in VBA can be more efficient than doing it manually, especially when dealing with large workbooks.
Common Errors When Adding New Worksheets in VBA
Here are some common errors to watch out for when adding new worksheets in VBA:
- Worksheet name already exists: Make sure to check if the worksheet name already exists before adding a new worksheet.
- Insufficient memory: Adding too many worksheets can cause memory issues, so make sure to monitor the workbook's size and performance.
- Incorrect worksheet type: Make sure to specify the correct worksheet type when adding a new worksheet.
VBA Worksheet Gallery
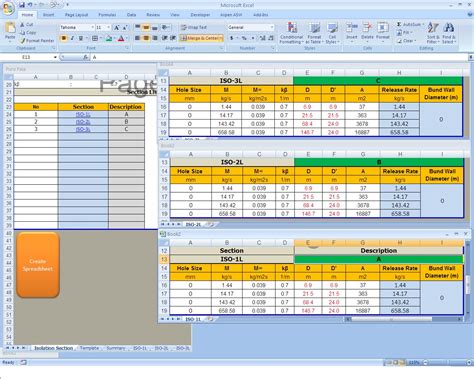
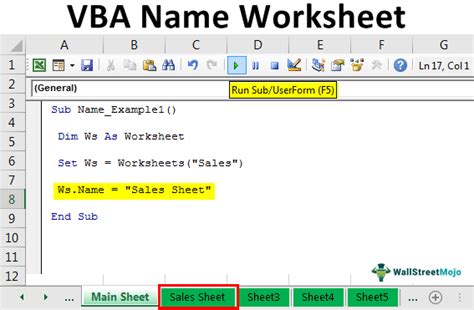
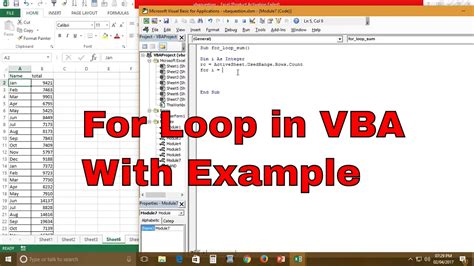
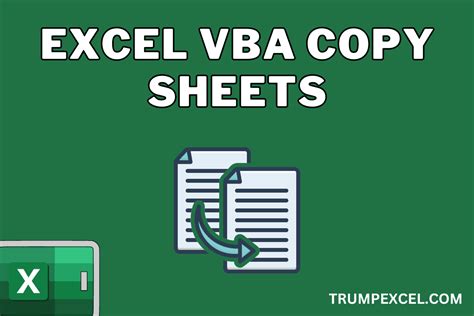
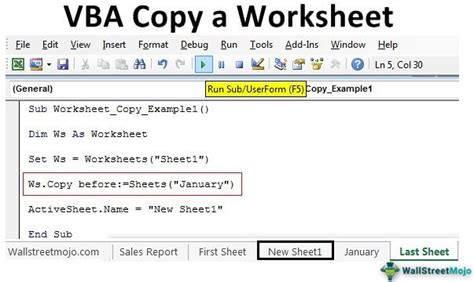
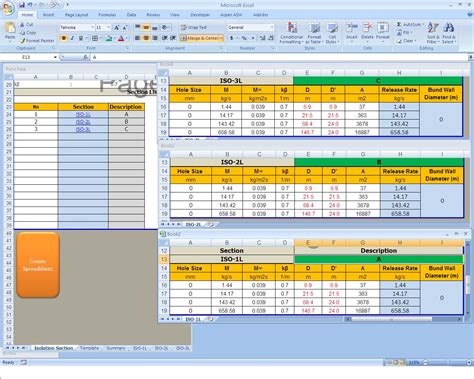
Conclusion
In this article, we explored five different ways to add new worksheets in VBA, including using the Worksheets.Add
method, Worksheets.Insert
method, copying an existing worksheet, using a template, and using a loop to add multiple worksheets. We also discussed the benefits of adding new worksheets in VBA and common errors to watch out for. By following these methods and tips, you can efficiently and effectively add new worksheets in VBA to enhance your Excel workflow.
We hope this article has been informative and helpful. If you have any questions or need further assistance, please don't hesitate to ask.