Intro
Unlock the power of automation in Google Sheets with App Script. Learn how to extract specific text from your spreadsheets using custom scripts. Discover techniques for parsing, filtering, and manipulating text data. Improve workflow efficiency and accuracy with this step-by-step guide to text extraction in Google Sheets using App Script.
Extracting specific text from Google Sheets using Google Apps Script can be a powerful way to automate tasks, such as data processing, reporting, and integration with other services. This article will guide you through the process of extracting specific text from Google Sheets using Google Apps Script.
Why Use Google Apps Script?
Google Apps Script is a cloud-based scripting platform that allows you to automate tasks within Google Sheets and other Google applications. It provides a powerful scripting language, similar to JavaScript, that can interact with Google Sheets APIs to extract, manipulate, and analyze data.
Setting Up Google Apps Script
To start using Google Apps Script, follow these steps:
- Open your Google Sheet.
- Click on "Tools" in the menu bar.
- Select "Script editor" from the drop-down menu.
- This will open the Google Apps Script editor.
Understanding Google Sheets APIs
Google Sheets provides several APIs that allow you to interact with your sheet data programmatically. The most commonly used APIs for extracting text are:
getRange()
: Retrieves a range of cells from the sheet.getValue()
: Retrieves the value of a single cell.getValues()
: Retrieves an array of values from a range of cells.
Extracting Specific Text
To extract specific text from Google Sheets using Google Apps Script, you can use the following methods:
Method 1: Extracting Text from a Single Cell
function extractTextFromCell() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var cell = sheet.getRange("A1"); // specify the cell range
var text = cell.getValue();
Logger.log(text);
}
In this example, we use the getRange()
method to select a single cell (A1) and then use the getValue()
method to retrieve the value of that cell.
Method 2: Extracting Text from a Range of Cells
function extractTextFromRange() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var range = sheet.getRange("A1:B2"); // specify the range
var values = range.getValues();
var text = values.join(","); // join the array of values into a single string
Logger.log(text);
}
In this example, we use the getRange()
method to select a range of cells (A1:B2) and then use the getValues()
method to retrieve an array of values from that range. We then use the join()
method to concatenate the array of values into a single string.
Method 3: Extracting Text using Regular Expressions
function extractTextUsingRegex() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var range = sheet.getRange("A1:B2"); // specify the range
var values = range.getValues();
var regex = /\b(text)\b/g; // specify the regular expression pattern
var text = [];
for (var i = 0; i < values.length; i++) {
for (var j = 0; j < values[i].length; j++) {
var value = values[i][j];
var match = value.match(regex);
if (match) {
text.push(match[1]);
}
}
}
Logger.log(text.join(","));
}
In this example, we use regular expressions to extract specific text from a range of cells. We define a regular expression pattern (\b(text)\b
) that matches the word "text" and then use the match()
method to search for matches in each cell value. If a match is found, we add the matched text to an array, which we then join into a single string using the join()
method.
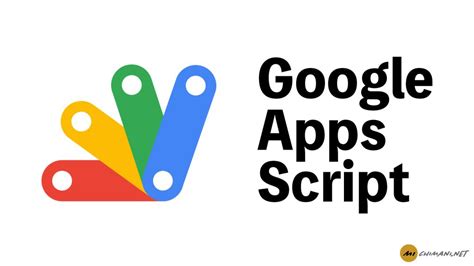
Conclusion
Extracting specific text from Google Sheets using Google Apps Script can be a powerful way to automate tasks and analyze data. By using the getRange()
, getValue()
, and getValues()
methods, you can extract text from single cells or ranges of cells. Additionally, using regular expressions can help you extract specific text patterns from your data. With Google Apps Script, the possibilities are endless!
FAQs
- Q: What is Google Apps Script? A: Google Apps Script is a cloud-based scripting platform that allows you to automate tasks within Google Sheets and other Google applications.
- Q: How do I access Google Apps Script? A: To access Google Apps Script, open your Google Sheet, click on "Tools" in the menu bar, and select "Script editor" from the drop-down menu.
- Q: What are the most commonly used APIs for extracting text in Google Sheets?
A: The most commonly used APIs for extracting text are
getRange()
,getValue()
, andgetValues()
.
Gallery of Google Apps Script
Google Apps Script Gallery
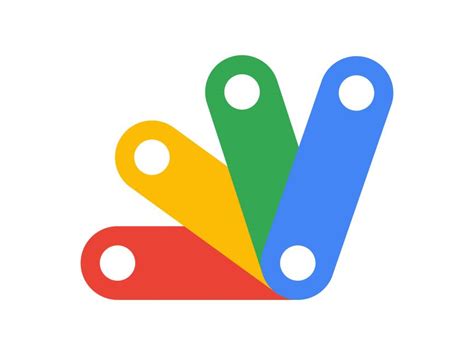
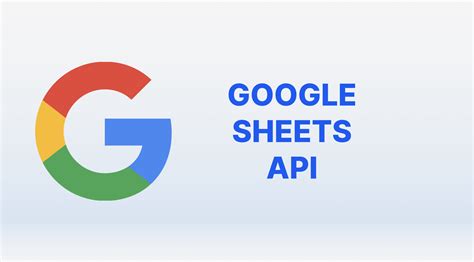
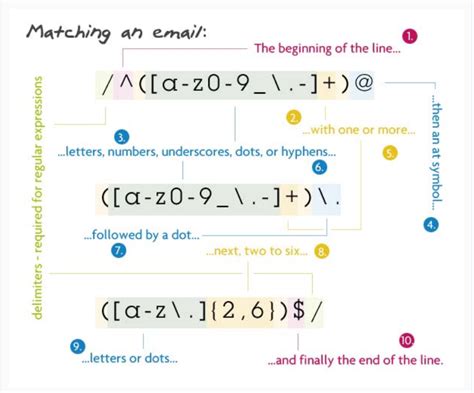
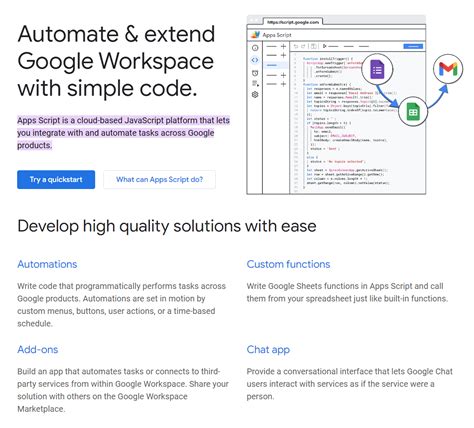
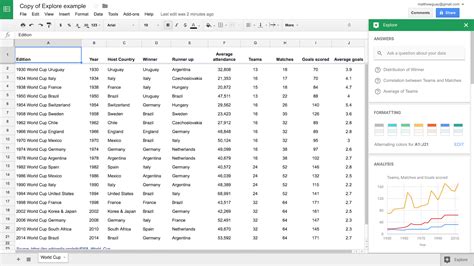
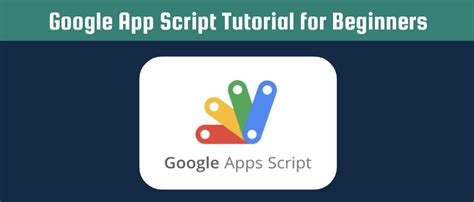
Share Your Thoughts!
What are some creative ways you've used Google Apps Script to extract specific text from Google Sheets? Share your experiences and tips in the comments below!