Intro
Unlock the power of Application.Match in VBA with 5 expert-approved techniques. Discover how to efficiently search, retrieve, and manipulate data using this powerful function. Improve your VBA skills and learn to harness the full potential of Application.Match with these actionable tips, covering topics like error handling, range matching, and formula optimization.
The world of VBA programming can be a complex and daunting place, especially when it comes to working with Excel applications. One of the most powerful and versatile tools in the VBA arsenal is the Application.Match function. In this article, we will delve into the world of Application.Match and explore five ways to master its use.
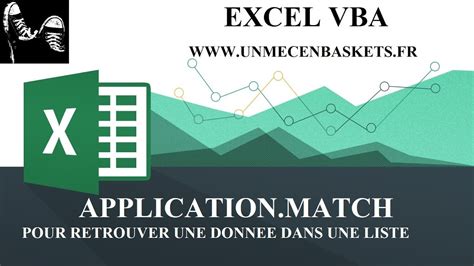
Mastering Application.Match can be a game-changer for any VBA programmer, allowing for more efficient and effective coding. So, let's dive in and explore the first way to master Application.Match.
Way 1: Understanding the Basics of Application.Match
Before we can start mastering Application.Match, we need to understand the basics of how it works. Application.Match is a function that searches for a value in a range of cells and returns the relative position of that value within the range. It is often used in conjunction with the Application.Index function to return the actual value at a specific position in a range.
The syntax for Application.Match is as follows:
Application.Match(lookup_value, lookup_array, [match_type])
lookup_value
is the value that we want to search for.lookup_array
is the range of cells that we want to search in.match_type
is an optional parameter that specifies the type of match that we want to perform. It can be one of the following values:- 1 (default): Exact match
- 0: Exact match ( legacy mode)
- -1: Exact match (same as 1)
- 2: Less than
- -2: Greater than
- 3: Wildcard match
Example 1: Using Application.Match to Find an Exact Match
In this example, we will use Application.Match to find the position of a specific value in a range of cells.
Sub Example1()
Dim rng As Range
Dim lookupValue As Variant
Dim position As Variant
' Set the range and lookup value
Set rng = Range("A1:A10")
lookupValue = "Apple"
' Use Application.Match to find the position of the lookup value
position = Application.Match(lookupValue, rng, 0)
' Check if the value was found
If IsError(position) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & position
End If
End Sub
In this example, we use Application.Match to search for the value "Apple" in the range A1:A10. If the value is found, the position is returned and we display a message box with the position. If the value is not found, we display a message box indicating that the value was not found.
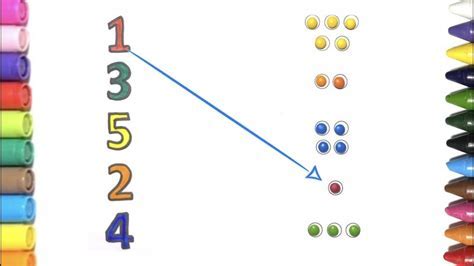
Way 2: Using Application.Match with Multiple Criteria
In the previous example, we used Application.Match to search for a single value in a range of cells. However, what if we want to search for multiple criteria? In this case, we can use the Application.Index function in conjunction with Application.Match to search for multiple criteria.
The syntax for Application.Index is as follows:
Application.Index(reference, row_num, col_num, [area_num])
reference
is the range of cells that we want to search in.row_num
is the row number that we want to return.col_num
is the column number that we want to return.area_num
is an optional parameter that specifies the area of the range that we want to return.
Example 2: Using Application.Match with Multiple Criteria
In this example, we will use Application.Match to search for multiple criteria in a range of cells.
Sub Example2()
Dim rng As Range
Dim lookupValue1 As Variant
Dim lookupValue2 As Variant
Dim position1 As Variant
Dim position2 As Variant
' Set the range and lookup values
Set rng = Range("A1:B10")
lookupValue1 = "Apple"
lookupValue2 = "Red"
' Use Application.Match to find the positions of the lookup values
position1 = Application.Match(lookupValue1, rng.Columns(1), 0)
position2 = Application.Match(lookupValue2, rng.Columns(2), 0)
' Check if the values were found
If IsError(position1) Or IsError(position2) Then
MsgBox "Values not found"
Else
MsgBox "Values found at positions " & position1 & " and " & position2
End If
End Sub
In this example, we use Application.Match to search for two values, "Apple" and "Red", in the range A1:B10. If both values are found, we display a message box with the positions. If either value is not found, we display a message box indicating that the values were not found.
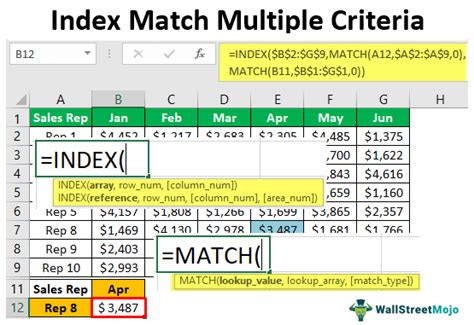
Way 3: Using Application.Match with Wildcards
In the previous examples, we used Application.Match to search for exact matches. However, what if we want to search for values that match a certain pattern? In this case, we can use wildcards with Application.Match to search for values that match a certain pattern.
Example 3: Using Application.Match with Wildcards
In this example, we will use Application.Match to search for values that match a certain pattern.
Sub Example3()
Dim rng As Range
Dim lookupValue As Variant
Dim position As Variant
' Set the range and lookup value
Set rng = Range("A1:A10")
lookupValue = "A*"
' Use Application.Match to find the position of the lookup value
position = Application.Match(lookupValue, rng, 0)
' Check if the value was found
If IsError(position) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & position
End If
End Sub
In this example, we use Application.Match to search for values that start with the letter "A". If a value is found, we display a message box with the position. If no value is found, we display a message box indicating that the value was not found.
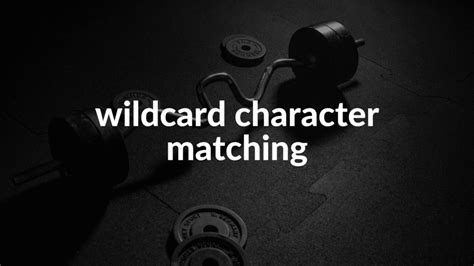
Way 4: Using Application.Match with Errors
In the previous examples, we used Application.Match to search for values and return the position of the value if found. However, what if we want to handle errors that may occur during the search process? In this case, we can use the IsError
function to check if an error occurred during the search process.
Example 4: Using Application.Match with Errors
In this example, we will use Application.Match to search for a value and handle any errors that may occur during the search process.
Sub Example4()
Dim rng As Range
Dim lookupValue As Variant
Dim position As Variant
' Set the range and lookup value
Set rng = Range("A1:A10")
lookupValue = "Apple"
' Use Application.Match to find the position of the lookup value
position = Application.Match(lookupValue, rng, 0)
' Check if an error occurred
If IsError(position) Then
MsgBox "An error occurred: " & Err.Description
Else
MsgBox "Value found at position " & position
End If
End Sub
In this example, we use Application.Match to search for the value "Apple" in the range A1:A10. If an error occurs during the search process, we display a message box with the error description. If no error occurs, we display a message box with the position of the value.
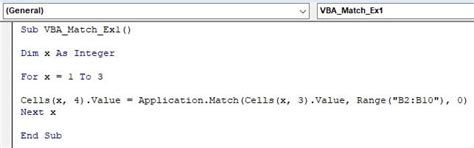
Way 5: Using Application.Match with Performance Optimization
In the previous examples, we used Application.Match to search for values in a range of cells. However, what if we want to optimize the performance of the search process? In this case, we can use the Application.ScreenUpdating
property to disable screen updating during the search process.
Example 5: Using Application.Match with Performance Optimization
In this example, we will use Application.Match to search for a value and optimize the performance of the search process.
Sub Example5()
Dim rng As Range
Dim lookupValue As Variant
Dim position As Variant
' Set the range and lookup value
Set rng = Range("A1:A10")
lookupValue = "Apple"
' Disable screen updating
Application.ScreenUpdating = False
' Use Application.Match to find the position of the lookup value
position = Application.Match(lookupValue, rng, 0)
' Enable screen updating
Application.ScreenUpdating = True
' Check if the value was found
If IsError(position) Then
MsgBox "Value not found"
Else
MsgBox "Value found at position " & position
End If
End Sub
In this example, we use Application.Match to search for the value "Apple" in the range A1:A10. We disable screen updating during the search process to optimize performance. If the value is found, we display a message box with the position. If the value is not found, we display a message box indicating that the value was not found.
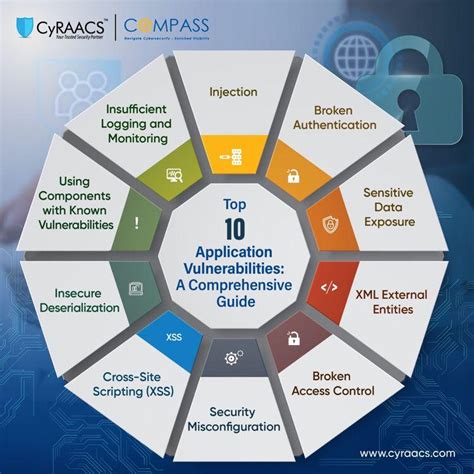
Application.Match Image Gallery
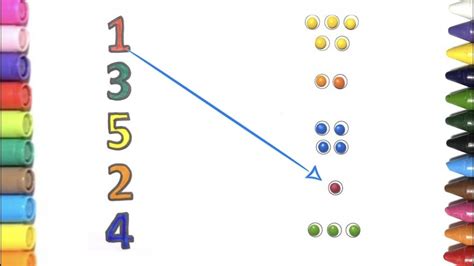
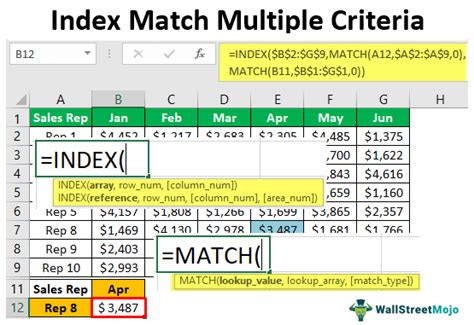
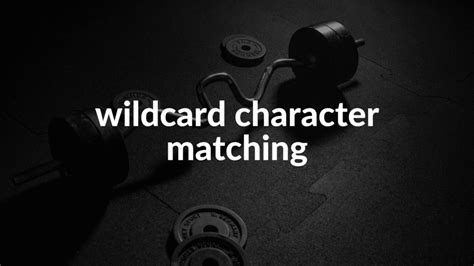
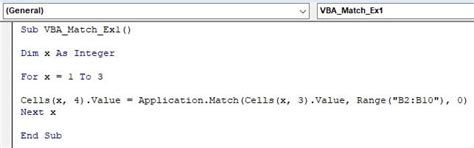
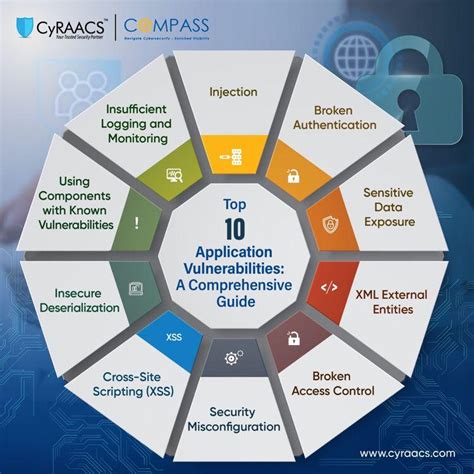
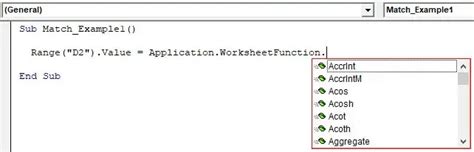
In conclusion, mastering Application.Match is a crucial skill for any VBA programmer. By understanding the basics of Application.Match, using it with multiple criteria, wildcards, errors, and performance optimization, we can write more efficient and effective code. We hope that this article has helped you to master Application.Match and improve your VBA programming skills.