Using Google Apps Script to create custom formulas can be a powerful tool for managing and analyzing data in Google Sheets. When working with formulas in Apps Script, it's not uncommon to want to leave certain cells empty based on specific conditions or requirements. This can be particularly useful when you're dealing with data validation, conditional formatting, or even dynamically generating content based on user input. In this article, we'll explore five ways to leave cells empty in Apps Script formulas, including using conditional statements, error handling, and custom functions.
Understanding the Need for Empty Cells
Before diving into the methods, it's essential to understand why leaving cells empty might be necessary. Imagine you're creating a template where certain cells should only display values under specific conditions. If those conditions aren't met, the cell should remain blank. This is crucial for maintaining the integrity of your data and avoiding unnecessary or misleading information.
Method 1: Using Conditional Statements
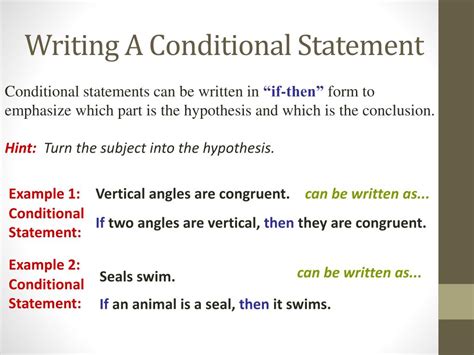
One of the most straightforward ways to leave a cell empty in Apps Script is by using conditional statements, such as if
statements. You can check for specific conditions, and if they're not met, you simply don't assign any value to the cell. Here's a basic example:
function myFunction() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var value = sheet.getRange("A1").getValue();
if (value > 10) {
sheet.getRange("B1").setValue("Greater than 10");
} else {
// Leave the cell empty
}
}
In this example, the cell B1 will only be filled with a value if the condition in A1 is met; otherwise, it remains blank.
Method 2: Using Error Handling
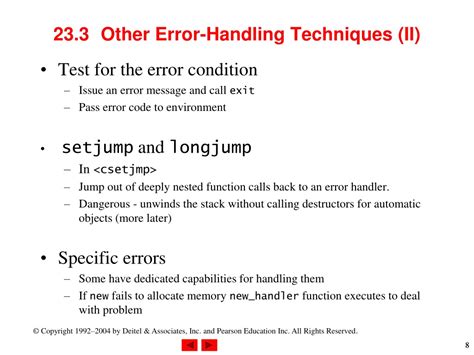
Another approach is to use error handling mechanisms. By intentionally creating a condition that will result in an error (which you then catch and handle), you can effectively leave a cell empty. Here's a simplistic example:
function myFunction() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var value = sheet.getRange("A1").getValue();
try {
if (value === "") {
throw new Error("Value is empty");
}
sheet.getRange("B1").setValue("Value is not empty");
} catch (e) {
// Leave the cell empty if an error occurs
}
}
This method is more about controlling the flow of your script rather than directly leaving a cell empty, but it can be useful in certain scenarios.
Using Custom Functions
Creating custom functions in Apps Script can provide a more elegant and reusable solution for leaving cells empty based on specific conditions. You can define a function that returns an empty string (""
)) or even a blank value (null
) under certain conditions.
function isEmptyCell(value) {
if (value > 10) {
return "Greater than 10";
} else {
return "";
}
}
You can then use this custom function in your sheet like any other formula, and it will return an empty string when the condition isn't met.
Method 4: Using Array Formulas
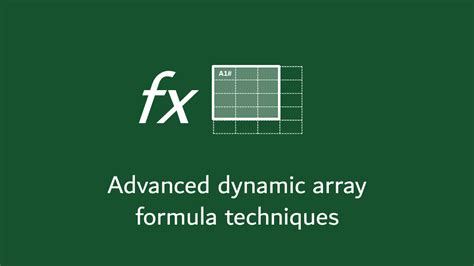
Array formulas can be incredibly powerful in Google Sheets, allowing you to perform operations on entire arrays of data. You can use array formulas in conjunction with conditional logic to leave certain cells empty. Here's a basic example of using an array formula to conditionally leave cells empty:
function myFunction() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var values = sheet.getRange("A1:A10").getValues();
var result = values.map(function(value) {
if (value[0] > 10) {
return ["Value is greater than 10"];
} else {
return [""];
}
});
sheet.getRange("B1:B10").setValues(result);
}
This script maps over an array of values, checks each value, and returns either a filled or empty string to the corresponding cell in column B.
Method 5: Using the `setDataValidation` Method
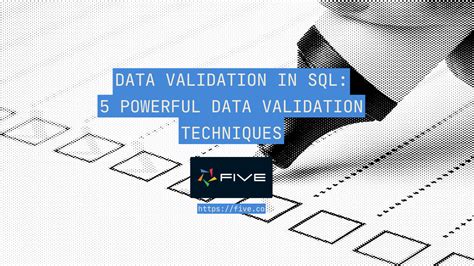
Lastly, you can use the setDataValidation
method to enforce data validation rules that, when not met, will leave a cell empty. This is more about preventing incorrect data entry rather than directly leaving cells empty based on a condition.
function myFunction() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var cell = sheet.getRange("A1");
var rule = SpreadsheetApp.DataValidation.createRule()
.requireNumberGreaterThan(10)
.setAllowInvalid(false)
.build();
cell.setDataValidation(rule);
}
This script sets a data validation rule on cell A1 that requires the entered value to be greater than 10. If the value is not valid, the cell remains empty.
Gallery of Empty Cell Techniques
Techniques for Leaving Cells Empty in Apps Script
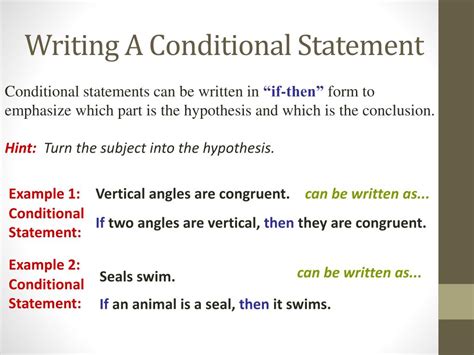
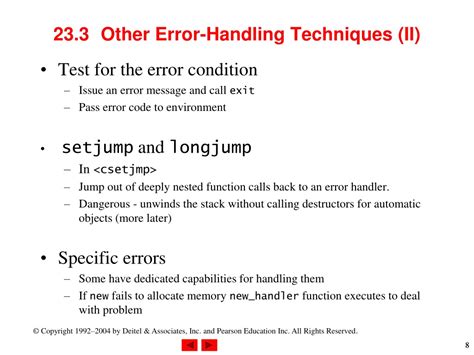
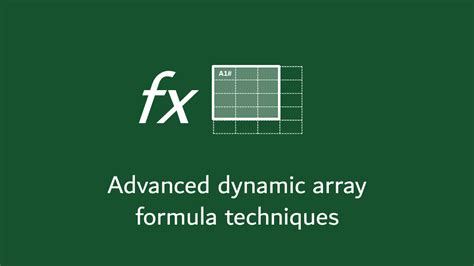
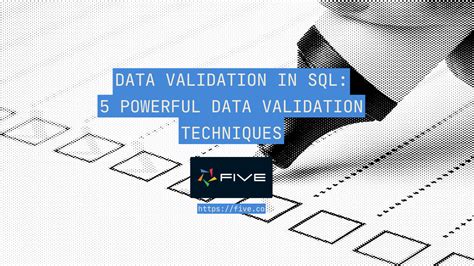
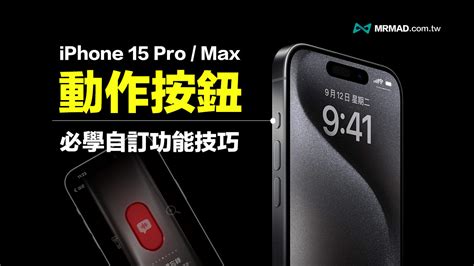
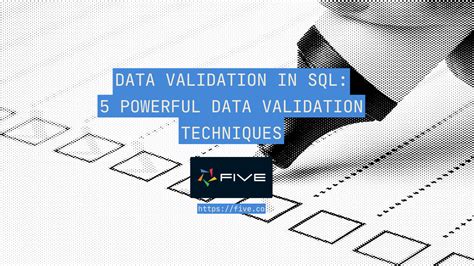
Conclusion
Leaving cells empty in Apps Script formulas can be a bit tricky, but with the right techniques, it becomes more manageable. Whether you're using conditional statements, error handling, array formulas, custom functions, or data validation rules, there's a method that fits your specific needs. By mastering these techniques, you can create more dynamic and interactive spreadsheets that provide meaningful insights into your data.
We hope this comprehensive guide has been helpful in your journey with Apps Script. If you have any questions or need further clarification on any of the methods discussed, please don't hesitate to reach out in the comments below. Share this article with anyone who might find it useful, and consider subscribing for more in-depth guides and tutorials on Google Apps Script and other productivity tools.