Intro
Resolve VBA errors with ease! Learn how to fix the Argument Not Optional error in VBA with 3 simple methods. Discover the causes of this error and master troubleshooting techniques to overcome issues with subroutine arguments, function calls, and variable declarations. Optimize your VBA code and improve productivity today!
When working with VBA, one of the most frustrating errors you can encounter is the "Argument not optional" error. This error occurs when you try to call a subroutine or function without providing all the required arguments. In this article, we will explore three ways to fix this error and get your VBA code up and running smoothly.
Understanding the Error
Before we dive into the solutions, it's essential to understand why this error occurs. When you create a subroutine or function in VBA, you can define arguments that the procedure requires to execute. These arguments are the inputs that the procedure needs to perform its task. If you try to call the procedure without providing all the required arguments, VBA will throw an "Argument not optional" error.
Solution 1: Provide All Required Arguments
The most straightforward way to fix this error is to provide all the required arguments when calling the subroutine or function. Let's consider an example:
Suppose you have a subroutine called CalculateArea
that takes two arguments, length
and width
, to calculate the area of a rectangle.
Sub CalculateArea(length As Double, width As Double)
' Calculate the area
Dim area As Double
area = length * width
MsgBox "The area is: " & area
End Sub
To call this subroutine, you need to provide both length
and width
arguments:
Sub TestCalculateArea()
CalculateArea 10, 5
End Sub
If you try to call CalculateArea
without providing both arguments, you'll get the "Argument not optional" error:
Sub TestCalculateArea()
CalculateArea 10
End Sub
To fix this, simply provide all the required arguments when calling the subroutine.
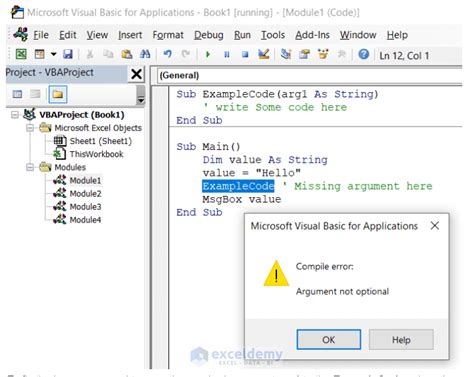
Solution 2: Use Optional Arguments
Another way to fix this error is to use optional arguments in your subroutine or function. You can do this by adding the Optional
keyword before the argument name. Here's an updated version of the CalculateArea
subroutine:
Sub CalculateArea(length As Double, Optional width As Double = 1)
' Calculate the area
Dim area As Double
area = length * width
MsgBox "The area is: " & area
End Sub
In this version, the width
argument is optional and defaults to 1 if not provided. You can now call CalculateArea
with only one argument:
Sub TestCalculateArea()
CalculateArea 10
End Sub
The subroutine will use the default value of 1 for the width
argument.
Solution 3: Use a ParamArray
A ParamArray
allows you to pass a variable number of arguments to a subroutine or function. You can use this feature to create a procedure that can handle different numbers of arguments. Here's an example:
Sub CalculateArea(ParamArray args())
' Calculate the area
Dim area As Double
area = args(0) * args(1)
MsgBox "The area is: " & area
End Sub
In this version, the CalculateArea
subroutine takes a ParamArray
called args
. You can call this subroutine with any number of arguments:
Sub TestCalculateArea()
CalculateArea 10, 5
End Sub
Note that when using a ParamArray
, you need to access the arguments using the args
array, like args(0)
and args(1)
.
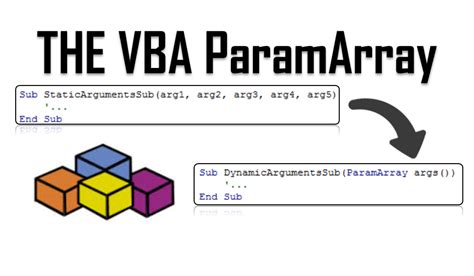
Conclusion
The "Argument not optional" error in VBA can be frustrating, but it's relatively easy to fix. By providing all required arguments, using optional arguments, or using a ParamArray
, you can create flexible and robust subroutines and functions that handle different scenarios. Remember to use these techniques to write better VBA code and avoid this error in the future.
VBA Error Gallery
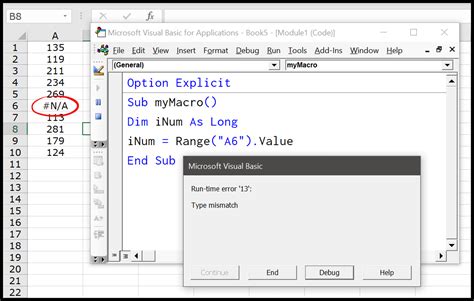
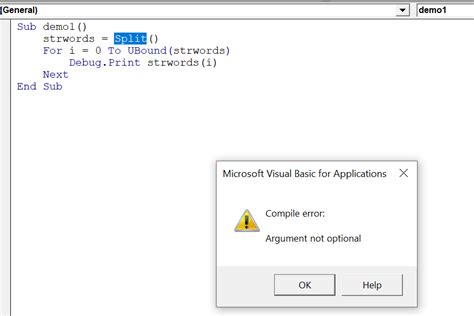
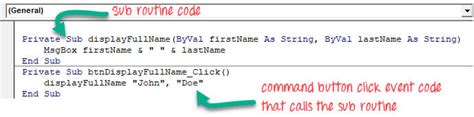
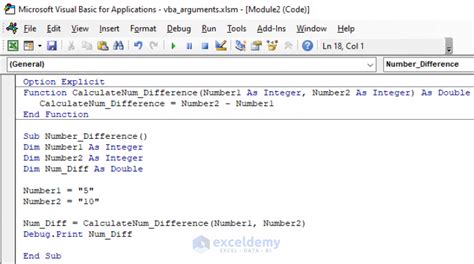
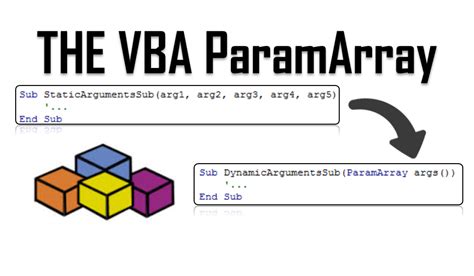
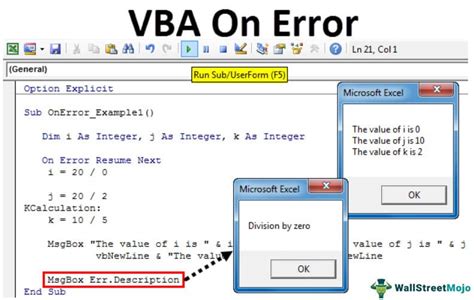
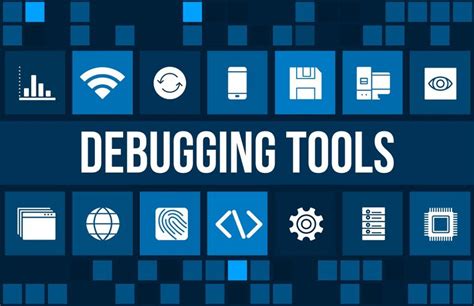
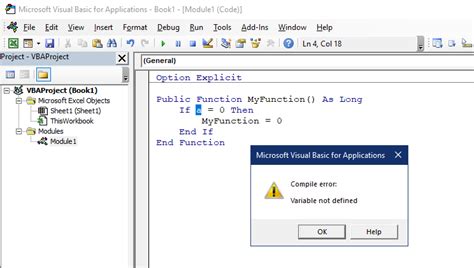
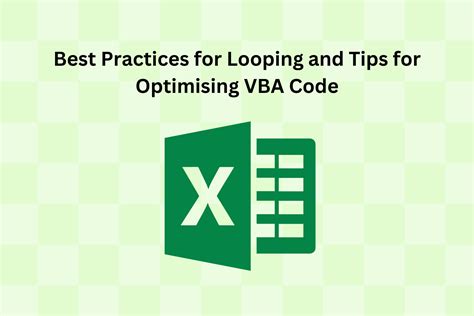
We hope this article has helped you fix the "Argument not optional" error in VBA. If you have any questions or need further assistance, please leave a comment below.