Intro
Troubleshoot ByRef argument type mismatch errors in VBA with ease. Learn how to identify and fix data type inconsistencies, incorrect variable declarations, and mismatched subroutine arguments. Master VBA debugging techniques and optimize your code with expert tips on ByRef, ByVal, and data type conversion.
Error messages can be frustrating, especially when working with VBA (Visual Basic for Applications). One common error that VBA developers encounter is the "ByRef argument type mismatch" error. This error occurs when a subroutine or function is called with an argument that is not of the expected type. In this article, we will delve into the causes of this error, provide examples, and offer step-by-step solutions to fix it.
Understanding the ByRef Argument Type Mismatch Error
When you pass an argument to a subroutine or function in VBA, it can be passed either by value ( ByVal ) or by reference ( ByRef ). ByRef means that the actual variable is passed to the procedure, and any changes made to the argument within the procedure will affect the original variable. Conversely, ByVal means that a copy of the variable is passed, and changes made within the procedure do not affect the original variable.
The "ByRef argument type mismatch" error typically occurs when a procedure is expecting an argument of a certain type (e.g., Integer, String, Range), but the argument passed is of a different type.
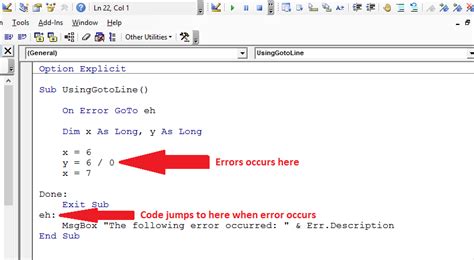
Causes of the ByRef Argument Type Mismatch Error
This error can arise from several scenarios:
- Passing an argument of the wrong data type to a procedure.
- Forgetting to specify the data type of a procedure's argument.
- Declaring an argument as ByRef when it should be ByVal, or vice versa.
Examples of ByRef Argument Type Mismatch Errors
To better understand this error, let's consider a few examples:
Example 1: Passing a Wrong Data Type
Suppose we have a procedure that expects an Integer argument, but we pass a String instead:
Sub myProcedure(ByRef myArg As Integer)
' Procedure code here
End Sub
Sub main()
Dim myString As String
myString = "Hello"
myProcedure myString ' This will cause a ByRef argument type mismatch error
End Sub
Example 2: Forgetting to Specify the Data Type
If we forget to specify the data type of an argument, VBA will assume it is a Variant, which can cause type mismatches:
Sub myProcedure(ByRef myArg)
' Procedure code here
End Sub
Sub main()
Dim myInteger As Integer
myInteger = 10
myProcedure myInteger ' This might cause issues if myProcedure expects a different type
End Sub
Solutions to Fix ByRef Argument Type Mismatch Errors
To resolve this error, follow these steps:
-
Check the Procedure Declaration: Verify that the procedure's argument is declared correctly, including its data type.
-
Match the Argument Type: Ensure that the argument you are passing to the procedure matches the expected data type.
-
Use ByVal for Value Types: When passing arguments that should not be modified by the procedure, use ByVal to prevent unintended changes.
-
Specify Argument Types: Always specify the data type for procedure arguments to avoid implicit Variants.
-
Test with Different Data Types: If you are unsure about the expected data type, try passing different types to see which one works as expected.
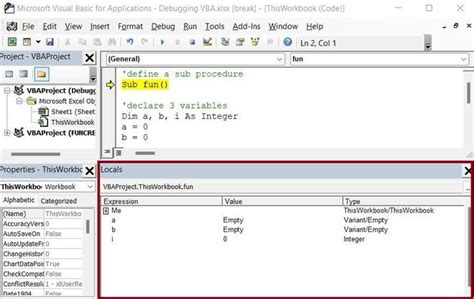
Best Practices to Avoid ByRef Argument Type Mismatch Errors
- Explicitly Declare Variables and Arguments: Always specify the data type for variables and procedure arguments.
- Use Option Explicit: This directive forces VBA to require explicit variable declarations, preventing implicit Variants.
- Document Your Code: Clearly comment your procedures to indicate the expected argument types.
By following these guidelines and understanding the causes of the ByRef argument type mismatch error, you can write more robust and error-free VBA code.
VBA Error Handling Image Gallery
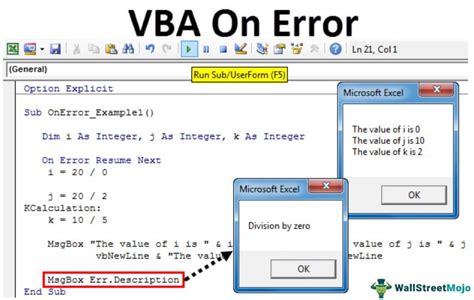
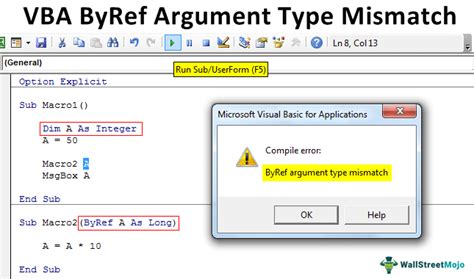
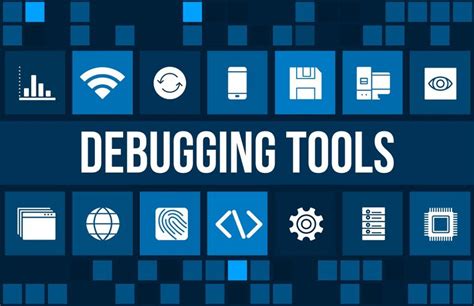
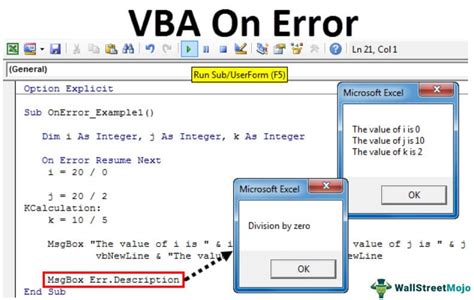
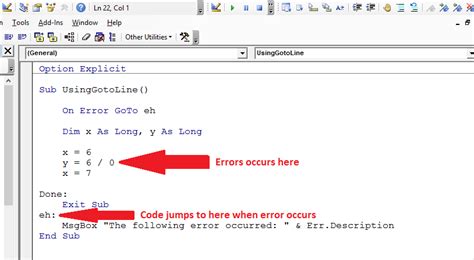
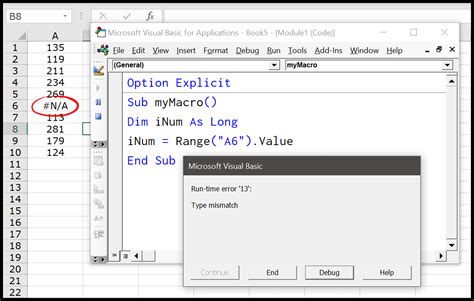
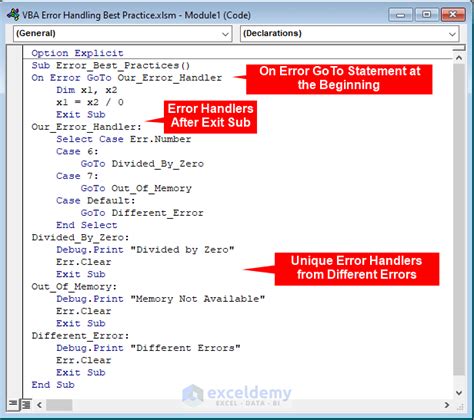
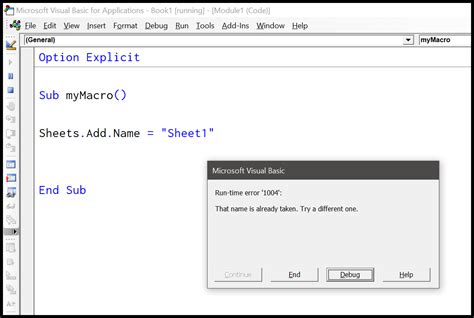
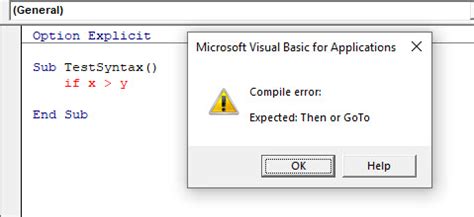
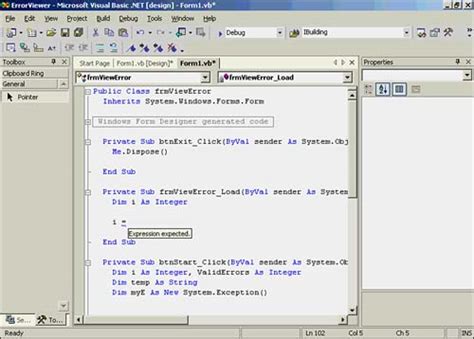
Take Action: Review your VBA code for potential type mismatches and take corrective action to prevent these errors. If you have experienced ByRef argument type mismatch errors, share your solutions in the comments below to help others learn from your experiences.