Intro
Discover how to rename files with LibreOffice Macro using 5 simple methods. Learn to automate file renaming tasks with LibreOffice Basic, using loops, arrays, and file system objects. Boost productivity and streamline file management with these easy-to-implement macro solutions, perfect for power users and developers.
Renaming files in bulk can be a daunting task, especially when dealing with a large number of files. LibreOffice, a popular open-source office suite, offers a powerful macro feature that can simplify this process. In this article, we will explore five ways to rename files using LibreOffice macros.
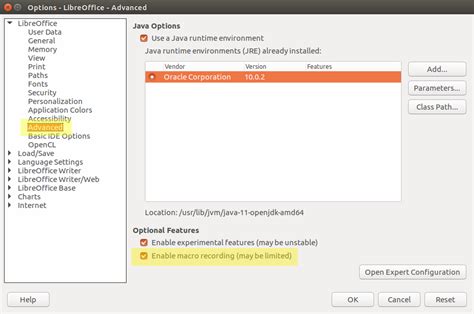
Why Use LibreOffice Macros for File Renaming?
Before we dive into the five methods, let's discuss why using LibreOffice macros for file renaming is a good idea. Macros are essentially automated scripts that can perform repetitive tasks, saving you time and effort. LibreOffice macros are particularly useful for file renaming because they can be customized to handle various file naming conventions, formats, and patterns.
Method 1: Using the FileDialog Object
The FileDialog object in LibreOffice allows you to interact with the file system, making it easy to rename files. Here's an example macro that demonstrates how to use the FileDialog object to rename files:
Sub RenameFiles()
Dim oFileDialog As Object
Dim oFile As Object
Dim sNewName As String
' Create a new FileDialog object
oFileDialog = CreateUnoService("com.sun.star.ui.dialogs.FilePicker")
' Set the dialog title and filter
oFileDialog.Title = "Select Files to Rename"
oFileDialog.Filter = "*.txt"
' Show the dialog
If oFileDialog.Execute() Then
' Get the selected file
oFile = oFileDialog.Files(0)
' Get the file name without extension
sNewName = Left(oFile.Name, Len(oFile.Name) - 4)
' Rename the file
oFile.Name = sNewName & "_renamed.txt"
End If
End Sub
Method 2: Using the Shell Object
The Shell object in LibreOffice allows you to execute system commands, including file renaming. Here's an example macro that demonstrates how to use the Shell object to rename files:
Sub RenameFiles()
Dim oShell As Object
Dim sCommand As String
Dim sFile As String
' Create a new Shell object
oShell = CreateUnoService("com.sun.star.system.SystemShellExecute")
' Set the file path and new name
sFile = "C:\path\to\file.txt"
sNewName = "file_renamed.txt"
' Construct the rename command
sCommand = "rename """ & sFile & """ """ & sNewName & """"
' Execute the command
oShell.Execute(sCommand)
End Sub
Method 3: Using Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching and string manipulation. LibreOffice macros support regex, making it easy to rename files based on complex patterns. Here's an example macro that demonstrates how to use regex to rename files:
Sub RenameFiles()
Dim oRegex As Object
Dim sPattern As String
Dim sReplace As String
Dim sFile As String
' Create a new regex object
oRegex = CreateUnoService("com.sun.star.util.RegExp")
' Set the pattern and replacement
sPattern = ".*\.(txt|docx)"
sReplace = "_renamed.$1"
' Set the file path
sFile = "C:\path\to\file.txt"
' Rename the file using regex
sFile = oRegex.Replace(sFile, sPattern, sReplace)
' Get the file object
Dim oFile As Object
oFile = CreateUnoService("com.sun.star.io.File")
oFile.Name = sFile
End Sub
Method 4: Using the Dir and FileSystemObject
The Dir and FileSystemObject in LibreOffice allow you to interact with the file system, making it easy to rename files. Here's an example macro that demonstrates how to use the Dir and FileSystemObject to rename files:
Sub RenameFiles()
Dim oFSO As Object
Dim sDir As String
Dim sFile As String
' Create a new FileSystemObject
oFSO = CreateUnoService("com.sun.star.script.provider ReadOnlyFileSystemObject")
' Set the directory path
sDir = "C:\path\to\directory"
' Get the first file in the directory
sFile = Dir(sDir & "\*.*")
' Loop through all files in the directory
While sFile <> ""
' Get the file object
Dim oFile As Object
oFile = oFSO.GetFile(sDir & "\" & sFile)
' Rename the file
oFile.Name = "file_renamed_" & Mid(sFile, InStrRev(sFile, ".") + 1)
' Get the next file
sFile = Dir()
Wend
End Sub
Method 5: Using the UnoScript
UnoScript is a scripting language used in LibreOffice to automate tasks. Here's an example macro that demonstrates how to use UnoScript to rename files:
Sub RenameFiles()
Dim oScript As Object
Dim sScript As String
' Create a new UnoScript object
oScript = CreateUnoService("com.sun.star.script.UnoScript")
' Set the script
sScript = "file = new File('C:\path\to\file.txt'); file.renameTo('file_renamed.txt');"
' Execute the script
oScript.Execute(sScript)
End Sub
Conclusion
In this article, we explored five ways to rename files using LibreOffice macros. Each method has its own strengths and weaknesses, and the choice of method depends on the specific requirements of your task. By using LibreOffice macros, you can automate file renaming tasks and save time and effort.
Gallery of LibreOffice Macro File Renaming
LibreOffice Macro File Renaming Image Gallery
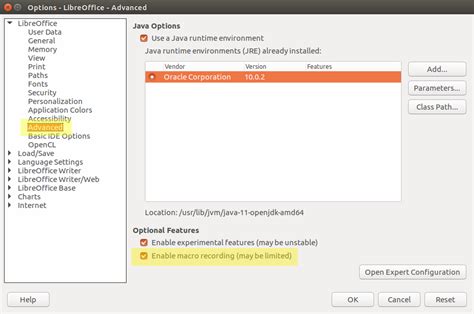
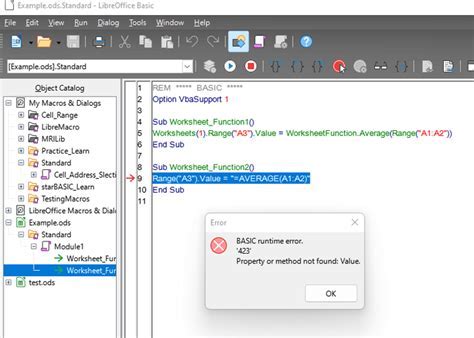
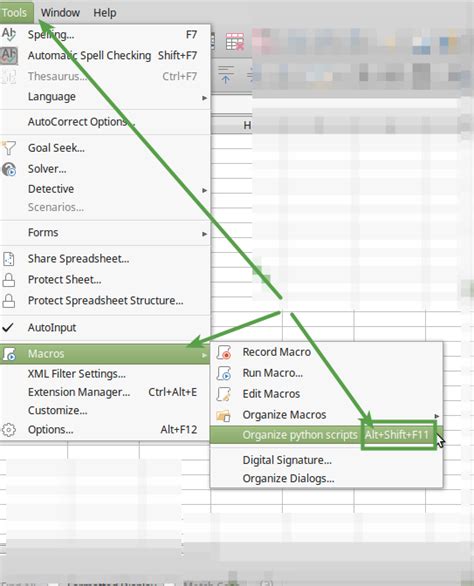
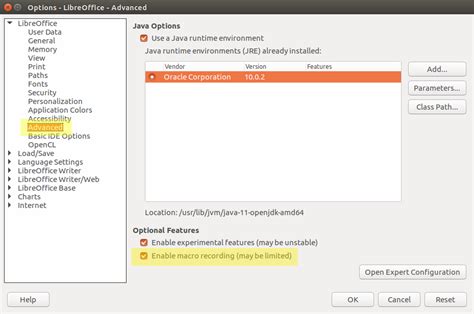
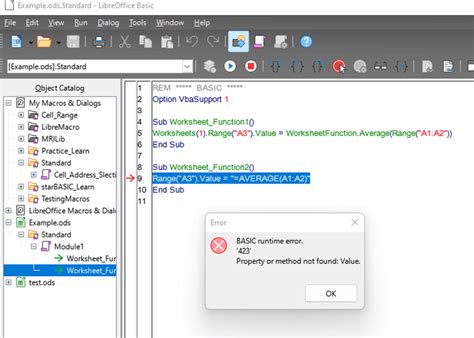
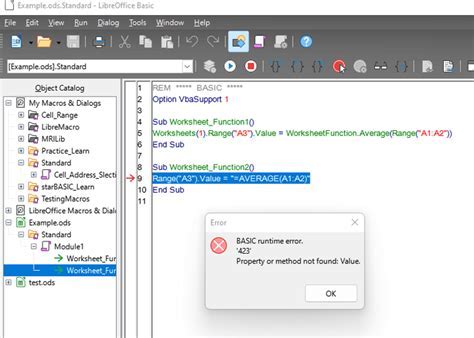
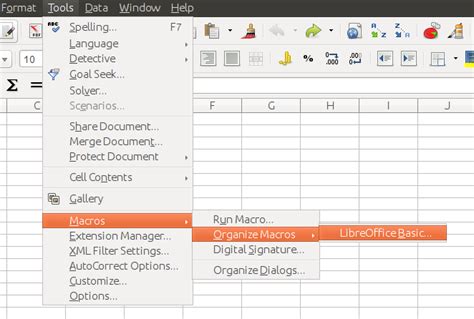
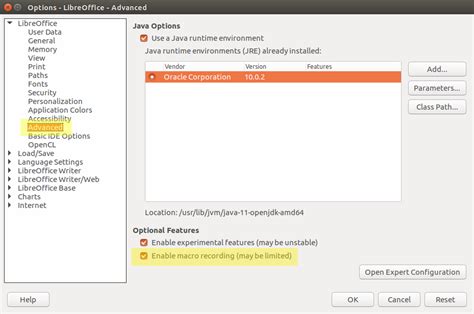
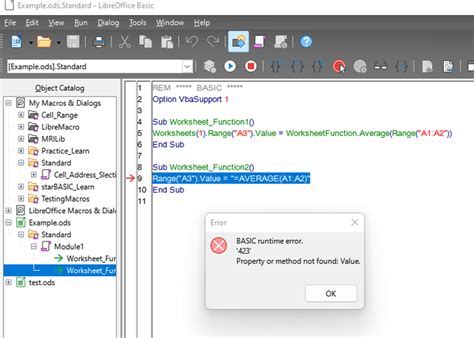
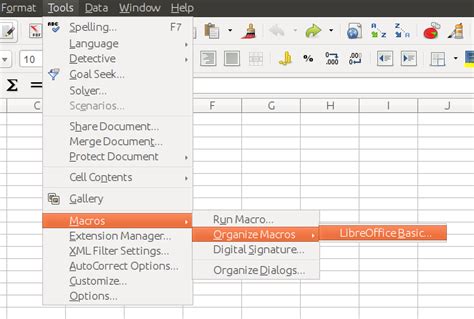
We hope this article has been helpful in demonstrating the power of LibreOffice macros for file renaming. If you have any questions or need further assistance, please don't hesitate to ask.