Intro
Master Excel VBA column width settings with 7 expert methods. Learn how to set column width in Excel VBA using Range, Columns, and Cells objects, as well as techniques for auto-fitting and resizing columns. Discover how to optimize your spreadsheets layout and improve readability with these actionable VBA code examples and tips.
When working with Excel, setting column widths is an essential task to ensure that your data is properly aligned and visible. While you can manually adjust column widths, using Excel VBA provides a more efficient and automated way to manage your spreadsheet's layout. In this article, we will explore 7 ways to set column width in Excel VBA, covering various methods and scenarios to help you master this skill.
Understanding Column Width in Excel VBA
Before diving into the methods, it's essential to understand how column width is measured in Excel VBA. Column width is measured in points, with one point equal to 1/72 of an inch. The ColumnWidth
property in VBA returns or sets the width of a column in points.
Method 1: Setting Column Width using the ColumnWidth
Property
One of the simplest ways to set column width in Excel VBA is by using the ColumnWidth
property.
Sub SetColumnWidth()
Range("A:A").ColumnWidth = 10
End Sub
In this example, the column width of column A is set to 10 points.
Method 2: Setting Column Width using the Range
Object
You can also use the Range
object to set column width. This method allows you to specify a range of columns to adjust.
Sub SetColumnWidthRange()
Range("A:C").ColumnWidth = 12
End Sub
In this example, the column width of columns A, B, and C is set to 12 points.
Method 3: Setting Column Width using the Cells
Object
Another way to set column width is by using the Cells
object. This method allows you to specify a specific cell or range of cells to adjust.
Sub SetColumnWidthCells()
Cells(1, 1).ColumnWidth = 15
End Sub
In this example, the column width of column A (column 1) is set to 15 points.
Method 4: Setting Column Width using the Columns
Object
You can also use the Columns
object to set column width. This method allows you to specify a range of columns to adjust.
Sub SetColumnWidthColumns()
Columns("A:C").ColumnWidth = 18
End Sub
In this example, the column width of columns A, B, and C is set to 18 points.
Method 5: Setting Column Width using a Loop
If you need to set column width for multiple columns, you can use a loop to iterate through the columns.
Sub SetColumnWidthLoop()
Dim i As Integer
For i = 1 To 10
Columns(i).ColumnWidth = 10
Next i
End Sub
In this example, the column width of columns 1 through 10 is set to 10 points.
Method 6: Setting Column Width using the AutoFit
Method
Another way to set column width is by using the AutoFit
method. This method automatically adjusts the column width to fit the contents of the cells.
Sub SetColumnWidthAutoFit()
Range("A:A").AutoFit
End Sub
In this example, the column width of column A is automatically adjusted to fit the contents of the cells.
Method 7: Setting Column Width using the AutoFit
Method with a Range
You can also use the AutoFit
method with a range of columns to adjust.
Sub SetColumnWidthAutoFitRange()
Range("A:C").AutoFit
End Sub
In this example, the column width of columns A, B, and C is automatically adjusted to fit the contents of the cells.
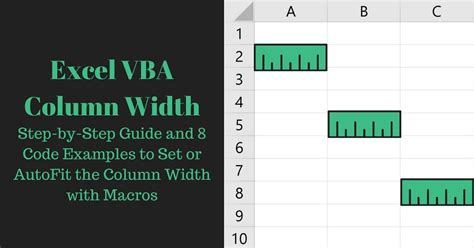
Gallery of Excel VBA Column Width Images
Excel VBA Column Width Image Gallery
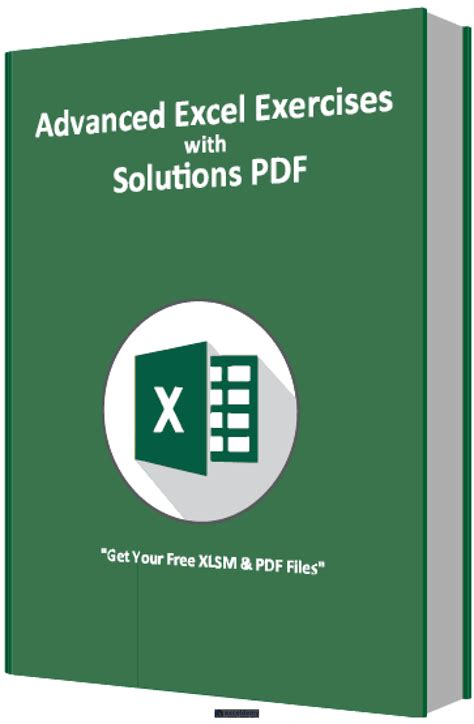
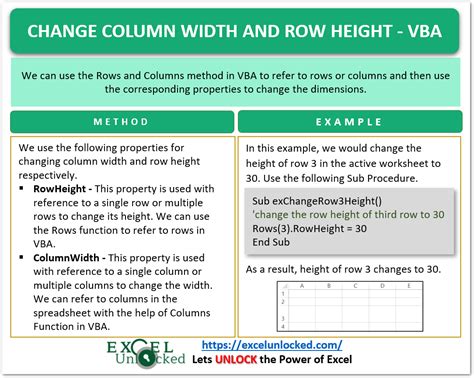
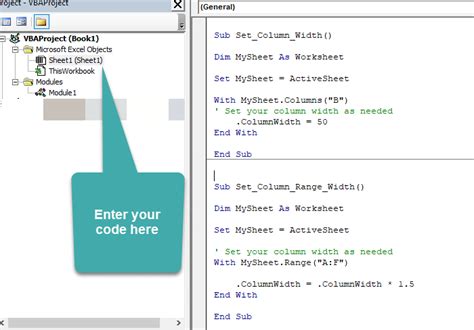
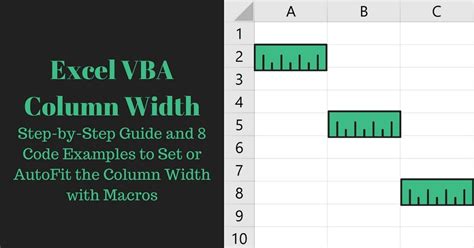
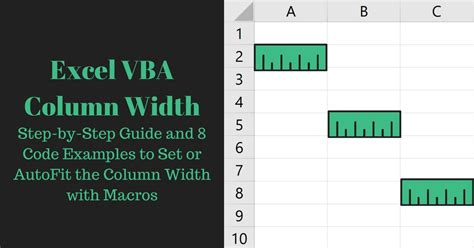
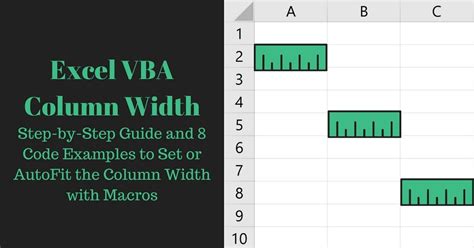
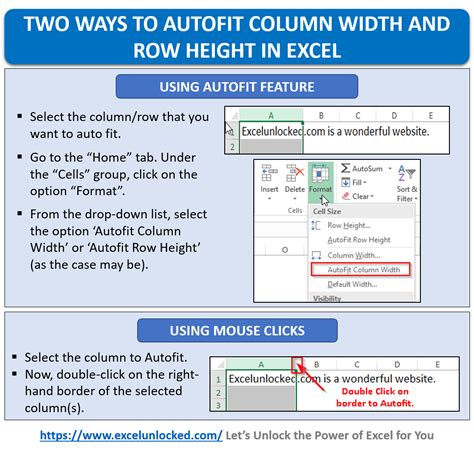
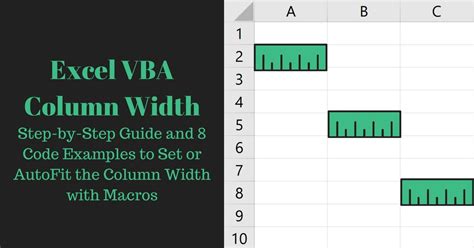
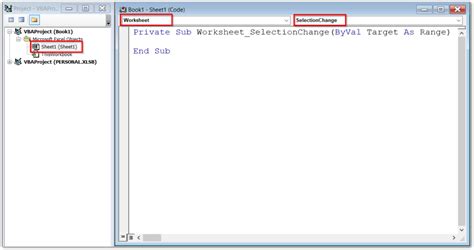
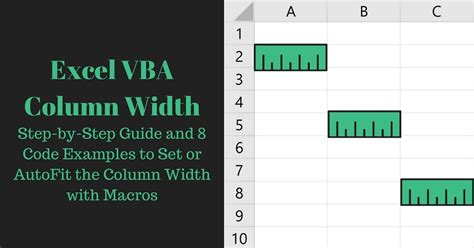
Frequently Asked Questions
-
How do I set column width in Excel VBA? There are several ways to set column width in Excel VBA, including using the
ColumnWidth
property, theRange
object, theCells
object, theColumns
object, a loop, and theAutoFit
method. -
What is the difference between the
ColumnWidth
property and theAutoFit
method? TheColumnWidth
property sets the column width to a specific value, while theAutoFit
method automatically adjusts the column width to fit the contents of the cells. -
Can I set column width for multiple columns at once? Yes, you can set column width for multiple columns at once using the
Range
object, theColumns
object, or a loop. -
How do I set column width using a loop? You can set column width using a loop by iterating through the columns and setting the column width for each column individually.
-
What is the
AutoFit
method? TheAutoFit
method automatically adjusts the column width to fit the contents of the cells.
Conclusion
Setting column width in Excel VBA is an essential task to ensure that your data is properly aligned and visible. In this article, we explored 7 ways to set column width in Excel VBA, including using the ColumnWidth
property, the Range
object, the Cells
object, the Columns
object, a loop, and the AutoFit
method. By mastering these methods, you can efficiently manage your spreadsheet's layout and ensure that your data is presented in a clear and concise manner.
We hope this article has been informative and helpful in your Excel VBA journey. If you have any further questions or would like to explore more topics, please feel free to ask in the comments below.