Intro
Master string concatenation in Excel VBA with ease. Learn how to combine text strings, numbers, and variables using various methods, including the & operator, CONCATENATE function, and JOIN function. Discover how to handle errors, format text, and optimize performance. Take your Excel VBA skills to the next level with this comprehensive guide on concatenating strings.
Concatenating strings in Excel VBA is a fundamental skill that can be used in a wide range of applications, from simple data manipulation to complex program development. In this article, we will explore the various methods of concatenating strings in Excel VBA, including the use of the &
operator, the Concatenate
function, and the Join
function.
Why Concatenate Strings in Excel VBA?
Concatenating strings is a common task in Excel VBA, as it allows you to combine text from different sources into a single string. This can be useful in a variety of scenarios, such as:
- Creating a full name by combining a first name and last name
- Building a file path by combining a directory and file name
- Creating a formatted string by combining text and variables
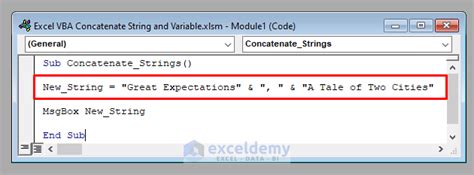
Method 1: Using the &
Operator
The &
operator is the most common method of concatenating strings in Excel VBA. This operator is used to combine two or more strings into a single string.
Dim firstName As String
Dim lastName As String
Dim fullName As String
firstName = "John"
lastName = "Doe"
fullName = firstName & " " & lastName
Debug.Print fullName ' Output: John Doe
Method 2: Using the Concatenate
Function
The Concatenate
function is a built-in Excel function that can be used to concatenate strings. This function is available in the Visual Basic Editor, but it is not as commonly used as the &
operator.
Dim firstName As String
Dim lastName As String
Dim fullName As String
firstName = "John"
lastName = "Doe"
fullName = Application.WorksheetFunction.Concatenate(firstName, " ", lastName)
Debug.Print fullName ' Output: John Doe
Method 3: Using the Join
Function
The Join
function is a built-in Visual Basic function that can be used to concatenate an array of strings into a single string. This function is commonly used when working with arrays or collections of strings.
Dim names() As String
Dim fullName As String
names = Array("John", "Doe")
fullName = Join(names, " ")
Debug.Print fullName ' Output: John Doe
Best Practices for Concatenating Strings in Excel VBA
When concatenating strings in Excel VBA, there are several best practices to keep in mind:
- Use the
&
operator: The&
operator is the most common and efficient method of concatenating strings in Excel VBA. - Use variables: Instead of concatenating strings directly in your code, use variables to store the individual strings and then concatenate them.
- Avoid using the
Concatenate
function: While theConcatenate
function is available, it is not as commonly used as the&
operator and can make your code more difficult to read. - Use the
Join
function with arrays: When working with arrays or collections of strings, use theJoin
function to concatenate them into a single string.
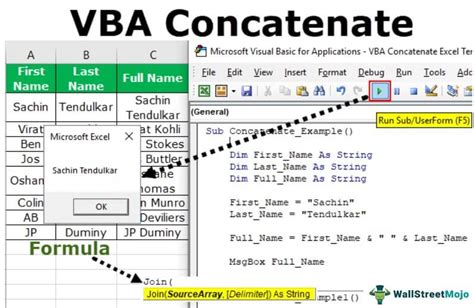
Common Errors When Concatenating Strings in Excel VBA
When concatenating strings in Excel VBA, there are several common errors to watch out for:
- Forgetting to declare variables: Make sure to declare all variables before using them in your code.
- Using the wrong operator: Make sure to use the
&
operator instead of the+
operator, which is used for arithmetic operations. - Not using quotes: Make sure to use quotes around text strings, especially when concatenating strings with variables.
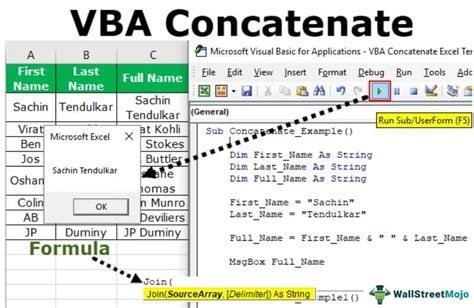
Conclusion
Concatenating strings in Excel VBA is a fundamental skill that can be used in a wide range of applications. By using the &
operator, variables, and best practices, you can make your code more efficient and easier to read. Remember to avoid common errors, such as forgetting to declare variables and using the wrong operator. With practice and experience, you can become proficient in concatenating strings in Excel VBA.
We hope this article has been helpful in explaining how to concatenate strings in Excel VBA. If you have any questions or need further clarification, please leave a comment below.
Excel VBA Concatenate Strings Image Gallery
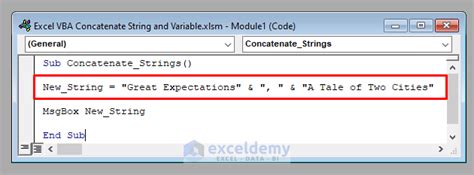
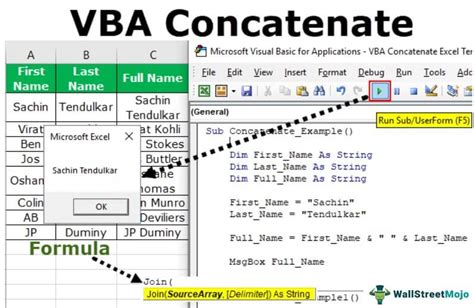
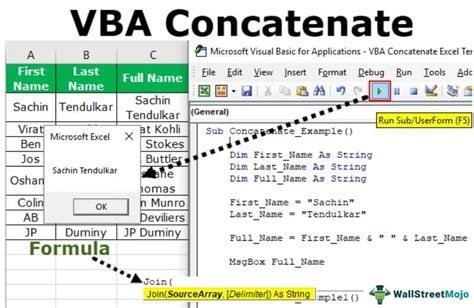
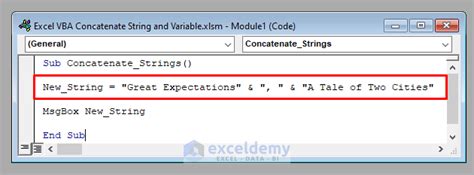
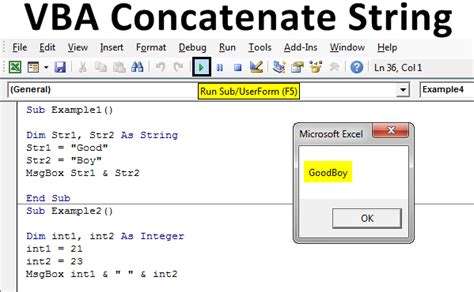
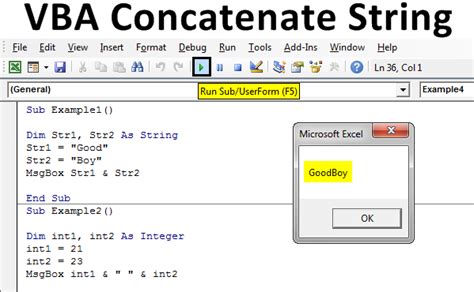
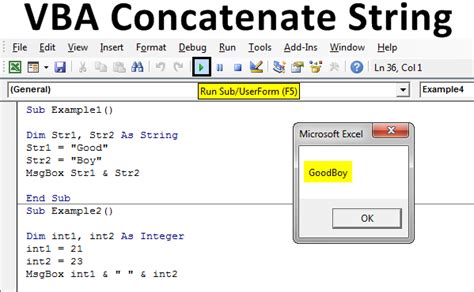
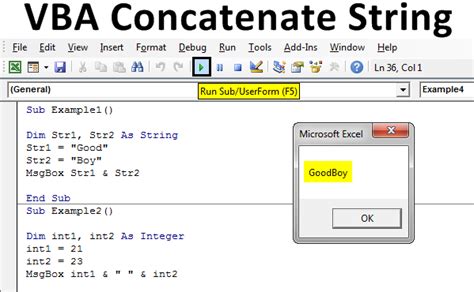
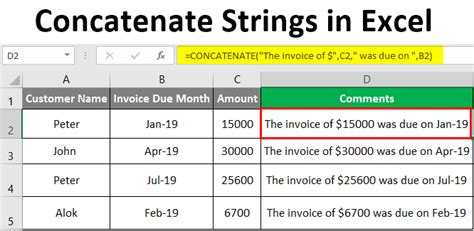
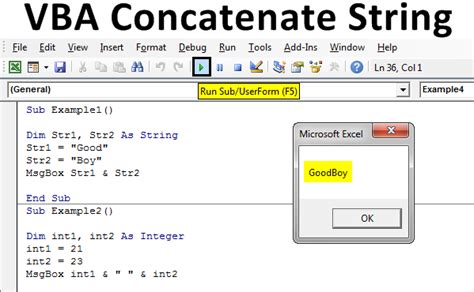