Intro
Master VBA loops with continue statements. Learn 5 practical ways to use Continue in VBA For Loops to improve code efficiency, skip iterations, and handle errors. Discover how to leverage Resume Next, Exit For, and conditional statements to refine your loop control and write more robust VBA macros.
Loops are a fundamental part of any programming language, and VBA (Visual Basic for Applications) is no exception. VBA's Continue statement is a powerful tool that allows you to control the flow of your loops, skipping over certain iterations or sections of code when needed. In this article, we'll explore five ways to use the Continue statement in VBA For loops, along with practical examples and explanations.
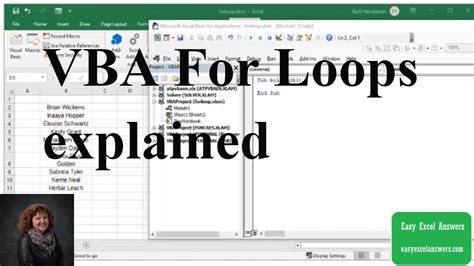
Understanding the Continue Statement
Before diving into the examples, let's quickly review what the Continue statement does in VBA. The Continue statement skips the rest of the code inside a loop and moves on to the next iteration. It's often used to avoid executing certain code blocks when a specific condition is met.
Example 1: Skipping Iterations
One common use of the Continue statement is to skip over certain iterations in a loop. Suppose you're looping through a range of cells, and you want to skip the cells that contain a specific value.
Sub SkipIterations()
Dim i As Long
For i = 1 To 10
If Cells(i, 1).Value = "Skip" Then
Continue For
End If
' Code to execute for non-skipped cells
Debug.Print Cells(i, 1).Value
Next i
End Sub
In this example, the Continue statement skips the iterations where the cell value is "Skip".
Example 2: Handling Errors
Another use of the Continue statement is to handle errors in a loop. You can use it to skip over the code that causes the error and move on to the next iteration.
Sub HandleErrors()
Dim i As Long
On Error Resume Next
For i = 1 To 10
' Code that might raise an error
On Error GoTo 0
If Err.Number <> 0 Then
Continue For
End If
' Code to execute for non-error iterations
Debug.Print Cells(i, 1).Value
Next i
End Sub
In this example, the Continue statement skips the iterations where an error occurs.
Example 3: Conditional Execution
You can also use the Continue statement to conditionally execute code inside a loop. Suppose you want to execute a block of code only when a certain condition is met.
Sub ConditionalExecution()
Dim i As Long
For i = 1 To 10
If Cells(i, 1).Value = "Execute" Then
' Code to execute for the "Execute" condition
Debug.Print Cells(i, 1).Value
Else
Continue For
End If
Next i
End Sub
In this example, the Continue statement skips the iterations where the condition is not met.
Example 4: Looping Through Arrays
When looping through arrays, you can use the Continue statement to skip over certain elements.
Sub LoopingThroughArrays()
Dim arr() As Variant
arr = Array("Apple", "Banana", "Cherry", "Date")
Dim i As Long
For i = LBound(arr) To UBound(arr)
If arr(i) = "Banana" Then
Continue For
End If
' Code to execute for non-skipped elements
Debug.Print arr(i)
Next i
End Sub
In this example, the Continue statement skips the element "Banana".
Example 5: Nested Loops
Finally, you can use the Continue statement in nested loops to skip over certain iterations in the inner loop.
Sub NestedLoops()
Dim i As Long
Dim j As Long
For i = 1 To 5
For j = 1 To 5
If j = 3 Then
Continue For
End If
' Code to execute for non-skipped iterations
Debug.Print i & "," & j
Next j
Next i
End Sub
In this example, the Continue statement skips the iterations where j = 3
.
VBA Loops Gallery
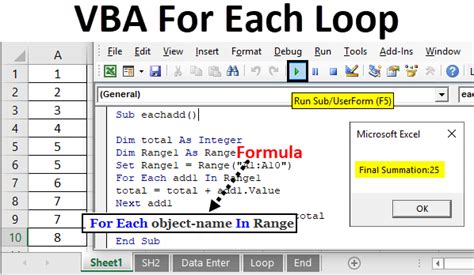
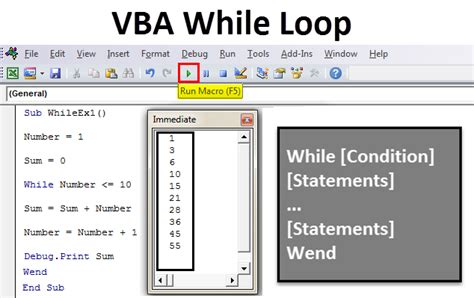
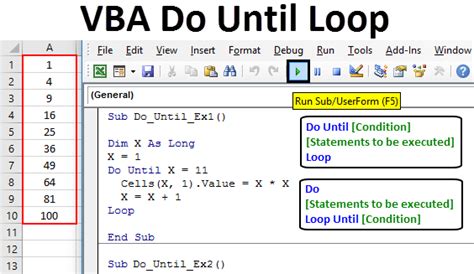
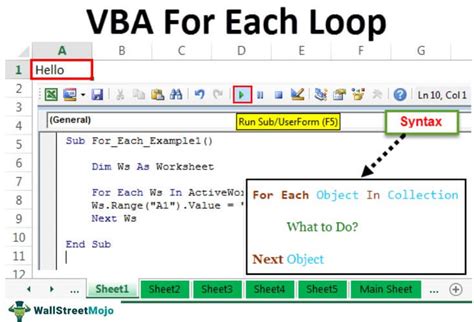
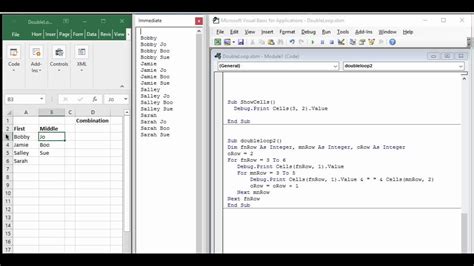
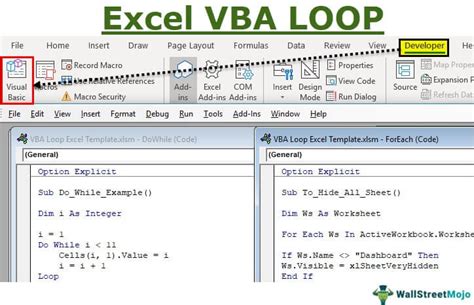
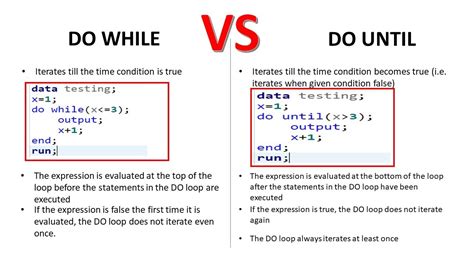
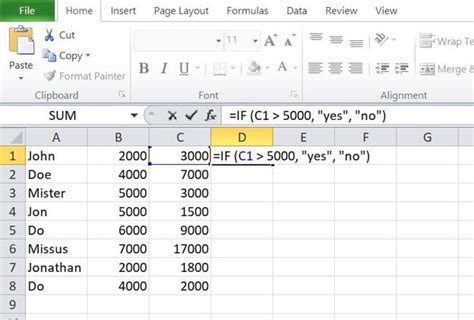
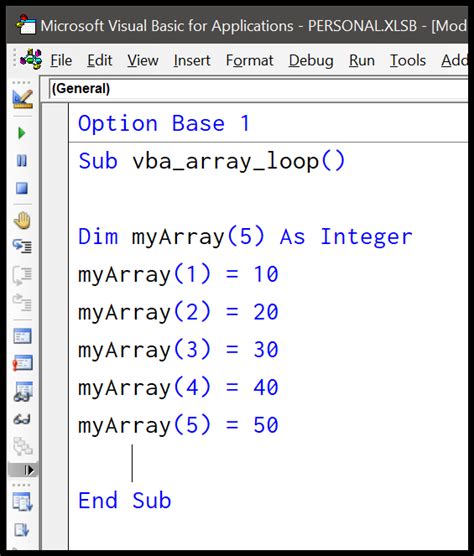
In conclusion, the Continue statement is a powerful tool in VBA that allows you to control the flow of your loops. By using it judiciously, you can skip over certain iterations, handle errors, and conditionally execute code. We hope this article has provided you with a deeper understanding of how to use the Continue statement in VBA For loops.