Intro
Learn 3 efficient ways to convert string to integer in VBA, including using the Val, CInt, and CLng functions. Discover how to handle errors and optimize your code for data type conversion. Master VBA data manipulation with expert tips and examples, and streamline your workflow with improved coding skills.
Converting strings to integers is a fundamental operation in programming, and Visual Basic for Applications (VBA) is no exception. In VBA, you may encounter situations where you need to convert a string to an integer, such as when reading data from a text file or user input. There are several ways to achieve this conversion, and in this article, we will explore three methods.
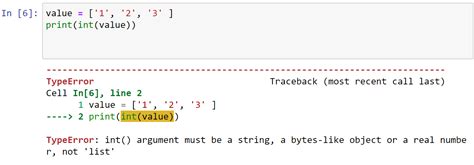
Method 1: Using the CInt() Function
The CInt() function is a built-in VBA function that converts a string to an integer. This function is the most straightforward way to convert a string to an integer. Here's an example:
Sub ConvertStringToInt()
Dim str As String
Dim int As Integer
str = "123"
int = CInt(str)
MsgBox int
End Sub
In this example, the CInt() function converts the string "123" to an integer, which is then stored in the int
variable.
Method 2: Using the CLng() Function and Type Casting
Another way to convert a string to an integer is by using the CLng() function, which converts a string to a long integer. You can then use type casting to convert the long integer to an integer. Here's an example:
Sub ConvertStringToInt()
Dim str As String
Dim lng As Long
Dim int As Integer
str = "123"
lng = CLng(str)
int = CInt(lng)
MsgBox int
End Sub
In this example, the CLng() function converts the string "123" to a long integer, which is then stored in the lng
variable. The CInt() function is then used to convert the long integer to an integer, which is stored in the int
variable.
Method 3: Using the Val() Function
The Val() function is another built-in VBA function that converts a string to a numeric value. This function can be used to convert a string to an integer. Here's an example:
Sub ConvertStringToInt()
Dim str As String
Dim int As Integer
str = "123"
int = Val(str)
MsgBox int
End Sub
In this example, the Val() function converts the string "123" to a numeric value, which is then stored in the int
variable.
Comparison of Methods
Each of the three methods has its own strengths and weaknesses. Here's a comparison of the methods:
- CInt() Function: This method is the most straightforward and efficient way to convert a string to an integer. However, it may throw an error if the string cannot be converted to an integer.
- CLng() Function and Type Casting: This method is more robust than the CInt() function, as it can handle larger numbers. However, it requires an extra step to convert the long integer to an integer.
- Val() Function: This method is more flexible than the CInt() function, as it can convert a string to a numeric value. However, it may return a decimal value if the string contains decimal points.
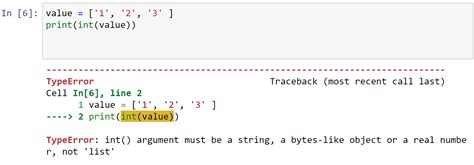
Best Practices
When converting strings to integers in VBA, it's essential to follow best practices to avoid errors and ensure data integrity. Here are some best practices:
- Validate User Input: Always validate user input to ensure that it can be converted to an integer. You can use the IsNumeric() function to check if a string can be converted to a number.
- Use Error Handling: Use error handling to catch and handle errors that may occur during the conversion process. You can use the On Error statement to catch and handle errors.
- Test Your Code: Test your code thoroughly to ensure that it works correctly and handles all possible scenarios.
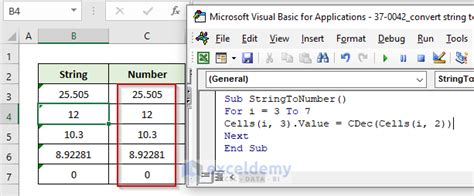
Conclusion
In this article, we've explored three ways to convert strings to integers in VBA. Each method has its own strengths and weaknesses, and the best method to use depends on the specific requirements of your project. By following best practices and using error handling, you can ensure that your code is robust and reliable.
Gallery of VBA String to Integer Conversion
VBA String to Integer Conversion Image Gallery
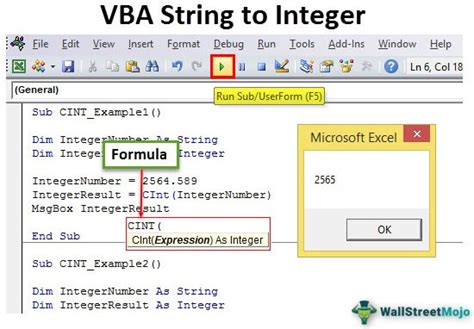
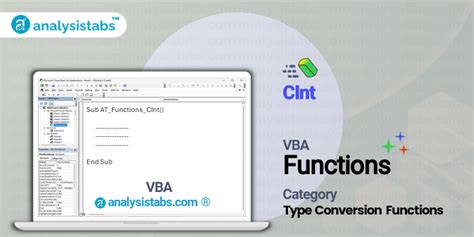
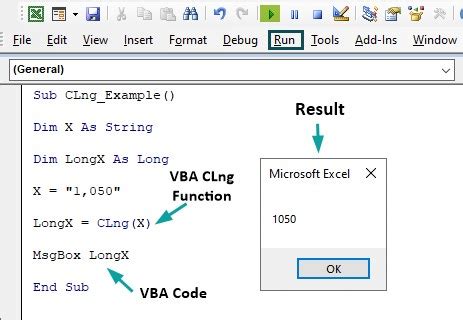
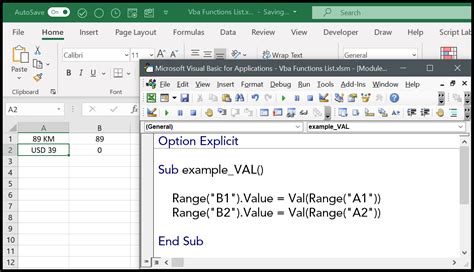
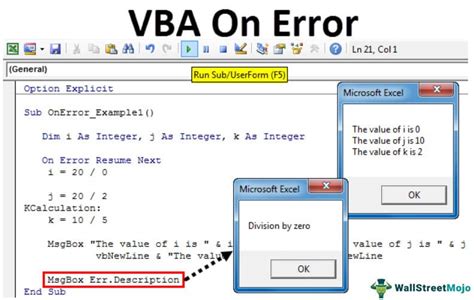
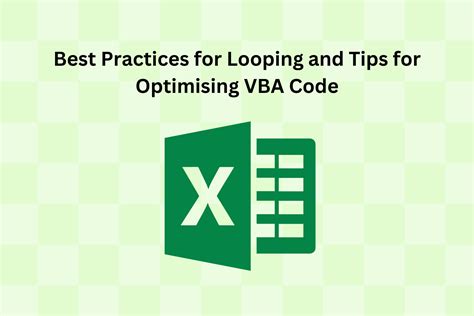
FAQs
Q: What is the best method to convert a string to an integer in VBA? A: The best method to use depends on the specific requirements of your project. The CInt() function is the most straightforward method, but it may throw an error if the string cannot be converted to an integer. The CLng() function and type casting is a more robust method, but it requires an extra step to convert the long integer to an integer.
Q: How do I validate user input to ensure that it can be converted to an integer? A: You can use the IsNumeric() function to check if a string can be converted to a number.
Q: What is the difference between the CInt() and CLng() functions? A: The CInt() function converts a string to an integer, while the CLng() function converts a string to a long integer.
Q: How do I use error handling to catch and handle errors during the conversion process? A: You can use the On Error statement to catch and handle errors.
We hope this article has helped you learn how to convert strings to integers in VBA. If you have any questions or need further assistance, please don't hesitate to ask.