Intro
Effortlessly convert strings to integers in VBA with our expert guide. Learn the most efficient methods to parse and convert string values to numerical integers in Visual Basic for Applications, including handling errors and edge cases, and discover how to improve your VBA coding skills with best practices and troubleshooting tips.
Working with strings and integers is a common task in VBA programming. Sometimes, you may need to convert a string to an integer, and this can be a bit tricky. In this article, we'll explore the easiest ways to convert a string to an integer in VBA.
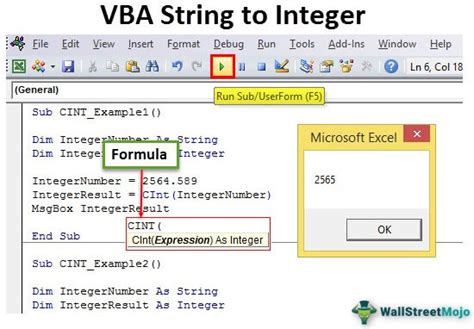
Why Convert a String to an Integer?
Before we dive into the methods, let's understand why you might need to convert a string to an integer. In VBA, strings and integers are two different data types, and you can't perform mathematical operations on strings. If you have a string that represents a number, you'll need to convert it to an integer to use it in calculations.
Method 1: Using the CInt Function
The easiest way to convert a string to an integer in VBA is to use the CInt function. This function takes a string as an argument and returns an integer value.
Here's an example:
Dim str As String
Dim int As Integer
str = "123"
int = CInt(str)
Debug.Print int ' Outputs: 123
Note that the CInt function will round the value to the nearest integer. If the string contains a decimal value, it will be truncated.
Method 2: Using the CLng Function
Another way to convert a string to an integer is to use the CLng function. This function is similar to CInt, but it returns a Long data type instead of an Integer.
Here's an example:
Dim str As String
Dim lng As Long
str = "1234567890"
lng = CLng(str)
Debug.Print lng ' Outputs: 1234567890
Keep in mind that the CLng function will also round the value to the nearest integer.
Method 3: Using the Val Function
The Val function is another way to convert a string to a number. It returns a Variant data type, which can be used as an integer.
Here's an example:
Dim str As String
Dim num As Variant
str = "123.45"
num = Val(str)
Debug.Print num ' Outputs: 123.45
Note that the Val function will return a decimal value if the string contains a decimal point.
Handling Errors
When converting a string to an integer, you may encounter errors if the string is not a valid number. To handle these errors, you can use the On Error statement or the IsNumeric function.
Here's an example:
Dim str As String
Dim int As Integer
str = "abc"
On Error Resume Next
int = CInt(str)
If Err.Number <> 0 Then
Debug.Print "Error: " & Err.Description
End If
Alternatively, you can use the IsNumeric function to check if the string is a valid number before attempting to convert it.
Here's an example:
Dim str As String
Dim int As Integer
str = "123"
If IsNumeric(str) Then
int = CInt(str)
Debug.Print int ' Outputs: 123
Else
Debug.Print "Error: Not a valid number"
End If
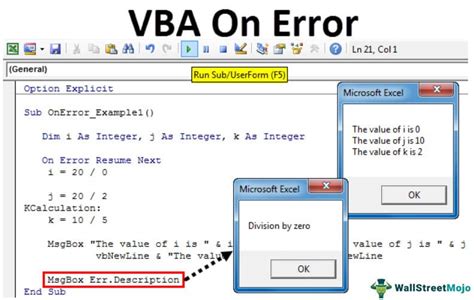
Best Practices
When converting strings to integers in VBA, keep the following best practices in mind:
- Always check if the string is a valid number before attempting to convert it.
- Use the On Error statement or the IsNumeric function to handle errors.
- Use the CInt or CLng function to convert strings to integers.
- Avoid using the Val function unless you need to work with decimal values.
By following these best practices and using the methods outlined in this article, you'll be able to easily convert strings to integers in VBA.
String to Integer Conversion Gallery
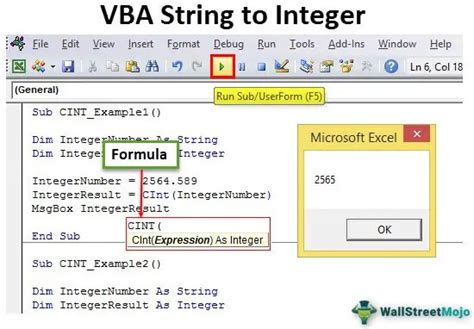
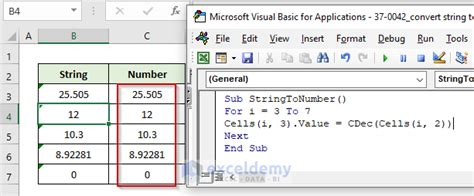
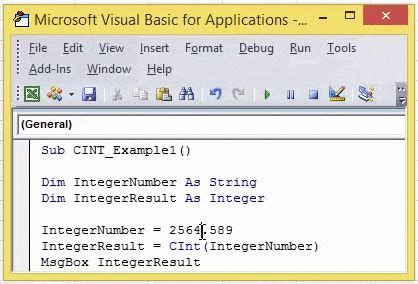
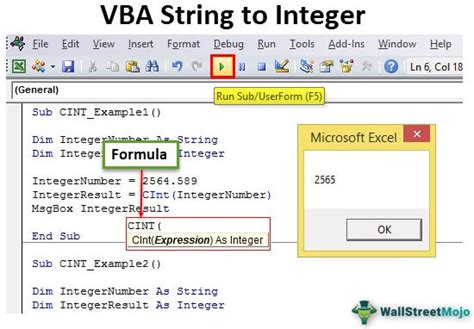
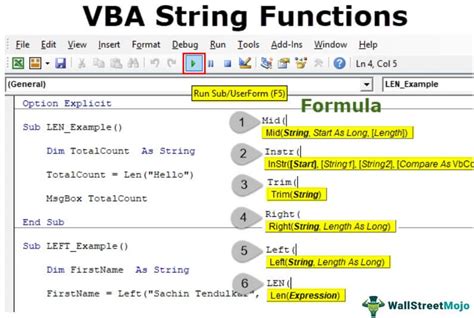
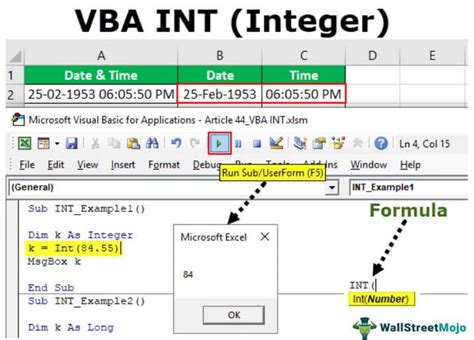
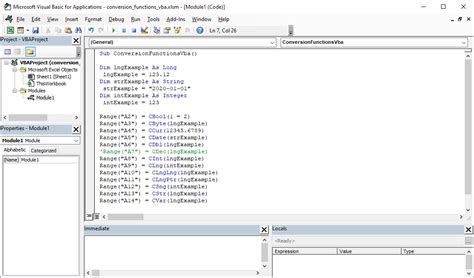
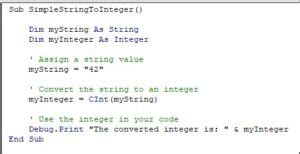
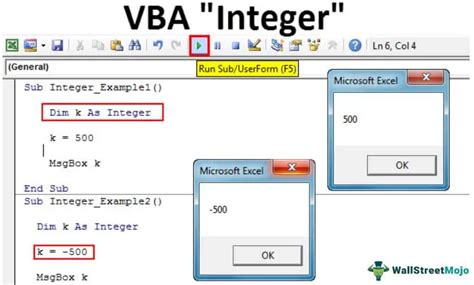
We hope this article has helped you learn how to convert strings to integers in VBA. If you have any questions or need further assistance, please don't hesitate to ask.