Copying rows from one cell to another in Google Sheets can greatly enhance your productivity and efficiency when managing and organizing data. This process can be especially useful for tasks such as creating backups of data, moving specific rows to another sheet for further analysis, or archiving completed tasks. Google Sheets provides several methods to achieve this, including using the user interface, formulas, and scripts. In this article, we'll delve into the script method, exploring how to create a custom script to copy rows to another cell or sheet in Google Sheets.
Why Use a Script?
While you can manually copy and paste rows or use drag-and-drop methods, a script offers more precision and automation, especially when dealing with large datasets or repetitive tasks. Scripts can also be triggered by specific events, such as changes to the sheet, making them incredibly powerful for automating tasks.
Setting Up Your Google Sheet for Scripting
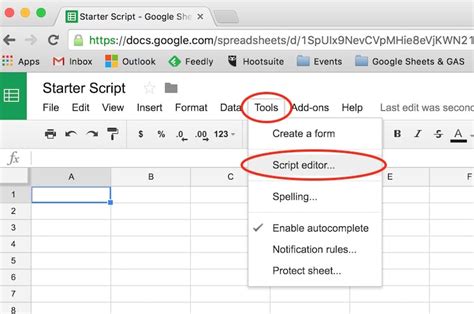
Before you start writing your script, ensure you have a Google Sheet set up with the data you wish to copy. For demonstration purposes, let's assume you have a sheet named "Source" and you want to copy certain rows to another sheet named "Target".
Accessing the Script Editor
- Open your Google Sheet.
- Click on
Extensions
>Apps Script
. This opens the Google Apps Script editor.
Writing the Script to Copy Rows
function copyRows() {
var ss = SpreadsheetApp.getActiveSpreadsheet();
var sourceSheet = ss.getSheetByName("Source");
var targetSheet = ss.getSheetByName("Target");
// Define the range you want to copy
var range = sourceSheet.getDataRange();
var values = range.getValues();
// Loop through the values to select specific rows
var rowsToCopy = [];
for (var i = 0; i < values.length; i++) {
// Condition to select rows, e.g., rows where column 1 is not empty
if (values[i][0]!= "") {
rowsToCopy.push(values[i]);
}
}
// Copy the selected rows to the target sheet
targetSheet.getRange(targetSheet.getLastRow() + 1, 1, rowsToCopy.length, rowsToCopy[0].length).setValues(rowsToCopy);
}
This script copies rows from the "Source" sheet to the "Target" sheet based on a simple condition (in this case, rows where the first column is not empty). You can modify the condition inside the if
statement to fit your specific needs.
Running the Script
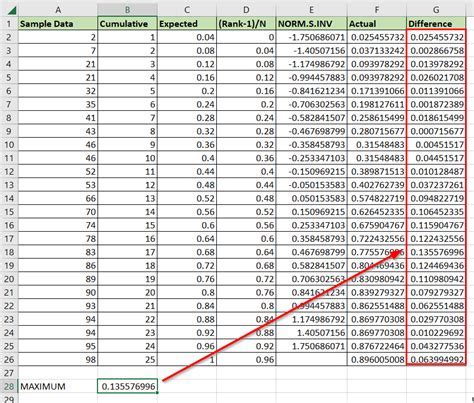
- Save the script by clicking on the floppy disk icon or pressing
Ctrl+S
(orCmd+S
on a Mac). - Click on the
Run
button (a "play" icon) or pressCtrl+Enter
to run thecopyRows
function. If prompted, authorize the script. - You might need to review permissions to allow the script to access your sheet.
Scheduling the Script
For scripts that need to run automatically at certain times or intervals, you can use Google Apps Script's trigger feature.
- In the Apps Script editor, click on the
Triggers
button on the left sidebar. - Click on the
Create trigger
button. - Set up the trigger with your desired time or event.
Gallery of Google Sheets Scripting
Google Sheets Scripting Gallery
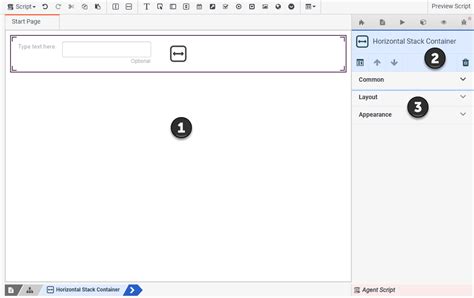
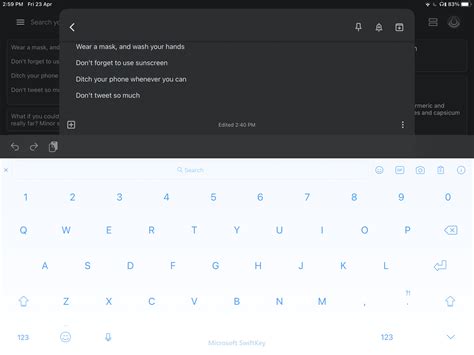
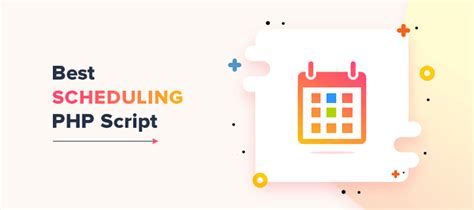
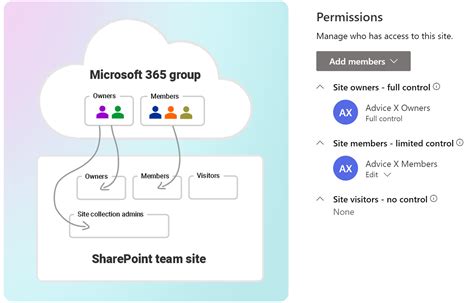
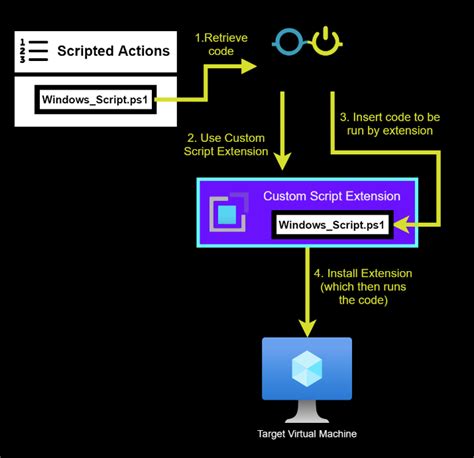
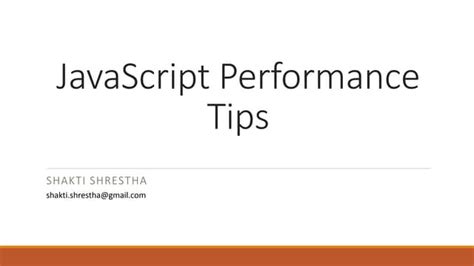
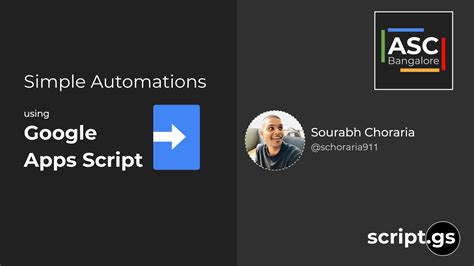
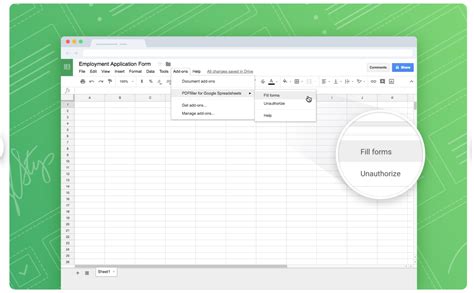
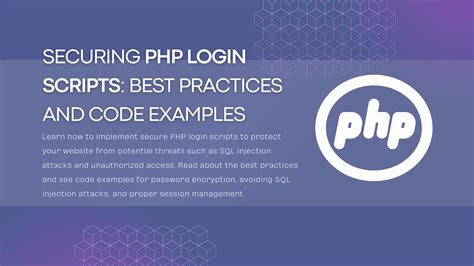
Engage with the Community
If you found this article helpful in understanding how to copy rows to another cell or sheet in Google Sheets using a script, we encourage you to share your experiences, ask questions, or request further clarification in the comments below. Your engagement helps build a community of Google Sheets enthusiasts and scripters, pushing the boundaries of what's possible in spreadsheet management and automation.