Intro
Learn how to create folders with VBA in Excel made easy. Master the art of automating folder creation with Visual Basic for Applications. Discover how to use VBA macros to create folders, subfolders, and file paths with ease. Boost your productivity and simplify file management with Excel VBA folder creation techniques.
Creating a folder with VBA in Excel is a simple process that can be achieved through a few lines of code. In this article, we will guide you through the steps to create a folder using VBA in Excel, along with some practical examples and explanations.
The Importance of Creating Folders with VBA
In many cases, Excel users need to create folders to organize their files and data. This can be particularly useful when working on projects that involve multiple files and folders. By creating folders with VBA, you can automate the process and save time. Additionally, VBA allows you to create folders with specific names and paths, making it easier to manage your files.
Getting Started with VBA
Before we dive into the code, make sure you have the Developer tab enabled in your Excel ribbon. To do this, go to File > Options > Customize Ribbon, and check the box next to "Developer". This will give you access to the Visual Basic Editor, where you can write and execute VBA code.
The VBA Code to Create a Folder
The VBA code to create a folder is relatively simple. You can use the following code as a starting point:
Sub CreateFolder()
Dim folderPath As String
folderPath = "C:\Path\To\Folder"
If Dir(folderPath, vbDirectory) = "" Then
MkDir folderPath
MsgBox "Folder created successfully!"
Else
MsgBox "Folder already exists!"
End If
End Sub
Let's break down the code:
Dim folderPath As String
: This line declares a variablefolderPath
to store the path of the folder you want to create.folderPath = "C:\Path\To\Folder"
: This line assigns the path of the folder to thefolderPath
variable. Make sure to replaceC:\Path\To\Folder
with the actual path you want to use.If Dir(folderPath, vbDirectory) = "" Then
: This line checks if the folder already exists. If it doesn't, the code inside theIf
statement will be executed.MkDir folderPath
: This line creates the folder using theMkDir
function.MsgBox "Folder created successfully!"
: This line displays a message box indicating that the folder has been created successfully.Else
: This line is executed if the folder already exists. In this case, a message box will be displayed indicating that the folder already exists.
Creating Folders with Specific Names and Paths
You can modify the code to create folders with specific names and paths. For example, you can use the following code to create a folder with a specific name and path:
Sub CreateFolderWithNameAndPath()
Dim folderName As String
Dim folderPath As String
folderName = "MyFolder"
folderPath = "C:\Path\To\" & folderName
If Dir(folderPath, vbDirectory) = "" Then
MkDir folderPath
MsgBox "Folder created successfully!"
Else
MsgBox "Folder already exists!"
End If
End Sub
In this example, the code creates a folder named "MyFolder" in the specified path.
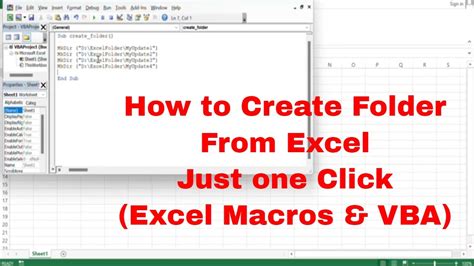
Creating Multiple Folders with VBA
You can also create multiple folders using VBA. One way to do this is to use a loop to iterate through an array of folder names and paths. Here's an example:
Sub CreateMultipleFolders()
Dim folderNames As Variant
Dim folderPaths As Variant
folderNames = Array("Folder1", "Folder2", "Folder3")
folderPaths = Array("C:\Path\To\Folder1", "C:\Path\To\Folder2", "C:\Path\To\Folder3")
For i = LBound(folderNames) To UBound(folderNames)
If Dir(folderPaths(i), vbDirectory) = "" Then
MkDir folderPaths(i)
MsgBox "Folder created successfully!"
Else
MsgBox "Folder already exists!"
End If
Next i
End Sub
In this example, the code creates three folders with specific names and paths.
Tips and Tricks
Here are some tips and tricks to keep in mind when creating folders with VBA:
- Make sure to use the correct path and folder name.
- Use the
Dir
function to check if the folder already exists. - Use the
MkDir
function to create the folder. - Use a loop to create multiple folders.
- Use message boxes to display feedback to the user.
Gallery of Create Folder with VBA in Excel
Create Folder with VBA in Excel Image Gallery
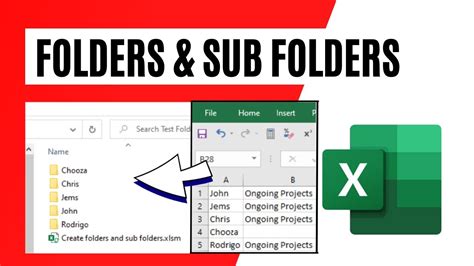
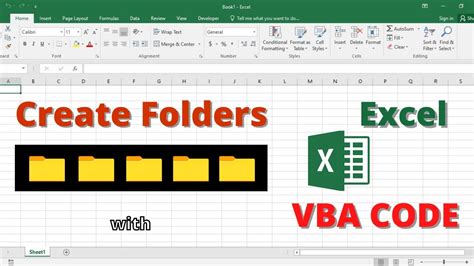
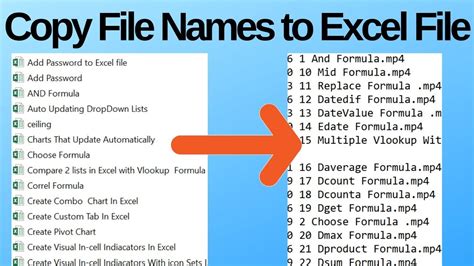
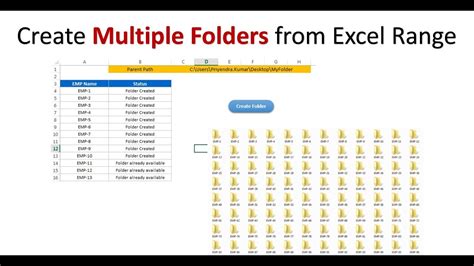
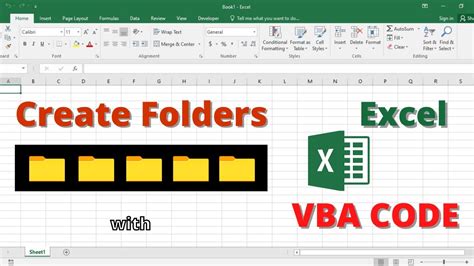
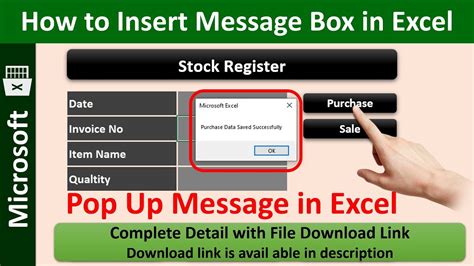
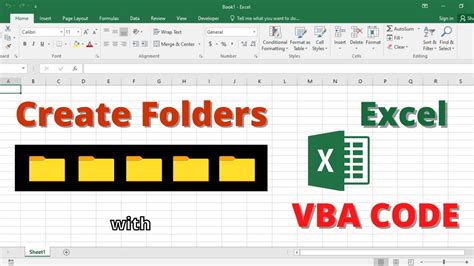
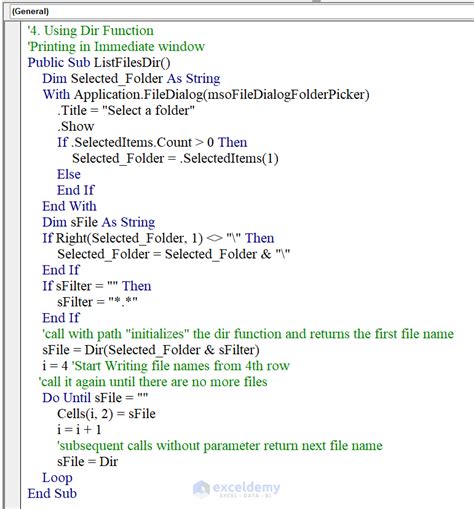
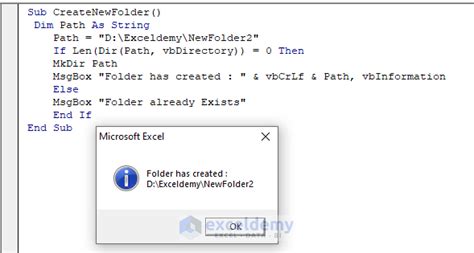
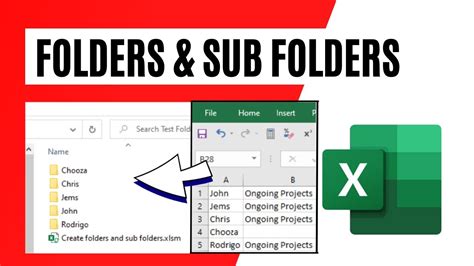
Frequently Asked Questions
Q: How do I create a folder with VBA in Excel?
A: You can create a folder with VBA in Excel by using the MkDir
function.
Q: How do I check if a folder already exists?
A: You can use the Dir
function to check if a folder already exists.
Q: Can I create multiple folders with VBA? A: Yes, you can create multiple folders with VBA by using a loop.
Q: How do I display feedback to the user? A: You can use message boxes to display feedback to the user.
Conclusion
Creating a folder with VBA in Excel is a simple process that can be achieved through a few lines of code. By using the MkDir
function and checking if the folder already exists, you can automate the process of creating folders and save time. Additionally, you can create multiple folders with VBA by using a loop. We hope this article has been helpful in guiding you through the process of creating folders with VBA in Excel.