Intro
Learn how to create new worksheets with VBA made easy. Discover simple and efficient methods to automate worksheet creation using Visual Basic for Applications. Master VBA worksheet manipulation, from inserting new sheets to formatting and data entry. Get expert tips and step-by-step guides to streamline your Excel workflow.
Creating a new worksheet with VBA can be a daunting task for beginners, but with the right guidance, it can be made easy. In this article, we will walk you through the process of creating a new worksheet using VBA, highlighting the benefits, steps, and best practices to get you started.
Why Use VBA to Create a New Worksheet?
Before we dive into the tutorial, let's explore why you might want to use VBA to create a new worksheet. VBA (Visual Basic for Applications) is a powerful programming language that allows you to automate tasks, create custom tools, and interact with Excel in ways that aren't possible with traditional methods.
By using VBA to create a new worksheet, you can:
- Automate repetitive tasks, such as creating a new worksheet with a specific layout or formatting
- Create custom templates with specific settings and formatting
- Interact with other Excel objects, such as charts, tables, and pivot tables
- Use VBA code to perform calculations and data analysis
Step 1: Setting Up Your VBA Environment
Before you can start creating a new worksheet with VBA, you need to set up your VBA environment. To do this, follow these steps:
- Open Excel and click on the "Developer" tab in the ribbon. If you don't see the Developer tab, you can add it by going to the "File" tab, clicking on "Options," and then selecting "Customize Ribbon."
- Click on the "Visual Basic" button in the Developer tab to open the Visual Basic Editor.
- In the Visual Basic Editor, click on "Insert" and then select "Module" to create a new module.
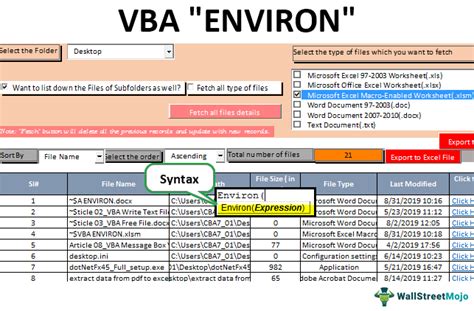
Step 2: Writing Your VBA Code
Now that you have your VBA environment set up, it's time to write your code. To create a new worksheet, you can use the following code:
Sub CreateNewWorksheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Add
ws.Name = "My New Worksheet"
End Sub
This code creates a new worksheet and names it "My New Worksheet." You can customize this code to fit your needs by changing the worksheet name and adding additional formatting or settings.
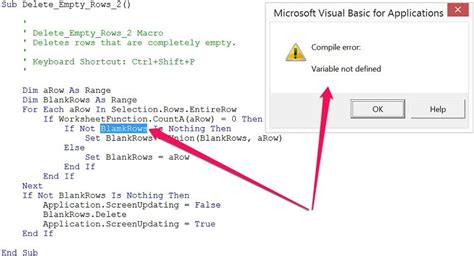
Step 3: Running Your VBA Code
To run your VBA code, follow these steps:
- Click on the "Run" button in the Visual Basic Editor or press F5 on your keyboard.
- The code will execute, creating a new worksheet with the specified name and formatting.
Tips and Best Practices
When working with VBA, there are several tips and best practices to keep in mind:
- Use meaningful variable names to make your code easier to read and understand.
- Use comments to explain what your code is doing and why.
- Use error handling to catch and handle errors that may occur during execution.
- Test your code thoroughly to ensure it works as expected.
Common Errors and Solutions
When working with VBA, you may encounter errors or issues that can be frustrating to resolve. Here are some common errors and solutions:
- Error: "Runtime Error 1004: Method 'Add' of object 'Worksheets' failed." Solution: This error occurs when you try to add a worksheet with a name that already exists. To resolve this error, check the worksheet name and ensure it is unique.
- Error: "Compile Error: Syntax Error." Solution: This error occurs when there is a syntax error in your code. To resolve this error, check your code for typos and ensure that all statements are properly formatted.
Gallery of VBA Worksheet Creation
VBA Worksheet Creation Gallery
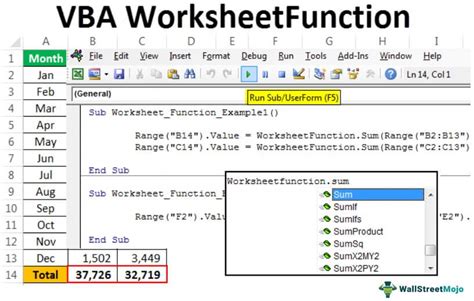
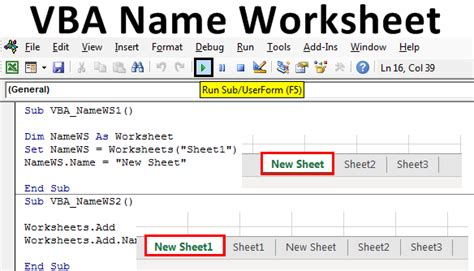
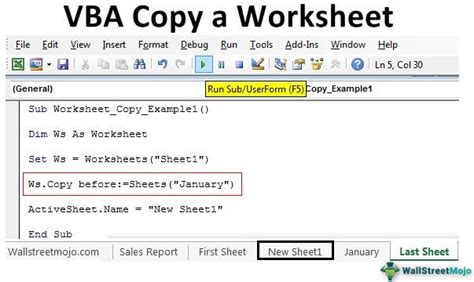
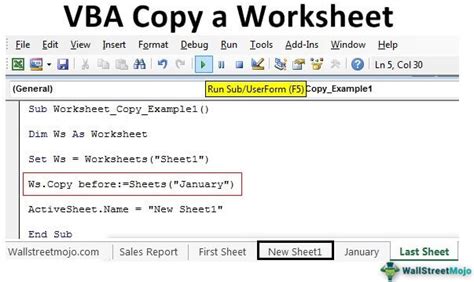
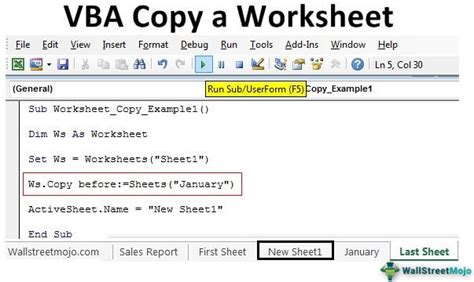
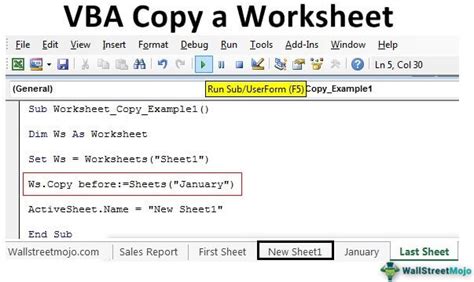
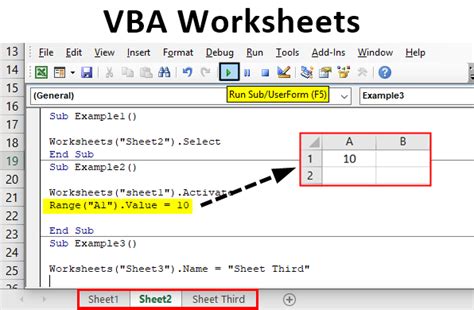
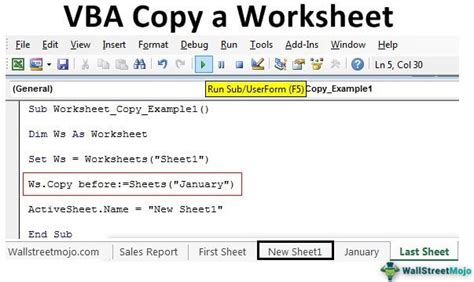
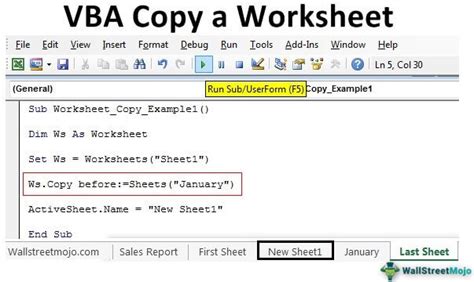
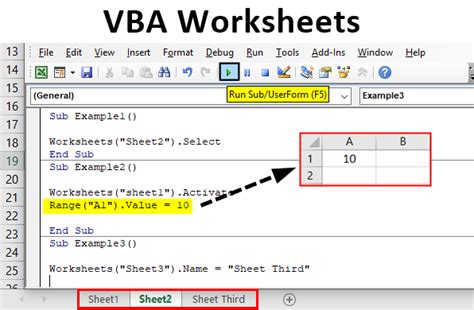
Frequently Asked Questions
Here are some frequently asked questions about creating a new worksheet with VBA:
Q: How do I create a new worksheet with VBA?
A: To create a new worksheet with VBA, you can use the Worksheets.Add
method, specifying the name of the worksheet and any additional formatting or settings.
Q: Can I customize the worksheet name and formatting? A: Yes, you can customize the worksheet name and formatting by modifying the VBA code.
Q: What if I encounter an error while running my VBA code? A: If you encounter an error while running your VBA code, check the error message and refer to the troubleshooting section above.
We hope this article has helped you create a new worksheet with VBA. With practice and patience, you can master the skills needed to automate tasks and create custom tools in Excel. Don't forget to share your own VBA creations and tips in the comments below!