Intro
Master creating tables in VBA with ease. Learn how to automatically generate tables in Excel using Visual Basic for Applications. Discover VBA table creation best practices, including dynamic range selection, formatting, and data manipulation. Boost your productivity with efficient VBA coding techniques and expert table creation strategies.
Creating tables in VBA (Visual Basic for Applications) can be a bit tricky, but don't worry, I'm here to guide you through it.
Introduction
When working with data in Excel, tables are an essential feature that helps organize and analyze data efficiently. In VBA, creating tables can be done using various methods, but we'll focus on the easiest way to create a table using VBA.
Why Create Tables in VBA?
Creating tables in VBA offers several benefits, including:
- Automating table creation and formatting
- Customizing table settings and styles
- Improving data organization and readability
- Enhancing data analysis and reporting capabilities
Easiest Way to Create a Table in VBA
To create a table in VBA, follow these simple steps:
Sub CreateTable()
Dim tbl As ListObject
Dim rng As Range
' Define the range for the table
Set rng = Range("A1:C10") ' Adjust the range to your needs
' Create the table
Set tbl = ActiveSheet.ListObjects.Add(xlSrcRange, rng,, xlYes)
' Format the table
tbl.TableStyle = "TableStyleMedium9"
' Add headers and formulas (optional)
tbl.HeaderRowRange(1).Value = "Header 1"
tbl.ListColumns(1).DataBodyRange.Formula = "=SUM(A2:A10)"
End Sub
In this example, we:
- Define the range for the table using
Range("A1:C10")
. - Create the table using
ActiveSheet.ListObjects.Add
. - Format the table using
TableStyleMedium9
. - Add headers and formulas (optional).
How to Use the Code
To use the code:
- Open the Visual Basic Editor (VBE) by pressing
Alt + F11
or navigating to Developer > Visual Basic. - In the VBE, create a new module by clicking
Insert > Module
. - Paste the code into the module.
- Adjust the range and formatting to suit your needs.
- Run the code by clicking
Run > Run Sub/UserForm
or pressingF5
.
Customizing Table Settings and Styles
To customize table settings and styles, you can use various VBA properties and methods, such as:
TableStyle
: Changes the table style.HeaderRowRange
: Sets the header row values.ListColumns
: Adds or modifies list columns.DataBodyRange
: Formats the data body range.
For example:
tbl.TableStyle = "TableStyleLight11"
tbl.HeaderRowRange(1).Value = "Custom Header"
tbl.ListColumns(1).DataBodyRange.Font.Color = vbBlue
Common Issues and Troubleshooting
When creating tables in VBA, you may encounter issues such as:
- Range errors: Ensure the range is correct and within the worksheet boundaries.
- Style errors: Verify the table style name is correct and available.
- Formula errors: Check the formula syntax and references.
To troubleshoot, use the VBE's built-in debugging tools, such as Debug.Print
statements and the Locals
window.
Best Practices and Tips
To create tables in VBA efficiently:
- Use meaningful variable names and comments.
- Test the code with different ranges and styles.
- Use error handling to catch and resolve issues.
- Keep the code organized and modular.
By following these best practices and tips, you'll be able to create tables in VBA easily and efficiently.
Gallery of Table Creation Examples
Table Creation Examples
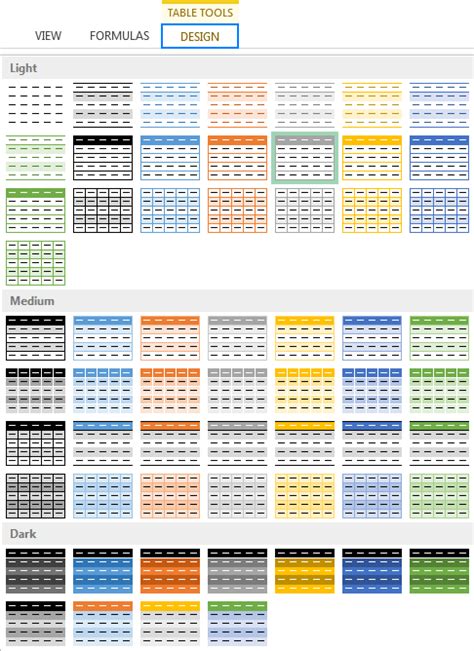
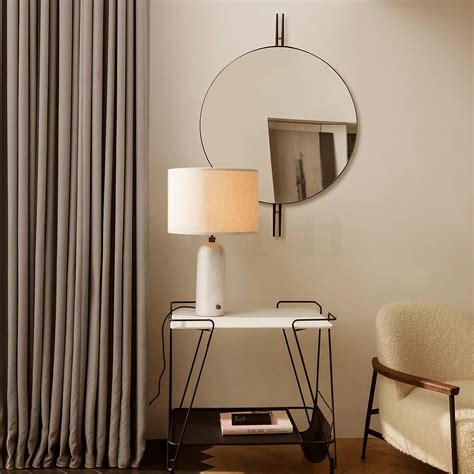
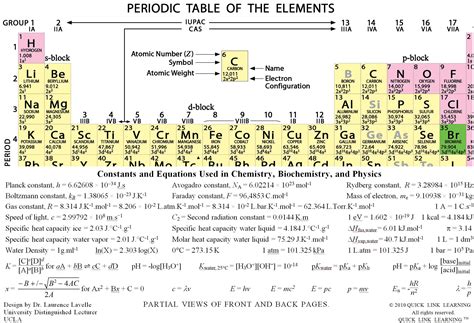
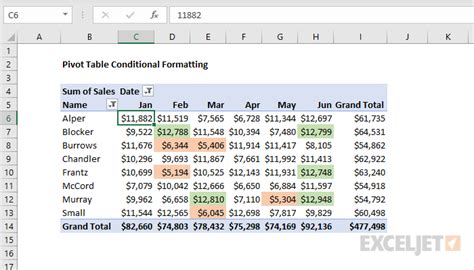
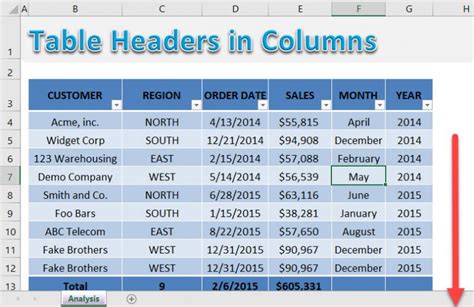
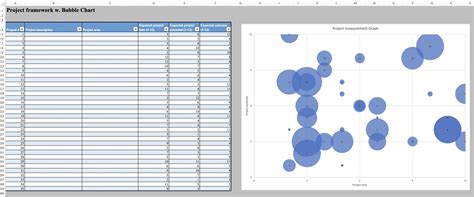
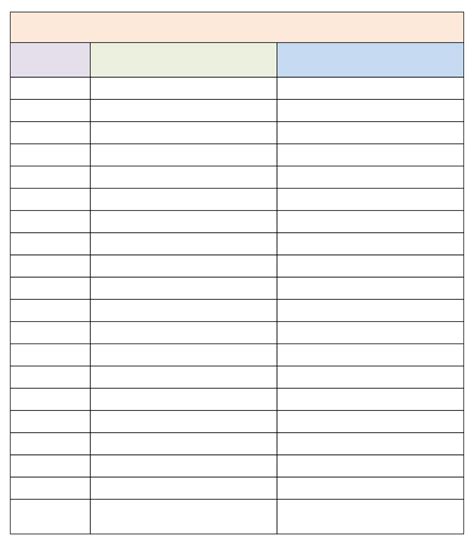
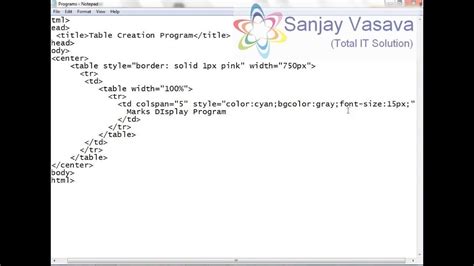
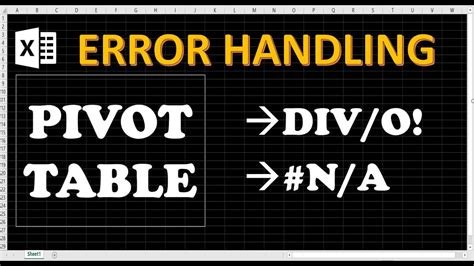
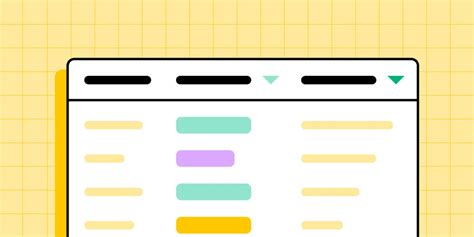
Conclusion
Creating tables in VBA is a powerful feature that can enhance your data analysis and reporting capabilities. By following the steps and best practices outlined in this article, you'll be able to create tables in VBA easily and efficiently.
Do you have any questions or need further assistance? Please leave a comment below!