Intro
Learn how to create a folder with VBA using our step-by-step guide. Master Excel automation by leveraging Visual Basic for Applications. Discover how to create folders, subfolders, and directories programmatically. Boost productivity and streamline workflows with VBA folder creation techniques.
Creating a folder using VBA (Visual Basic for Applications) is a valuable skill that can help automate various tasks in Microsoft Office applications, such as Excel, Word, and Access. In this article, we will explore the importance of VBA folder creation, its benefits, and provide a step-by-step guide on how to create a folder using VBA.
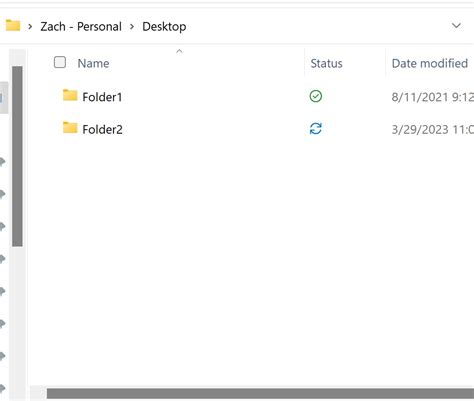
The Importance of VBA Folder Creation
VBA folder creation is a crucial aspect of automating tasks in Microsoft Office applications. By creating folders using VBA, you can streamline your workflow, reduce manual errors, and increase productivity. For instance, you can use VBA to create folders for storing files, organizing data, or creating backups.
Benefits of VBA Folder Creation
- Automation: VBA folder creation allows you to automate the process of creating folders, which can save time and reduce manual errors.
- Flexibility: You can create folders with specific names, dates, or timestamps using VBA, making it easier to organize and manage files.
- Integration: VBA folder creation can be integrated with other VBA scripts or macros, enabling you to automate complex tasks and workflows.
Step-by-Step Guide to Creating a Folder Using VBA
Step 1: Open the Visual Basic Editor
To create a folder using VBA, you need to open the Visual Basic Editor. You can do this by:
- Pressing
Alt + F11
in Excel or Word - Going to
Developer
tab and clicking onVisual Basic
in Excel or Word - Pressing
Ctrl + F11
in Access
Step 2: Create a New Module
In the Visual Basic Editor, create a new module by:
- Going to
Insert
>Module
in the menu bar - Right-clicking on any of the objects in the
Project Explorer
and selectingInsert
>Module
Step 3: Write the VBA Code
In the new module, write the following VBA code to create a folder:
Sub CreateFolder()
Dim folderPath As String
folderPath = "C:\Path\To\New\Folder"
' Create the folder if it doesn't exist
If Dir(folderPath, vbDirectory) = "" Then
MkDir folderPath
MsgBox "Folder created successfully!"
Else
MsgBox "Folder already exists!"
End If
End Sub
Step 4: Run the VBA Code
To run the VBA code, press F5
or go to Run
> Run Sub/UserForm
in the menu bar.
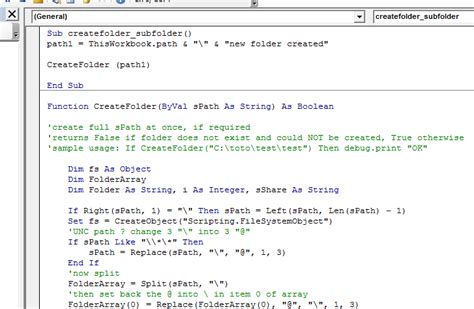
Tips and Variations
- Error Handling: You can add error handling to the VBA code to handle situations where the folder cannot be created.
- Folder Path: You can use variables or user input to specify the folder path.
- Recursive Folder Creation: You can modify the VBA code to create recursive folders.
Best Practices for VBA Folder Creation
- Use meaningful variable names: Use descriptive variable names to make the code easier to understand.
- Test the code: Test the VBA code thoroughly to ensure it works as expected.
- Use error handling: Use error handling to handle unexpected situations.
Common Errors and Troubleshooting
- Permission errors: Ensure that you have the necessary permissions to create folders.
- Path errors: Double-check the folder path to ensure it is correct.
VBA Folder Creation Gallery
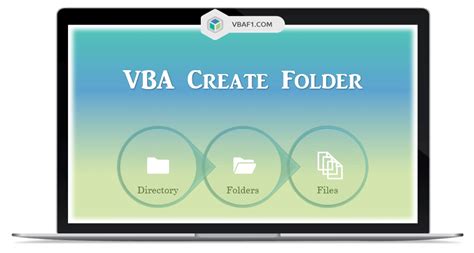
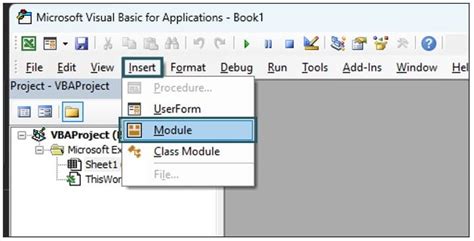
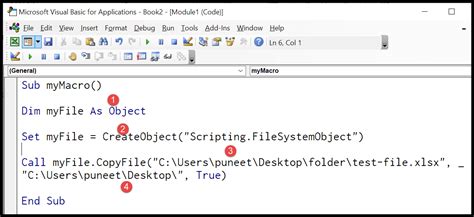
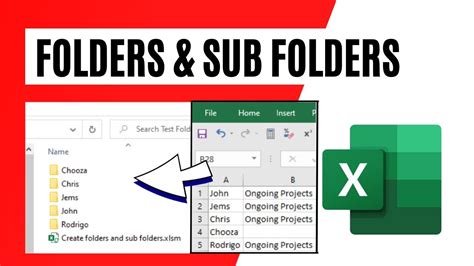
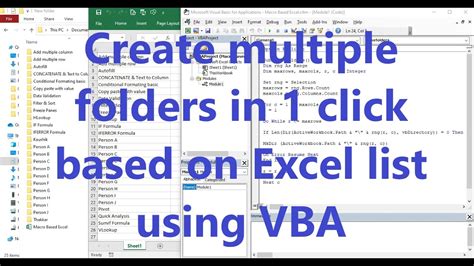
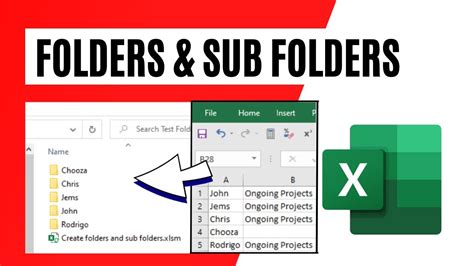
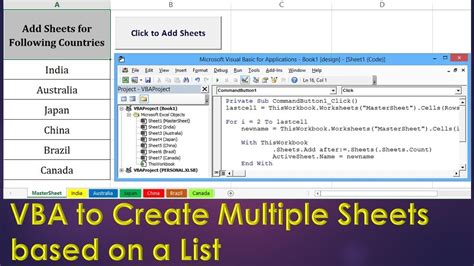
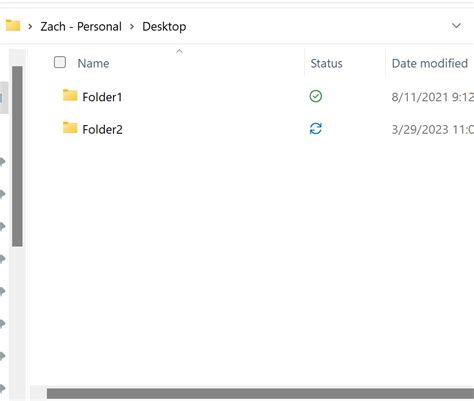
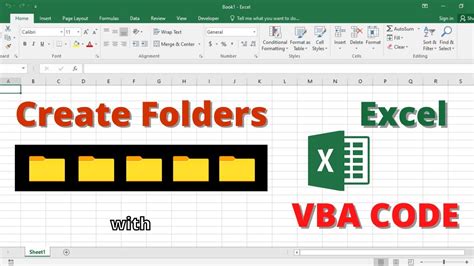
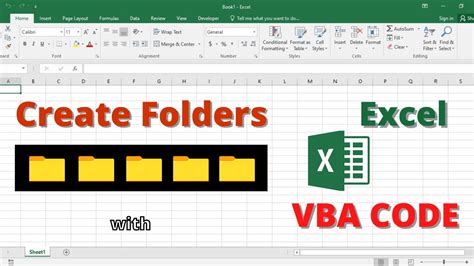
Conclusion
In conclusion, creating a folder using VBA is a valuable skill that can help automate various tasks in Microsoft Office applications. By following the step-by-step guide and tips provided in this article, you can create folders using VBA with ease. Remember to test the code thoroughly and use error handling to handle unexpected situations.
We hope this article has been helpful in teaching you how to create a folder using VBA. If you have any questions or need further assistance, please don't hesitate to ask.