Deleting a column in Excel VBA can be a straightforward process if you know the right methods. In this article, we will explore three different ways to delete a column in Excel VBA, including using the Columns
method, the Range
method, and the ListObjects
method.
Understanding the Basics of Excel VBA
Before we dive into the methods for deleting a column in Excel VBA, it's essential to understand the basics of Excel VBA. Excel VBA (Visual Basic for Applications) is a programming language used to create and automate tasks in Excel. VBA is used to write macros, which are sets of instructions that can be executed with a single command.
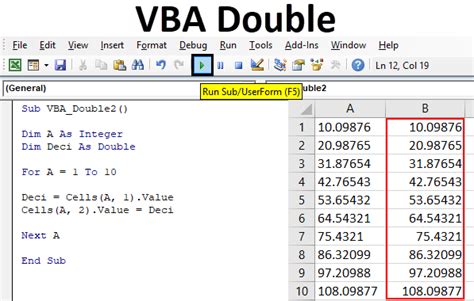
Method 1: Deleting a Column Using the `Columns` Method
The first method for deleting a column in Excel VBA is by using the Columns
method. This method is straightforward and can be used to delete a single column or multiple columns.
Sub DeleteColumnUsingColumnsMethod()
' Delete a single column
Columns(2).Delete
' Delete multiple columns
Columns("B:C").Delete
End Sub
In the above code, we use the Columns
method to delete a single column (column B) and multiple columns (columns B and C).
Advantages of Using the `Columns` Method
- Easy to use and understand
- Can be used to delete single or multiple columns
- Fast execution time
Disadvantages of Using the `Columns` Method
- Can be slow when deleting large ranges of columns
- Does not provide an option to specify a range of cells to delete
Method 2: Deleting a Column Using the `Range` Method
The second method for deleting a column in Excel VBA is by using the Range
method. This method is more flexible than the Columns
method and allows you to specify a range of cells to delete.
Sub DeleteColumnUsingRangeMethod()
' Delete a single column
Range("B:B").Delete
' Delete multiple columns
Range("B:C").Delete
End Sub
In the above code, we use the Range
method to delete a single column (column B) and multiple columns (columns B and C).
Advantages of Using the `Range` Method
- More flexible than the
Columns
method - Allows you to specify a range of cells to delete
- Faster execution time when deleting large ranges of columns
Disadvantages of Using the `Range` Method
- More complex to use than the
Columns
method - Requires specifying a range of cells to delete
Method 3: Deleting a Column Using the `ListObjects` Method
The third method for deleting a column in Excel VBA is by using the ListObjects
method. This method is used to delete a column in a table.
Sub DeleteColumnUsingListObjectsMethod()
' Delete a column in a table
ListObjects("Table1").ListColumns("Column2").Delete
End Sub
In the above code, we use the ListObjects
method to delete a column (column 2) in a table (Table1).
Advantages of Using the `ListObjects` Method
- Used to delete columns in tables
- Fast execution time
- Easy to use and understand
Disadvantages of Using the `ListObjects` Method
- Limited to deleting columns in tables
- Requires specifying the table and column name
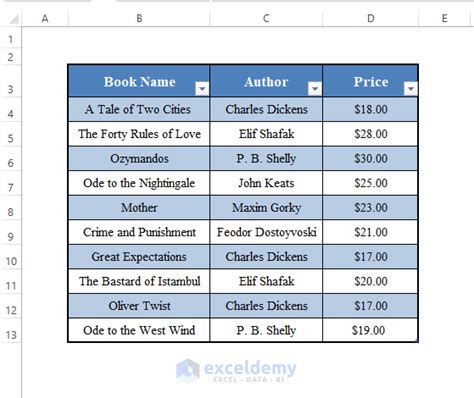
Gallery of Excel VBA Column Deletion Methods
Excel VBA Column Deletion Methods
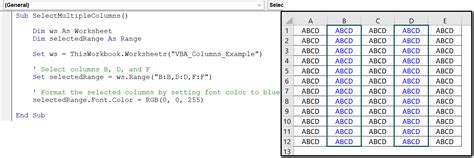
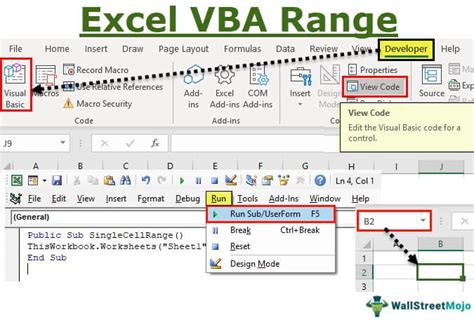
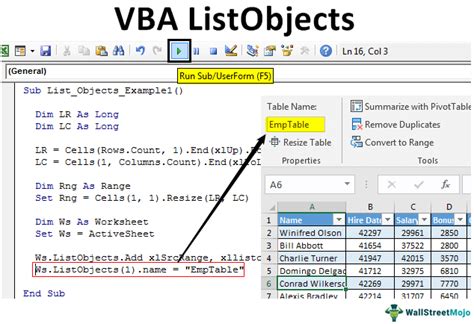
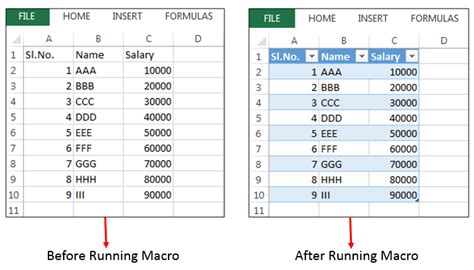
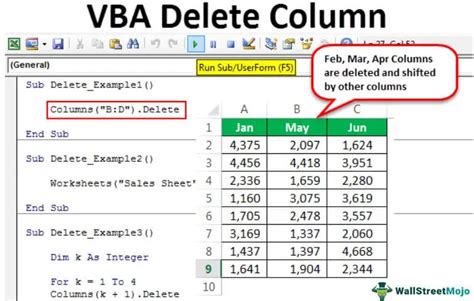
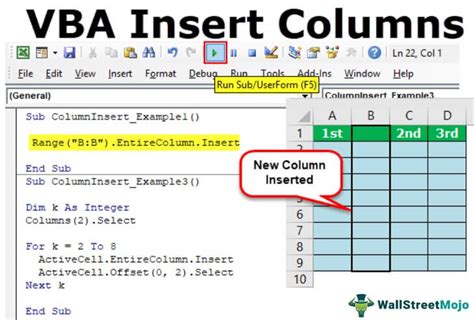
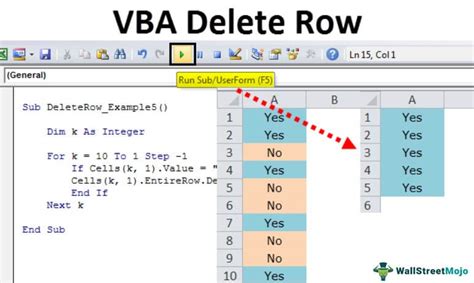
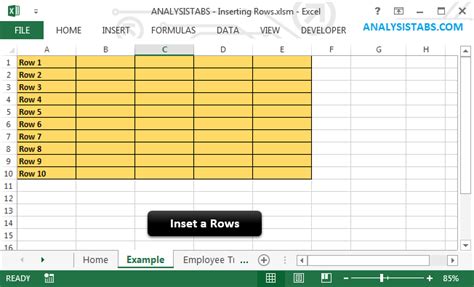
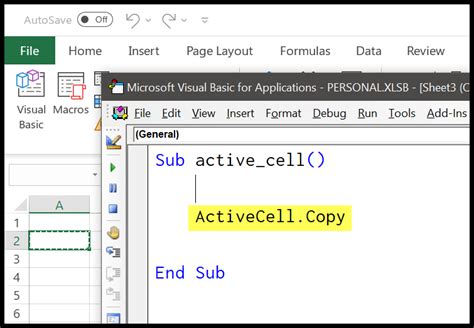
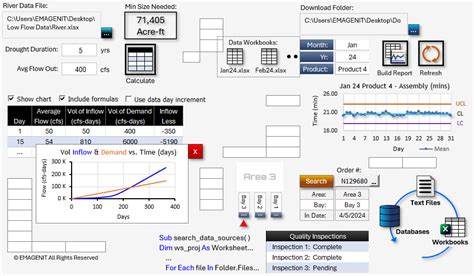
Conclusion
In this article, we explored three different methods for deleting a column in Excel VBA, including using the Columns
method, the Range
method, and the ListObjects
method. Each method has its advantages and disadvantages, and the choice of method depends on the specific requirements of the task.
We hope this article has provided you with a comprehensive understanding of the different methods for deleting a column in Excel VBA. If you have any questions or need further clarification, please feel free to ask in the comments section below.
Remember to subscribe to our blog for more tutorials, tips, and tricks on Excel VBA programming.