Deleting rows in Excel can be a tedious task, especially when dealing with large datasets. However, with the help of VBA (Visual Basic for Applications), you can automate this process and make it more efficient. In this article, we will explore the different ways to delete rows in Excel using VBA and provide step-by-step instructions on how to do so.
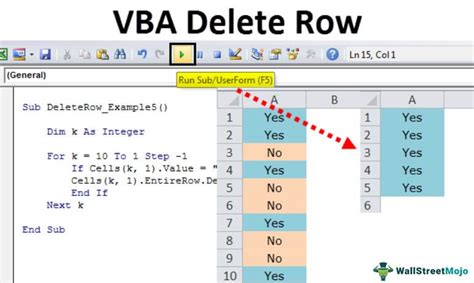
Why Use VBA to Delete Rows in Excel?
Before we dive into the code, let's discuss why using VBA to delete rows in Excel is beneficial. Here are a few reasons:
- Efficiency: VBA can automate the process of deleting rows, saving you time and effort.
- Accuracy: By using VBA, you can avoid manual errors that can occur when deleting rows manually.
- Flexibility: VBA allows you to customize the deletion process based on specific conditions or criteria.
Basic Syntax for Deleting Rows in Excel VBA
The basic syntax for deleting rows in Excel VBA is as follows:
Rows("RowNumber").Delete
Where "RowNumber" is the number of the row you want to delete.
For example:
Rows(5).Delete
This code will delete the fifth row in the active worksheet.
Deleting Multiple Rows in Excel VBA
To delete multiple rows, you can use the following syntax:
Rows("RowNumber1:RowNumber2").Delete
Where "RowNumber1" is the starting row number and "RowNumber2" is the ending row number.
For example:
Rows("5:10").Delete
This code will delete rows 5 through 10 in the active worksheet.
Deleting Rows Based on Conditions in Excel VBA
You can also delete rows based on specific conditions using VBA. For example, you can delete rows that contain a specific value or text string.
Here's an example:
For Each cell In Range("A1:A10") If cell.Value = "Delete Me" Then cell.EntireRow.Delete End If Next cell
This code will loop through cells A1 through A10 and delete any rows that contain the value "Delete Me".
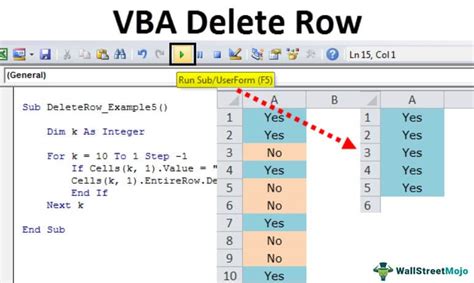
Deleting Blank Rows in Excel VBA
You can also use VBA to delete blank rows in a worksheet. Here's an example:
For Each cell In Range("A1:A10") If IsEmpty(cell) Then cell.EntireRow.Delete End If Next cell
This code will loop through cells A1 through A10 and delete any rows that are blank.
Common Errors When Deleting Rows in Excel VBA
When deleting rows in Excel VBA, there are a few common errors to watch out for:
- Runtime Error 1004: This error occurs when you try to delete a row that is out of range.
- Runtime Error 424: This error occurs when you try to delete a row that is not found.
To avoid these errors, make sure to specify the correct row number or range, and use the On Error Resume Next
statement to handle any errors that may occur.
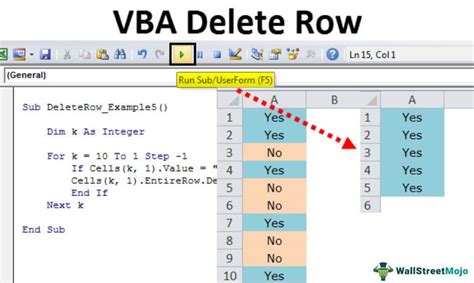
Best Practices for Deleting Rows in Excel VBA
Here are some best practices to keep in mind when deleting rows in Excel VBA:
- Use specific references: Instead of using the
ActiveCell
orActiveRange
objects, use specific references to the cells or ranges you want to delete. - Use error handling: Use the
On Error Resume Next
statement to handle any errors that may occur when deleting rows. - Test your code: Before running your code, test it in a small sample range to make sure it works as expected.
By following these best practices, you can avoid common errors and ensure that your code runs smoothly.
Gallery of Delete Rows in Excel Using VBA Made Easy
Delete Rows in Excel Using VBA Made Easy Image Gallery
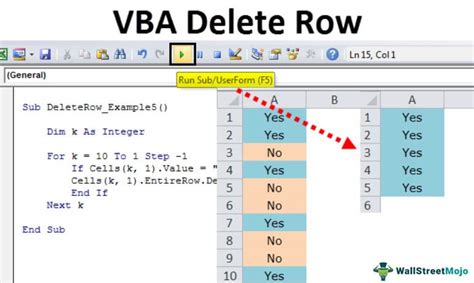
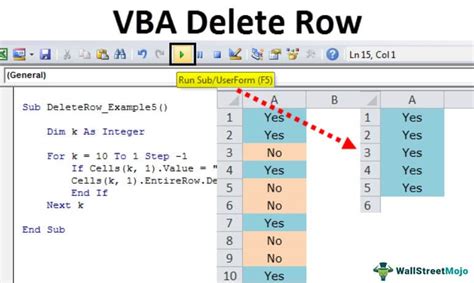
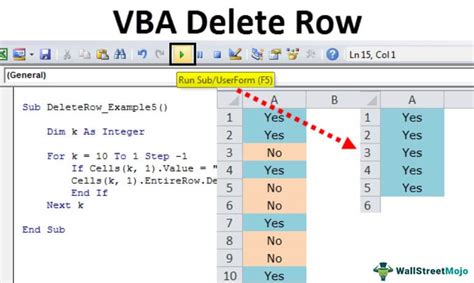
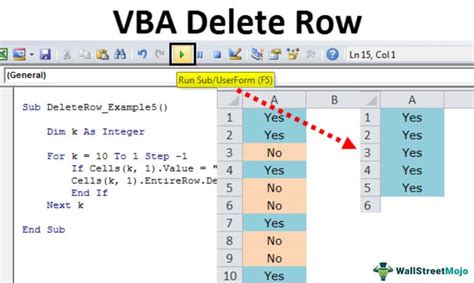
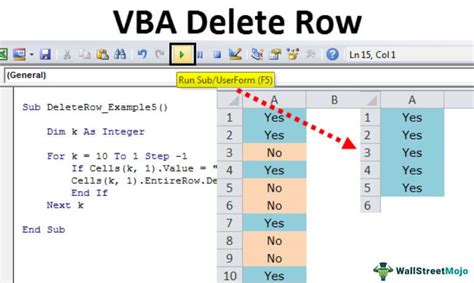
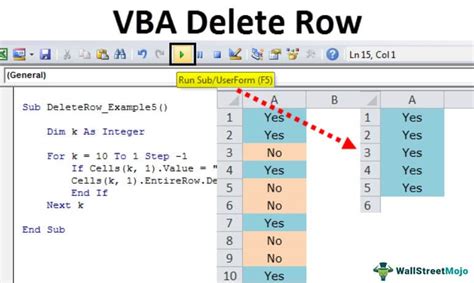
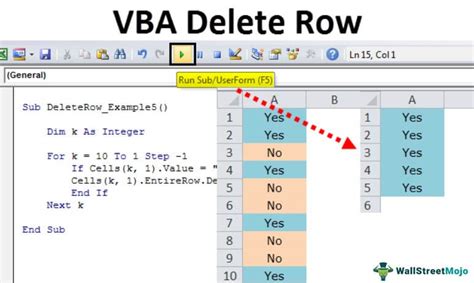
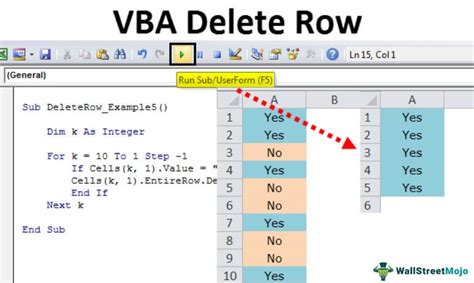
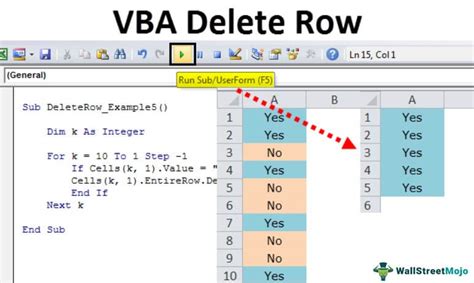
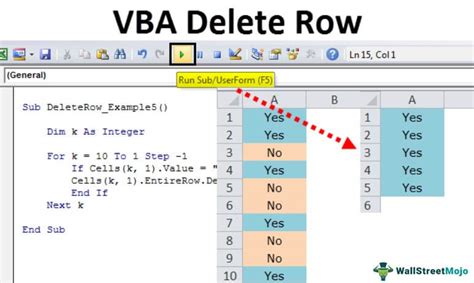
Conclusion
Deleting rows in Excel can be a tedious task, but with the help of VBA, you can automate this process and make it more efficient. By following the best practices outlined in this article, you can avoid common errors and ensure that your code runs smoothly. Whether you're a beginner or an advanced user, this article has provided you with the tools and knowledge to delete rows in Excel using VBA with ease.