Intro
Master Excel VBA with our expert guide on deleting sheets. Learn 3 efficient methods to remove unwanted sheets using VBA code, including deleting sheets by name, index, and active sheet. Streamline your workflow and improve productivity with these simple yet powerful VBA techniques.
Working with spreadsheets in Microsoft Excel can be a tedious task, especially when dealing with multiple sheets. However, with the power of VBA (Visual Basic for Applications), you can automate various tasks, including deleting sheets. Deleting a sheet in VBA can be a bit tricky, but don't worry, we've got you covered. In this article, we'll explore three ways to delete a sheet in VBA.
The Importance of Deleting Sheets in VBA
Before we dive into the methods, let's understand why deleting sheets in VBA is important. When working with large datasets or complex spreadsheets, it's common to create temporary sheets for calculations, data manipulation, or testing. However, once these sheets have served their purpose, they can clutter your workbook and make it harder to navigate. By deleting unnecessary sheets, you can keep your workbook organized, reduce file size, and improve overall performance.
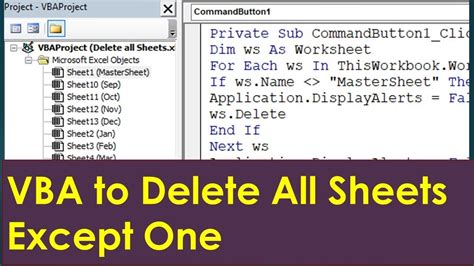
Method 1: Using the Delete
Method
The first method to delete a sheet in VBA is by using the Delete
method. This method is straightforward and works by specifying the sheet you want to delete.
Sub DeleteSheetByName()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
ws.Delete
End Sub
In this example, we set a worksheet object ws
to the sheet named "Sheet1" in the active workbook. Then, we use the Delete
method to delete the sheet.
Method 2: Using the Worksheets
Collection
The second method to delete a sheet in VBA is by using the Worksheets
collection. This method allows you to delete a sheet by its index or name.
Sub DeleteSheetByIndex()
ThisWorkbook.Worksheets(1).Delete
End Sub
In this example, we delete the first sheet in the active workbook by its index (1). You can also specify the sheet name instead of the index.
Method 3: Using the Application
Object
The third method to delete a sheet in VBA is by using the Application
object. This method allows you to delete a sheet by its name or index, and also provides additional options, such as prompting the user for confirmation.
Sub DeleteSheetWithPrompt()
Application.DisplayAlerts = False
ThisWorkbook.Worksheets("Sheet1").Delete
Application.DisplayAlerts = True
End Sub
In this example, we set the DisplayAlerts
property to False
to suppress the confirmation prompt, delete the sheet, and then set it back to True
.
Best Practices for Deleting Sheets in VBA
When deleting sheets in VBA, it's essential to follow best practices to avoid errors and ensure data integrity. Here are some tips:
- Always specify the workbook and sheet name or index to avoid deleting the wrong sheet.
- Use the
Application.DisplayAlerts
property to control the confirmation prompt. - Use error handling to catch and handle errors that may occur during the deletion process.
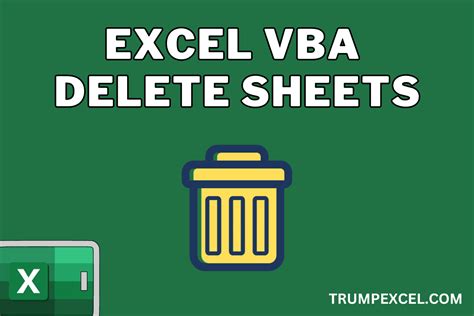
Common Errors When Deleting Sheets in VBA
When deleting sheets in VBA, you may encounter errors due to various reasons. Here are some common errors and their solutions:
- Error 1004: Delete method of Worksheet class failed: This error occurs when the sheet is protected or has a password. Solution: Unprotect the sheet or provide the password.
- Error 9: Subscript out of range: This error occurs when the sheet does not exist. Solution: Check the sheet name or index and correct it.
Conclusion
Deleting sheets in VBA can be a bit tricky, but with the right methods and best practices, you can automate this task with ease. By using the Delete
method, Worksheets
collection, or Application
object, you can delete sheets efficiently and keep your workbook organized. Remember to follow best practices and handle errors to ensure data integrity.
Delete Sheet VBA Image Gallery
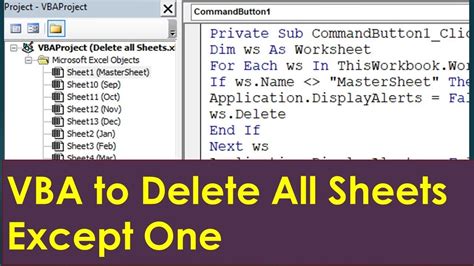
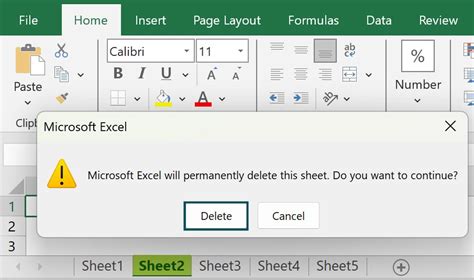
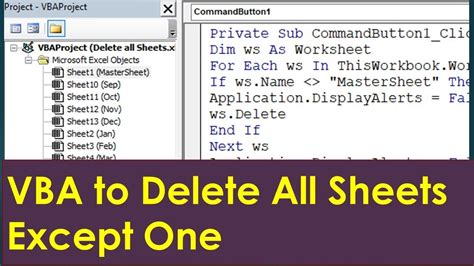
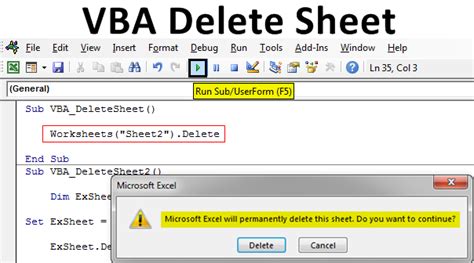
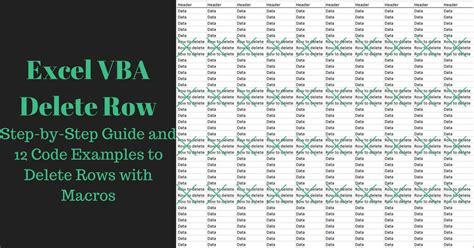
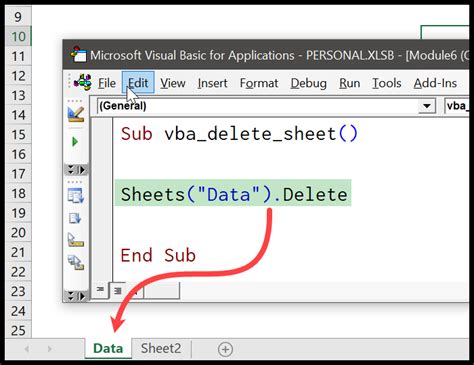
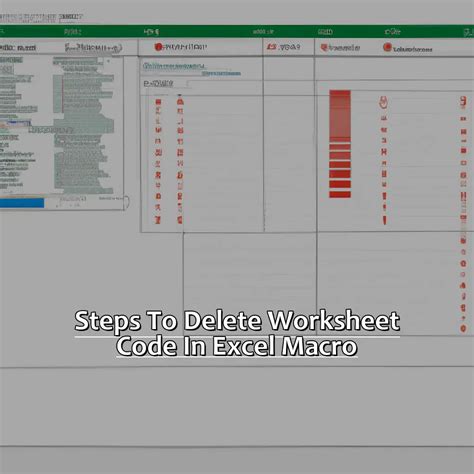
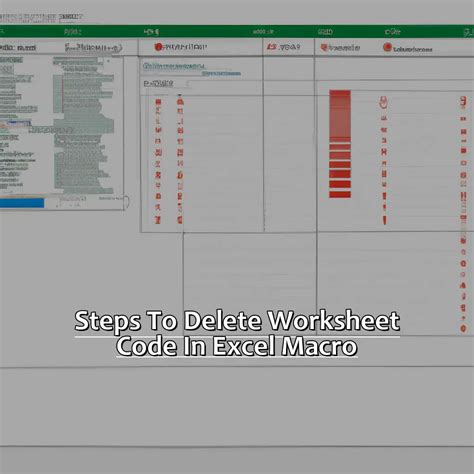
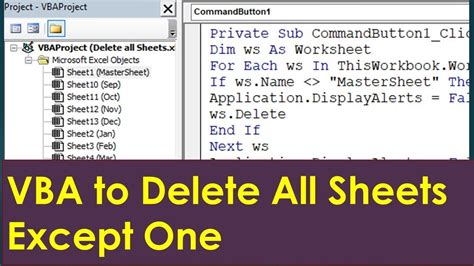
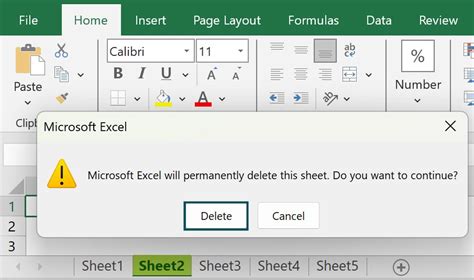
We hope this article has helped you learn how to delete sheets in VBA. If you have any questions or need further assistance, please leave a comment below. Don't forget to share this article with your friends and colleagues who may find it useful. Happy coding!