Intro
Learn how to delete an Excel sheet using VBA without displaying a warning message. Discover the code to bypass the confirmation prompt and permanently delete worksheets programmatically. Master VBA sheet deletion, error handling, and optimize your Excel automation workflows with this step-by-step guide and expert code examples.
Deleting an Excel sheet can be a straightforward process, but sometimes, you may encounter a warning message that asks for confirmation before the sheet is deleted. This can be annoying, especially if you're working with a large number of sheets or automating tasks using VBA. In this article, we'll explore how to delete an Excel sheet using VBA without displaying a warning message.
Why Delete an Excel Sheet using VBA?
There are several scenarios where deleting an Excel sheet using VBA is useful:
- Automating tasks: You can use VBA to automate repetitive tasks, such as deleting sheets that are no longer needed.
- Streamlining workflows: Deleting unnecessary sheets can help declutter your workbook and improve performance.
- Preventing accidental edits: By deleting sheets that are no longer required, you can prevent accidental edits or changes.
How to Delete an Excel Sheet using VBA
To delete an Excel sheet using VBA, you can use the Delete
method of the Worksheet
object. Here's a simple example:
Sub DeleteSheet()
Worksheets("Sheet1").Delete
End Sub
In this example, replace "Sheet1" with the name of the sheet you want to delete.
Disabling Warning Messages
To disable the warning message that appears when deleting a sheet, you can use the Application.DisplayAlerts
property. Set this property to False
before deleting the sheet, and then set it back to True
after deletion.
Here's an updated example:
Sub DeleteSheetWithoutWarning()
Application.DisplayAlerts = False
Worksheets("Sheet1").Delete
Application.DisplayAlerts = True
End Sub
By setting Application.DisplayAlerts
to False
, you suppress the warning message that would normally appear when deleting a sheet.
Best Practices
When deleting sheets using VBA, keep the following best practices in mind:
- Use error handling: Wrap your code in error-handling statements to catch any unexpected errors that may occur during deletion.
- Verify sheet existence: Before deleting a sheet, verify that it exists to avoid errors.
- Backup your data: Always backup your data before making significant changes, such as deleting sheets.
Example Use Cases
Here are some example use cases for deleting Excel sheets using VBA:
- Deleting multiple sheets: You can use a loop to delete multiple sheets at once.
Sub DeleteMultipleSheets()
Dim sheetName As String
Dim i As Integer
For i = 1 To 5
sheetName = "Sheet" & i
Worksheets(sheetName).Delete
Next i
End Sub
- Deleting sheets based on conditions: You can use conditions, such as sheet names or contents, to determine which sheets to delete.
Sub DeleteSheetsBasedOnConditions()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name Like "Template*" Then
ws.Delete
End If
Next ws
End Sub
Gallery of Deleting Excel Sheets using VBA
Deleting Excel Sheets using VBA Image Gallery
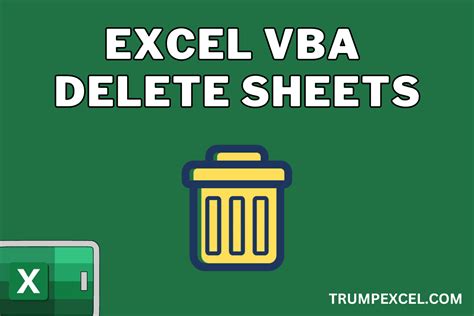
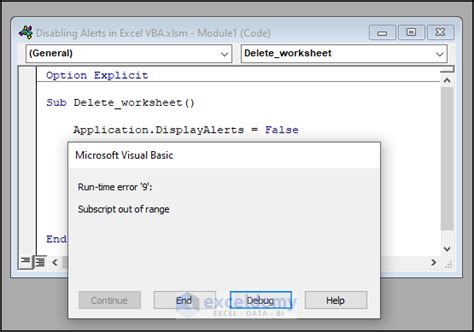
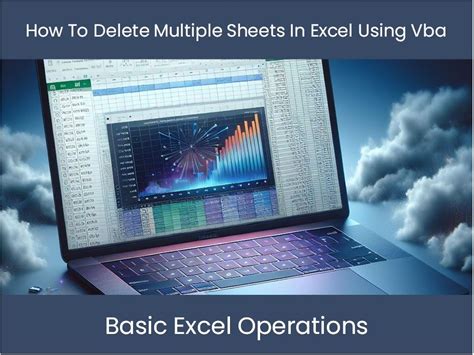
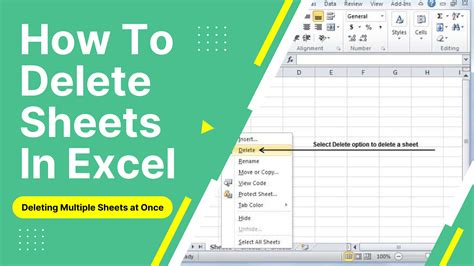
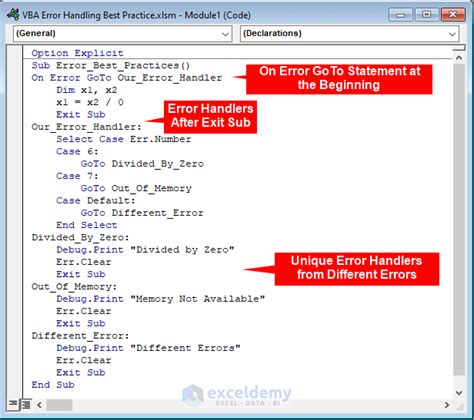
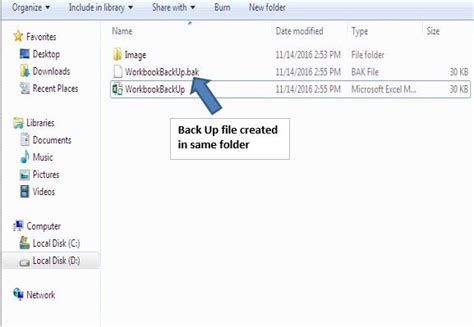
Conclusion
Deleting Excel sheets using VBA can be a powerful tool for automating tasks and streamlining workflows. By disabling warning messages and using error handling, you can ensure that your code runs smoothly and efficiently. Remember to always backup your data before making significant changes, and use conditions to determine which sheets to delete. With these best practices in mind, you'll be able to delete Excel sheets like a pro!