Understanding Datasheet Row Resize in Access VBA
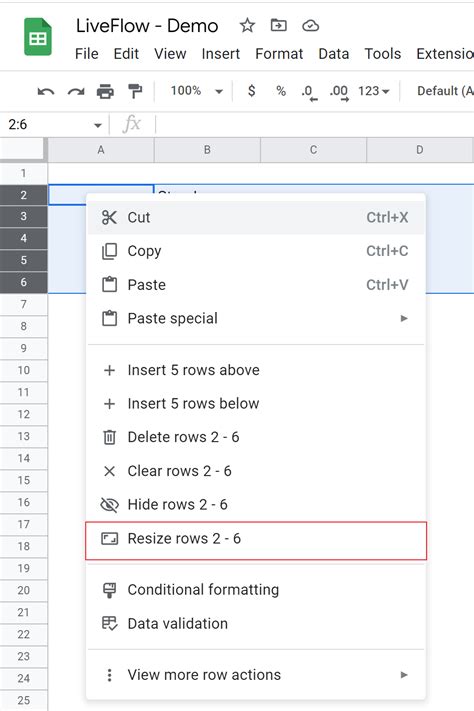
When working with datasheets in Microsoft Access, you may have noticed that users can resize rows by dragging the row borders. While this feature can be useful, there may be situations where you want to disable it to maintain a consistent layout or to prevent users from accidentally changing the row heights. In this article, we will explore four ways to disable datasheet row resize in Access VBA.
Method 1: Using the AllowRowResize Property
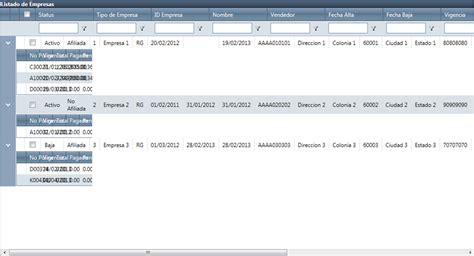
The AllowRowResize property is a built-in property in Access that allows you to control whether users can resize rows in a datasheet. To disable row resizing, you can set this property to False. Here's an example of how to do this in VBA:
Sub DisableRowResize()
Forms!YourFormName.AllowRowResize = False
End Sub
Replace "YourFormName" with the actual name of your form.
When to Use This Method
This method is useful when you want to disable row resizing for a specific form or datasheet. However, keep in mind that this property only applies to the current form or datasheet and does not affect other forms or datasheets in your database.
Method 2: Using the Datasheet Properties
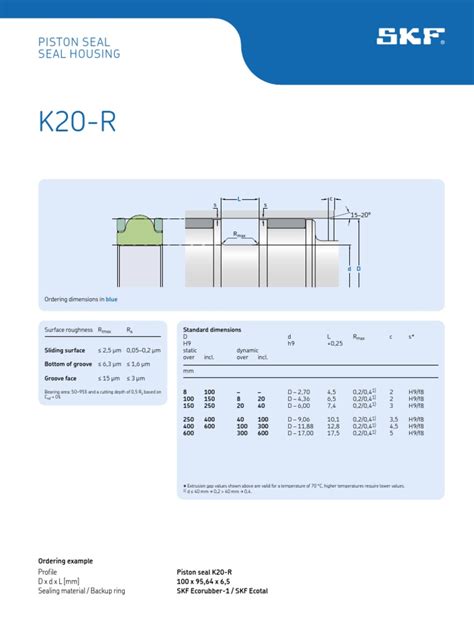
Another way to disable row resizing is to use the datasheet properties. To do this, follow these steps:
- Open your form or datasheet in Design view.
- Click on the datasheet to select it.
- In the Property Sheet, scroll down to the "Other" tab.
- Set the "Allow Row Resize" property to "No".
When to Use This Method
This method is useful when you want to disable row resizing for a specific datasheet or form and you don't need to do it programmatically.
Method 3: Using VBA Code in the Form's Open Event
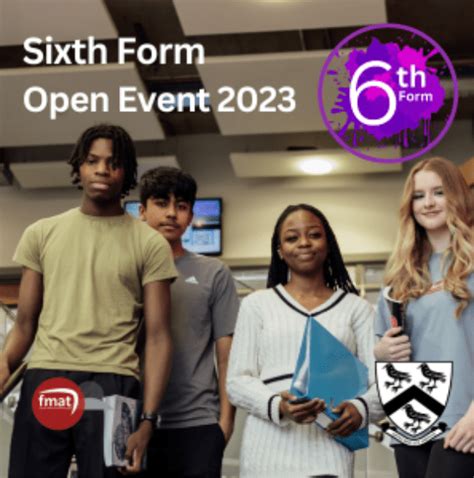
You can also use VBA code in the form's Open event to disable row resizing. Here's an example of how to do this:
Private Sub Form_Open(Cancel As Integer)
Me.AllowRowResize = False
End Sub
When to Use This Method
This method is useful when you want to disable row resizing for a specific form and you want to do it programmatically. This code will run every time the form is opened.
Method 4: Using a Class Module
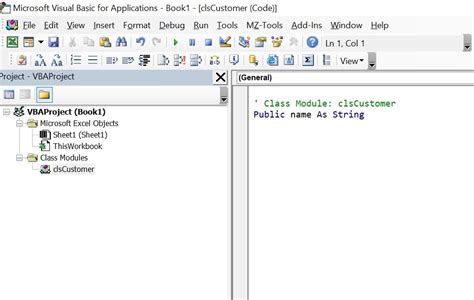
You can also use a class module to disable row resizing for multiple forms or datasheets. Here's an example of how to do this:
' clsDatasheetUtilities
' --------------------------------
' Purpose: Disable row resizing for datasheets
' --------------------------------
Option Explicit
Private WithEvents m_frm As Form
Public Sub Initialize(frm As Form)
Set m_frm = frm
m_frm.AllowRowResize = False
End Sub
Private Sub m_frm_Open(Cancel As Integer)
m_frm.AllowRowResize = False
End Sub
To use this class module, you need to create an instance of it and pass the form object to the Initialize method.
When to Use This Method
This method is useful when you want to disable row resizing for multiple forms or datasheets and you want to do it programmatically. This method is more flexible and reusable than the other methods.
Disable Datasheet Row Resize Image Gallery
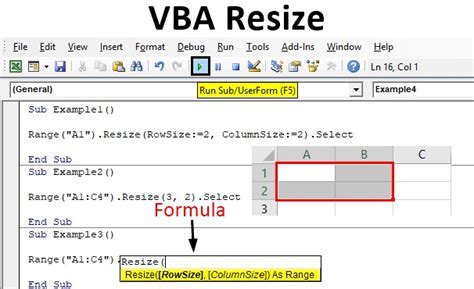
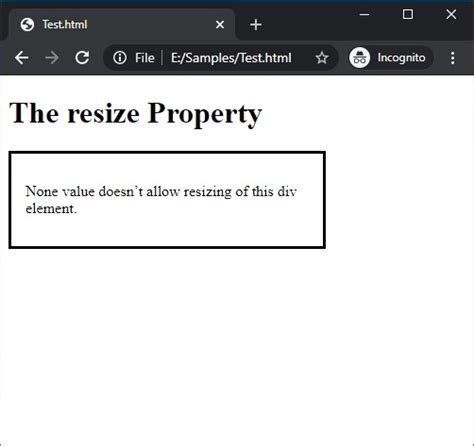
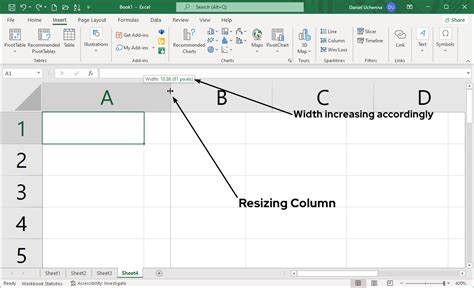
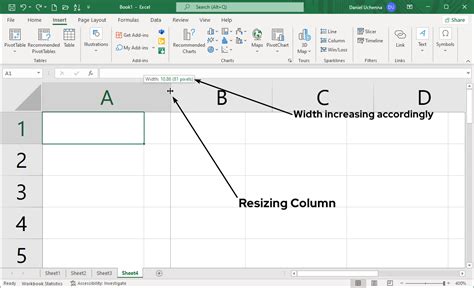
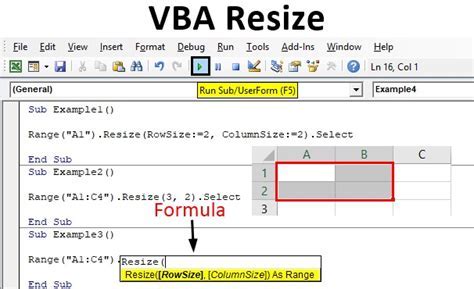
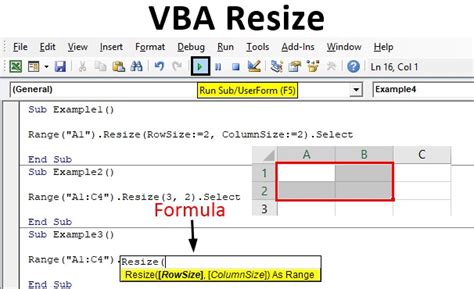
Conclusion
In this article, we explored four ways to disable datasheet row resize in Access VBA. Each method has its own advantages and disadvantages, and the choice of method depends on your specific needs and requirements. By using one of these methods, you can prevent users from resizing rows in your datasheets and maintain a consistent layout.