Intro
Learn how to fix the else without if error in Java. Discover the common causes, symptoms, and step-by-step solutions to resolve this compilation error. Understand how to properly use if-else statements, troubleshoot syntax issues, and write error-free Java code. Master Java error handling and coding best practices.
Fixing Else Without If Error In Java
The "else without if" error is a common mistake in Java programming. It occurs when the compiler encounters an "else" clause without a corresponding "if" statement. This error can be frustrating, especially for beginners. In this article, we will explore the causes of this error, provide examples, and offer solutions to fix it.
Understanding the Error
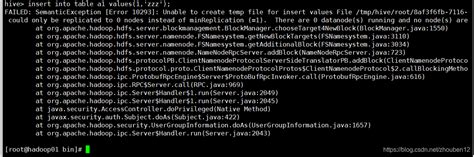
The "else without if" error typically occurs when the compiler expects an "if" statement but finds an "else" clause instead. This can happen due to several reasons, including:
- Missing or misplaced "if" statement
- Incorrect indentation or formatting
- Forgotten or misplaced curly brackets
Causes of the Error
- Missing "if" statement: The most common cause of this error is a missing "if" statement. The compiler expects an "if" statement to precede an "else" clause. If the "if" statement is missing, the compiler will throw an error.
- Incorrect indentation or formatting: Java is sensitive to indentation and formatting. If the code is not properly indented or formatted, the compiler may interpret the code incorrectly, leading to the "else without if" error.
- Forgotten or misplaced curly brackets: Curly brackets are used to group statements in Java. If a curly bracket is forgotten or misplaced, the compiler may interpret the code incorrectly, leading to the "else without if" error.
Examples of the Error
Here are some examples of code that may produce the "else without if" error:
public class Example {
public static void main(String[] args) {
else {
System.out.println("This will produce an error");
}
}
}
In this example, the "else" clause is not preceded by an "if" statement, which will produce the "else without if" error.
public class Example {
public static void main(String[] args) {
if (true) {
System.out.println("This will print");
} else {
System.out.println("This will not print");
}
}
In this example, the "else" clause is correctly preceded by an "if" statement, but the code is missing a closing curly bracket, which will produce the "else without if" error.
Solutions to Fix the Error
To fix the "else without if" error, you need to ensure that the "else" clause is correctly preceded by an "if" statement. Here are some solutions:
- Add the missing "if" statement: If the "if" statement is missing, add it before the "else" clause.
- Check the indentation and formatting: Ensure that the code is properly indented and formatted. Use a consistent number of spaces for indentation, and ensure that the curly brackets are correctly placed.
- Add the forgotten or misplaced curly bracket: If a curly bracket is forgotten or misplaced, add it to the correct location.
Here is an example of corrected code:
public class Example {
public static void main(String[] args) {
if (true) {
System.out.println("This will print");
} else {
System.out.println("This will not print");
}
}
}
In this example, the "else" clause is correctly preceded by an "if" statement, and the code is properly indented and formatted.
Best Practices to Avoid the Error
To avoid the "else without if" error, follow these best practices:
- Always ensure that an "else" clause is preceded by an "if" statement.
- Use a consistent number of spaces for indentation, and ensure that the curly brackets are correctly placed.
- Use a code editor or IDE that provides syntax highlighting and error checking to help identify and fix errors.
Conclusion
The "else without if" error is a common mistake in Java programming. By understanding the causes of this error and following the solutions provided, you can fix the error and ensure that your code compiles correctly. Remember to always follow best practices to avoid this error and write clean, readable code.
Gallery of Java Errors
Java Error Gallery
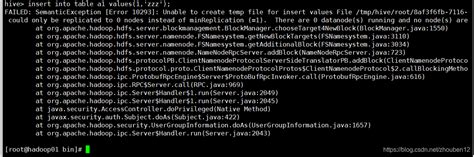
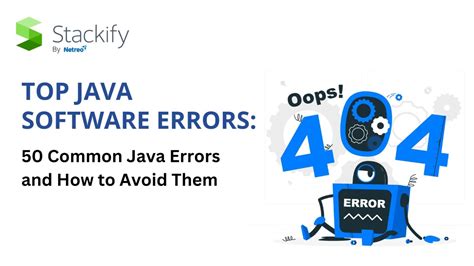
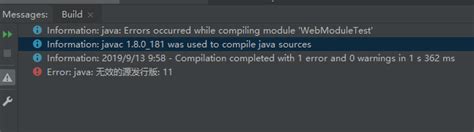
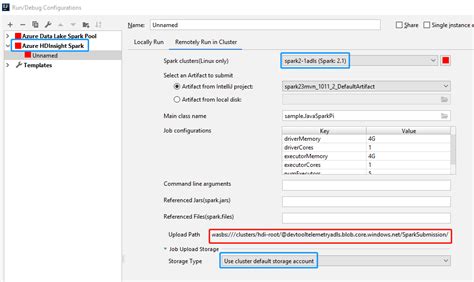
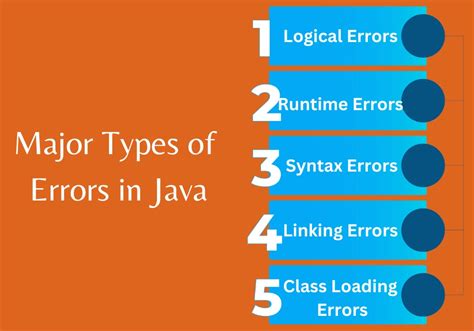
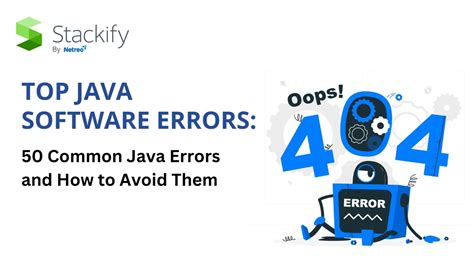
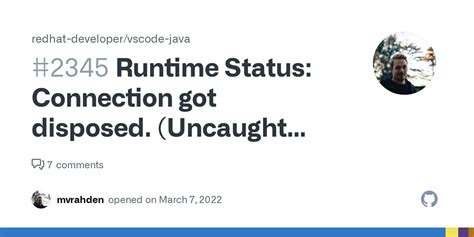
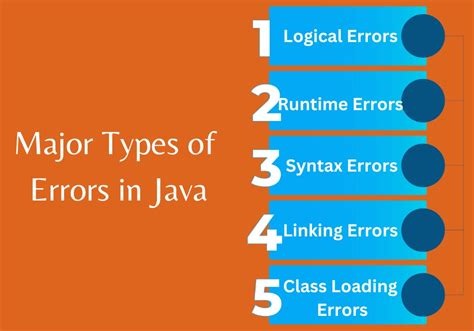
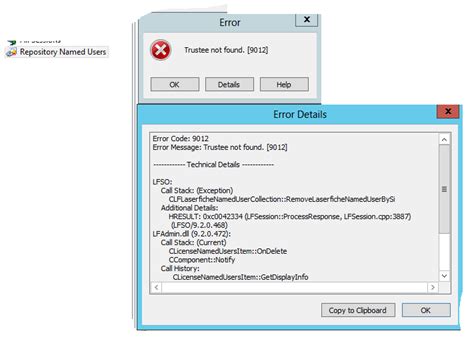
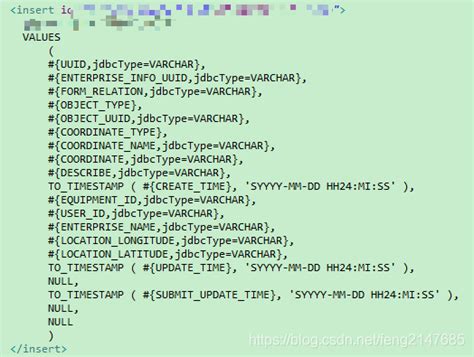
We hope this article has been helpful in fixing the "else without if" error in Java. If you have any questions or comments, please feel free to share them below.