Finding values in one column but not another is a common data analysis task that can be accomplished using various methods, depending on the tools and programming languages you are working with. Here, we'll explore five ways to achieve this using popular data manipulation and analysis tools, including Microsoft Excel, Google Sheets, Python (with Pandas), SQL, and JavaScript (with arrays). Each method is tailored to a specific environment, ensuring you can adapt the solution to your specific needs.
Method 1: Using Microsoft Excel

In Excel, you can find values in one column that are not in another using a combination of the IF, ISERROR, and VLOOKUP functions. Here’s how you can do it:
- Assumptions: Let's say you have values in Column A that you want to check against values in Column B.
- Formula: In a new column (e.g., Column C), enter the following formula:
=IF(ISERROR(VLOOKUP(A2, B:B, 1, FALSE)), "Not Found", "Found")
. This checks if the value in cell A2 exists in Column B. If it does, it returns "Found"; otherwise, it returns "Not Found." - Drag Down: Copy the formula down to apply it to all cells in Column A.
Filtering Results
To see only the values not found in Column B, you can filter the results:
- Select Data: Select the entire range of data including headers.
- Data > Filter: Apply a filter to the data.
- Filter Column C: Click on the filter icon in Column C's header and select "Not Found."
Method 2: Using Google Sheets
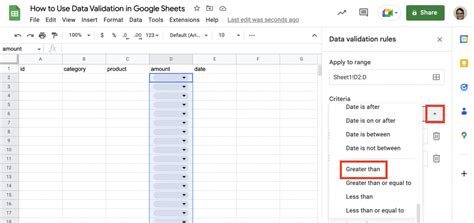
Similar to Excel, Google Sheets uses the FILTER and ISERROR functions along with VLOOKUP for this task.
- Assumptions: Assume values are in Column A and to be checked against Column B.
- Formula: In a new column (e.g., Column C), use
=FILTER(A:A, ISERROR(VLOOKUP(A:A, B:B, 1, FALSE)))
. This formula directly filters out values that are found in Column B, showing only those not present.
Method 3: Using Python with Pandas
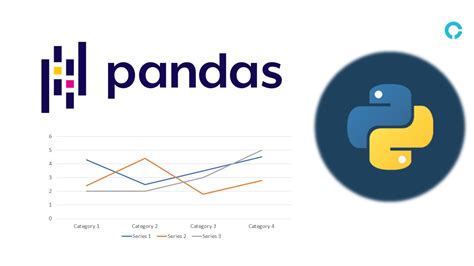
Pandas offers a powerful and flexible way to manipulate data in Python.
import pandas as pd
# Sample data
data = {
'ColumnA': ['Value1', 'Value2', 'Value3', 'Value4'],
'ColumnB': ['Value2', 'Value3', 'Value5', 'Value6']
}
df = pd.DataFrame(data)
# Find values in ColumnA not in ColumnB
not_in_column_b = df[~df['ColumnA'].isin(df['ColumnB'])]['ColumnA']
print(not_in_column_b)
Method 4: Using SQL
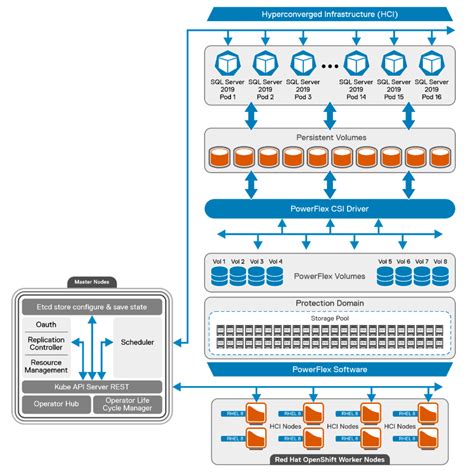
SQL provides a straightforward way to find values in one column but not another using the NOT IN or NOT EXISTS clause.
SELECT ColumnA
FROM your_table
WHERE ColumnA NOT IN (SELECT ColumnB FROM your_table);
Or, using JOINs for a more complex scenario:
SELECT t1.ColumnA
FROM your_table t1
LEFT JOIN your_table t2 ON t1.ColumnA = t2.ColumnB
WHERE t2.ColumnB IS NULL;
Method 5: Using JavaScript
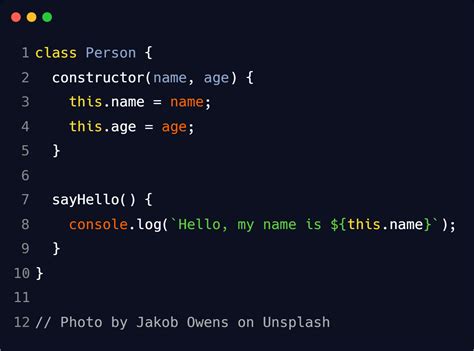
In JavaScript, you can use the filter method to achieve this.
const arrayA = ['Value1', 'Value2', 'Value3', 'Value4'];
const arrayB = ['Value2', 'Value3', 'Value5', 'Value6'];
const notInArrayB = arrayA.filter(value =>!arrayB.includes(value));
console.log(notInArrayB);
Gallery of Finding Values in One Column But Not Another
Find Values in One Column But Not Another Gallery

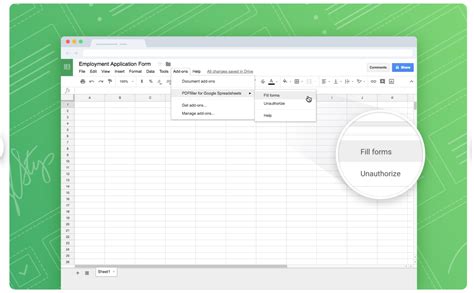
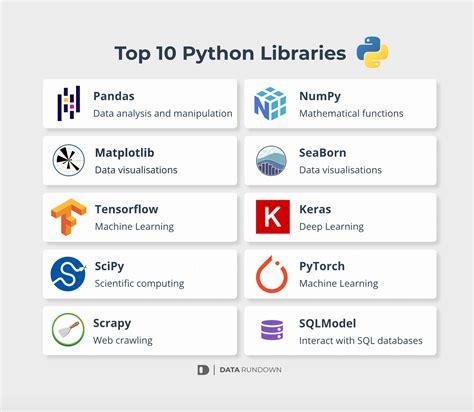
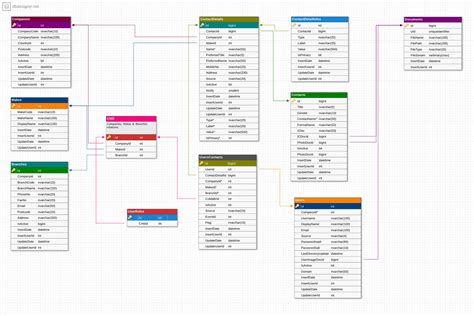
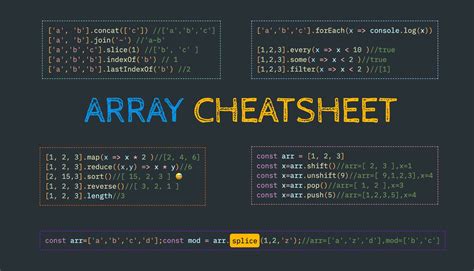
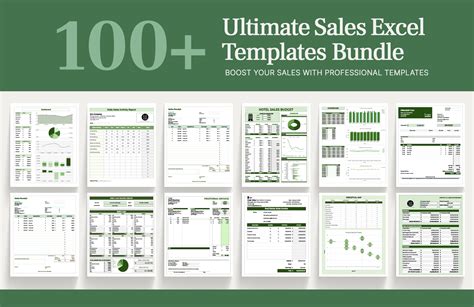

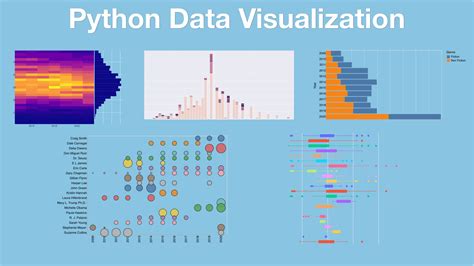
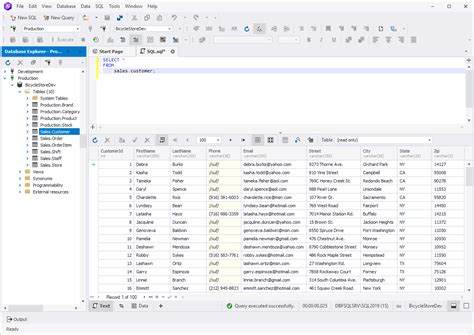
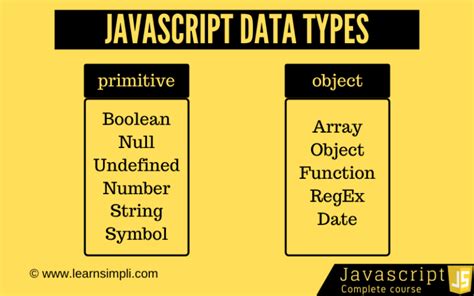
In conclusion, finding values in one column but not another is a fundamental task in data analysis, crucial for data cleansing, comparison, and understanding relationships between datasets. By mastering the methods outlined above for various tools and programming languages, you'll be well-equipped to handle a wide range of data analysis tasks efficiently. Whether you're working in a spreadsheet, scripting in Python, querying a database, or coding in JavaScript, these techniques will help you navigate your data with precision.