Intro
Master the art of finding the last row in Excel with VBA! Learn how to effortlessly navigate worksheets and identify the final row using Visual Basic for Applications. Discover VBA codes, functions, and techniques to automate tasks and boost productivity. Say goodbye to manual scrolling and hello to efficient Excel management with our expert VBA tutorials.
Finding the last row in Excel with VBA is a crucial skill for any aspiring Excel developer. Whether you're dealing with a small dataset or a massive spreadsheet, identifying the last row is essential for tasks like data analysis, reporting, and automation. In this article, we'll explore the various methods to find the last row in Excel using VBA, along with practical examples and tips to make your coding journey smoother.
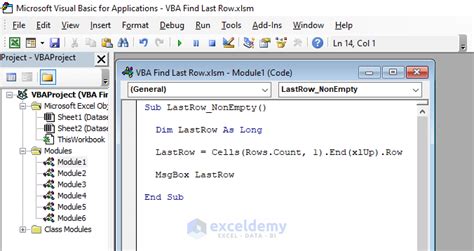
Why Find the Last Row in Excel?
Before diving into the VBA code, let's quickly discuss why finding the last row is important:
- Data Analysis: When working with large datasets, identifying the last row helps you understand the scope of your data and make informed decisions.
- Automation: In VBA automation, finding the last row is crucial for tasks like data formatting, validation, and reporting.
- Error Prevention: By knowing the last row, you can avoid errors like overwriting data or referencing non-existent cells.
Method 1: Using the Cells.Find
Method
One of the most common methods to find the last row is by using the Cells.Find
method. This approach is simple and efficient:
Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells.Find(What:="*", SearchOrder:=xlRows, SearchDirection:=xlPrevious).Row
MsgBox "The last row is: " & lastRow
End Sub
In this code, we're using the Cells.Find
method to search for any non-blank cell (What:="*"
). The SearchOrder
is set to xlRows
to search by row, and SearchDirection
is set to xlPrevious
to find the last row.
Method 2: Using the Range.End
Property
Another popular method is using the Range.End
property. This approach is useful when you know the starting cell:
Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim startCell As Range
Set startCell = ws.Range("A1")
Dim lastRow As Long
lastRow = startCell.End(xlDown).Row
MsgBox "The last row is: " & lastRow
End Sub
In this code, we're setting the startCell
to cell A1, and then using the End
property with xlDown
to find the last row.
Method 3: Using the UsedRange.Rows.Count
Property
If you want to find the last row without using any specific cell or range, you can use the UsedRange.Rows.Count
property:
Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim lastRow As Long
lastRow = ws.UsedRange.Rows.Count
MsgBox "The last row is: " & lastRow
End Sub
This method is useful when you're dealing with a large dataset and want a quick way to find the last row.
Method 4: Using the SpecialCells
Method
You can also use the SpecialCells
method to find the last row:
Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells.SpecialCells(xlCellTypeLastCell).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is useful when you want to find the last row in a specific range or worksheet.
Best Practices and Tips
When finding the last row in Excel with VBA, keep the following best practices and tips in mind:
- Use the
ActiveWorkbook
orThisWorkbook
objects to reference the current workbook, rather than usingWorkbooks("your_workbook_name")
. - Use the
ActiveSheet
orws
objects to reference the current worksheet, rather than usingWorksheets("your_worksheet_name")
. - Test your code with different datasets and scenarios to ensure it works as expected.
- Use the
On Error Resume Next
statement to handle errors and exceptions. - Keep your code organized by using modules, procedures, and comments.
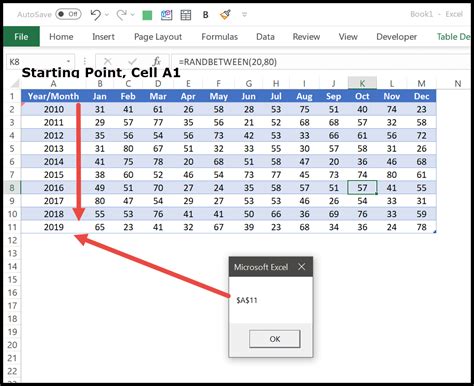
Conclusion
Finding the last row in Excel with VBA is a fundamental skill for any Excel developer. By using one of the methods outlined in this article, you can quickly and efficiently find the last row in your dataset. Remember to follow best practices and tips to ensure your code is robust and reliable.
Gallery of Finding Last Row in Excel with VBA
Finding Last Row in Excel with VBA Image Gallery
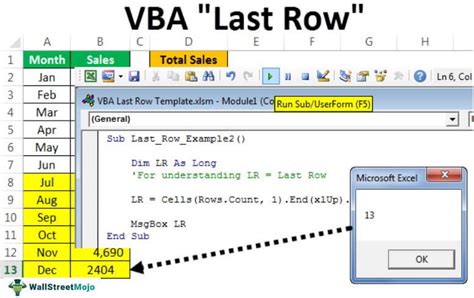
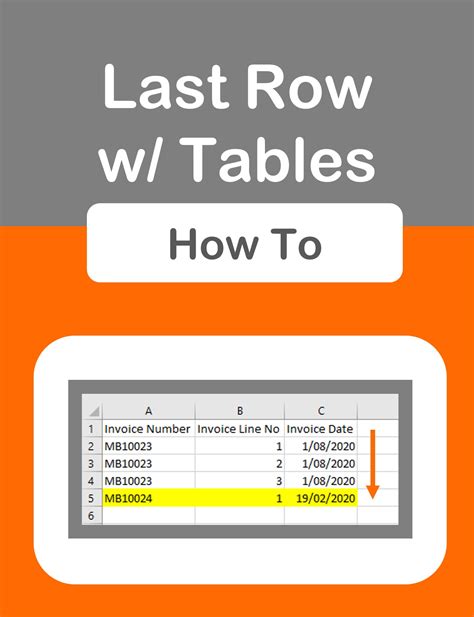
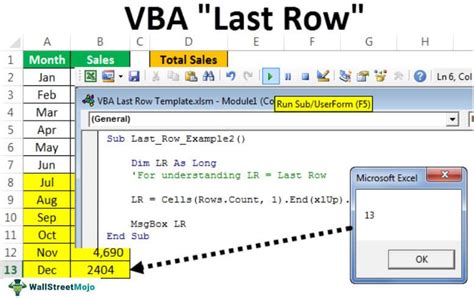
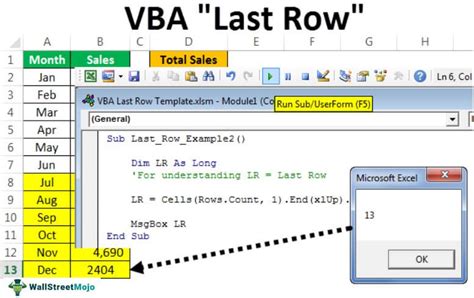
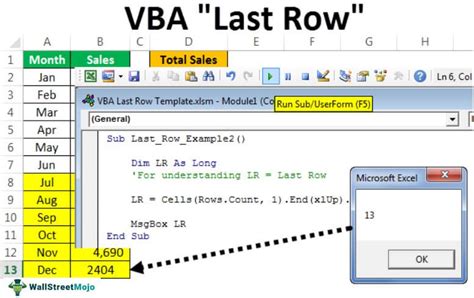
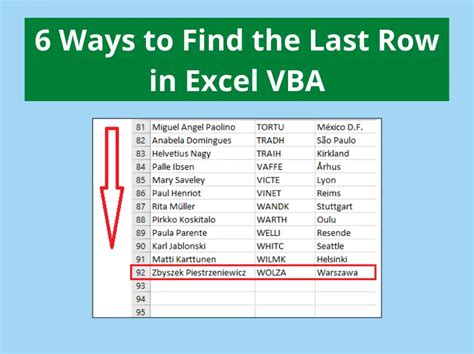
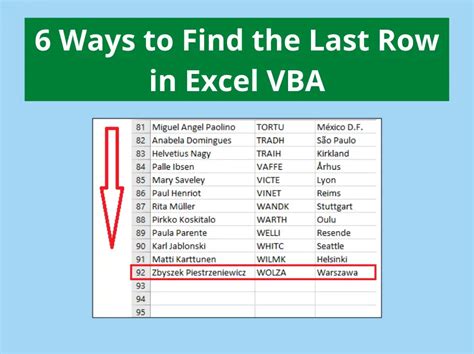
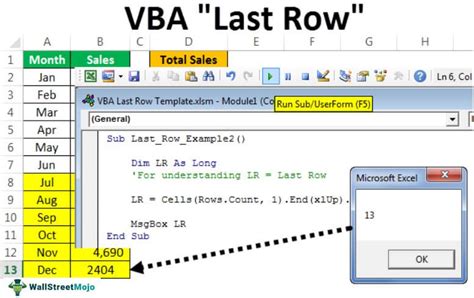
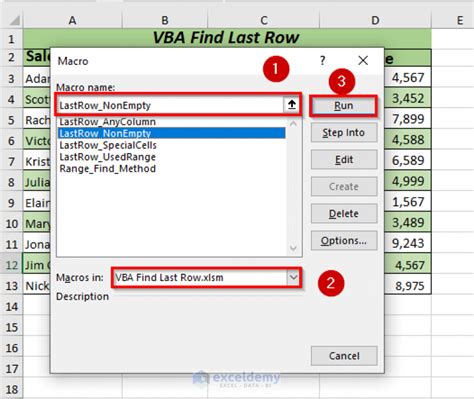
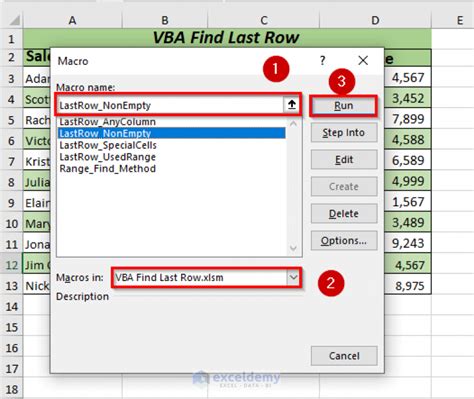
Frequently Asked Questions
Q: What is the best method to find the last row in Excel with VBA?
A: The best method depends on your specific needs and scenario. However, the Cells.Find
method is a popular and efficient approach.
Q: How do I find the last row in a specific range or worksheet?
A: You can use the Range.End
property or the SpecialCells
method to find the last row in a specific range or worksheet.
Q: What are some best practices for finding the last row in Excel with VBA?
A: Use the ActiveWorkbook
or ThisWorkbook
objects, use the ActiveSheet
or ws
objects, test your code, and keep your code organized.