Renaming Excel sheet names can be a tedious task, especially when dealing with a large number of worksheets. Fortunately, VBA (Visual Basic for Applications) provides a solution to automate this process. In this article, we will explore five ways to rename Excel sheet names using VBA.
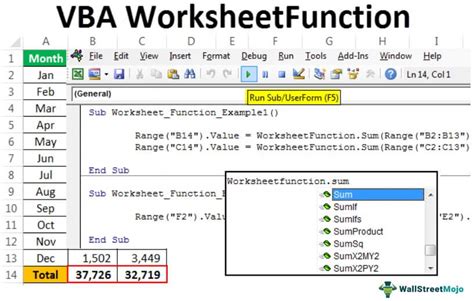
Why Rename Excel Sheet Names with VBA?
Renaming Excel sheet names manually can be time-consuming and prone to errors. By using VBA, you can automate the process, saving time and reducing the risk of mistakes. Additionally, VBA allows you to perform complex renaming tasks that would be difficult or impossible to achieve manually.
Method 1: Rename a Single Sheet with VBA
To rename a single sheet with VBA, you can use the following code:
Sub RenameSheet()
Sheets("OldSheetName").Name = "NewSheetName"
End Sub
Replace "OldSheetName" with the current name of the sheet you want to rename, and "NewSheetName" with the desired new name.
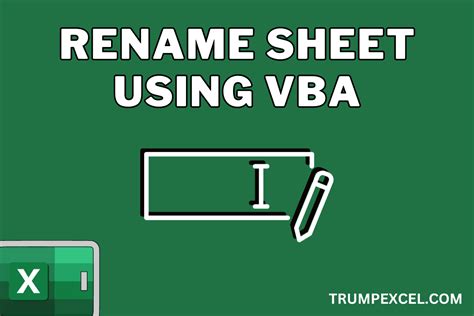
How to Use This Code
- Open the Visual Basic Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, insert a new module by clicking Insert > Module.
- Paste the code into the module.
- Replace "OldSheetName" and "NewSheetName" with the desired names.
- Click Run or press F5 to execute the code.
Method 2: Rename Multiple Sheets with VBA
To rename multiple sheets with VBA, you can use a loop to iterate through the sheets and rename them individually. Here's an example code:
Sub RenameMultipleSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = "NewName" & ws.Index
Next ws
End Sub
This code renames each sheet to "NewName" followed by the sheet's index number (e.g., "NewName1", "NewName2", etc.).
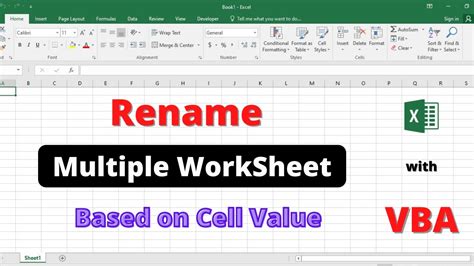
How to Use This Code
- Open the Visual Basic Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, insert a new module by clicking Insert > Module.
- Paste the code into the module.
- Replace "NewName" with the desired prefix for the new sheet names.
- Click Run or press F5 to execute the code.
Method 3: Rename Sheets Based on Cell Values with VBA
To rename sheets based on cell values with VBA, you can use the following code:
Sub RenameSheetsBasedOnCellValues()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = ws.Range("A1").Value
Next ws
End Sub
This code renames each sheet to the value in cell A1 of that sheet.
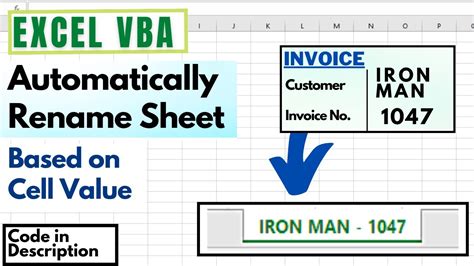
How to Use This Code
- Open the Visual Basic Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, insert a new module by clicking Insert > Module.
- Paste the code into the module.
- Make sure the cell values in column A of each sheet are the desired new names.
- Click Run or press F5 to execute the code.
Method 4: Rename Sheets Using an Array with VBA
To rename sheets using an array with VBA, you can use the following code:
Sub RenameSheetsUsingArray()
Dim oldNames() As Variant
Dim newNames() As Variant
Dim i As Integer
oldNames = Array("OldSheet1", "OldSheet2", "OldSheet3")
newNames = Array("NewSheet1", "NewSheet2", "NewSheet3")
For i = 0 To UBound(oldNames)
ThisWorkbook.Worksheets(oldNames(i)).Name = newNames(i)
Next i
End Sub
This code renames the sheets listed in the oldNames
array to the corresponding names in the newNames
array.
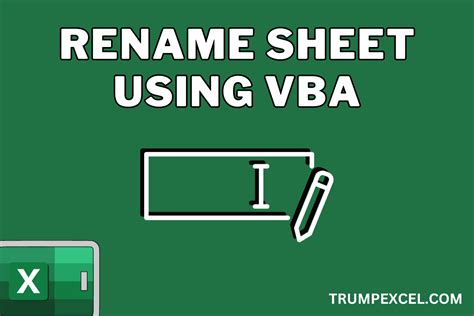
How to Use This Code
- Open the Visual Basic Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, insert a new module by clicking Insert > Module.
- Paste the code into the module.
- Replace the
oldNames
andnewNames
arrays with the desired names. - Click Run or press F5 to execute the code.
Method 5: Rename Sheets Using a UserForm with VBA
To rename sheets using a UserForm with VBA, you can create a UserForm with text boxes for the old and new sheet names, and a button to execute the renaming process. Here's an example code:
Sub RenameSheetsUsingUserForm()
Dim oldName As String
Dim newName As String
oldName = Me.OldNameTextBox.Value
newName = Me.NewNameTextBox.Value
ThisWorkbook.Worksheets(oldName).Name = newName
End Sub
This code renames the sheet with the name entered in the OldNameTextBox
to the name entered in the NewNameTextBox
.
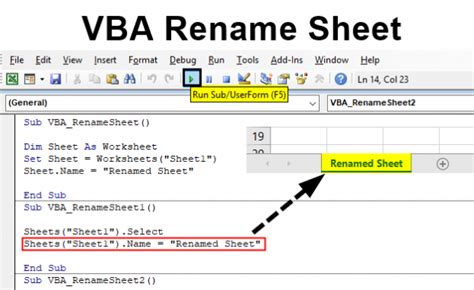
How to Use This Code
- Open the Visual Basic Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, insert a new UserForm by clicking Insert > UserForm.
- Add two text boxes and a button to the UserForm.
- Paste the code into the UserForm's code module.
- Replace the
OldNameTextBox
andNewNameTextBox
with the actual names of the text boxes. - Click Run or press F5 to execute the code.
Rename Excel Sheet Names with VBA Image Gallery
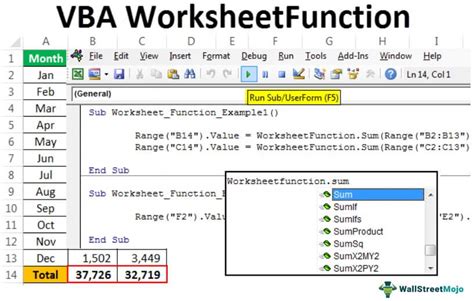
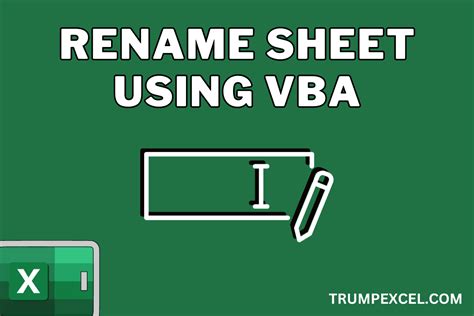
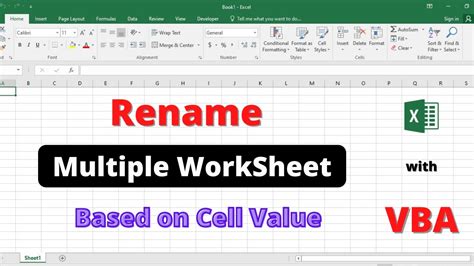
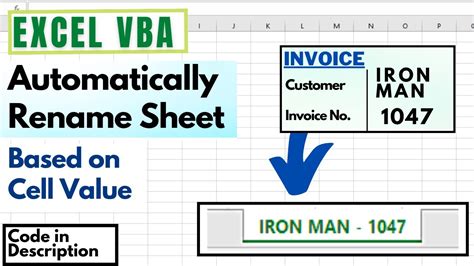
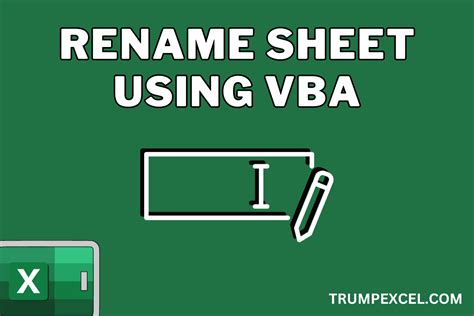
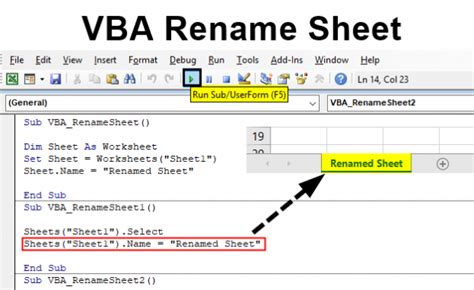
We hope this article has helped you learn how to rename Excel sheet names with VBA. Whether you need to rename a single sheet or multiple sheets, these methods will save you time and effort. Don't forget to explore other VBA tutorials on our website to improve your Excel skills!