Intro
In Microsoft Excel, adjusting column widths is a common task that can be performed manually or through VBA (Visual Basic for Applications) programming. While manual adjustments are straightforward, using VBA can automate the process, especially when dealing with large datasets or repetitive tasks. Here, we'll explore five different ways to adjust column widths in Excel VBA, catering to various scenarios and preferences.
Why Adjust Column Widths in Excel?
Before diving into the VBA methods, it's essential to understand why adjusting column widths is important. Properly sized columns enhance the readability of your data, making it easier to analyze and work with. Columns that are too narrow can truncate data, leading to potential errors or misunderstandings, while columns that are too wide can waste space, making your worksheet less efficient.
Method 1: AutoFit Columns
The most straightforward method to adjust column widths in VBA is to use the AutoFit feature, which automatically adjusts the column width to fit the content.
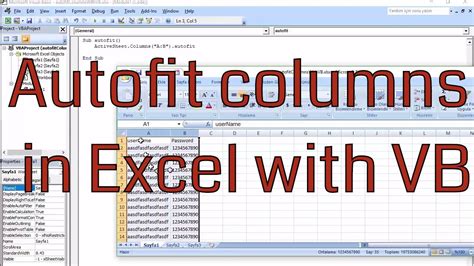
Sub AutoFitColumns()
Range("A1").EntireColumn.AutoFit
End Sub
This code adjusts the width of the entire column A to fit the content of the cells within that column.
Method 2: Specifying Column Width
Sometimes, you might want to set a specific width for a column instead of relying on AutoFit. This can be useful for creating uniformity across your worksheet or for preparing data for printing.
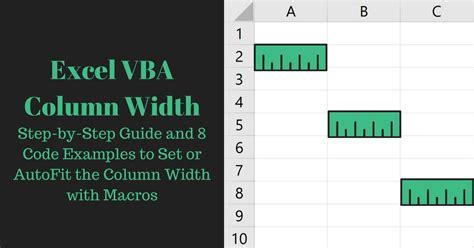
Sub SetColumnWidth()
Columns("A").ColumnWidth = 20
End Sub
This code sets the width of column A to 20 points.
Method 3: Adjusting Column Width Based on Maximum Content Length
For more dynamic control, you can adjust column widths based on the maximum content length found within the column.
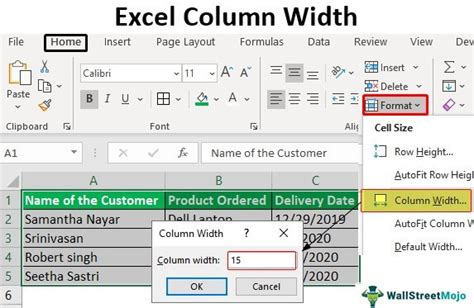
Sub AdjustColumnWidth()
Dim maxLength As Long
Dim cell As Range
'Assuming we're checking column A
For Each cell In Range("A:A")
If Len(cell.Value) > maxLength Then
maxLength = Len(cell.Value)
End If
Next cell
Columns("A").ColumnWidth = maxLength + 2 'Adding 2 for padding
End Sub
This code finds the maximum content length in column A and sets the column width accordingly, adding a couple of points for padding.
Method 4: Using a Loop to Adjust Multiple Columns
When you need to adjust the widths of multiple columns, using a loop can streamline your VBA code.
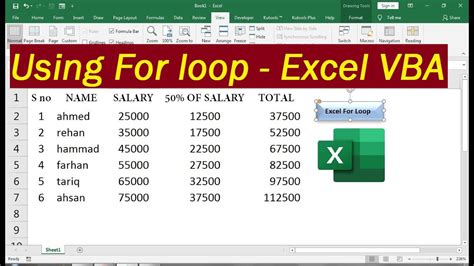
Sub AdjustMultipleColumns()
Dim col As Range
For Each col In Range("A:E").Columns 'Adjust columns A through E
col.AutoFit
Next col
End Sub
This code uses a loop to AutoFit columns A through E.
Method 5: Combining AutoFit with Conditional Formatting
For a more advanced approach, you can combine AutoFit with conditional formatting rules to dynamically adjust column widths based on specific conditions.
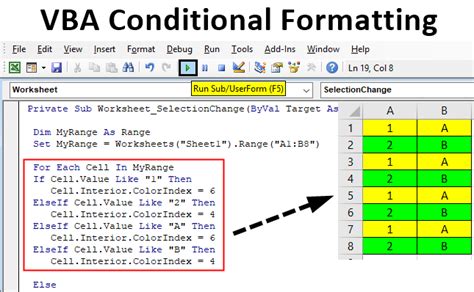
Sub AutoFitConditionalFormatting()
'Assuming a condition where cells in column A with values greater than 100 should have wider columns
If Range("A1").Value > 100 Then
Range("A:A").AutoFit
Else
Columns("A").ColumnWidth = 10 'Default width
End If
End Sub
This code checks the value in cell A1 and adjusts the width of column A accordingly.
Gallery of Excel VBA Column Width Adjustment
Excel VBA Column Width Adjustment Gallery
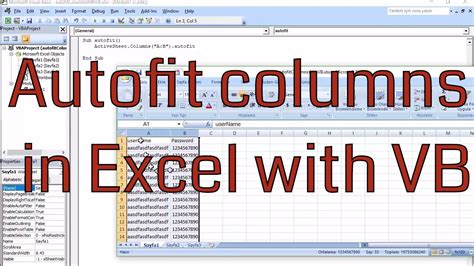
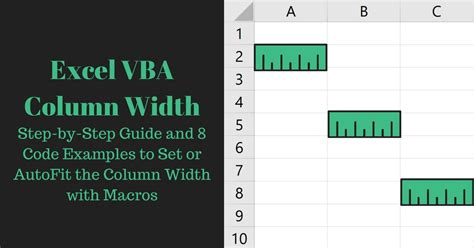
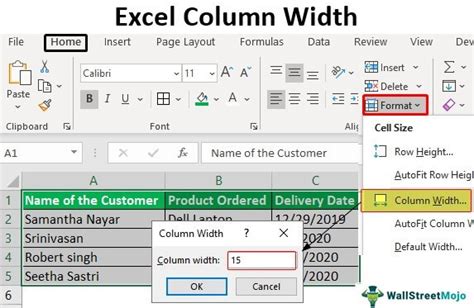
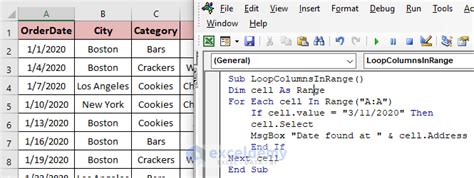
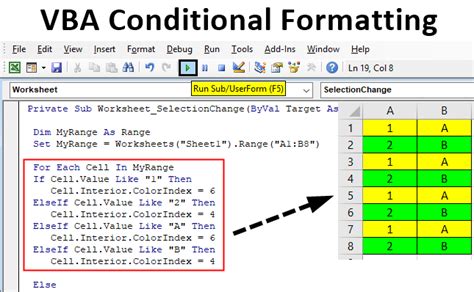
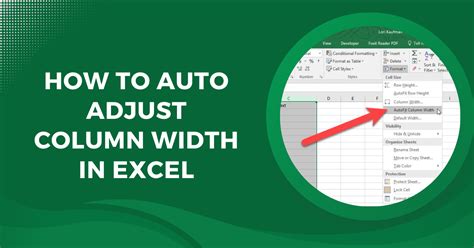
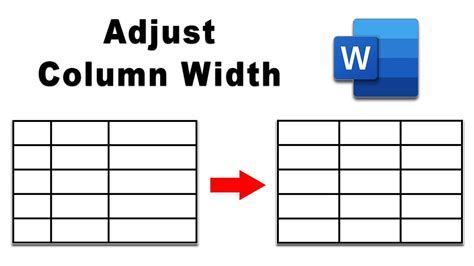
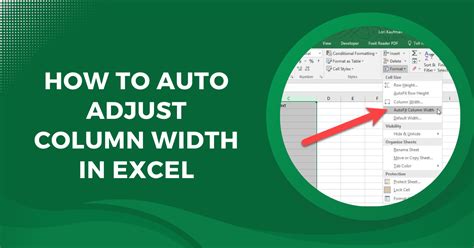
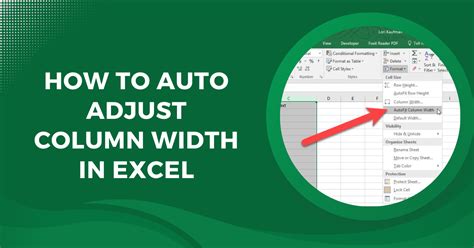
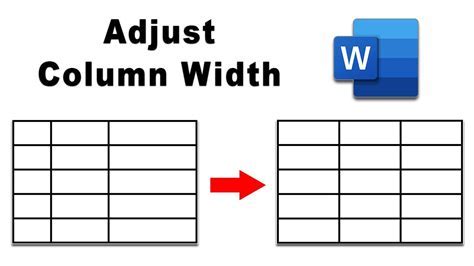
Conclusion: Streamlining Excel with VBA
Adjusting column widths in Excel is a fundamental task that can significantly enhance the usability and readability of your worksheets. By leveraging VBA, you can automate and customize this process, adapting it to your specific needs and workflows. Whether you prefer straightforward AutoFit methods or more complex conditional adjustments, the options outlined above offer a solid foundation for streamlining your Excel workflow.
Get Involved: Share Your Excel VBA Tips and Tricks
Have you developed innovative VBA solutions for column width adjustment or other Excel tasks? Share your insights and code snippets in the comments below. Let's collaborate to create a more efficient and automated Excel experience for everyone.