Intro
Master Excel VBA calculations without tables! Discover 5 efficient methods to perform calculations in Excel VBA, including using variables, loops, and formulas. Learn how to calculate averages, sums, and more using VBA code. Improve your automation skills and boost productivity with these expert-approved techniques.
Excel VBA is a powerful tool that allows users to automate tasks, create custom interfaces, and perform complex calculations. One of the most common uses of Excel VBA is to perform calculations without using tables. In this article, we will explore five ways to calculate in Excel VBA without using tables.
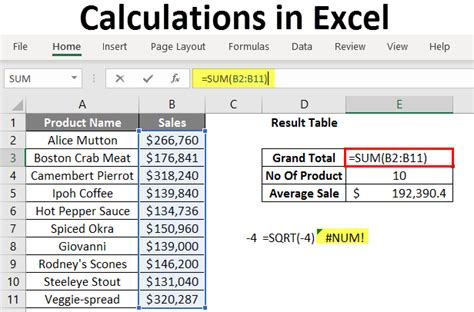
1. Using Variables and Operators
One way to calculate in Excel VBA without using tables is to use variables and operators. Variables are used to store values, and operators are used to perform mathematical operations on those values. For example, you can use the "+" operator to add two numbers together, or the "*" operator to multiply two numbers together.
Here is an example of how to use variables and operators to calculate the sum of two numbers:
Sub CalculateSum()
Dim num1 As Integer
Dim num2 As Integer
Dim sum As Integer
num1 = 10
num2 = 20
sum = num1 + num2
MsgBox "The sum is: " & sum
End Sub
In this example, we define three variables: num1
, num2
, and sum
. We assign the values 10 and 20 to num1
and num2
, respectively. Then, we use the "+" operator to add num1
and num2
together and store the result in the sum
variable. Finally, we use the MsgBox
function to display the result.
2. Using VBA Functions
Another way to calculate in Excel VBA without using tables is to use VBA functions. VBA functions are pre-built functions that perform specific calculations, such as calculating the sum of a range of numbers or calculating the average of a set of numbers.
Here is an example of how to use the WorksheetFunction.Sum
function to calculate the sum of a range of numbers:
Sub CalculateSum()
Dim sum As Double
sum = WorksheetFunction.Sum(10, 20, 30)
MsgBox "The sum is: " & sum
End Sub
In this example, we use the WorksheetFunction.Sum
function to calculate the sum of the numbers 10, 20, and 30. The result is stored in the sum
variable, which is then displayed using the MsgBox
function.
3. Using VBA Formulas
You can also use VBA formulas to perform calculations without using tables. VBA formulas are similar to Excel formulas, but they are used in VBA code instead of in a worksheet.
Here is an example of how to use a VBA formula to calculate the area of a rectangle:
Sub CalculateArea()
Dim length As Double
Dim width As Double
Dim area As Double
length = 10
width = 20
area = length * width
MsgBox "The area is: " & area
End Sub
In this example, we use a VBA formula to calculate the area of a rectangle with a length of 10 and a width of 20. The result is stored in the area
variable, which is then displayed using the MsgBox
function.
4. Using Excel Worksheet Functions
You can also use Excel worksheet functions in VBA code to perform calculations without using tables. Excel worksheet functions are functions that are built into Excel, such as the SUM
function or the AVERAGE
function.
Here is an example of how to use the Application.Sum
function to calculate the sum of a range of numbers:
Sub CalculateSum()
Dim sum As Double
sum = Application.Sum(Range("A1:A10"))
MsgBox "The sum is: " & sum
End Sub
In this example, we use the Application.Sum
function to calculate the sum of the range of numbers in cells A1 through A10. The result is stored in the sum
variable, which is then displayed using the MsgBox
function.
5. Using VBA User-Defined Functions (UDFs)
Finally, you can use VBA user-defined functions (UDFs) to perform calculations without using tables. UDFs are custom functions that you create in VBA code, and they can be used to perform complex calculations.
Here is an example of how to create a UDF to calculate the sum of two numbers:
Function AddNumbers(x As Double, y As Double) As Double
AddNumbers = x + y
End Function
In this example, we create a UDF called AddNumbers
that takes two arguments, x
and y
. The function calculates the sum of x
and y
and returns the result.
To use the UDF, we can call it from another VBA procedure, like this:
Sub CalculateSum()
Dim sum As Double
sum = AddNumbers(10, 20)
MsgBox "The sum is: " & sum
End Sub
In this example, we call the AddNumbers
UDF and pass it the arguments 10 and 20. The result is stored in the sum
variable, which is then displayed using the MsgBox
function.
Excel VBA Calculations Gallery
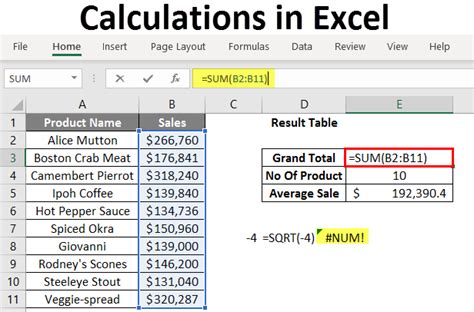
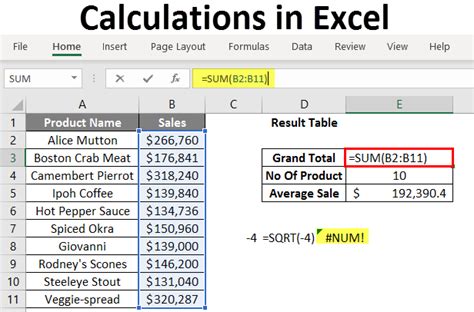
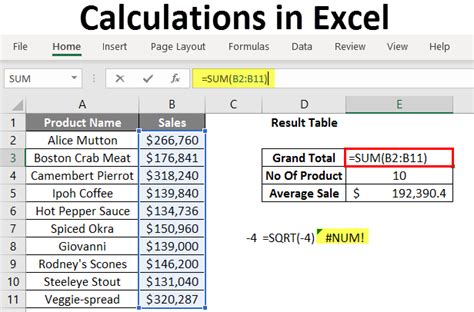
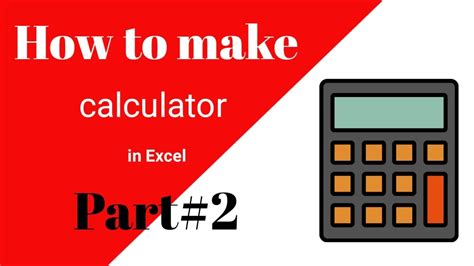
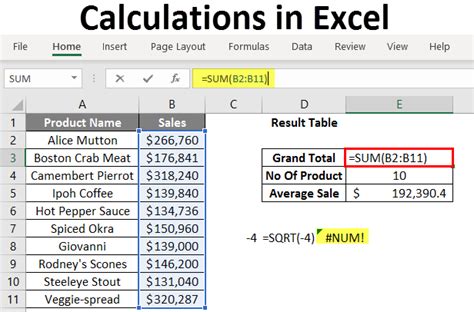
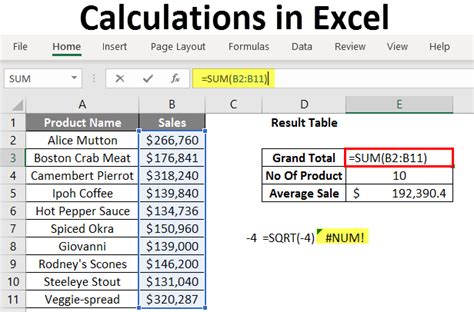
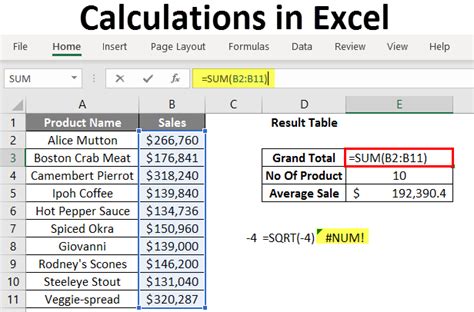
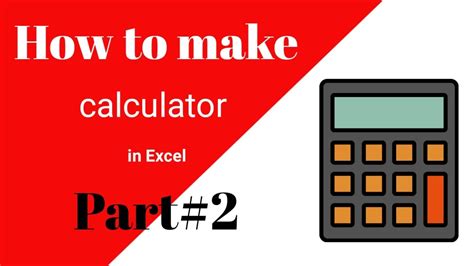
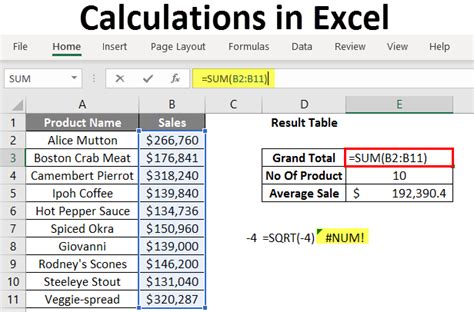
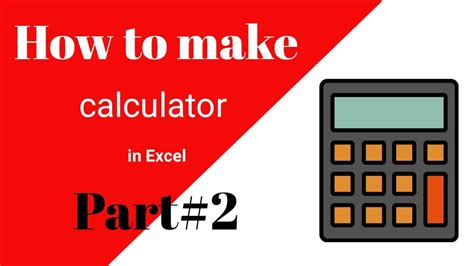
In conclusion, there are many ways to calculate in Excel VBA without using tables. By using variables and operators, VBA functions, VBA formulas, Excel worksheet functions, and VBA user-defined functions, you can perform complex calculations and automate tasks in Excel. We hope this article has been helpful in demonstrating these different methods. Do you have any experience with Excel VBA calculations? Share your thoughts and examples in the comments below!