Intro
Mastering Excel VBA can be a daunting task, especially for beginners. With its vast array of features and capabilities, it's easy to get lost in the world of macros, modules, and subroutines. However, with the right guidance, you can unlock the full potential of Excel VBA and become a productivity powerhouse. In this article, we'll cover the top 10 Excel VBA cheat sheet essentials that every user should know.
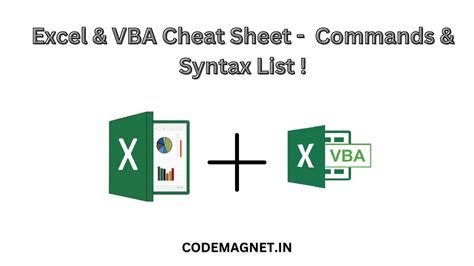
Understanding the Basics of Excel VBA
Before diving into the advanced topics, it's essential to understand the basics of Excel VBA. This includes:
- What is VBA?: VBA stands for Visual Basic for Applications, a programming language developed by Microsoft.
- Why use VBA?: VBA allows users to automate tasks, create custom tools, and interact with other Microsoft Office applications.
- How to access VBA: Press Alt + F11 or navigate to Developer > Visual Basic in the Excel ribbon.
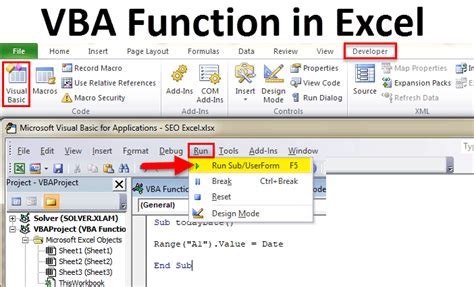
1. Declaring Variables
Declaring variables is a fundamental concept in VBA programming. Variables store values that can be used throughout your code.
- Dim: Use the Dim statement to declare a variable, e.g.,
Dim myVariable As Integer
. - Data Types: Common data types include Integer, String, Date, and Boolean.
- Scope: Variables can be declared at the module or procedure level.
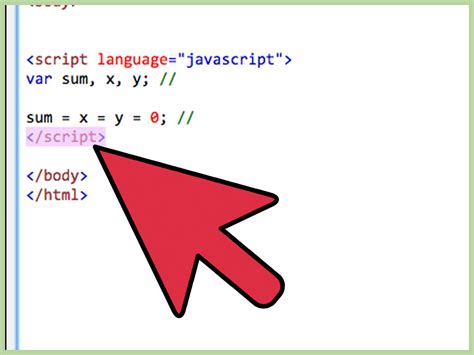
2. Working with Worksheets and Ranges
Worksheets and ranges are essential components of Excel VBA.
- Worksheets: Use the
Worksheets
collection to access and manipulate worksheets, e.g.,Worksheets("Sheet1").Range("A1").Value = "Hello"
. - Ranges: Use the
Range
object to interact with cells, e.g.,Range("A1:B2").Value = "Hello World"
.
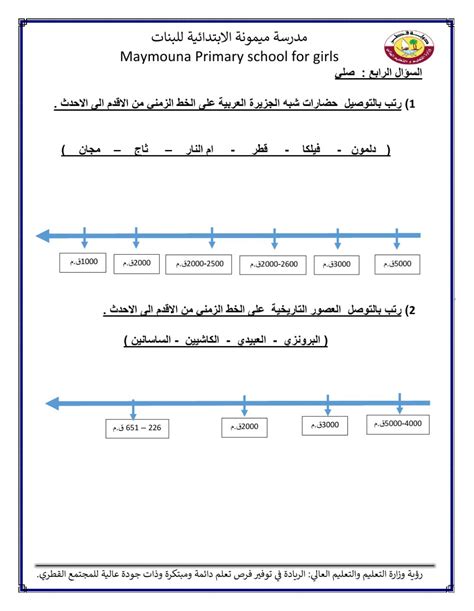
Controlling Flow and Making Decisions
Flow control and decision-making are crucial aspects of VBA programming.
- If Statements: Use the
If
statement to make decisions, e.g.,If myVariable > 10 Then MsgBox "Greater than 10"
. - Select Case: Use the
Select Case
statement to evaluate multiple conditions, e.g.,Select Case myVariable: Case 1: MsgBox "One": Case 2: MsgBox "Two": End Select
. - Loops: Use loops to iterate through code, e.g.,
For i = 1 To 10: MsgBox i: Next i
.
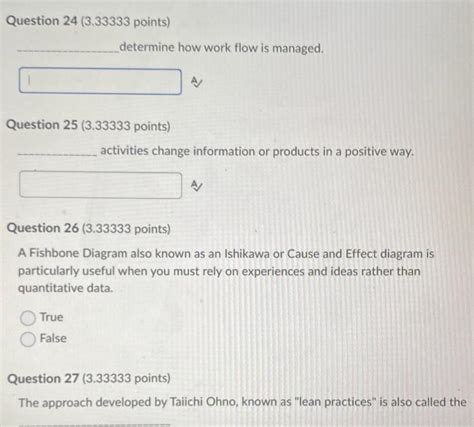
3. Working with Arrays
Arrays are powerful data structures in VBA.
- Declaring Arrays: Use the
Dim
statement to declare an array, e.g.,Dim myArray(1 To 10) As Integer
. - Assigning Values: Use the
=
operator to assign values to an array, e.g.,myArray(1) = 10
. - Iterating through Arrays: Use loops to iterate through arrays, e.g.,
For i = 1 To 10: MsgBox myArray(i): Next i
.
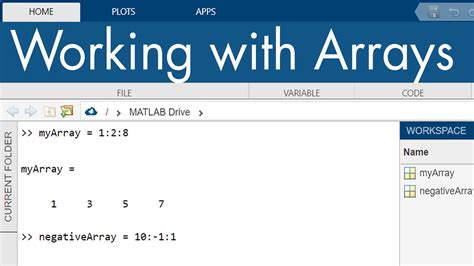
4. Creating Custom Functions
Custom functions allow you to extend the functionality of Excel.
- Function Syntax: Use the
Function
keyword to declare a custom function, e.g.,Function MyFunction(x As Integer) As Integer
. - Function Arguments: Use the
(
and)
characters to enclose function arguments, e.g.,MyFunction(10)
. - Returning Values: Use the
Return
statement to return values from a function, e.g.,MyFunction = x * 2
.
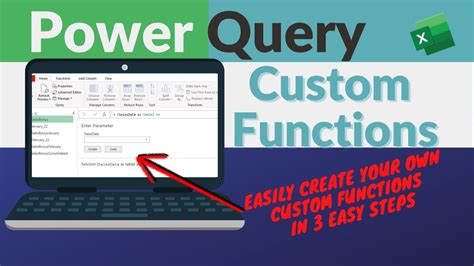
Interacting with Other Applications
VBA allows you to interact with other Microsoft Office applications.
- Creating Objects: Use the
CreateObject
function to create objects from other applications, e.g.,Set myWordApp = CreateObject("Word.Application")
. - Using Late Binding: Use late binding to interact with objects without declaring them, e.g.,
myWordApp.Documents.Add
. - Using Early Binding: Use early binding to declare objects and interact with them, e.g.,
Dim myWordApp As Word.Application: Set myWordApp = New Word.Application
.
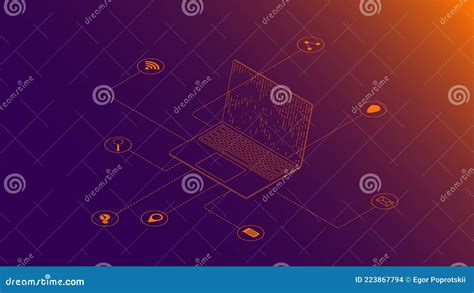
5. Debugging and Error Handling
Debugging and error handling are essential skills for any VBA developer.
- Debugging Tools: Use the VBA debugger to step through code, set breakpoints, and inspect variables.
- Error Handling: Use the
On Error
statement to handle errors, e.g.,On Error GoTo ErrorHandler
. - Error Messages: Use the
Err
object to access error messages, e.g.,MsgBox Err.Description
.
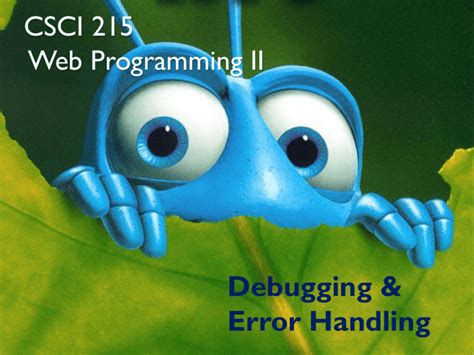
6. Optimizing Performance
Optimizing performance is crucial for large-scale VBA applications.
- Optimizing Loops: Use loops efficiently by minimizing iterations and using
Do While
instead ofFor
. - Avoiding Select Statements: Use
If
statements instead ofSelect
statements for better performance. - Minimizing Object Creation: Minimize object creation by reusing objects instead of creating new ones.
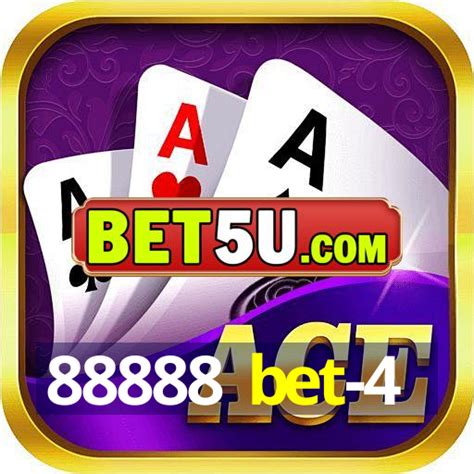
Best Practices and Tips
Here are some best practices and tips for VBA development:
- Use Meaningful Variable Names: Use descriptive variable names to improve code readability.
- Use Comments: Use comments to explain complex code and improve maintainability.
- Test Your Code: Test your code thoroughly to ensure it works as expected.
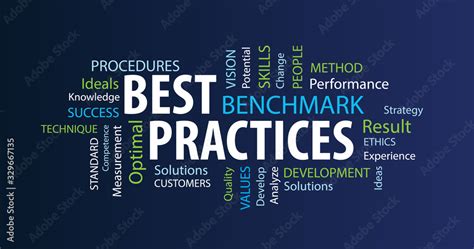
7. Working with Excel Events
Excel events allow you to respond to user interactions and system events.
- Worksheet Events: Use worksheet events to respond to user interactions, e.g.,
Worksheet_SelectionChange
. - Workbook Events: Use workbook events to respond to system events, e.g.,
Workbook_Open
.
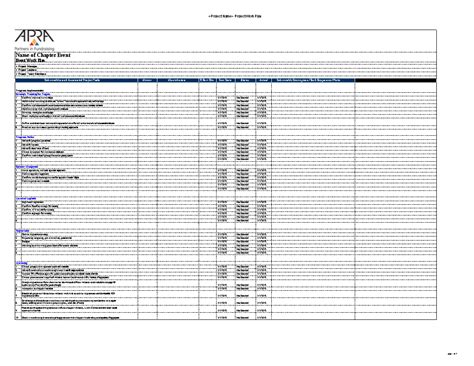
8. Creating Custom Tools and Add-ins
Custom tools and add-ins allow you to extend the functionality of Excel.
- Creating Custom Tools: Use VBA to create custom tools, e.g.,
CommandBars
. - Creating Add-ins: Use VBA to create add-ins, e.g.,
Add-ins
.
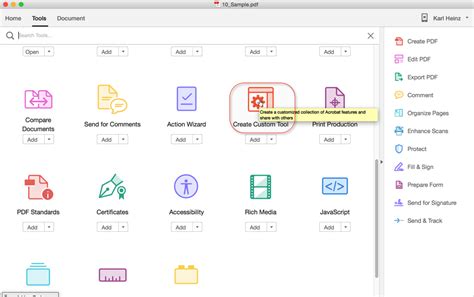
9. Working with PivotTables and Charts
PivotTables and charts are powerful data visualization tools in Excel.
- Creating PivotTables: Use VBA to create PivotTables, e.g.,
PivotTables.Add
. - Working with Charts: Use VBA to work with charts, e.g.,
Charts.Add
.
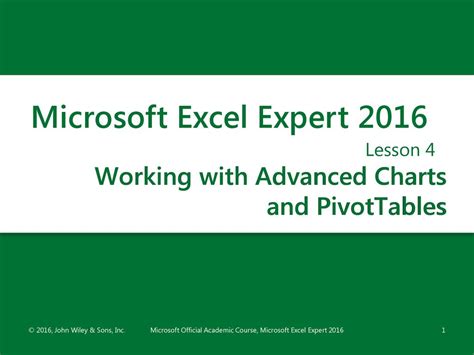
10. Mastering VBA Security
VBA security is crucial to prevent malicious code from running.
- Understanding VBA Security: Understand the VBA security model, e.g.,
Trust Center
. - Setting Security Levels: Set security levels, e.g.,
High
,Medium
, orLow
. - Digitally Signing Macros: Digitally sign macros to ensure authenticity.
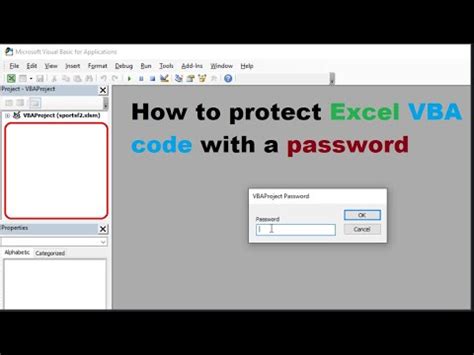
Excel VBA Cheat Sheet Essentials Gallery
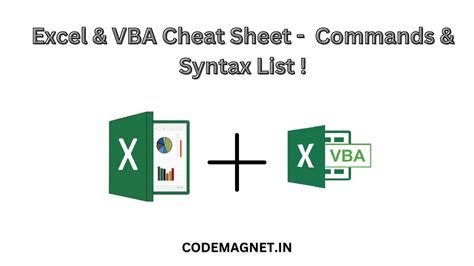
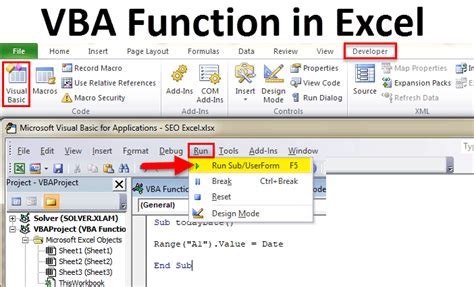
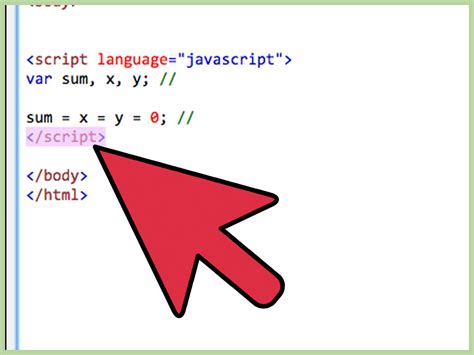
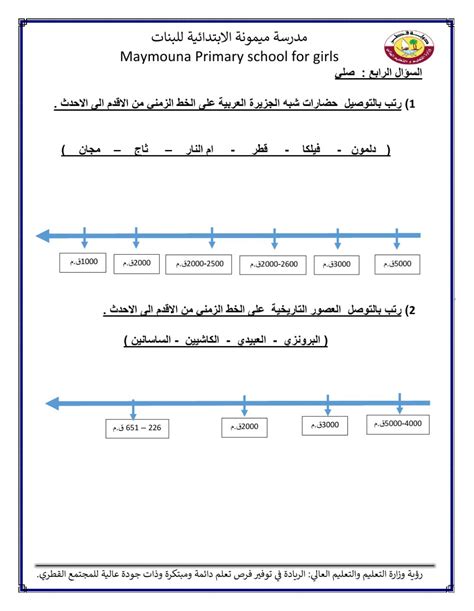
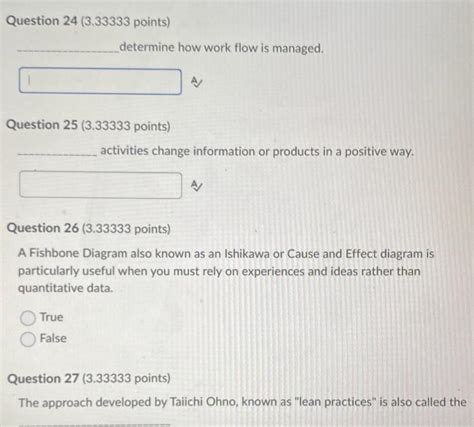
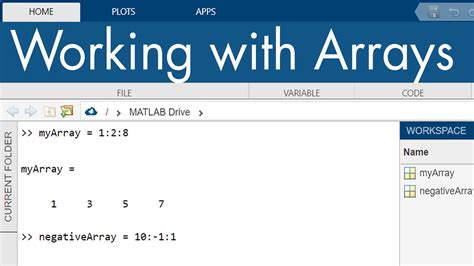
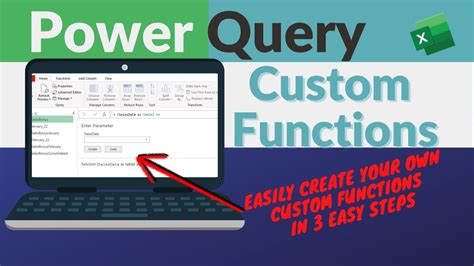
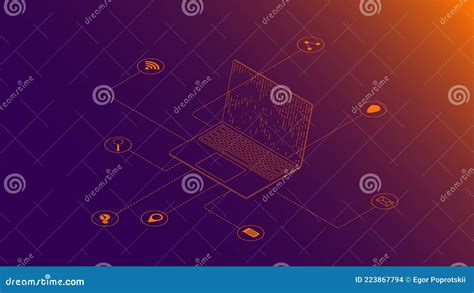
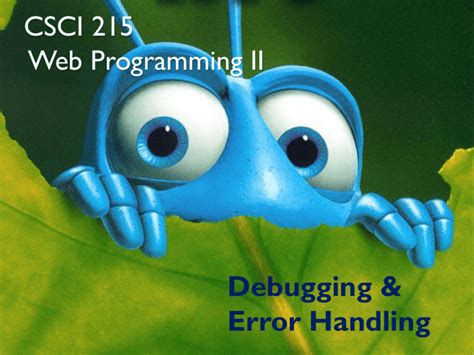
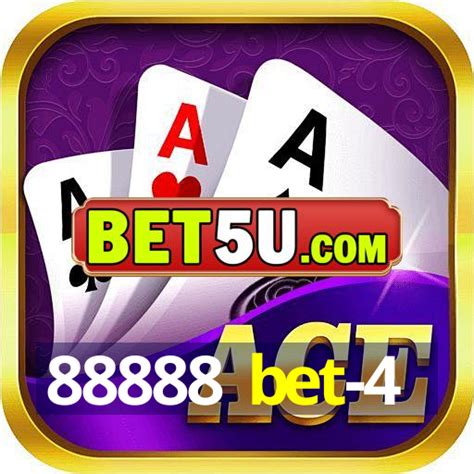
By mastering these top 10 Excel VBA cheat sheet essentials, you'll be well on your way to becoming a VBA expert. Remember to practice regularly and explore more advanced topics to further enhance your skills. Happy coding!