Intro
Discover how to easily check if a file exists in Excel VBA with our step-by-step guide. Learn to use the Dir() function, FileSystemObject, and error handling to verify file existence. Master file management and automate tasks with Excel VBA macros. Boost productivity and accuracy with this essential skill.
Excel VBA Check If File Exists Easily
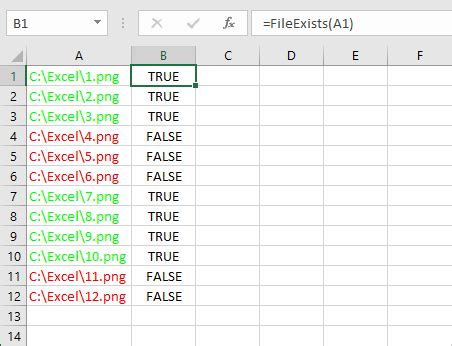
When working with Excel VBA, it's often necessary to check if a file exists before attempting to open or manipulate it. This can help prevent errors and ensure that your code runs smoothly. In this article, we'll explore the different ways to check if a file exists in Excel VBA.
The Importance of Checking File Existence
Checking if a file exists is crucial in many VBA applications, especially when working with external files or network paths. Without proper checks, your code may crash or produce unexpected results, leading to frustration and wasted time. By incorporating file existence checks, you can:
- Prevent errors and crashes
- Improve code reliability and stability
- Enhance user experience
Methods to Check If a File Exists in Excel VBA
1. Using the Dir() Function
The Dir() function is a simple and effective way to check if a file exists. It returns the name of the file if it exists, or an empty string if it doesn't.
Sub CheckFileExists_Dir()
Dim filePath As String
filePath = "C:\Path\To\File.txt"
If Dir(filePath) <> "" Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
End Sub
2. Using the FileExists() Function
The FileExists() function is a more robust method that checks for the existence of a file. It returns True if the file exists, and False otherwise.
Sub CheckFileExists_FileExists()
Dim filePath As String
filePath = "C:\Path\To\File.txt"
If FileExists(filePath) Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
End Sub
Function FileExists(filePath As String) As Boolean
FileExists = (Len(Dir(filePath)) > 0)
End Function
3. Using the FileSystemObject
The FileSystemObject (FSO) is a powerful tool for working with files and folders. It provides a more comprehensive way to check if a file exists.
Sub CheckFileExists_FSO()
Dim fso As Object
Dim filePath As String
filePath = "C:\Path\To\File.txt"
Set fso = CreateObject("Scripting.FileSystemObject")
If fso.FileExists(filePath) Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
Set fso = Nothing
End Sub
4. Using the VBA built-in function
Excel VBA has a built-in function to check if a file exists.
Sub CheckFileExists_VBA()
Dim filePath As String
filePath = "C:\Path\To\File.txt"
If Not IsEmpty(Dir(filePath)) Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
End Sub
Comparing the Methods
Each method has its own advantages and disadvantages. The Dir() function is simple and easy to use, but it may not work correctly with long file paths. The FileExists() function is more robust and reliable, but it requires defining a separate function. The FileSystemObject provides a comprehensive way to work with files and folders, but it requires creating an object and can be slower. The VBA built-in function is simple and easy to use, but it may not work correctly with long file paths.
Best Practices for Checking File Existence
- Use the most suitable method: Choose the method that best fits your needs, considering factors like simplicity, reliability, and performance.
- Handle errors: Anticipate and handle potential errors, such as file not found or permission denied.
- Test thoroughly: Verify the correctness of your code by testing it with different file paths and scenarios.
- Optimize performance: Consider using faster methods, like the Dir() function, when working with large numbers of files.
Conclusion
Checking if a file exists is a crucial step in many Excel VBA applications. By using the right method, you can ensure that your code runs smoothly and efficiently. Remember to follow best practices and test your code thoroughly to avoid errors and improve performance.
Gallery of Excel VBA File Existence Checks
Excel VBA File Existence Checks
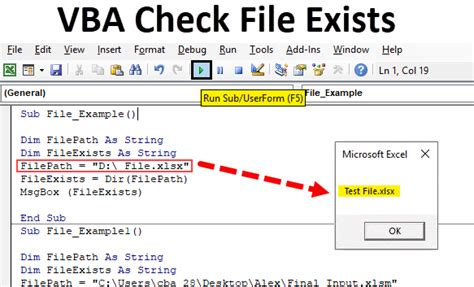
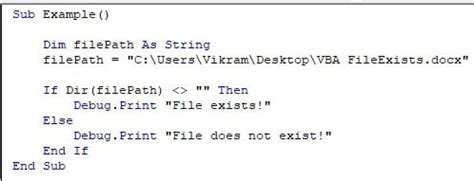
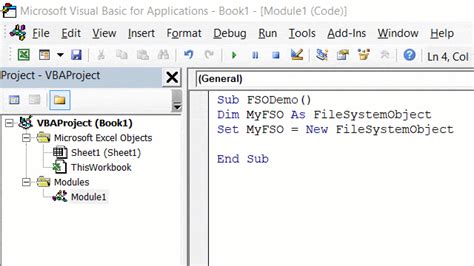
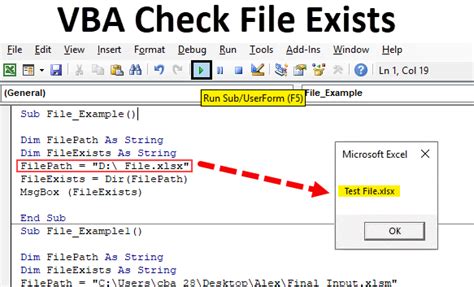
We hope you found this article helpful! What are your favorite methods for checking file existence in Excel VBA? Share your thoughts and experiences in the comments below!