Intro
Unlock the power of VBA in Excel with our easy-to-follow guide on copying ranges with VBA. Learn how to automate tasks, streamline workflows, and boost productivity with simple VBA code. Master the art of copying ranges with VBA and take your Excel skills to the next level with our expert tips and tricks.
Working with Excel ranges can be a daunting task, especially when it comes to copying and pasting data. However, with the help of VBA (Visual Basic for Applications), this process can be made easy and efficient. In this article, we will explore the different ways to copy Excel ranges using VBA and provide you with practical examples and code snippets to get you started.
Why Use VBA to Copy Excel Ranges?
Before we dive into the details of copying Excel ranges with VBA, let's first discuss why you would want to use VBA in the first place. Here are a few reasons:
- Automation: VBA allows you to automate repetitive tasks, such as copying and pasting data, which can save you a significant amount of time and effort.
- Accuracy: By using VBA, you can ensure that your data is copied accurately and consistently, reducing the risk of human error.
- Flexibility: VBA provides a wide range of tools and features that allow you to customize and tailor your code to meet your specific needs.
Basic VBA Concepts
Before we start exploring the different ways to copy Excel ranges with VBA, let's cover some basic VBA concepts that you need to know:
- Range: A range refers to a selection of cells in an Excel worksheet. You can think of it as a rectangular area of cells.
- Worksheet: A worksheet is a single sheet in an Excel workbook.
- Workbook: A workbook is the entire Excel file that contains one or more worksheets.
Copying a Single Cell Range
Let's start with a simple example of copying a single cell range using VBA. Here's an example code snippet:
Sub CopySingleCellRange()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("B1")
sourceRange.Copy targetRange
End Sub
In this example, we define two range objects, sourceRange
and targetRange
, which represent the cell ranges we want to copy from and to, respectively. We then use the Copy
method to copy the data from the source range to the target range.
Copying a Multiple Cell Range
Now that we've covered copying a single cell range, let's move on to copying a multiple cell range. Here's an example code snippet:
Sub CopyMultipleCellRange()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:B2")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("C1:D2")
sourceRange.Copy targetRange
End Sub
In this example, we define two range objects, sourceRange
and targetRange
, which represent the multiple cell ranges we want to copy from and to, respectively. We then use the Copy
method to copy the data from the source range to the target range.
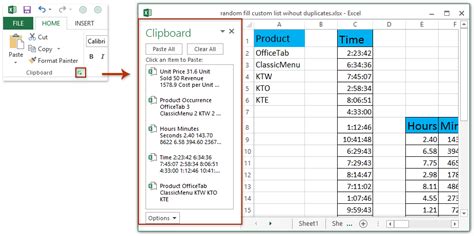
Copying a Dynamic Range
In some cases, you may need to copy a dynamic range, which means the range is not fixed and can change depending on the data. Here's an example code snippet:
Sub CopyDynamicRange()
Dim sourceRange As Range
Dim targetRange As Range
Dim lastRow As Long
lastRow = ThisWorkbook.Worksheets("Sheet1").Cells.Find("*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:A" & lastRow)
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("B1")
sourceRange.Copy targetRange
End Sub
In this example, we use the Find
method to determine the last row of data in the source range. We then define the source range and target range using the Range
method. Finally, we use the Copy
method to copy the data from the source range to the target range.
Copying a Range with Formulas
When copying a range with formulas, you need to use the PasteSpecial
method to preserve the formulas. Here's an example code snippet:
Sub CopyRangeWithFormulas()
Dim sourceRange As Range
Dim targetRange As Range
Set sourceRange = ThisWorkbook.Worksheets("Sheet1").Range("A1:B2")
Set targetRange = ThisWorkbook.Worksheets("Sheet2").Range("C1:D2")
sourceRange.Copy
targetRange.PasteSpecial xlPasteFormulas
Application.CutCopyMode = False
End Sub
In this example, we use the Copy
method to copy the source range. We then use the PasteSpecial
method to paste the formulas into the target range. Finally, we use the Application.CutCopyMode
property to clear the clipboard.
Conclusion
In this article, we've explored the different ways to copy Excel ranges using VBA. We've covered copying single cell ranges, multiple cell ranges, dynamic ranges, and ranges with formulas. By using VBA to copy Excel ranges, you can automate repetitive tasks, ensure accuracy, and customize your code to meet your specific needs.
We hope this article has been helpful in making you more productive in Excel. Do you have any questions or comments about copying Excel ranges with VBA? Share them with us in the comments below!
Excel VBA Gallery
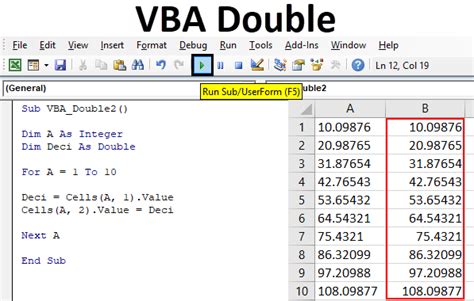
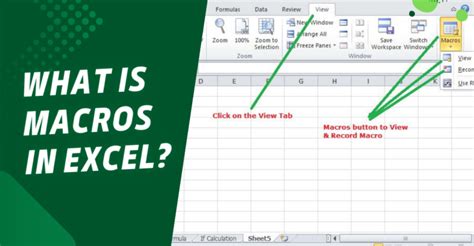
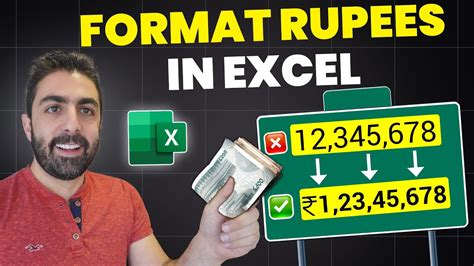
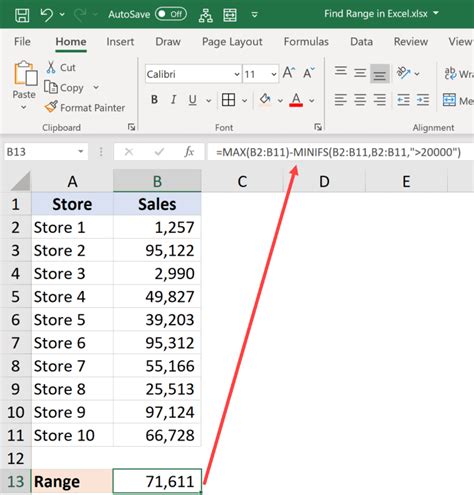
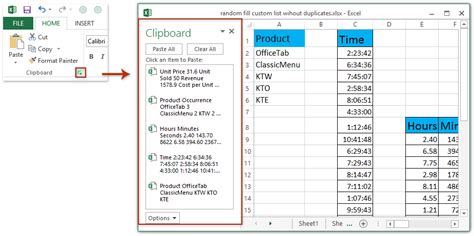
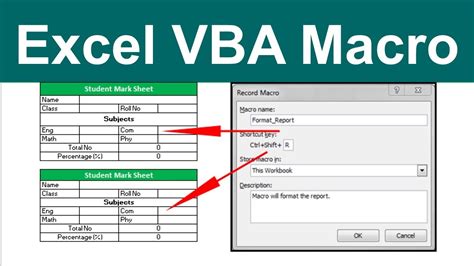
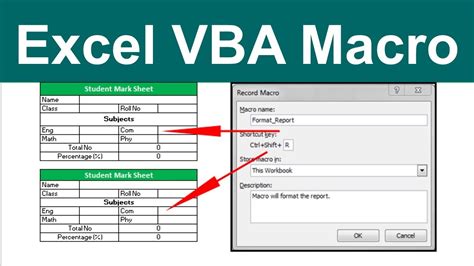
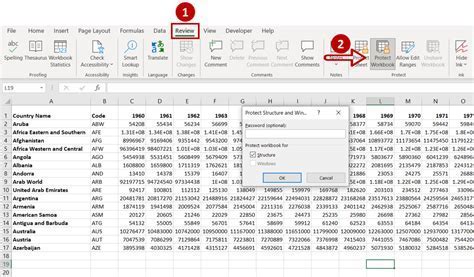
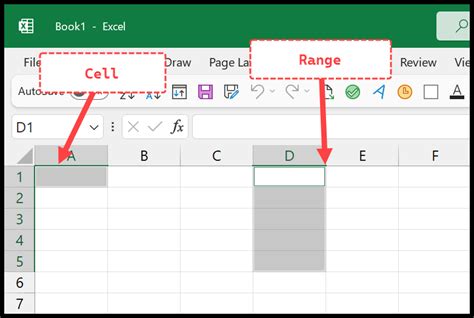
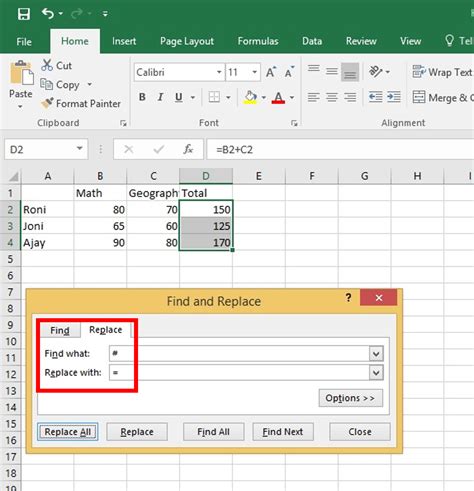