Intro
Copying a range to another sheet in Excel VBA is a common task that can be accomplished in several ways, each with its own advantages and use cases. Excel VBA provides a powerful platform for automating tasks, and understanding how to copy ranges efficiently is crucial for creating dynamic and automated spreadsheets. Here, we will explore five different methods to copy a range to another sheet using Excel VBA, providing examples and highlighting the benefits of each approach.
Understanding the Basics of Range Copying in Excel VBA
Before diving into the methods, it's essential to understand the basic syntax and objects involved in copying ranges in Excel VBA. The Range
object is fundamental in Excel VBA, representing a cell, a row, a column, a selection of cells, or even a 3D range. To copy a range, you generally need to specify the source range (Source
) and the destination range (Destination
).
' Example: Basic Range Copying
Range("A1:B2").Copy Destination:=Range("C1")
This example copies the range A1:B2 to the range starting from C1.
Method 1: Using the `Copy` Method
The most straightforward way to copy a range to another sheet is by using the Copy
method directly on a Range
object, as shown in the basic example above.
Sub CopyRangeUsingCopyMethod()
' Declare variables
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
' Set source and target sheets
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set targetSheet = ThisWorkbook.Sheets("Sheet2")
' Copy range A1:B2 from source sheet to range C1 in target sheet
sourceSheet.Range("A1:B2").Copy Destination:=targetSheet.Range("C1")
End Sub
Method 2: Using the `Worksheet.Paste` Method
Another approach is to use the Worksheet.Paste
method after copying the range. This method can be useful when you want to paste only values or formats, offering more control over what is pasted.
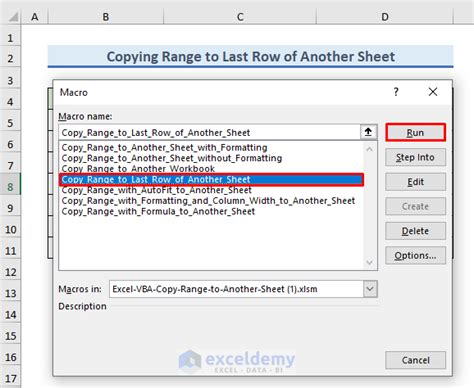
Sub CopyRangeUsingPasteMethod()
' Declare variables
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
' Set source and target sheets
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set targetSheet = ThisWorkbook.Sheets("Sheet2")
' Copy range A1:B2 from source sheet
sourceSheet.Range("A1:B2").Copy
' Paste values to range C1 in target sheet
targetSheet.Range("C1").PasteSpecial xlPasteValues
' Clean up
Application.CutCopyMode = False
End Sub
Method 3: Assigning Values Directly
For a more efficient approach, especially when dealing with large datasets, you can assign the values of one range directly to another without using the clipboard. This method is faster and more reliable than the traditional copy and paste.
Sub AssignValuesDirectly()
' Declare variables
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
' Set source and target sheets
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set targetSheet = ThisWorkbook.Sheets("Sheet2")
' Assign values from range A1:B2 in source sheet to range C1 in target sheet
targetSheet.Range("C1:D2").Value = sourceSheet.Range("A1:B2").Value
End Sub
Method 4: Using the `AutoFill` Method
If you need to copy a formula down a range based on another range's size, you can use the AutoFill
method. This approach is particularly useful for dynamic data ranges.
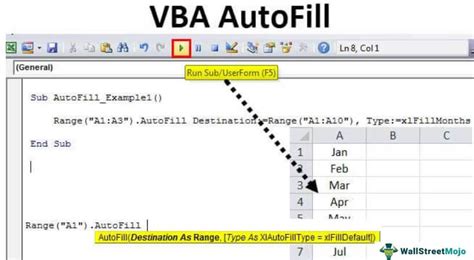
Sub AutoFillRange()
' Declare variables
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
' Set source and target sheets
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set targetSheet = ThisWorkbook.Sheets("Sheet2")
' AutoFill formula in cell A1 down to the last row with data in column B
sourceSheet.Range("A1").AutoFill Destination:=sourceSheet.Range("A1:A" & sourceSheet.Cells(Rows.Count, "B").End(xlUp).Row)
End Sub
Method 5: Using the `Copy` Method with Multiple Ranges
Finally, if you need to copy multiple ranges to another sheet in a single operation, you can use the Union
method to combine these ranges and then apply the Copy
method.
Sub CopyMultipleRanges()
' Declare variables
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
' Set source and target sheets
Set sourceSheet = ThisWorkbook.Sheets("Sheet1")
Set targetSheet = ThisWorkbook.Sheets("Sheet2")
' Define multiple ranges
Dim rng1 As Range
Set rng1 = sourceSheet.Range("A1:B2")
Dim rng2 As Range
Set rng2 = sourceSheet.Range("D1:E2")
' Union multiple ranges and copy to target sheet
Union(rng1, rng2).Copy Destination:=targetSheet.Range("C1")
End Sub
Gallery of Copy Range to Another Sheet VBA Methods
Copy Range to Another Sheet VBA Methods

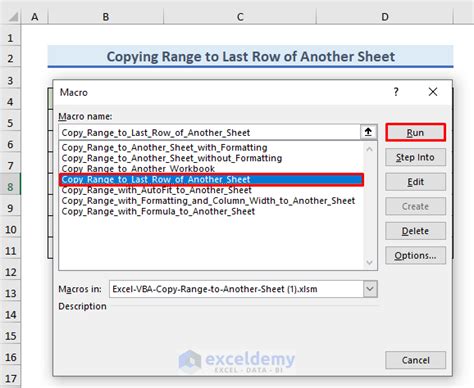
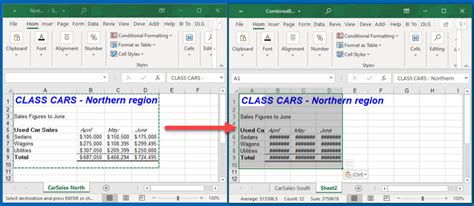
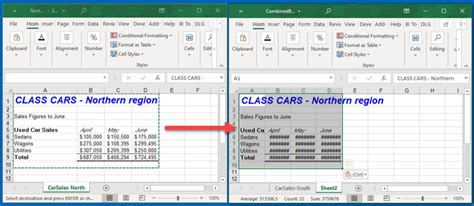
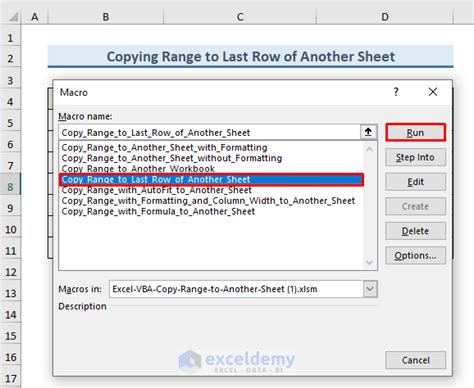
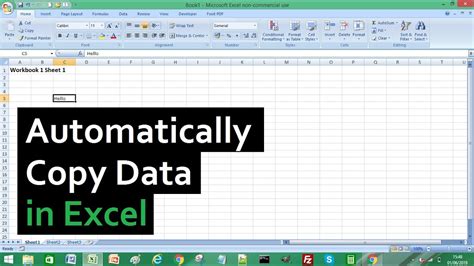
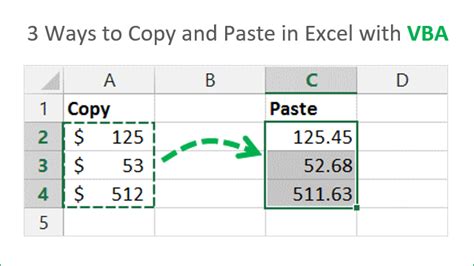

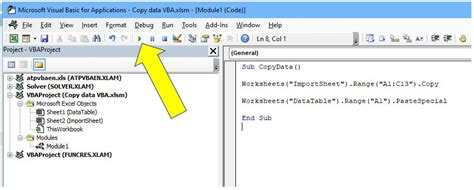
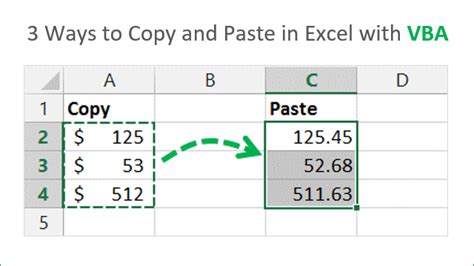
Conclusion and Next Steps
Copying ranges to another sheet in Excel VBA is a fundamental skill that opens doors to a wide range of automation and data manipulation possibilities. Whether you're dealing with simple data copying tasks or complex data processing scenarios, mastering these methods can significantly enhance your productivity and the efficiency of your Excel applications.
For further learning, it's beneficial to explore more advanced VBA concepts such as error handling, loops, and conditional statements, which can help you tackle more complex automation challenges. Additionally, familiarizing yourself with Excel's built-in functions and features can help you identify opportunities for automation and optimization in your workbooks.
We invite you to share your experiences, tips, or questions about copying ranges in Excel VBA in the comments below. This community-driven discussion can help spread knowledge and inspire new solutions for tackling everyday challenges in Excel.