Intro
Learn how to efficiently copy Excel sheets to new workbooks using VBA with our expert guide. Discover 5 ways to automate the process, including worksheets, ranges, and entire workbooks. Master VBA coding techniques and streamline your workflow with these easy-to-follow methods, perfect for Excel users seeking to boost productivity and accuracy.
In the world of Excel, automating repetitive tasks can significantly boost productivity. One such task that can be automated is copying an Excel sheet to a new workbook. This can be particularly useful when you need to create multiple versions of the same data or when you want to share the data with others without affecting the original workbook. Here, we'll explore five ways to copy an Excel sheet to a new workbook using VBA.
Excel Visual Basic for Applications (VBA) is a powerful tool that allows you to create and automate tasks in Excel. By writing VBA code, you can automate many tasks that would otherwise require manual effort, saving you time and reducing the likelihood of errors.
Why Use VBA to Copy Excel Sheets?
Before diving into the methods, let's quickly cover why using VBA for this task is beneficial:
- Efficiency: Automating the copying process saves time and reduces the chance of human error.
- Flexibility: VBA allows you to customize the copying process based on specific criteria or conditions.
- Scalability: You can easily apply VBA scripts to copy multiple sheets or perform other actions in bulk.
Method 1: Basic Sheet Copy
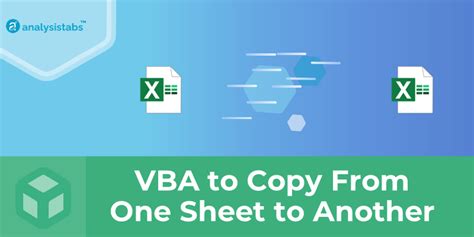
Let's start with the simplest method to copy a sheet to a new workbook. This VBA code snippet does exactly that:
Sub CopySheetToNewWorkbook()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1") 'Specify the sheet you want to copy
'Copy the sheet to a new workbook
ws.Copy
Application.ActiveWorkbook.SaveAs "C:\Path\To\NewWorkbook.xlsx" 'Save the new workbook
End Sub
Replace "Sheet1"
with the name of the sheet you want to copy and specify the path where you want to save the new workbook.
Method 2: Copy Sheet with Data Validation
Sometimes, you might want to copy a sheet while maintaining data validation rules. The following code ensures that data validation is preserved during the copying process:
Sub CopySheetWithDataValidation()
Dim sourceSheet As Worksheet
Dim targetWorkbook As Workbook
Dim targetSheet As Worksheet
'Set the source sheet
Set sourceSheet = ThisWorkbook.Worksheets("Sheet1")
'Create a new workbook
Set targetWorkbook = Workbooks.Add
'Set the target sheet
Set targetSheet = targetWorkbook.Worksheets(1)
'Copy the source sheet to the target sheet, preserving data validation
sourceSheet.Cells.Copy Destination:=targetSheet.Cells
'Save the target workbook
targetWorkbook.SaveAs "C:\Path\To\NewWorkbook.xlsx"
End Sub
Method 3: Copying Multiple Sheets
If you need to copy multiple sheets to a new workbook, you can modify the code to loop through the specified sheets. Here's how you can do it:
Sub CopyMultipleSheets()
Dim ws As Worksheet
Dim targetWorkbook As Workbook
Dim sourceSheets() As String
'List the sheets you want to copy
sourceSheets = Array("Sheet1", "Sheet2", "Sheet3")
'Create a new workbook
Set targetWorkbook = Workbooks.Add
'Loop through the source sheets and copy them to the target workbook
For Each ws In ThisWorkbook.Worksheets
If IsInArray(ws.Name, sourceSheets) Then
ws.Copy after:=targetWorkbook.Sheets(targetWorkbook.Sheets.Count)
End If
Next ws
'Save the target workbook
targetWorkbook.SaveAs "C:\Path\To\NewWorkbook.xlsx"
End Sub
Function IsInArray(val As String, arr As Variant) As Boolean
IsInArray = (UBound(Filter(arr, val)) > -1)
End Function
Replace "Sheet1"
, "Sheet2"
, and "Sheet3"
with the names of the sheets you want to copy.
Method 4: Using Workbook_ADD Method
Another way to copy a sheet to a new workbook is by using the Workbook.Add
method followed by the Worksheet.Copy
method. Here's an example:
Sub CopySheetUsingWorkbookAdd()
Dim ws As Worksheet
Dim newWorkbook As Workbook
'Set the source sheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
'Create a new workbook
Set newWorkbook = Workbooks.Add
'Copy the source sheet to the new workbook
ws.Copy before:=newWorkbook.Sheets(1)
'Remove the original sheet from the new workbook
Application.DisplayAlerts = False
newWorkbook.Sheets(2).Delete
Application.DisplayAlerts = True
'Save the new workbook
newWorkbook.SaveAs "C:\Path\To\NewWorkbook.xlsx"
End Sub
Method 5: Using Worksheet.Copy Method Directly
Finally, you can directly use the Worksheet.Copy
method to copy a sheet to a new workbook without explicitly creating a new workbook. Here's how:
Sub CopySheetDirectly()
Dim ws As Worksheet
'Set the source sheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
'Copy the source sheet to a new workbook
ws.Copy
'Save the new workbook
ActiveWorkbook.SaveAs "C:\Path\To\NewWorkbook.xlsx"
End Sub
This method is more straightforward but achieves the same result as the previous methods.
Gallery of Excel VBA Copying Sheets
Excel VBA Copying Sheets Gallery
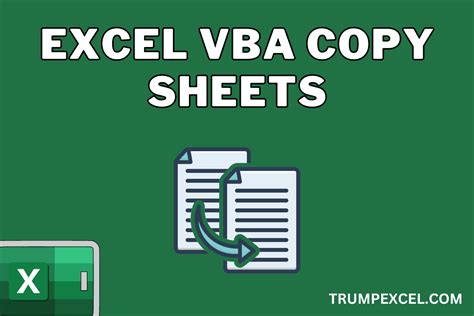
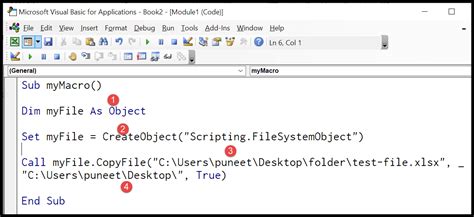
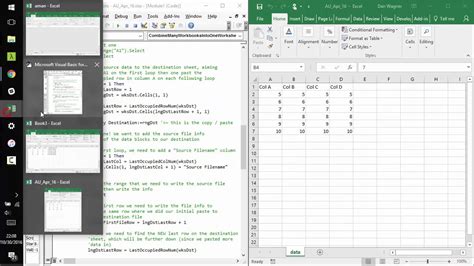
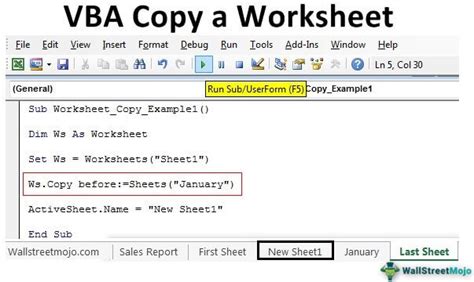
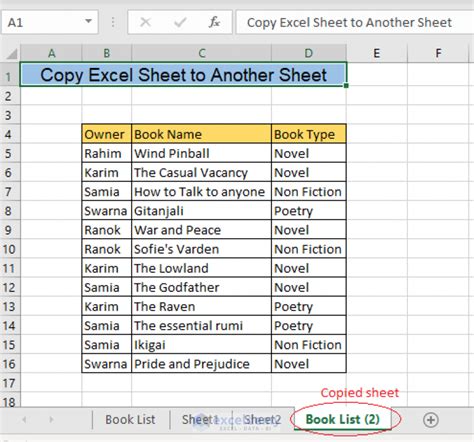
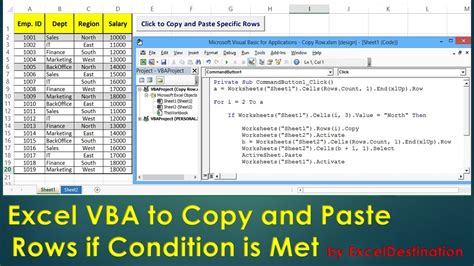
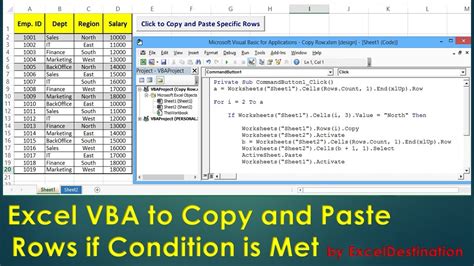
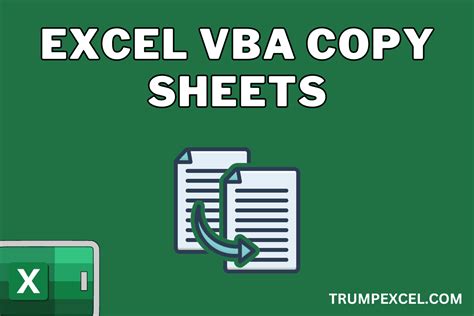
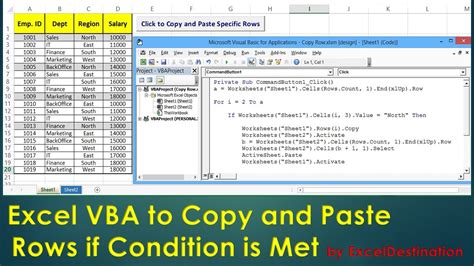
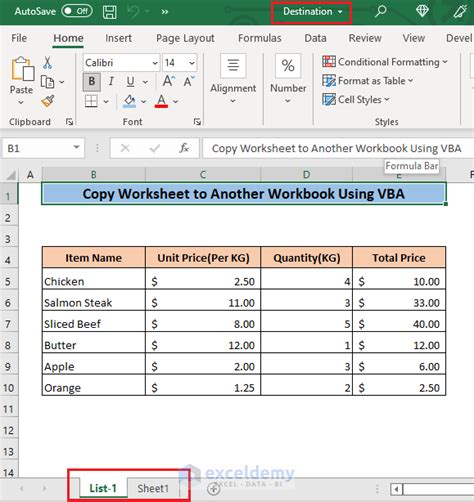
Conclusion and Next Steps
Copying an Excel sheet to a new workbook using VBA is a straightforward process that can significantly streamline your workflow. Whether you need to copy a single sheet or multiple sheets, the methods outlined above provide flexible solutions. Remember to adjust the code snippets to match your specific needs and the names of your worksheets.
We hope this guide has been informative and helpful. If you have any questions or need further assistance, don't hesitate to reach out. Feel free to share your experiences with copying Excel sheets using VBA in the comments below.