Mastering the Art of Copying Visible Cells in Excel VBA
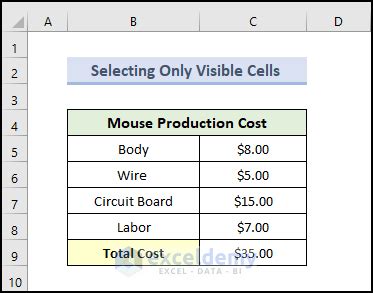
In the world of Excel VBA, manipulating data is an essential skill for any programmer. One of the most common tasks is copying visible cells, which can be a bit tricky, especially when dealing with filtered data or hidden rows. In this article, we will delve into the world of Excel VBA and explore the easiest ways to copy visible cells.
Understanding the Problem
When working with large datasets in Excel, it's common to hide or filter out unnecessary data to focus on the information that matters. However, when it comes to copying data, things can get complicated. Excel's built-in copy function often includes hidden or filtered cells, which can lead to errors or unexpected results. This is where VBA comes to the rescue.
Method 1: Using the `SpecialCells` Method
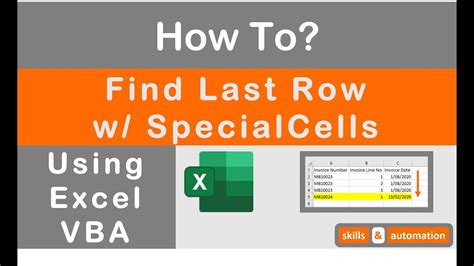
One of the simplest ways to copy visible cells in Excel VBA is by using the SpecialCells
method. This method allows you to select specific types of cells, including visible cells.
Sub CopyVisibleCells()
Range("A1:A100").SpecialCells(xlCellTypeVisible).Copy
End Sub
In this example, we're selecting the range A1:A100 and using the SpecialCells
method to copy only the visible cells.
Method 2: Using the `AutoFilter` Method
When working with filtered data, you can use the AutoFilter
method to copy visible cells.
Sub CopyFilteredCells()
Range("A1:A100").AutoFilter Field:=1, Criteria1:="<>隐藏"
Range("A1:A100").SpecialCells(xlCellTypeVisible).Copy
Range("A1:A100").AutoFilter
End Sub
In this example, we're applying an auto-filter to the range A1:A100, selecting only the visible cells, and then copying them.
Method 3: Using the `Loop` Method
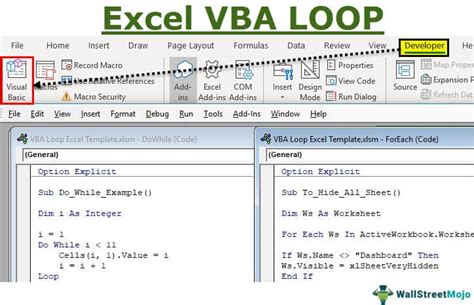
Another way to copy visible cells is by using a loop to iterate through the range and check each cell's visibility.
Sub CopyVisibleCellsLoop()
Dim cell As Range
For Each cell In Range("A1:A100")
If cell.Rows.Hidden = False Then
cell.Copy
End If
Next cell
End Sub
In this example, we're looping through the range A1:A100 and checking each cell's Rows.Hidden
property. If the cell is not hidden, we copy it.
Method 4: Using the `Union` Method
You can also use the Union
method to combine multiple ranges into a single range and then copy the visible cells.
Sub CopyVisibleCellsUnion()
Dim rng As Range
Set rng = Range("A1:A100")
rng.SpecialCells(xlCellTypeVisible).Copy
End Sub
In this example, we're using the Union
method to combine the range A1:A100 and then copying the visible cells.
Best Practices and Tips
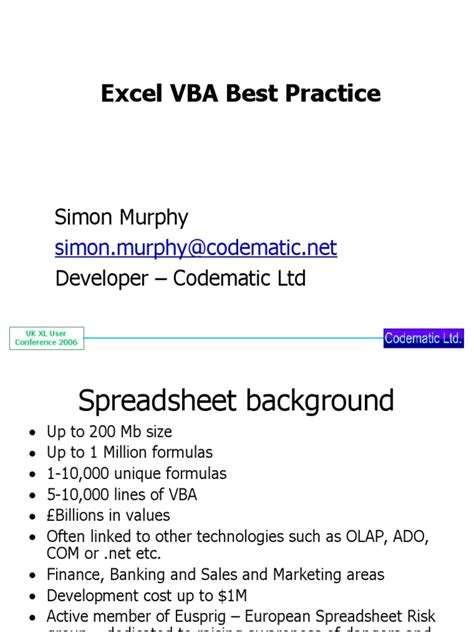
When working with VBA, it's essential to follow best practices to ensure your code is efficient, readable, and maintainable. Here are some tips to keep in mind:
- Always use meaningful variable names and comments to explain your code.
- Use the
Option Explicit
statement to declare all variables. - Avoid using
Select
andActivate
methods, instead, useRange
andWorksheet
objects. - Use error handling to catch and handle runtime errors.
Conclusion: Mastering the Art of Copying Visible Cells
Copying visible cells in Excel VBA can be a bit tricky, but with the right techniques and best practices, you can master this essential skill. Whether you're using the SpecialCells
method, AutoFilter
method, Loop
method, or Union
method, the key is to understand the problem and choose the right approach. By following the tips and best practices outlined in this article, you'll be well on your way to becoming an Excel VBA expert.
Excel VBA Image Gallery
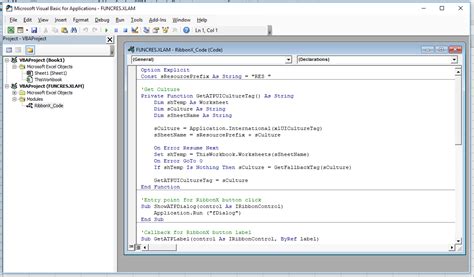
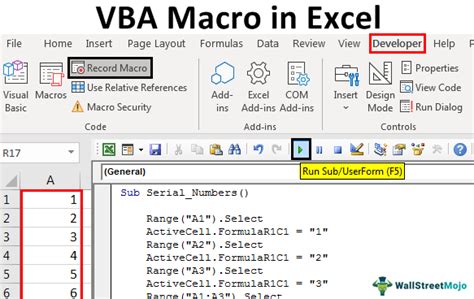
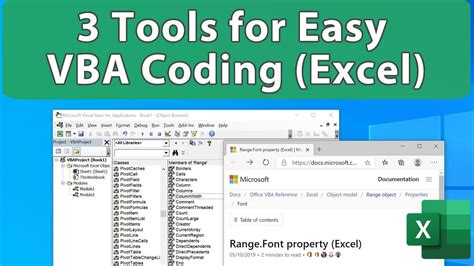
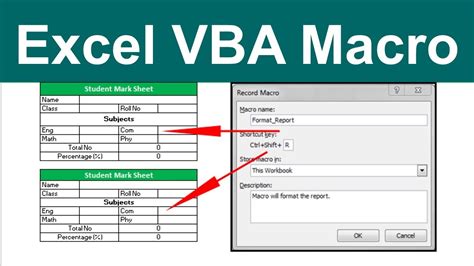
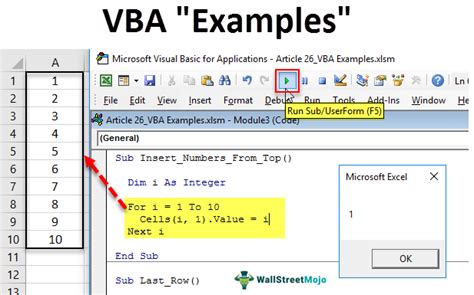
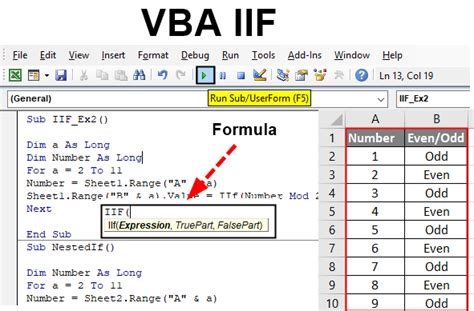
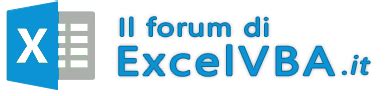
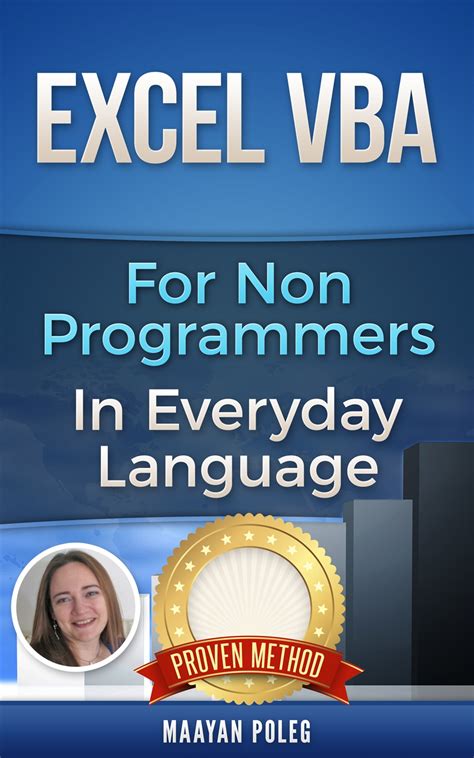
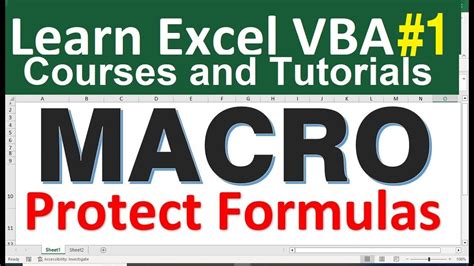
What's your favorite method for copying visible cells in Excel VBA? Share your thoughts and experiences in the comments below!