Intro
Master Excel VBA row counting with 5 expert methods. Learn to efficiently count rows with data in Excel VBA, including using Range.Find, Looping, SpecialCells, and more. Discover how to accurately count rows, handle errors, and optimize performance with these actionable VBA coding techniques and examples.
Counting rows with data in Excel VBA can be an essential task in many automation projects. Whether you're a beginner or an advanced VBA developer, knowing the most efficient methods to count rows can save you a lot of time and effort. In this article, we'll explore five different ways to count rows with data in Excel VBA.
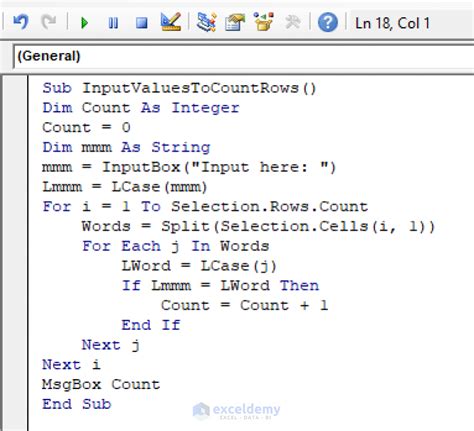
Why Count Rows in Excel VBA?
Before we dive into the different methods, let's quickly discuss why counting rows is important in Excel VBA. Counting rows can help you:
- Determine the number of records in a dataset
- Set the range for a loop
- Resize an array
- Check if data exists in a worksheet
- And many more!
Method 1: Using the CountA Function
The CountA function is a simple and straightforward way to count rows with data in a specific range. Here's an example:
Sub CountRowsMethod1()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim rowCount As Long
rowCount = Application.WorksheetFunction.CountA(ws.Range("A1:A100"))
MsgBox "Number of rows with data: " & rowCount
End Sub
In this example, the CountA function counts the number of non-empty cells in the range A1:A100.
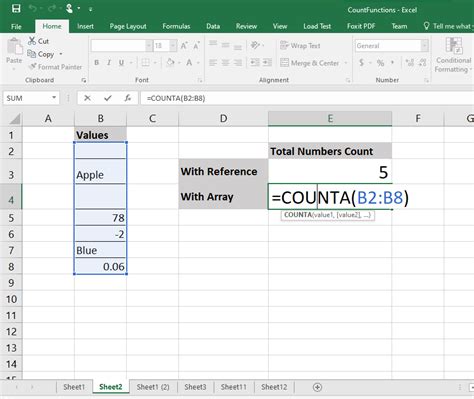
Method 2: Using the Cells.Find Method
The Cells.Find method is another way to count rows with data. This method is particularly useful when you need to search for a specific value or pattern in a range. Here's an example:
Sub CountRowsMethod2()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim foundCell As Range
Set foundCell = ws.Cells.Find("*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious)
If Not foundCell Is Nothing Then
Dim rowCount As Long
rowCount = foundCell.Row
MsgBox "Number of rows with data: " & rowCount
Else
MsgBox "No data found"
End If
End Sub
In this example, the Cells.Find method searches for any value (denoted by the asterisk "*") in the entire worksheet, starting from the top-left cell. The SearchOrder parameter is set to xlByRows to search row by row, and the SearchDirection parameter is set to xlPrevious to start searching from the bottom of the range.

Method 3: Using the UsedRange Property
The UsedRange property is a convenient way to count rows with data in a worksheet. This property returns the range of cells that have been used in the worksheet. Here's an example:
Sub CountRowsMethod3()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim usedRange As Range
Set usedRange = ws.UsedRange
Dim rowCount As Long
rowCount = usedRange.Rows.Count
MsgBox "Number of rows with data: " & rowCount
End Sub
In this example, the UsedRange property returns the range of cells that have been used in the worksheet, and the Rows.Count property returns the number of rows in that range.
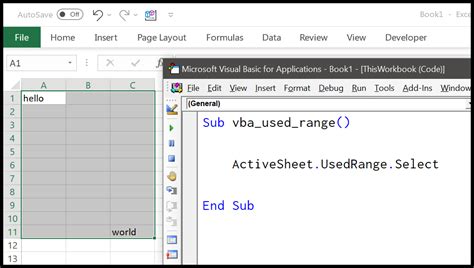
Method 4: Using the Range.Find Method
The Range.Find method is similar to the Cells.Find method, but it allows you to search within a specific range. Here's an example:
Sub CountRowsMethod4()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim searchRange As Range
Set searchRange = ws.Range("A1:A100")
Dim foundCell As Range
Set foundCell = searchRange.Find("*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious)
If Not foundCell Is Nothing Then
Dim rowCount As Long
rowCount = foundCell.Row
MsgBox "Number of rows with data: " & rowCount
Else
MsgBox "No data found"
End If
End Sub
In this example, the Range.Find method searches for any value (denoted by the asterisk "*") within the range A1:A100, starting from the top-left cell. The SearchOrder parameter is set to xlByRows to search row by row, and the SearchDirection parameter is set to xlPrevious to start searching from the bottom of the range.

Method 5: Using a Loop
The final method is to use a loop to count rows with data. This method is particularly useful when you need to perform additional operations on each row. Here's an example:
Sub CountRowsMethod5()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
Dim rowCount As Long
rowCount = 0
Dim i As Long
For i = 1 To 100
If ws.Cells(i, 1).Value <> "" Then
rowCount = rowCount + 1
End If
Next i
MsgBox "Number of rows with data: " & rowCount
End Sub
In this example, the loop iterates through each row in the range A1:A100, checking if the cell in column A is not empty. If the cell is not empty, the rowCount variable is incremented.
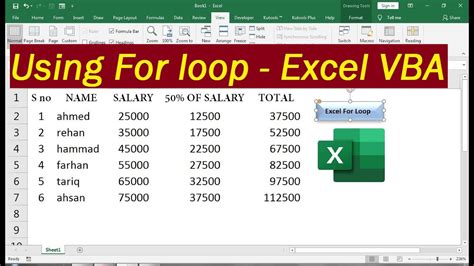
Gallery of Excel VBA Methods
Excel VBA Methods Gallery
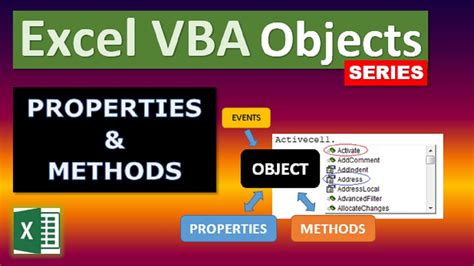
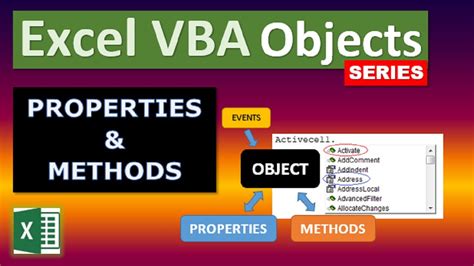
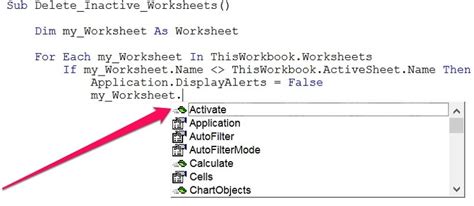
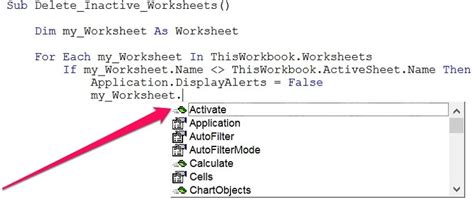
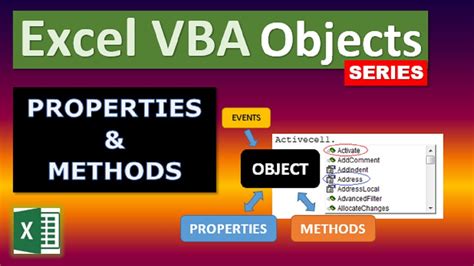

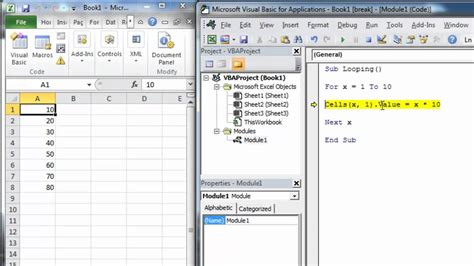
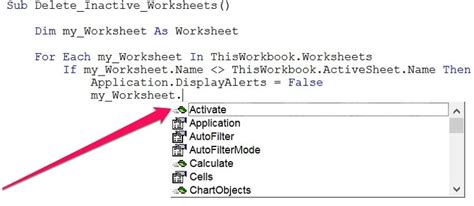
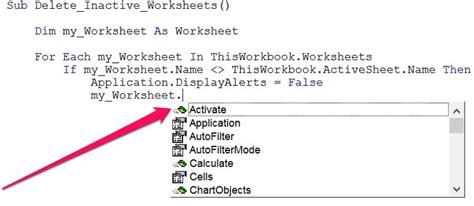
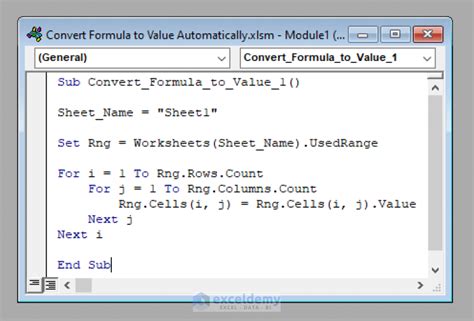
Conclusion
In this article, we've explored five different ways to count rows with data in Excel VBA. Each method has its own strengths and weaknesses, and the choice of method will depend on your specific needs and requirements. Whether you're a beginner or an advanced VBA developer, mastering these methods will help you to write more efficient and effective code.
FAQ
Q: What is the fastest method to count rows with data in Excel VBA? A: The fastest method is often the UsedRange property, but this can depend on the specific circumstances.
Q: Can I use these methods to count rows with data in multiple columns? A: Yes, you can modify the methods to count rows with data in multiple columns by adjusting the range or loop accordingly.
Q: How can I count rows with data in a specific range, but ignore blank cells? A: You can use the Range.Find method or a loop to count rows with data in a specific range, and ignore blank cells by checking for non-empty cells.
We hope this article has been helpful in your Excel VBA journey. If you have any further questions or comments, please don't hesitate to reach out. Happy coding!