Intro
Master referencing the current worksheet in Excel VBA with these 5 expert techniques. Learn how to dynamically refer to the active worksheet using methods like ActiveSheet, Worksheet.Name, Me, ThisWorkbook, and more. Boost your VBA coding skills and efficiency with these practical tips and examples.
Working with Excel VBA can be a powerful way to automate tasks and improve productivity, but it can also be confusing, especially when it comes to referencing specific worksheets. If you're working with multiple worksheets in a single workbook, it's essential to reference the correct worksheet to avoid errors and ensure your code runs smoothly. Here are five ways to reference the current worksheet in Excel VBA:
Why is referencing the current worksheet important?
Before we dive into the different methods, it's essential to understand why referencing the current worksheet is crucial. When you're working with multiple worksheets, Excel VBA needs to know which worksheet you want to perform actions on. If you don't specify the correct worksheet, your code may not work as intended, or worse, it may modify the wrong worksheet.
Method 1: Using the ActiveSheet
Property
One of the simplest ways to reference the current worksheet is by using the ActiveSheet
property. This property returns the worksheet that is currently active, which is usually the worksheet that you're working on.
Sub Example()
MsgBox ActiveSheet.Name
End Sub
In this example, the code will display the name of the current worksheet in a message box.
Method 2: Using the Me
Keyword
If you're working within a worksheet module, you can use the Me
keyword to reference the current worksheet. The Me
keyword is a shorthand way of referring to the current object, which in this case is the worksheet.
Sub Example()
MsgBox Me.Name
End Sub
This code will also display the name of the current worksheet in a message box.
Method 3: Using the ThisWorkbook
Object
Another way to reference the current worksheet is by using the ThisWorkbook
object. This object returns the workbook that contains the code, and you can use the ActiveSheet
property to get the current worksheet.
Sub Example()
MsgBox ThisWorkbook.ActiveSheet.Name
End Sub
This code will also display the name of the current worksheet in a message box.
Method 4: Using the Worksheets
Collection
If you need to reference a specific worksheet, you can use the Worksheets
collection. This collection contains all the worksheets in the workbook, and you can use the Index
property to get a specific worksheet.
Sub Example()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(1)
MsgBox ws.Name
End Sub
In this example, the code will display the name of the first worksheet in the workbook.
Method 5: Using the Worksheet
Object
Finally, you can also reference the current worksheet using the Worksheet
object. This object is used to represent a worksheet, and you can use it to perform actions on the worksheet.
Sub Example()
Dim ws As Worksheet
Set ws = ActiveSheet
MsgBox ws.Name
End Sub
This code will also display the name of the current worksheet in a message box.
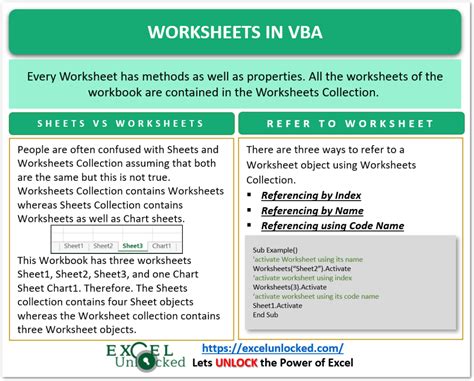
In conclusion, there are several ways to reference the current worksheet in Excel VBA, each with its own advantages and disadvantages. By using the ActiveSheet
property, Me
keyword, ThisWorkbook
object, Worksheets
collection, or Worksheet
object, you can ensure that your code runs smoothly and accurately. Remember to always specify the correct worksheet to avoid errors and improve productivity.
Gallery of Excel VBA Worksheets
Excel VBA Worksheets Gallery
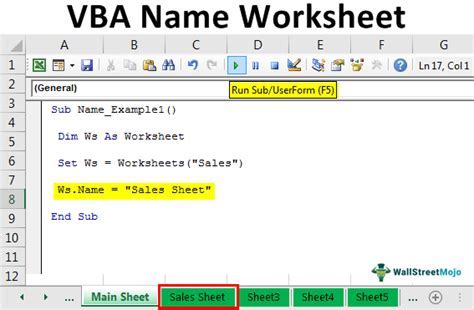
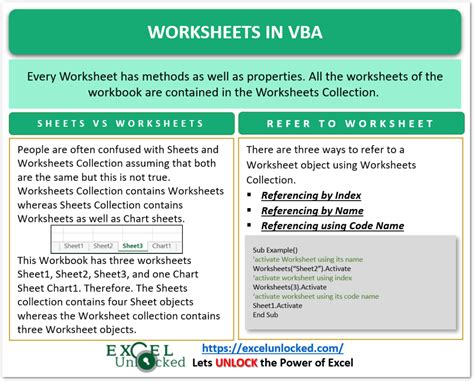
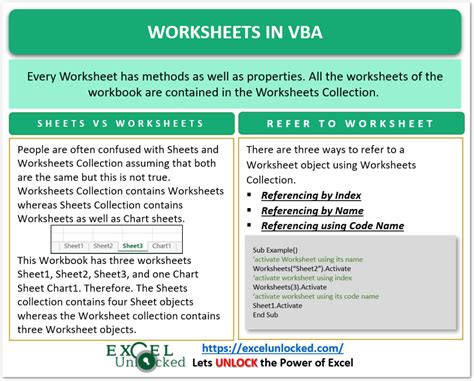
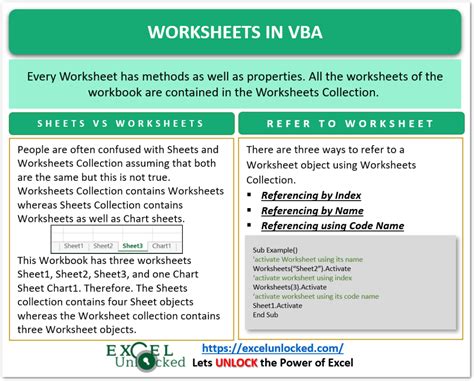
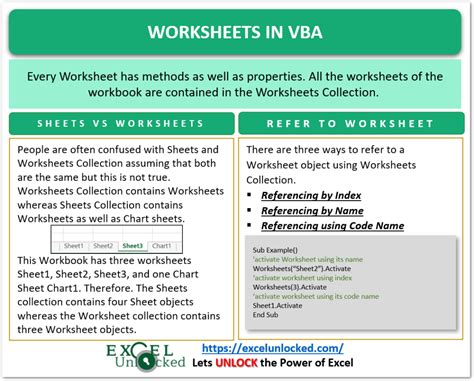
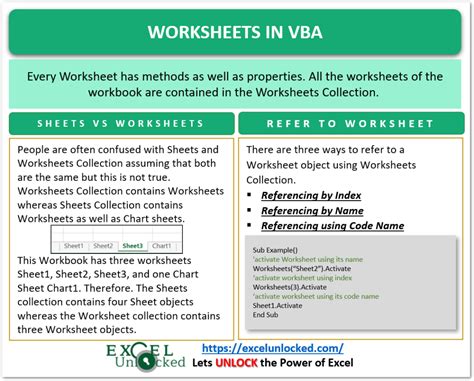
Share Your Thoughts
Do you have any experience with referencing worksheets in Excel VBA? Share your thoughts and tips in the comments below!