Delete Empty Rows in Excel VBA
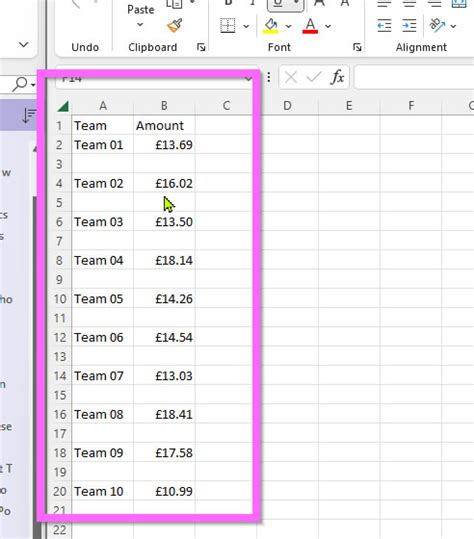
Deleting empty rows in Excel VBA can be a tedious task, especially when dealing with large datasets. However, with the right techniques and codes, you can automate this process and save time. In this article, we will explore five different ways to delete empty rows in Excel VBA.
Method 1: Using the SpecialCells Method
The SpecialCells method is a powerful tool in Excel VBA that allows you to select specific cells or ranges based on certain criteria. To delete empty rows using this method, you can use the following code:Sub DeleteEmptyRows()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Cells.SpecialCells(xlCellTypeBlanks).EntireRow.Delete
End Sub
This code selects all blank cells in the active worksheet and then deletes the entire row.
Method 2: Using the Loop Method
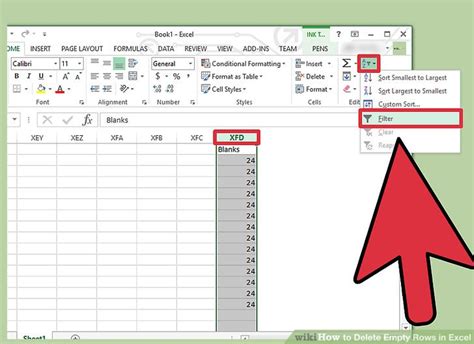
Another way to delete empty rows is by using a loop to iterate through each row in the worksheet. Here is an example code:
Sub DeleteEmptyRows()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim i As Long
For i = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row To 1 Step -1
If ws.Cells(i, 1).Value = "" Then
ws.Rows(i).Delete
End If
Next i
End Sub
This code loops through each row in the worksheet, starting from the bottom, and checks if the value in the first column is blank. If it is, the row is deleted.
Method 3: Using the AutoFilter Method
The AutoFilter method is a convenient way to filter data in Excel VBA. To delete empty rows using this method, you can use the following code:Sub DeleteEmptyRows()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Cells.AutoFilter Field:=1, Criteria1:="<>"
ws.Cells.SpecialCells(xlCellTypeVisible).EntireRow.Delete
End Sub
This code applies an AutoFilter to the first column, hiding all rows with blank values. It then deletes the visible rows, effectively deleting the empty rows.
Method 4: Using the Range.Find Method
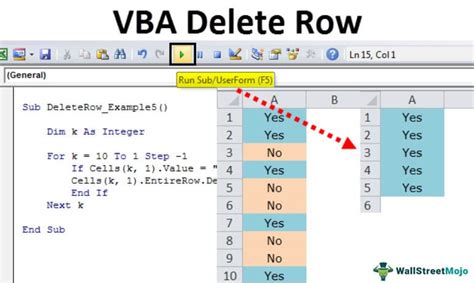
The Range.Find method is another way to locate and delete empty rows in Excel VBA. Here is an example code:
Sub DeleteEmptyRows()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim rng As Range
Set rng = ws.Cells.Find("")
While Not rng Is Nothing
rng.EntireRow.Delete
Set rng = ws.Cells.Find("")
Wend
End Sub
This code uses the Range.Find method to locate the first blank cell in the worksheet. It then deletes the entire row and repeats the process until all empty rows are deleted.
Method 5: Using the Worksheet.UsedRange.SpecialCells Method
The Worksheet.UsedRange.SpecialCells method is a combination of the SpecialCells method and the UsedRange property. To delete empty rows using this method, you can use the following code:Sub DeleteEmptyRows()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.UsedRange.SpecialCells(xlCellTypeBlanks).EntireRow.Delete
End Sub
This code selects all blank cells within the used range of the worksheet and then deletes the entire row.
Gallery of Delete Empty Rows in Excel VBA
Delete Empty Rows in Excel VBA Image Gallery
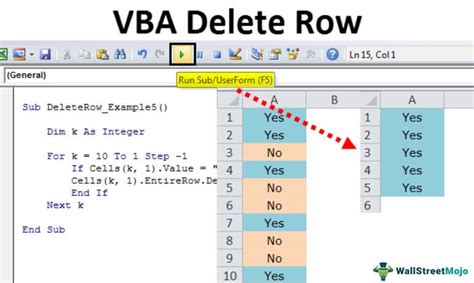
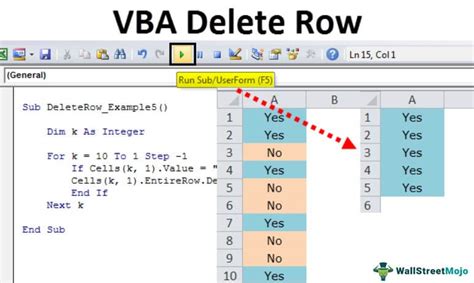
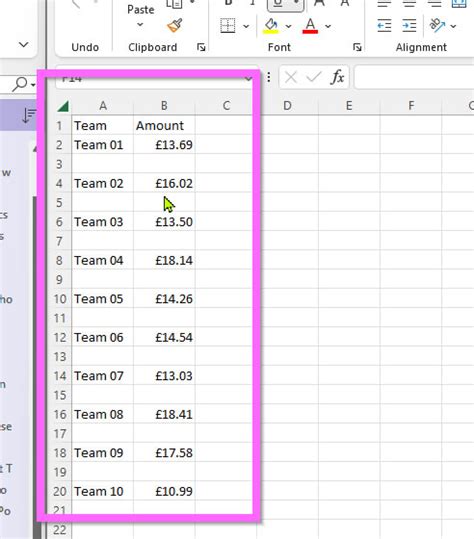
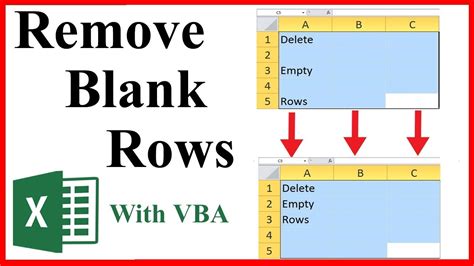
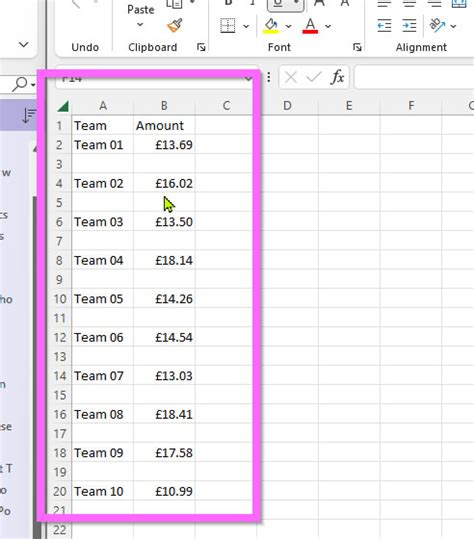
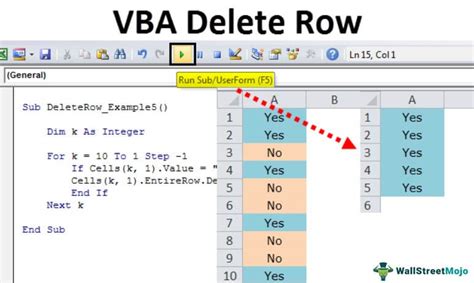
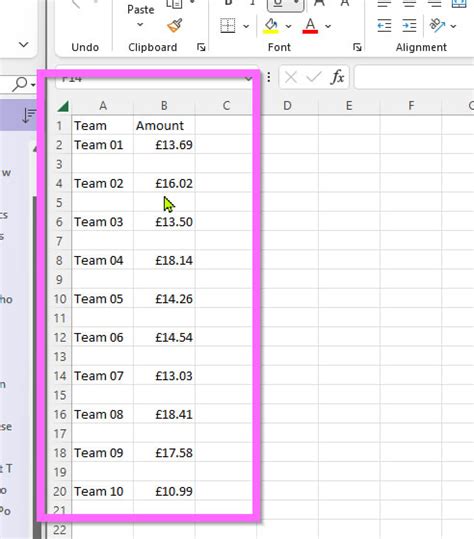
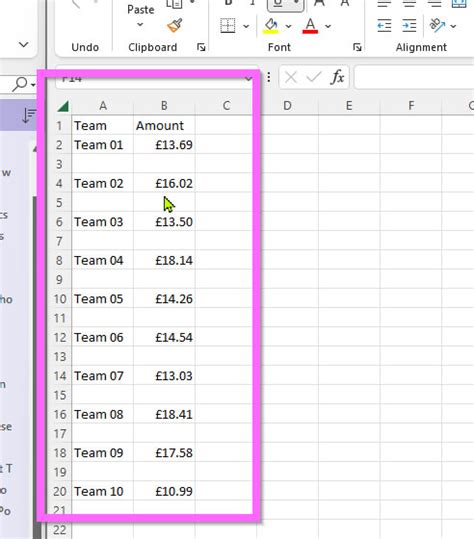
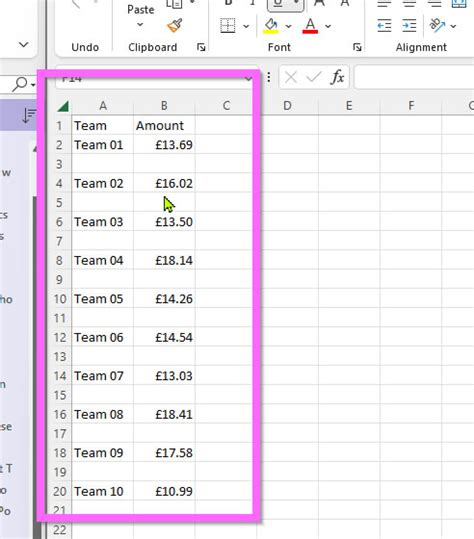
Conclusion
Deleting empty rows in Excel VBA can be a challenging task, but with the right techniques and codes, you can automate this process and save time. In this article, we explored five different ways to delete empty rows in Excel VBA, including using the SpecialCells method, loop method, AutoFilter method, Range.Find method, and Worksheet.UsedRange.SpecialCells method. Each method has its own advantages and disadvantages, and you can choose the one that best suits your needs.We hope this article has been helpful in providing you with the knowledge and skills to delete empty rows in Excel VBA. If you have any questions or need further assistance, please don't hesitate to ask.