In the world of Microsoft Excel, Visual Basic for Applications (VBA) is a powerful tool that allows users to automate various tasks and streamline their workflow. One of the most common tasks in Excel is deleting rows, and VBA provides several ways to accomplish this. In this article, we will explore five different methods to delete rows in Excel VBA.
Understanding the Importance of Deleting Rows in Excel VBA
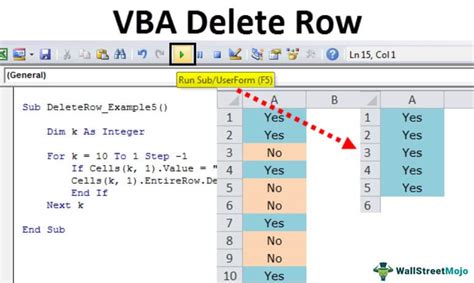
Before we dive into the methods, it's essential to understand why deleting rows is crucial in Excel VBA. Deleting rows can help you remove unnecessary data, improve data analysis, and enhance the overall performance of your Excel spreadsheet. By using VBA, you can automate the process of deleting rows, making it faster and more efficient.
Method 1: Delete Rows Using the Rows Property
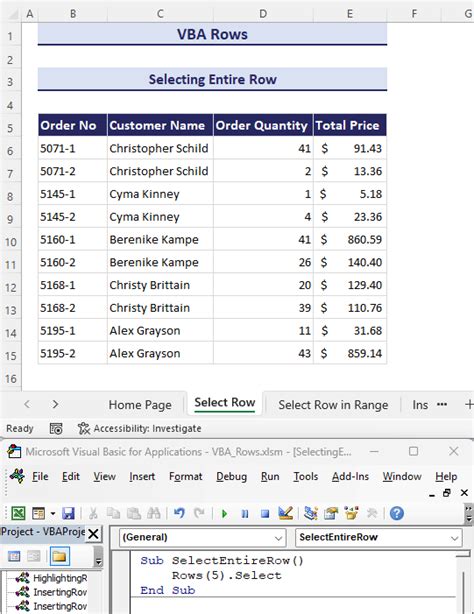
One of the simplest ways to delete rows in Excel VBA is by using the Rows property. This method allows you to delete rows based on their index number. Here's an example code:
Sub DeleteRowsUsingRowsProperty()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Delete row 5
ws.Rows(5).Delete
End Sub
In this code, we declare a variable ws
to represent the worksheet where we want to delete rows. We then use the Rows property to delete row 5.
Method 1.1: Delete Multiple Rows Using the Rows Property
You can also delete multiple rows using the Rows property by specifying a range of rows. Here's an example:
Sub DeleteMultipleRowsUsingRowsProperty()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Delete rows 5 to 10
ws.Rows("5:10").Delete
End Sub
In this code, we delete rows 5 to 10 using the Rows property.
Method 2: Delete Rows Using the Delete Method
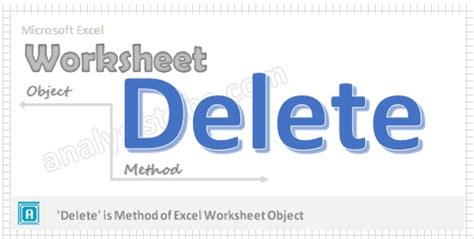
Another way to delete rows in Excel VBA is by using the Delete method. This method allows you to delete rows based on a specific range. Here's an example code:
Sub DeleteRowsUsingDeleteMethod()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Delete row 5
ws.Range("5:5").Delete
End Sub
In this code, we use the Range property to specify the range of cells we want to delete, and then use the Delete method to delete the row.
Method 2.1: Delete Multiple Rows Using the Delete Method
You can also delete multiple rows using the Delete method by specifying a range of cells. Here's an example:
Sub DeleteMultipleRowsUsingDeleteMethod()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Delete rows 5 to 10
ws.Range("5:10").Delete
End Sub
In this code, we delete rows 5 to 10 using the Delete method.
Method 3: Delete Rows Using the AutoFilter Method
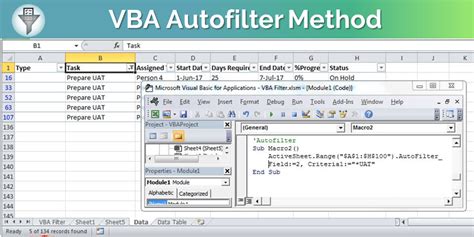
The AutoFilter method is another way to delete rows in Excel VBA. This method allows you to delete rows based on a specific criteria. Here's an example code:
Sub DeleteRowsUsingAutoFilterMethod()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Apply AutoFilter
ws.Range("A1:A10").AutoFilter Field:=1, Criteria1:="<> Criteria"
' Delete visible rows
ws.Range("A1:A10").SpecialCells(xlCellTypeVisible).Delete
End Sub
In this code, we apply an AutoFilter to the range A1:A10, and then delete the visible rows.
Method 4: Delete Rows Using the Find Method
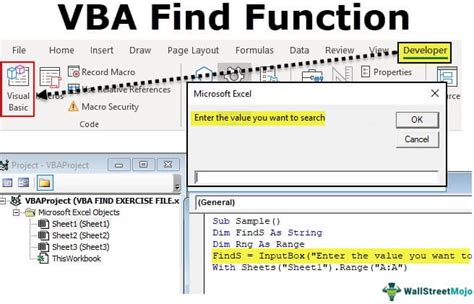
The Find method is another way to delete rows in Excel VBA. This method allows you to delete rows based on a specific criteria. Here's an example code:
Sub DeleteRowsUsingFindMethod()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Find and delete rows
Dim cel As Range
For Each cel In ws.Range("A1:A10")
If cel.Value = "Criteria" Then
cel.EntireRow.Delete
End If
Next cel
End Sub
In this code, we use the Find method to find and delete rows based on a specific criteria.
Method 5: Delete Rows Using the ListObject Method
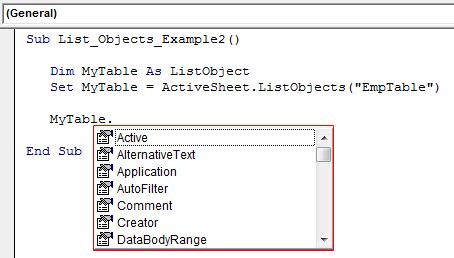
The ListObject method is another way to delete rows in Excel VBA. This method allows you to delete rows based on a specific table. Here's an example code:
Sub DeleteRowsUsingListObjectMethod()
' Declare variables
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Delete rows from table
Dim lo As ListObject
Set lo = ws.ListObjects("Table1")
lo.ListRows(5).Delete
End Sub
In this code, we use the ListObject method to delete rows from a specific table.
Gallery of Excel VBA Delete Rows
Excel VBA Delete Rows Image Gallery
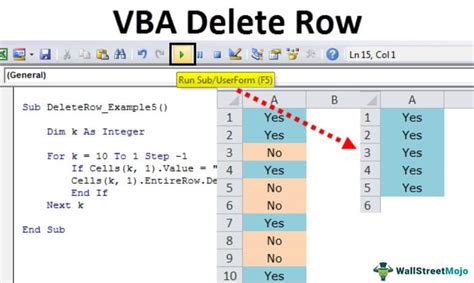
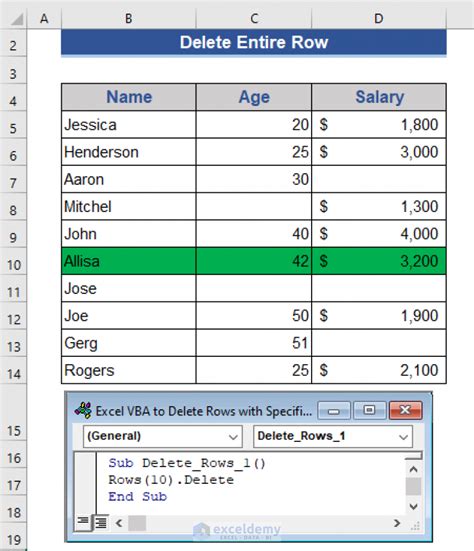
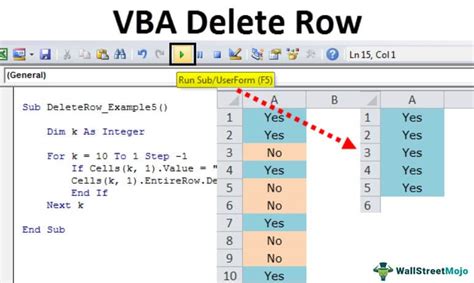
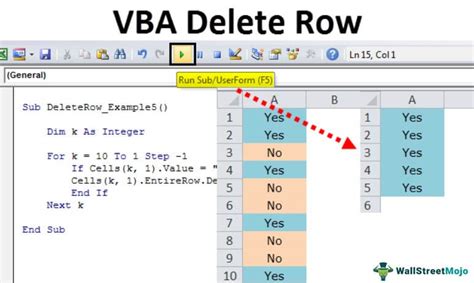
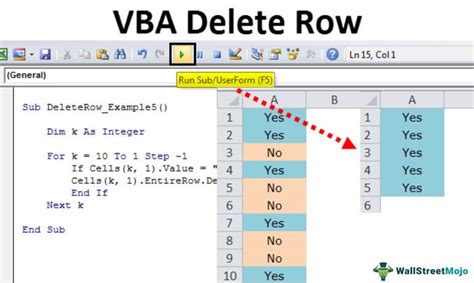
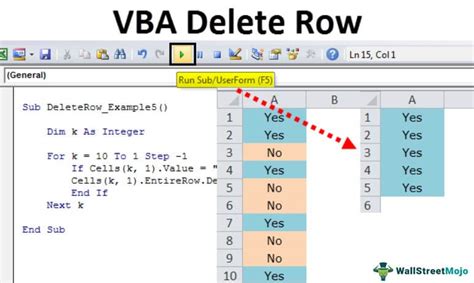
In conclusion, deleting rows in Excel VBA can be accomplished using various methods, including the Rows property, Delete method, AutoFilter method, Find method, and ListObject method. Each method has its own advantages and disadvantages, and the choice of method depends on the specific requirements of your project. By mastering these methods, you can improve your productivity and efficiency in working with Excel VBA.