Intro
Master Excel VBA File Selector in 5 easy steps. Learn how to create a user-friendly file selector dialog box using VBA, streamlining file management and data import. Discover how to code a flexible file selector, handle file paths, and integrate with Excel worksheets, macros, and automation tools for efficient data processing.
Mastering Excel VBA file selectors is a crucial skill for anyone looking to automate tasks and streamline their workflow in Excel. With a file selector, you can easily interact with files and folders, making it a fundamental component of many VBA scripts. In this article, we'll break down the process of using an Excel VBA file selector into 5 easy steps.
What is a File Selector in Excel VBA?
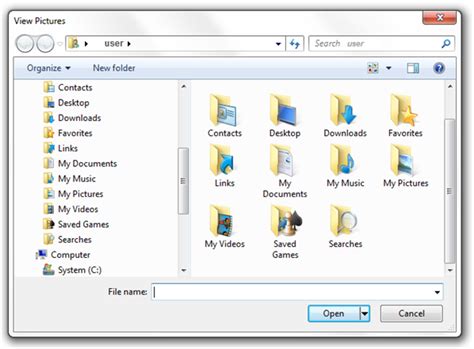
Before we dive into the steps, let's quickly define what a file selector is in the context of Excel VBA. A file selector is a dialog box that allows users to select files or folders from their computer. It's an essential tool for automating tasks that involve interacting with external files, such as importing data or exporting reports.
Step 1: Create a New VBA Module
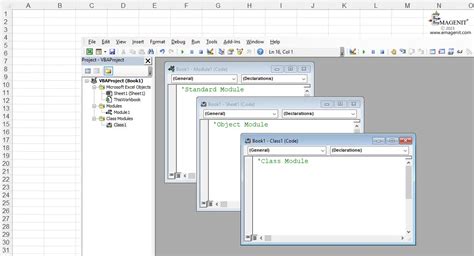
To start using a file selector in Excel VBA, you'll need to create a new VBA module. To do this, follow these steps:
- Open the Visual Basic Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon.
- In the Visual Basic Editor, click Insert > Module to create a new module.
- Give your module a name, such as "FileSelector".
Inserting the FileDialog Object
In your new module, you'll need to insert the FileDialog object. This object is what will allow you to create a file selector dialog box. To insert the FileDialog object, follow these steps:
- In the Visual Basic Editor, click Tools > References.
- In the References dialog box, scroll down and check the box next to "Microsoft Office 14.0 Object Library".
- Click OK to close the dialog box.
Step 2: Declare the FileDialog Object
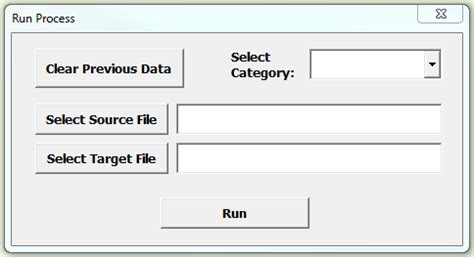
Now that you've inserted the FileDialog object, you'll need to declare it in your code. To do this, add the following line of code to your module:
Dim fileDialog As FileDialog
This line of code declares a new FileDialog object called "fileDialog".
Step 3: Set Up the FileDialog Object
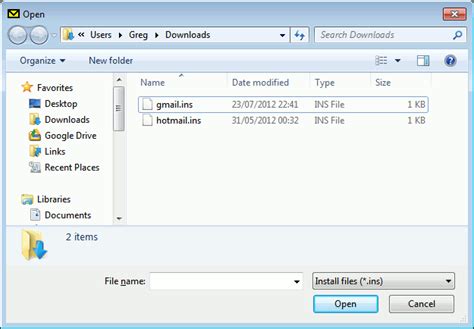
Next, you'll need to set up the FileDialog object. This involves specifying the type of dialog box you want to display, as well as any other settings you want to apply. To set up the FileDialog object, add the following lines of code:
Set fileDialog = Application.FileDialog(msoFileDialogFilePicker)
fileDialog.Title = "Select a File"
fileDialog.AllowMultiSelect = False
These lines of code set up a file picker dialog box with the title "Select a File". The AllowMultiSelect property is set to False, which means that the user can only select one file at a time.
Step 4: Display the FileDialog Object
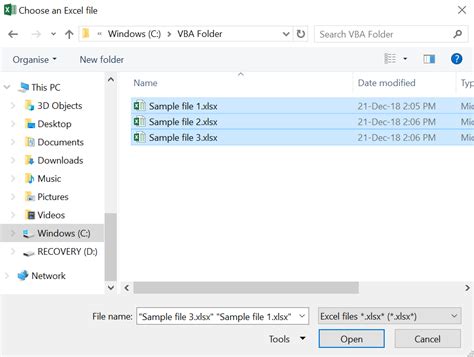
Now that you've set up the FileDialog object, you can display it to the user. To do this, add the following line of code:
fileDialog.Show
This line of code displays the file picker dialog box to the user.
Step 5: Get the Selected File
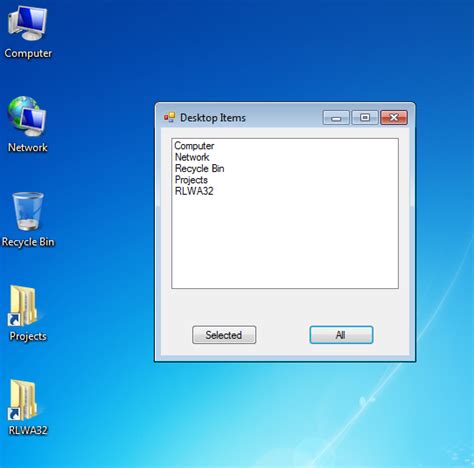
Finally, you'll need to get the file that the user selected. To do this, you can use the following line of code:
Dim selectedFile As String
selectedFile = fileDialog.SelectedItems(1)
This line of code gets the first selected item from the file dialog box and stores it in the "selectedFile" variable.
Putting it all Together
Here's the complete code:
Dim fileDialog As FileDialog
Sub SelectFile()
Set fileDialog = Application.FileDialog(msoFileDialogFilePicker)
fileDialog.Title = "Select a File"
fileDialog.AllowMultiSelect = False
fileDialog.Show
Dim selectedFile As String
selectedFile = fileDialog.SelectedItems(1)
MsgBox "You selected: " & selectedFile
End Sub
This code creates a new file picker dialog box, displays it to the user, and gets the selected file.
Excel VBA File Selector Image Gallery
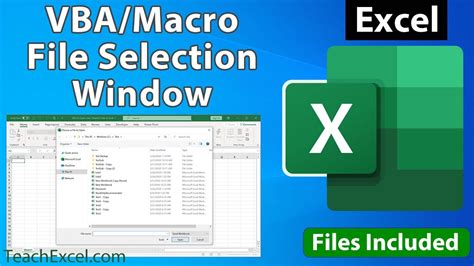
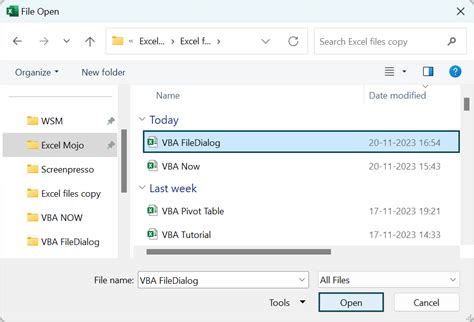
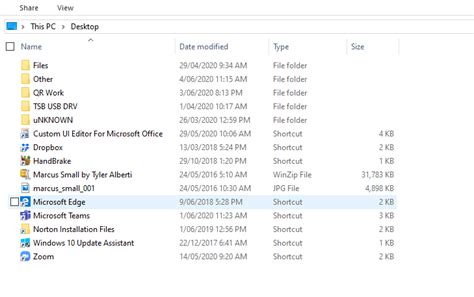
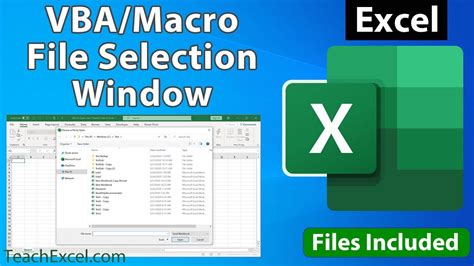
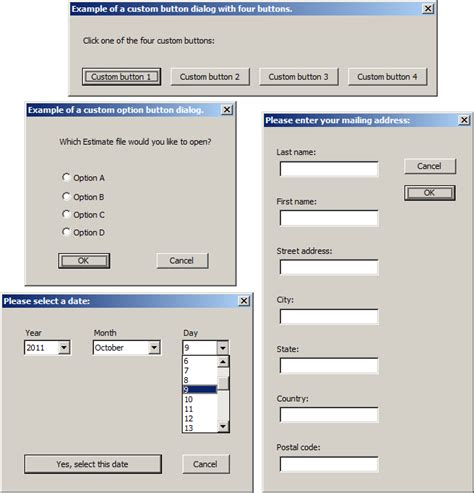
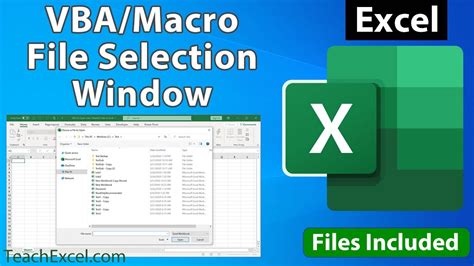
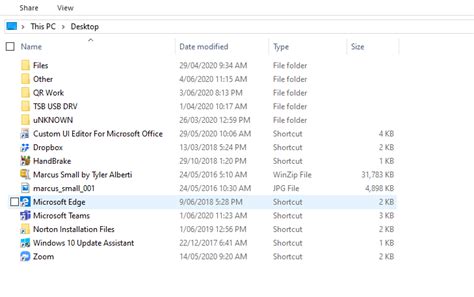
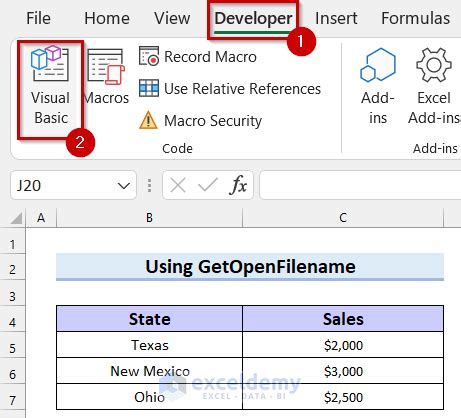
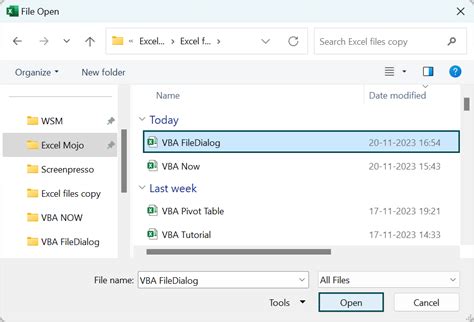
We hope this tutorial has helped you master the Excel VBA file selector. With these 5 easy steps, you can create your own file selector dialog box and automate tasks in Excel. If you have any questions or need further assistance, please don't hesitate to comment below.