Intro
Master Excel VBA loops with our expert guide. Learn 5 efficient ways to loop through cells in Excel VBA, including For Each, For Next, Do While, and Do Until loops. Discover how to iterate through ranges, handle errors, and optimize performance using VBA coding best practices and examples.
Looping through cells in Excel VBA is a fundamental skill for any aspiring Excel developer. It allows you to automate tasks, manipulate data, and create powerful tools. In this article, we'll explore five ways to loop through cells in Excel VBA, each with its own strengths and weaknesses.
The Importance of Looping
Looping is a programming concept that involves iterating over a set of data or a range of cells. In Excel VBA, looping is essential for tasks such as data manipulation, formatting, and analysis. By using loops, you can automate repetitive tasks, reduce errors, and increase productivity.
Method 1: Using the For Next Loop
The For Next loop is a classic method for looping through cells in Excel VBA. It allows you to specify a range of cells and iterate over each cell in that range.
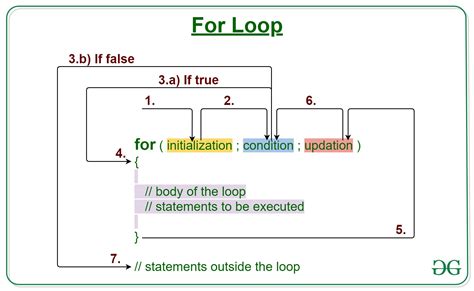
Example code:
Sub LoopThroughCells()
Dim cell As Range
For Each cell In Range("A1:A10")
' do something with the cell
cell.Value = cell.Value * 2
Next cell
End Sub
Method 2: Using the Do While Loop
The Do While loop is another popular method for looping through cells in Excel VBA. It allows you to specify a condition and iterate over each cell until that condition is met.
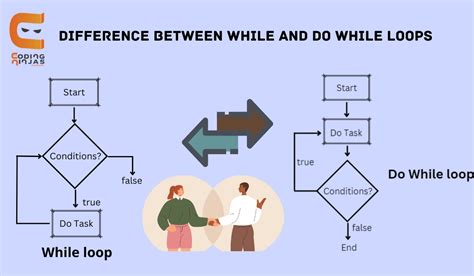
Example code:
Sub LoopThroughCells()
Dim cell As Range
Set cell = Range("A1")
Do While cell.Value <> ""
' do something with the cell
cell.Value = cell.Value * 2
Set cell = cell.Offset(1, 0)
Loop
End Sub
Method 3: Using the For Each Loop with a Collection
The For Each loop can also be used with a collection of cells, such as a Range or a Selection. This method is useful when you need to iterate over a specific set of cells.
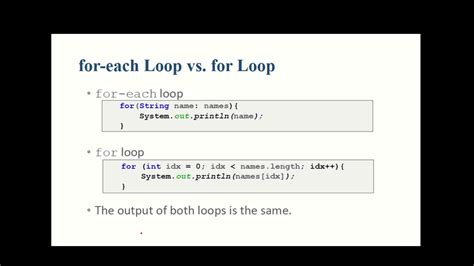
Example code:
Sub LoopThroughCells()
Dim cell As Range
For Each cell In Selection
' do something with the cell
cell.Value = cell.Value * 2
Next cell
End Sub
Method 4: Using the Loop with a Step Value
The For Next loop can also be used with a step value, which allows you to iterate over every nth cell.
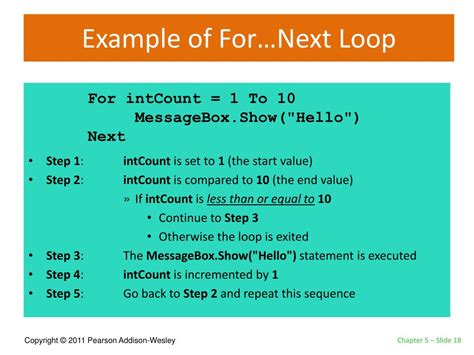
Example code:
Sub LoopThroughCells()
Dim cell As Range
For i = 1 To 10 Step 2
Set cell = Range("A" & i)
' do something with the cell
cell.Value = cell.Value * 2
Next i
End Sub
Method 5: Using the Loop with a Dynamic Range
The For Next loop can also be used with a dynamic range, which allows you to iterate over a range of cells that changes dynamically.
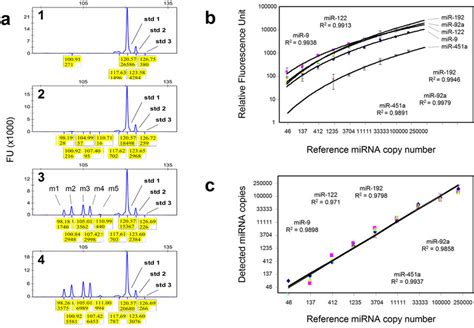
Example code:
Sub LoopThroughCells()
Dim cell As Range
Dim lastRow As Long
lastRow = Cells(Rows.Count, "A").End(xlUp).Row
For i = 1 To lastRow
Set cell = Range("A" & i)
' do something with the cell
cell.Value = cell.Value * 2
Next i
End Sub
Gallery of Excel VBA Loop Examples
Excel VBA Loop Examples
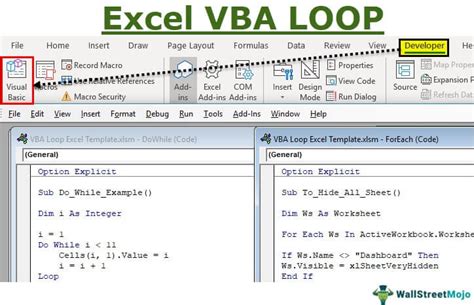
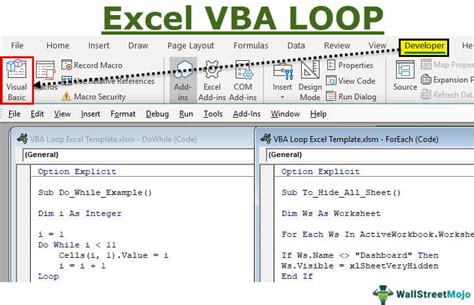
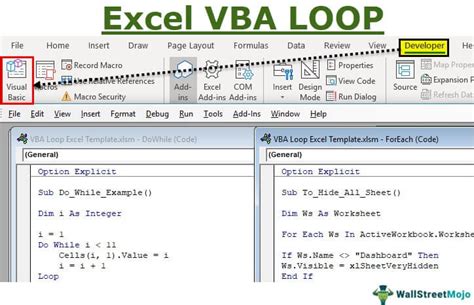
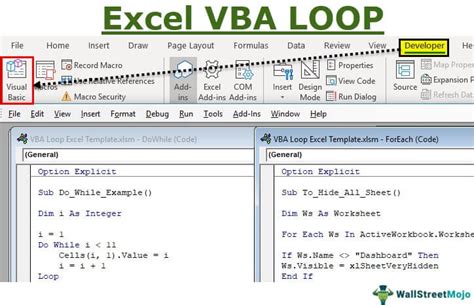
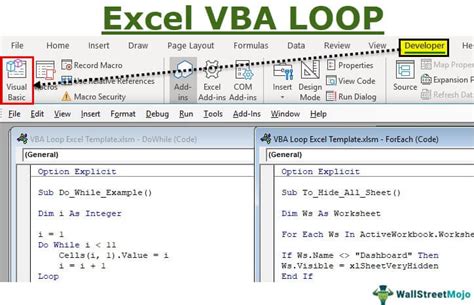
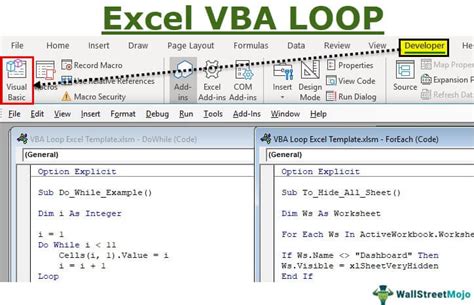
Conclusion
Looping through cells in Excel VBA is a fundamental skill that can help you automate tasks, manipulate data, and create powerful tools. By using the five methods outlined in this article, you can take your Excel development skills to the next level. Remember to practice and experiment with different looping techniques to become proficient in Excel VBA.