Intro
Unlock the power of Excel VBA functions by learning how to return values effectively. Discover 5 expert-approved methods to return values in VBA functions, including using function return types, ByRef arguments, and more. Master VBA value returns and boost your spreadsheet productivity with these actionable tips and tricks.
Returning values from Excel VBA functions is a crucial aspect of programming in Excel. When you create a function in VBA, you often want to return a value to the calling procedure or to a cell in your worksheet. In this article, we will explore five ways to return values from Excel VBA functions.
Understanding the Basics of VBA Functions
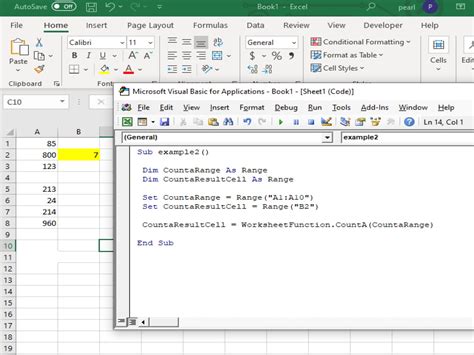
Before we dive into the different methods of returning values, let's quickly review the basics of VBA functions. A function in VBA is a block of code that performs a specific task and returns a value. Functions can take arguments, which are values passed to the function when it is called. The function then uses these arguments to perform its task and return a value.
Why Return Values from VBA Functions?
Returning values from VBA functions is essential in many situations. For example, you might want to create a function that calculates the sum of a range of cells and returns the result to a cell in your worksheet. Alternatively, you might want to create a function that takes a string as input and returns a modified version of the string.
Method 1: Using the Return Statement
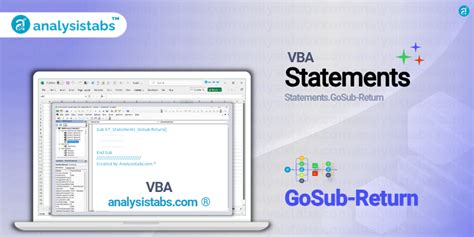
The most common way to return a value from a VBA function is to use the Return statement. The Return statement is used to specify the value that the function should return. Here is an example of a simple function that uses the Return statement:
Function AddNumbers(x As Integer, y As Integer) As Integer
AddNumbers = x + y
End Function
In this example, the function AddNumbers takes two integer arguments, x and y, and returns their sum. The Return statement is implicit in the function, and the value of x + y is returned to the calling procedure.
Method 2: Using a Variable to Store the Return Value
Another way to return a value from a VBA function is to use a variable to store the return value. This method is useful when you need to perform complex calculations or manipulate data before returning the value. Here is an example of a function that uses a variable to store the return value:
Function CalculateArea(length As Double, width As Double) As Double
Dim area As Double
area = length * width
CalculateArea = area
End Function
In this example, the function CalculateArea takes two double arguments, length and width, and returns their product. The variable area is used to store the return value, which is then assigned to the function name using the Return statement.
Method 3: Using an Array to Return Multiple Values
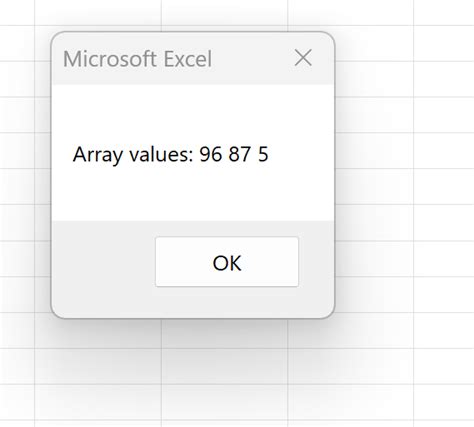
Sometimes, you might need to return multiple values from a VBA function. In this case, you can use an array to store the return values. Here is an example of a function that uses an array to return multiple values:
Function GetStudentInfo() As Variant
Dim studentInfo(2) As Variant
studentInfo(0) = "John Doe"
studentInfo(1) = 20
studentInfo(2) = "Math"
GetStudentInfo = studentInfo
End Function
In this example, the function GetStudentInfo returns an array of three values: the student's name, age, and major. The array is declared as a Variant data type, which can hold any type of data.
Method 4: Using a Collection to Return Multiple Values
Another way to return multiple values from a VBA function is to use a Collection object. A Collection is a built-in VBA object that can store multiple values of different data types. Here is an example of a function that uses a Collection to return multiple values:
Function GetEmployeeInfo() As Collection
Dim employeeInfo As New Collection
employeeInfo.Add "Jane Doe"
employeeInfo.Add 30
employeeInfo.Add "Sales"
Set GetEmployeeInfo = employeeInfo
End Function
In this example, the function GetEmployeeInfo returns a Collection object that contains three values: the employee's name, age, and department.
Method 5: Using a User-Defined Type to Return Multiple Values
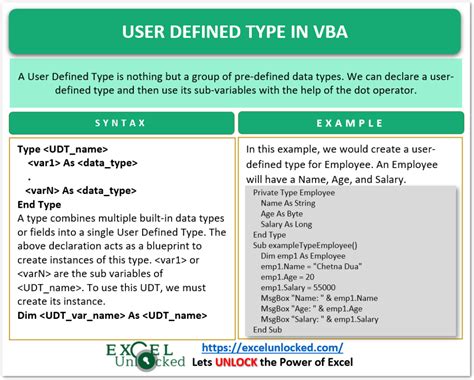
Finally, you can use a user-defined type (UDT) to return multiple values from a VBA function. A UDT is a custom data type that you can define in VBA to hold multiple values of different data types. Here is an example of a function that uses a UDT to return multiple values:
Type Person
Name As String
Age As Integer
Department As String
End Type
Function GetPersonInfo() As Person
Dim person As Person
person.Name = "Bob Smith"
person.Age = 40
person.Department = "IT"
GetPersonInfo = person
End Function
In this example, the function GetPersonInfo returns a UDT called Person, which contains three values: the person's name, age, and department.
Gallery of VBA Return Types
Here is a gallery of different VBA return types:
VBA Return Types Gallery
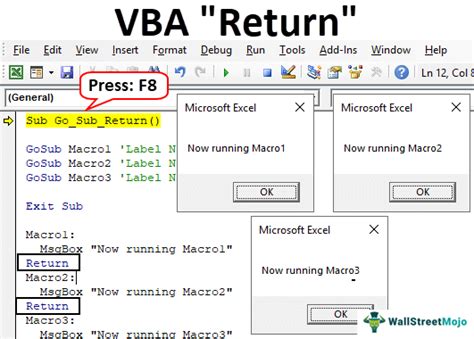
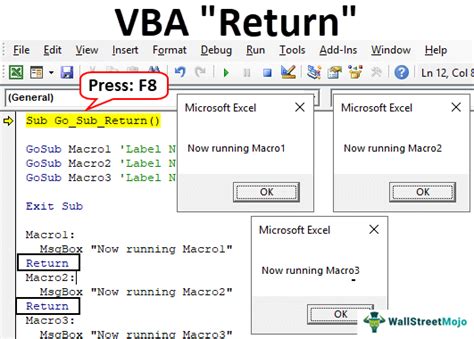
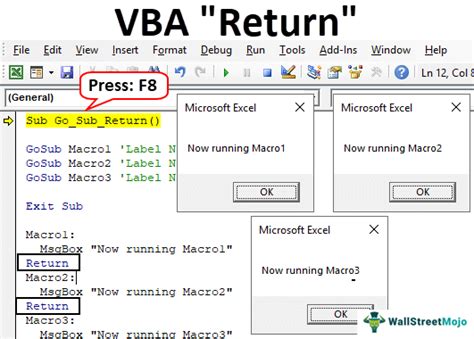
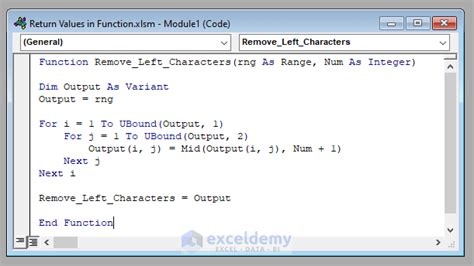
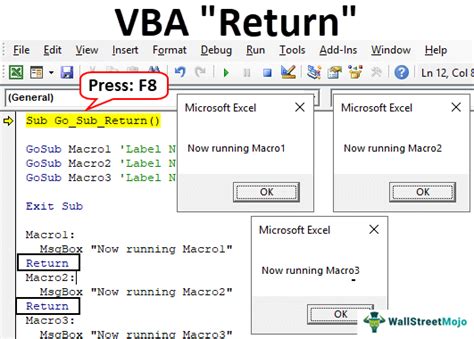
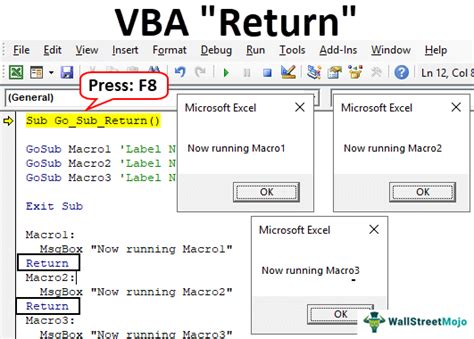
We hope this article has helped you understand the different ways to return values from Excel VBA functions. Whether you need to return a single value or multiple values, there is a method that can help you achieve your goal. Experiment with different return types and methods to find the one that works best for your needs.
Take the Next Step
Now that you have learned about the different ways to return values from Excel VBA functions, it's time to put your knowledge into practice. Try creating your own VBA functions using the methods described in this article. Experiment with different return types and methods to find the one that works best for your needs. Happy coding!