Intro
Master Excel VBA with our easy-to-follow guide on getting the last row with code examples. Learn how to efficiently retrieve the last row in Excel using VBA, including handling blank cells and dynamic ranges. Discover how to optimize your workflow with practical code snippets and expert tips on Excel VBA programming.
The art of finding the last row in Excel VBA! It's a crucial skill for any VBA enthusiast, and today, we'll dive into the world of easy code examples to master this technique.
The importance of finding the last row in Excel VBA cannot be overstated. Whether you're working with a small dataset or a massive spreadsheet, identifying the last row is essential for various tasks, such as looping through data, inserting new rows, or performing calculations. In this article, we'll explore the most efficient ways to get the last row in Excel VBA, along with practical examples and tips to enhance your coding skills.
Understanding the Last Row Concept
Before we dive into the code examples, it's essential to understand what we mean by the "last row" in Excel VBA. The last row refers to the row that contains data in a worksheet, and it can vary depending on the range or column you're working with. In Excel VBA, we can use various methods to determine the last row, including using the Range
, Worksheet
, and Cells
objects.
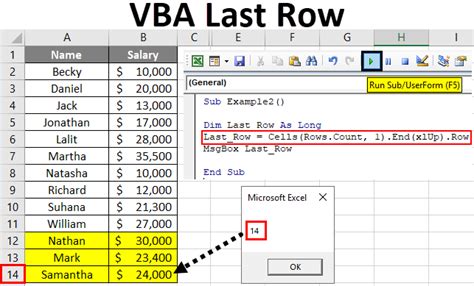
Method 1: Using the `Range.Find` Method
One of the most straightforward ways to find the last row in Excel VBA is by using the Range.Find
method. This method searches for a specific value in a range and returns the last row that contains data.
Sub GetLastRowWithFind()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells.Find("*", searchorder:=xlByRows, searchdirection:=xlPrevious).Row
MsgBox "The last row is: " & lastRow
End Sub
This code searches for any value ("*"
) in the worksheet, starting from the bottom-right corner, and returns the row number of the last cell that contains data.
Method 2: Using the `Worksheet.UsedRange` Property
Another approach to finding the last row is by using the Worksheet.UsedRange
property. This property returns the range of cells that contains data in the worksheet.
Sub GetLastRowWithUsedRange()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
Dim lastRow As Long
lastRow = ws.UsedRange.Rows.Count
MsgBox "The last row is: " & lastRow
End Sub
This code returns the number of rows in the used range, which can be used to determine the last row.
Method 3: Using the `Cells` Object
You can also use the Cells
object to find the last row in a worksheet. This method involves looping through the cells in a column and checking for the last cell that contains data.
Sub GetLastRowWithCells()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
MsgBox "The last row is: " & lastRow
End Sub
This code starts from the bottom of the worksheet ( ws.Rows.Count
) and moves up until it finds the last cell that contains data in column A ( Cells(ws.Rows.Count, 1)
).
Method 4: Using the `Range.CurrentRegion` Property
The Range.CurrentRegion
property returns the range of cells that surrounds the active cell. We can use this property to find the last row in a worksheet.
Sub GetLastRowWithCurrentRegion()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Range("A1").CurrentRegion.Rows.Count
MsgBox "The last row is: " & lastRow
End Sub
This code returns the number of rows in the current region, starting from cell A1.
Comparing the Methods
Each method has its strengths and weaknesses. Here's a summary of the methods we've covered:
Method | Description | Performance |
---|---|---|
Range.Find |
Searches for a value in a range | Fast |
Worksheet.UsedRange |
Returns the range of cells that contains data | Medium |
Cells Object |
Loops through cells in a column | Slow |
Range.CurrentRegion |
Returns the range of cells that surrounds the active cell | Medium |
In general, the Range.Find
method is the fastest and most efficient way to find the last row in a worksheet. However, the other methods can be useful in specific situations, such as when working with large datasets or complex ranges.
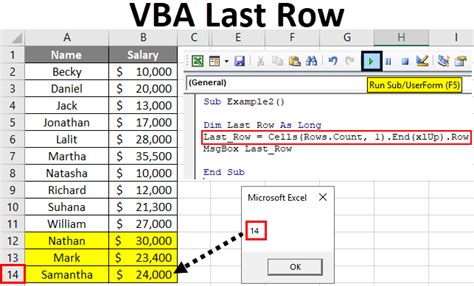
Conclusion
Finding the last row in Excel VBA is a crucial skill for any VBA enthusiast. By mastering the methods outlined in this article, you'll be able to write more efficient and effective code. Whether you're working with small datasets or massive spreadsheets, understanding the last row concept is essential for tasks like looping through data, inserting new rows, or performing calculations.
Remember to choose the method that best suits your needs, and don't hesitate to experiment with different approaches to optimize your code.
What's your favorite method for finding the last row in Excel VBA? Share your thoughts in the comments below!
Excel VBA Last Row Image Gallery
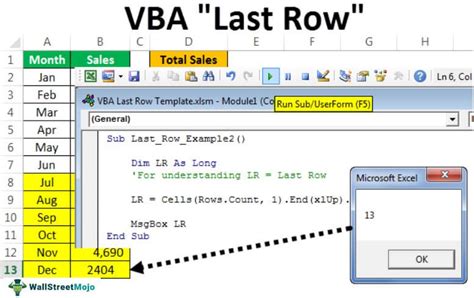
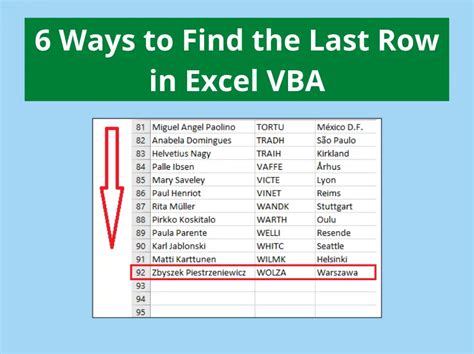
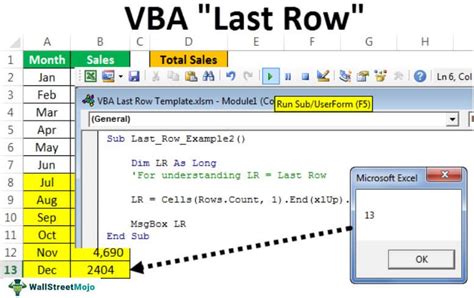
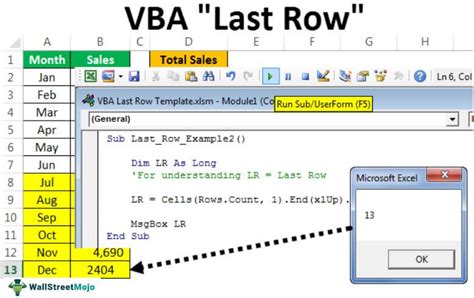
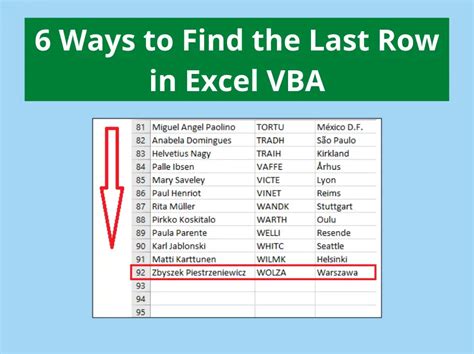
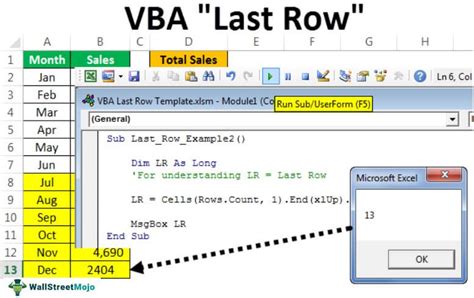
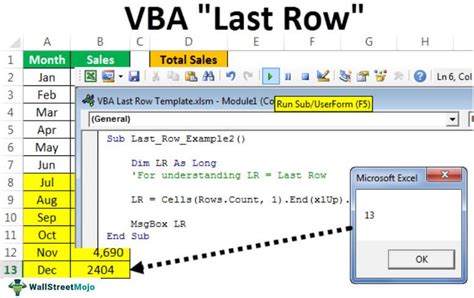
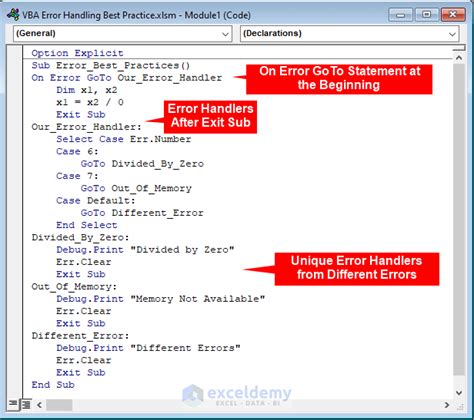
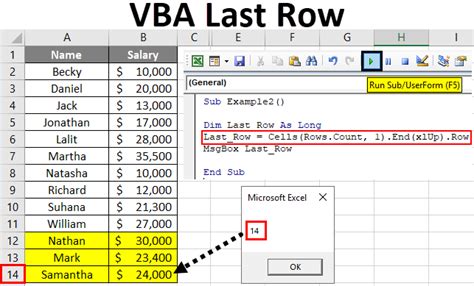
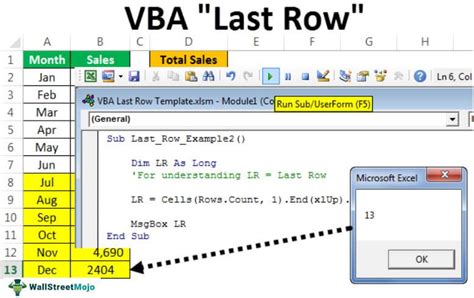