Intro
Discover how to seamlessly conceal data in Excel using VBA. Learn 5 expert methods to hide rows in Excel VBA, including using loops, conditional statements, and worksheet events. Master auto-hide techniques, error handling, and more. Optimize your spreadsheets with these efficient VBA row hiding strategies and take your data management to the next level.
Working with large datasets in Excel can sometimes be overwhelming, especially when you need to focus on specific data while hiding the rest. This is where hiding rows in Excel comes in handy. While you can manually hide rows, using Excel VBA (Visual Basic for Applications) provides a more efficient and automated way to manage your data. In this article, we will explore five different methods to hide rows in Excel using VBA, each serving a unique purpose based on your data analysis needs.
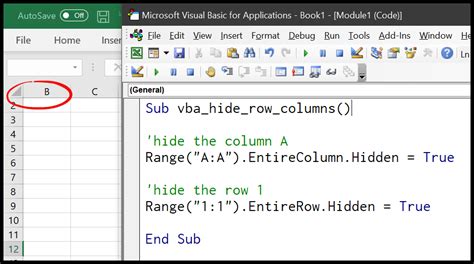
1. Hiding Rows Based on a Specific Condition
One of the most common reasons to hide rows is to conceal data that does not meet a certain condition. For example, if you have a list of sales data and you want to hide all rows where the sales amount is less than a certain threshold, you can use the following VBA script:
Sub HideRowsBasedOnCondition()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Dim i As Long
For i = lastRow To 1 Step -1
If ws.Cells(i, "B").Value < 100 Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script hides rows where the value in column B is less than 100. You can adjust the condition to fit your specific needs.
2. Hiding Rows with Errors
Data errors can significantly impact your analysis. Hiding rows with errors can help you focus on accurate data. Here’s how you can do it:
Sub HideRowsWithErrors()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Dim i As Long
For i = lastRow To 1 Step -1
If IsError(ws.Cells(i, "B").Value) Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script hides rows where the value in column B is an error.
3. Hiding Duplicate Rows
Duplicate data can skew your analysis. Here’s a VBA script to hide duplicate rows based on values in a specific column:
Sub HideDuplicateRows()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Dim i As Long
For i = lastRow To 2 Step -1
If ws.Cells(i, "B").Value = ws.Cells(i - 1, "B").Value Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script hides rows that have the same value in column B as the row above it.
4. Hiding Rows with Blank Cells
Blank cells can also be a nuisance in your data analysis. Here’s how you can hide rows with blank cells in a specific column:
Sub HideRowsWithBlanks()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Dim i As Long
For i = lastRow To 1 Step -1
If ws.Cells(i, "B").Value = "" Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script hides rows where the cell in column B is blank.
5. Hiding Rows Based on User Input
Sometimes, you might want to hide rows based on criteria provided by the user. This can be achieved through an input box:
Sub HideRowsBasedOnUserInput()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Dim userInput As Variant
userInput = InputBox("Please enter the threshold value", "Threshold Value")
Dim i As Long
For i = lastRow To 1 Step -1
If ws.Cells(i, "B").Value < userInput Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script prompts the user to enter a threshold value and then hides rows where the value in column B is less than the input value.
Excel VBA Hide Rows Gallery
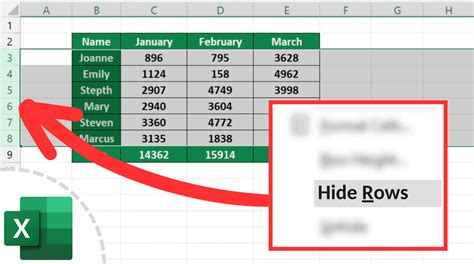
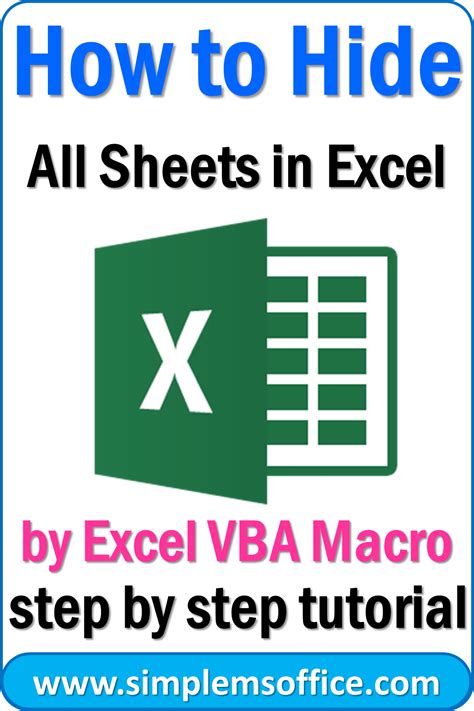
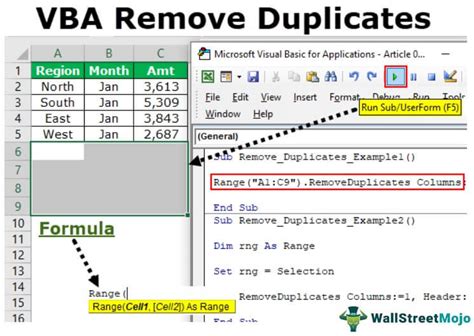
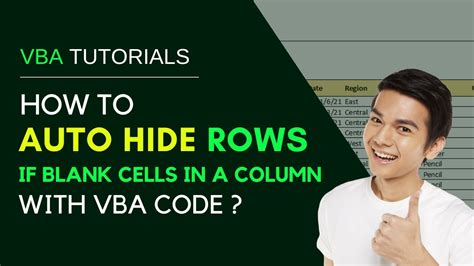
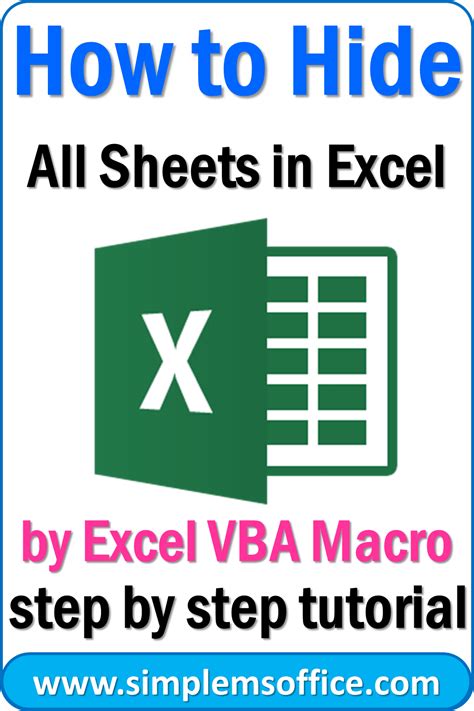
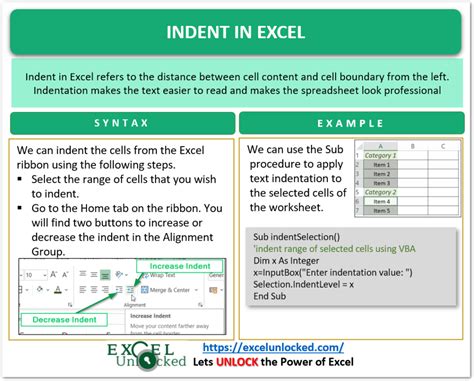
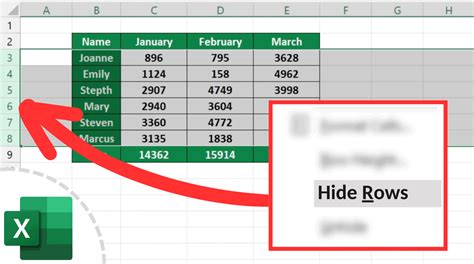
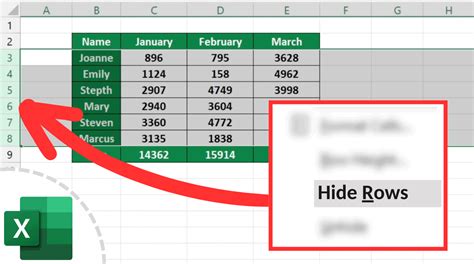
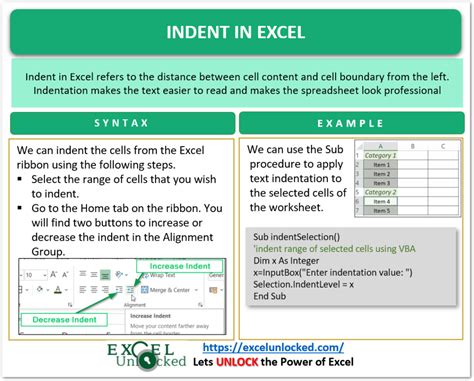
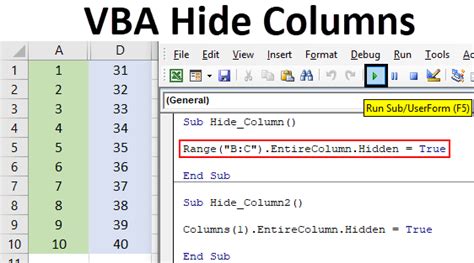
Final Thoughts
Hiding rows in Excel using VBA is a powerful tool for managing your data. By automating the process, you can save time and focus on more critical aspects of your analysis. Whether you're dealing with conditions, errors, duplicates, blanks, or user input, there's a VBA script that can help. Experiment with the scripts provided above, and don’t hesitate to adapt them to fit your specific needs. Remember, the key to mastering VBA is practice and patience.
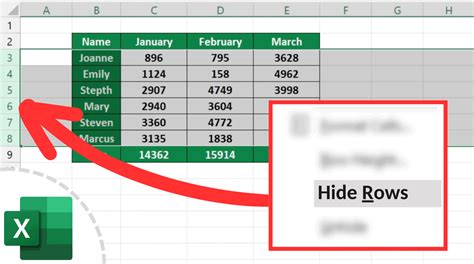
If you have any questions or need further assistance with hiding rows in Excel using VBA, feel free to ask in the comments below. Share your own experiences or tips for working with VBA, and let’s continue the conversation.