Intro
Resolve Excel VBA invalid forward reference issues with ease. Learn how to identify and fix errors, avoiding compile-time problems. Master debugging techniques, VBA module management, and referencing external libraries. Boost productivity with our expert tips on Excel VBA development, code optimization, and error handling best practices.
The world of Excel VBA can be a wonderful place, full of automation and efficiency. However, it can also be a frustrating one, especially when dealing with errors like the "Invalid Forward Reference" issue. This error can occur when Excel VBA is unable to resolve a reference to a variable, function, or other element that has not been defined yet. In this article, we will explore the reasons behind this error and provide step-by-step solutions to resolve it.
What is an Invalid Forward Reference in Excel VBA?
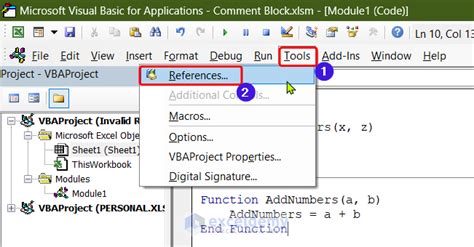
An Invalid Forward Reference occurs when Excel VBA encounters a reference to a variable, function, or other element that has not been defined yet. This can happen when the code is executed in a top-down manner, and the referenced element is defined later in the code.
Causes of Invalid Forward References
- Out of Order Declarations: When variables or functions are declared in the wrong order, it can lead to forward reference errors.
- Undeclared Variables: Using variables without declaring them first can cause forward reference errors.
- Missing or Incorrect Type Libraries: Missing or incorrect type libraries can lead to forward reference errors, especially when working with external libraries.
Resolving Invalid Forward Reference Issues in Excel VBA
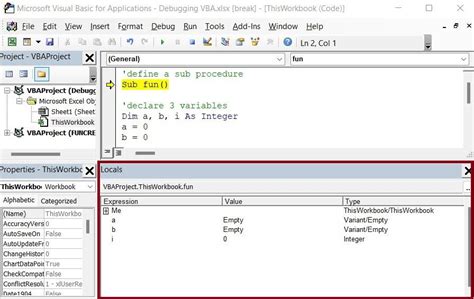
To resolve Invalid Forward Reference issues in Excel VBA, follow these step-by-step solutions:
1. Rearrange Declarations
Rearrange the declarations of variables, functions, and other elements to ensure that they are in the correct order. Declare variables and functions before they are used.
2. Declare Variables Explicitly
Use the Dim
statement to declare variables explicitly, and ensure that they are declared before they are used.
3. Check Type Libraries
Check that the correct type libraries are referenced in the project. Go to Tools > References in the Visual Basic Editor to check the referenced libraries.
4. Use Option Explicit
Use Option Explicit
at the top of the module to force explicit declaration of variables.
5. Break Down Large Code Blocks
Break down large code blocks into smaller, more manageable pieces. This can help identify and resolve forward reference errors.
6. Use Debugging Tools
Use debugging tools like the Immediate window, Watch window, and Step Through feature to identify and resolve forward reference errors.
Best Practices to Avoid Forward Reference Errors
- Use Modular Code: Break down large code blocks into smaller, modular pieces.
- Use Descriptive Variable Names: Use descriptive variable names to avoid confusion.
- Use Option Explicit: Use
Option Explicit
to force explicit declaration of variables. - Check References: Check that the correct type libraries are referenced in the project.
Example Code to Demonstrate Forward Reference Errors
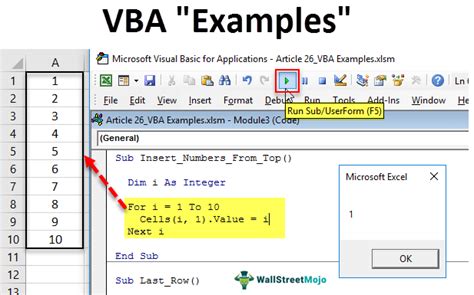
Here is an example code that demonstrates forward reference errors:
Sub ExampleCode()
' Declare variables
Dim x As Integer
Dim y As Integer
' Use variables before they are declared
z = x + y
' Declare variable z after it is used
Dim z As Integer
End Sub
This code will result in a forward reference error because variable z
is used before it is declared.
Conclusion
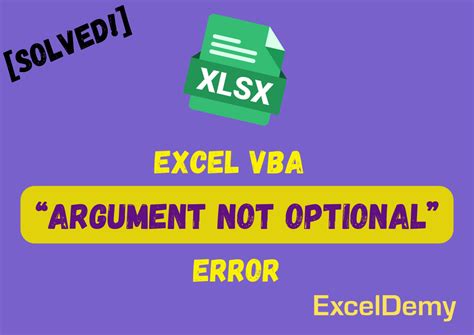
Forward reference errors can be frustrating, but by following the step-by-step solutions and best practices outlined in this article, you can easily resolve them. Remember to rearrange declarations, declare variables explicitly, check type libraries, use Option Explicit
, break down large code blocks, and use debugging tools to resolve forward reference errors.
Excel VBA Image Gallery
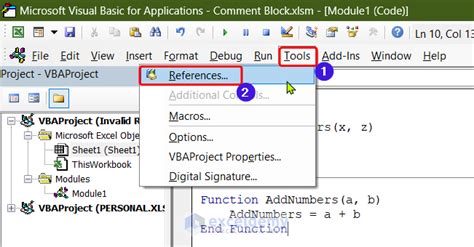
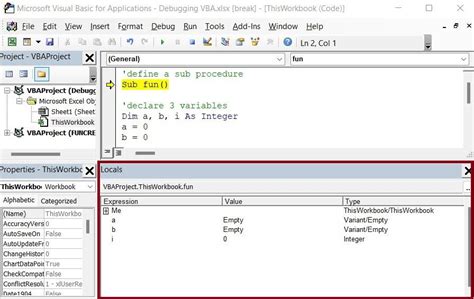
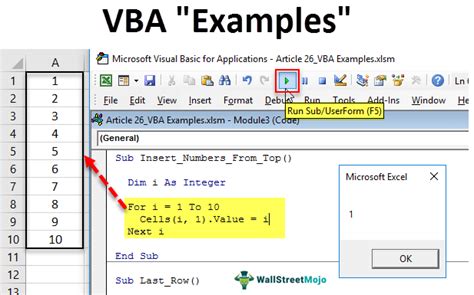
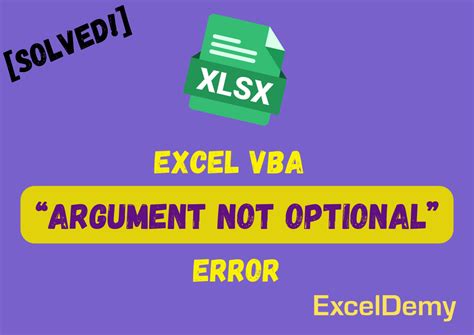
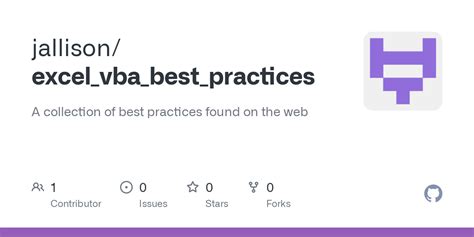
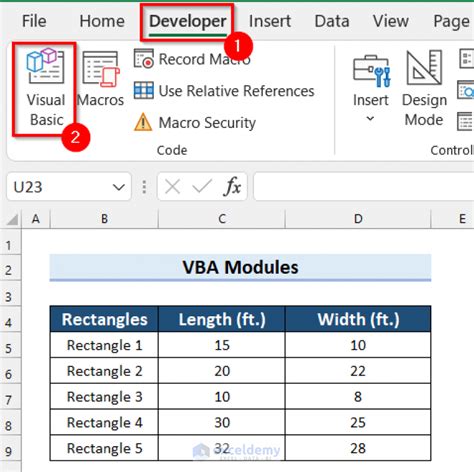
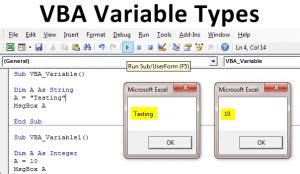
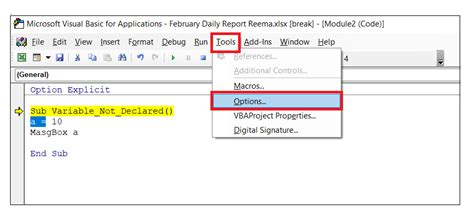
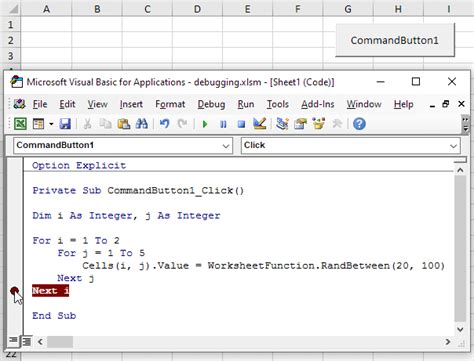
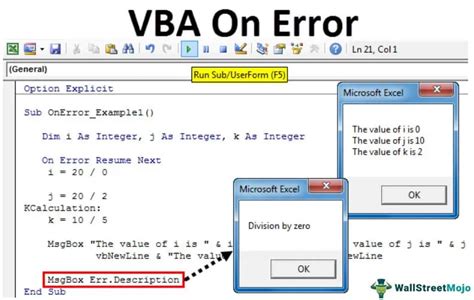
Feel free to comment, share this article, or ask questions about resolving forward reference errors in Excel VBA.