Intro
Unlock the full potential of Excel VBA with merged cells. Master the art of working with merged cells in VBA, including referencing, formatting, and manipulating data. Learn how to overcome common challenges and optimize your VBA code for efficiency and performance. Take your Excel skills to the next level with expert tips and tricks.
Mastering Excel VBA can be a game-changer for anyone who works with spreadsheets regularly. However, one of the most common challenges that VBA programmers face is dealing with merged cells. Merged cells can make it difficult to navigate and manipulate data in a worksheet, but with the right techniques, you can master working with them in VBA.
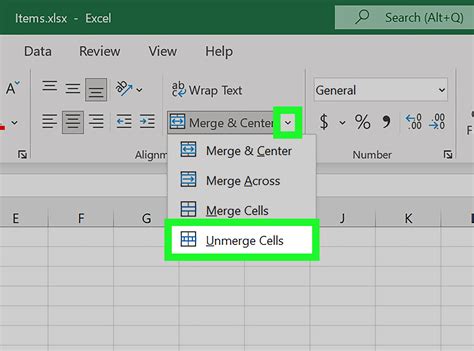
In this article, we'll explore the different ways you can work with merged cells in Excel VBA. We'll cover the basics of merged cells, how to identify and select them, and how to manipulate data within them. By the end of this article, you'll be equipped with the knowledge and skills to tackle even the most complex merged cell tasks.
Understanding Merged Cells
Merged cells are cells that have been combined into a single cell. When you merge cells, you can create a single cell that spans multiple rows and columns. Merged cells can be useful for creating headers, titles, and other types of content that need to span multiple cells.
However, merged cells can also cause problems when working with VBA. Because merged cells are treated as a single cell, they can be difficult to navigate and manipulate using VBA code.
Identifying Merged Cells
To identify merged cells in a worksheet, you can use the MergeCells
property of the Range
object. This property returns a Boolean
value indicating whether the cells in the range are merged.
Here's an example of how to use the MergeCells
property:
Sub CheckForMergedCells()
Dim rng As Range
Set rng = Range("A1:E1")
If rng.MergeCells Then
MsgBox "The cells in the range are merged."
Else
MsgBox "The cells in the range are not merged."
End If
End Sub
This code checks whether the cells in the range A1:E1 are merged. If they are, it displays a message box indicating that the cells are merged.
Selecting Merged Cells
Selecting merged cells can be tricky, but there are a few techniques you can use to make it easier. One way to select merged cells is to use the MergeArea
property of the Range
object. This property returns a Range
object that represents the merged area of the cell.
Here's an example of how to use the MergeArea
property:
Sub SelectMergedCells()
Dim rng As Range
Set rng = Range("A1")
If rng.MergeCells Then
rng.MergeArea.Select
End If
End Sub
This code selects the merged area of the cell in range A1. If the cell is not merged, it does nothing.
Manipulating Data in Merged Cells
Manipulating data in merged cells can be challenging, but there are a few techniques you can use to make it easier. One way to manipulate data in merged cells is to use the Value
property of the Range
object. This property allows you to get and set the value of the cell.
Here's an example of how to use the Value
property:
Sub ManipulateMergedCells()
Dim rng As Range
Set rng = Range("A1:E1")
If rng.MergeCells Then
rng.Value = "Hello World"
End If
End Sub
This code sets the value of the merged cells in range A1:E1 to "Hello World". If the cells are not merged, it does nothing.
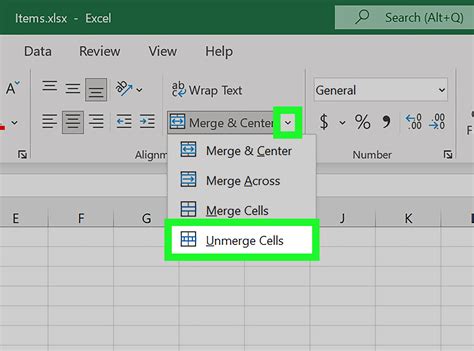
Working with Merged Cell Ranges
Working with merged cell ranges can be complex, but there are a few techniques you can use to make it easier. One way to work with merged cell ranges is to use the Range
object's Cells
property. This property allows you to access individual cells within a range.
Here's an example of how to use the Cells
property:
Sub WorkWithMergedCellRanges()
Dim rng As Range
Set rng = Range("A1:E1")
If rng.MergeCells Then
For Each cell In rng.Cells
cell.Value = "Hello World"
Next cell
End If
End Sub
This code sets the value of each cell in the merged range A1:E1 to "Hello World". If the cells are not merged, it does nothing.
Unmerging Cells
Unmerging cells can be useful when you need to split a merged cell into individual cells. To unmerge cells, you can use the UnMerge
method of the Range
object.
Here's an example of how to use the UnMerge
method:
Sub UnmergeCells()
Dim rng As Range
Set rng = Range("A1:E1")
If rng.MergeCells Then
rng.UnMerge
End If
End Sub
This code unmerges the cells in range A1:E1. If the cells are not merged, it does nothing.
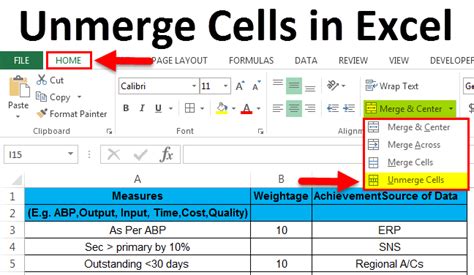
Best Practices for Working with Merged Cells
Here are some best practices to keep in mind when working with merged cells in Excel VBA:
- Always check whether a range is merged before attempting to manipulate it.
- Use the
MergeArea
property to select merged cells. - Use the
Value
property to get and set the value of merged cells. - Use the
Cells
property to access individual cells within a merged range. - Avoid using the
Range
object'sAddress
property to access merged cells, as this can cause errors.
Conclusion
Mastering Excel VBA with merged cells requires practice and patience, but with the right techniques, you can tackle even the most complex tasks. By following the best practices outlined in this article, you can ensure that your VBA code is robust, efficient, and easy to maintain.
We hope this article has been helpful in your journey to master Excel VBA with merged cells. If you have any questions or need further assistance, please don't hesitate to ask.
Excel VBA Merged Cells Gallery
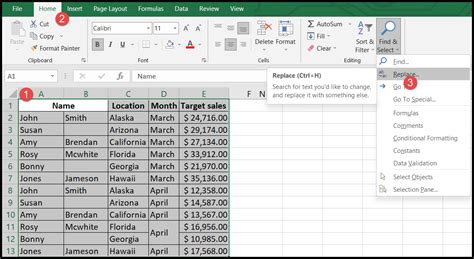
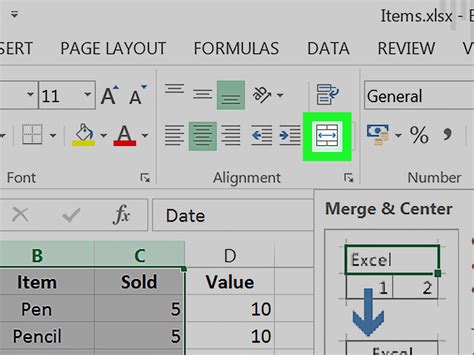
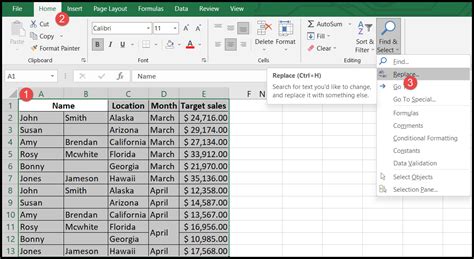
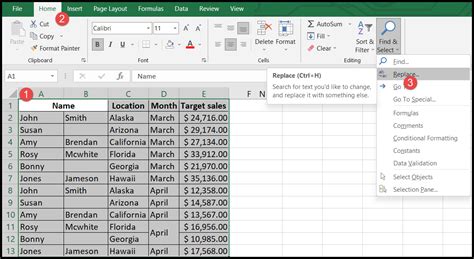
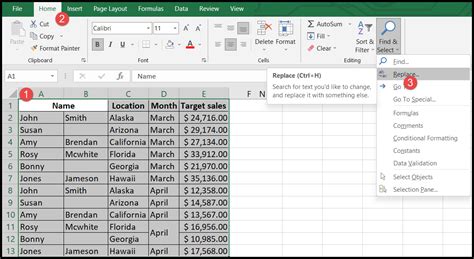
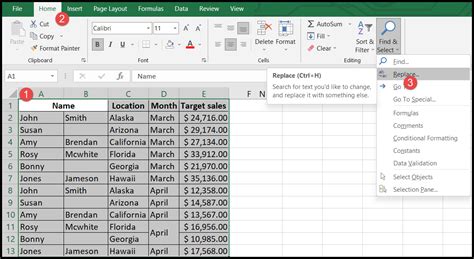
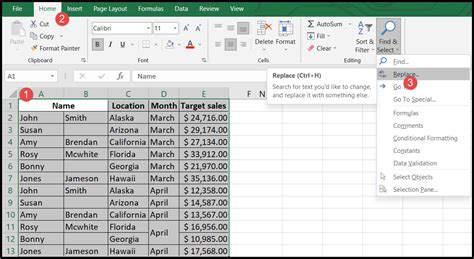
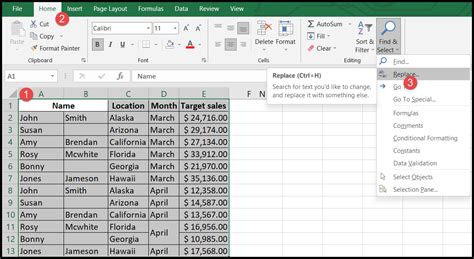
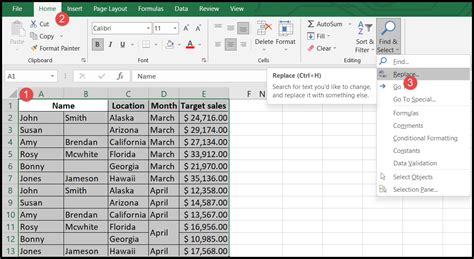
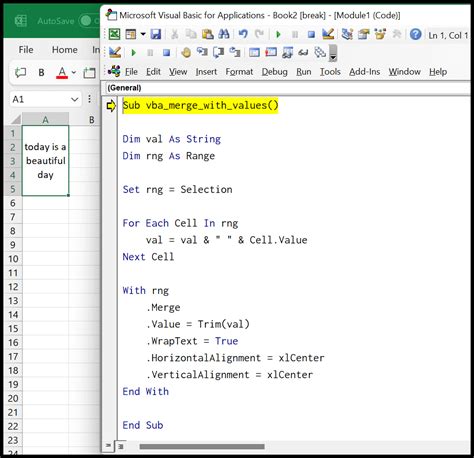