Excel VBA message boxes are a crucial tool for interacting with users and getting their input. One of the most common uses of message boxes is to ask users a yes or no question. In this article, we will explore how to create and use yes/no message boxes in Excel VBA, making it easy for you to implement this functionality in your own projects.
Why Use Message Boxes?
Message boxes are an excellent way to communicate with users and get their input. They provide a simple and intuitive way to ask users questions, display information, and even warn them about potential issues. By using message boxes, you can make your VBA code more user-friendly and interactive.
Creating a Yes/No Message Box
To create a yes/no message box in Excel VBA, you can use the MsgBox
function. This function takes two arguments: the prompt (the text to be displayed in the message box) and the buttons (the type of buttons to be displayed).
Here is an example of how to create a simple yes/no message box:
Sub YesNoMessageBox()
Dim response As Variant
response = MsgBox("Do you want to proceed?", vbYesNo)
End Sub
In this example, the MsgBox
function displays a message box with the prompt "Do you want to proceed?" and two buttons: Yes and No. The vbYesNo
argument specifies that the message box should display only two buttons.
Understanding the MsgBox Function
The MsgBox
function is a versatile tool that can be used to create a wide range of message boxes. Here are some key things to understand about the MsgBox
function:
- The first argument is the prompt, which is the text to be displayed in the message box.
- The second argument is the buttons, which specifies the type of buttons to be displayed.
- The
MsgBox
function returns a value indicating which button the user clicked.
Here are some common values for the buttons argument:
vbOKOnly
: Displays only an OK button.vbOKCancel
: Displays OK and Cancel buttons.vbYesNo
: Displays Yes and No buttons.vbYesNoCancel
: Displays Yes, No, and Cancel buttons.
Getting the User's Response
To get the user's response from a yes/no message box, you can use the return value of the MsgBox
function. The return value will be one of the following:
vbYes
: The user clicked the Yes button.vbNo
: The user clicked the No button.vbCancel
: The user clicked the Cancel button (if displayed).
Here is an example of how to use the return value to get the user's response:
Sub YesNoMessageBox()
Dim response As Variant
response = MsgBox("Do you want to proceed?", vbYesNo)
If response = vbYes Then
' User clicked Yes
ElseIf response = vbNo Then
' User clicked No
End If
End Sub
Adding More Functionality to Your Message Box
While the basic yes/no message box is useful, you can add more functionality to your message box by using other arguments of the MsgBox
function. Here are some examples:
vbExclamation
: Displays an exclamation mark icon in the message box.vbInformation
: Displays an information icon in the message box.vbCritical
: Displays a critical icon in the message box.vbDefaultButton1
: Specifies that the first button (e.g., Yes) is the default button.vbDefaultButton2
: Specifies that the second button (e.g., No) is the default button.
Here is an example of how to use these arguments to add more functionality to your message box:
Sub YesNoMessageBox()
Dim response As Variant
response = MsgBox("Do you want to proceed?", vbYesNo + vbExclamation + vbDefaultButton1)
End Sub
In this example, the message box displays an exclamation mark icon and specifies that the Yes button is the default button.
Embedding Images in Message Boxes
You can also embed images in message boxes using the LoadPicture
function. Here is an example of how to do this:
Sub YesNoMessageBox()
Dim response As Variant
response = MsgBox("Do you want to proceed?", vbYesNo, "Embed Image", LoadPicture("C:\image.jpg"))
End Sub
In this example, the message box displays an image loaded from the file "C:\image.jpg".
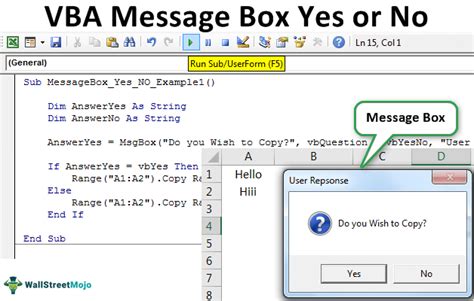
Gallery of Excel VBA Message Box Yes No Examples
Excel VBA Message Box Yes No Gallery
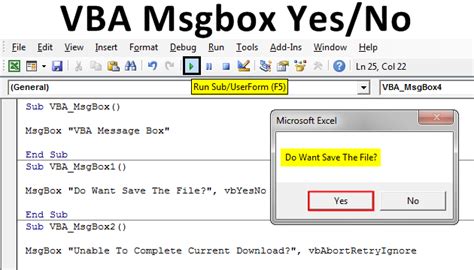
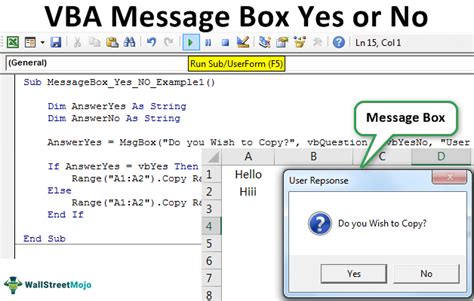
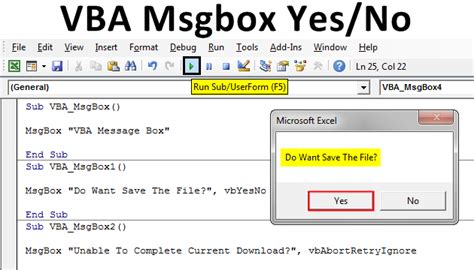
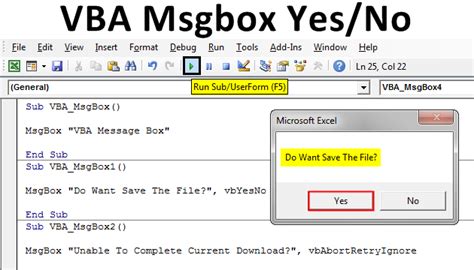
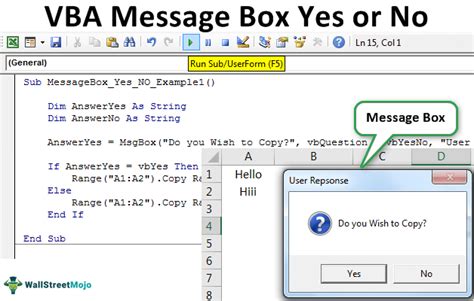
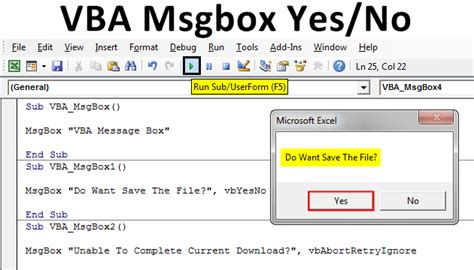
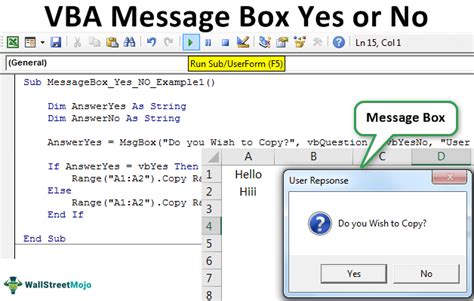
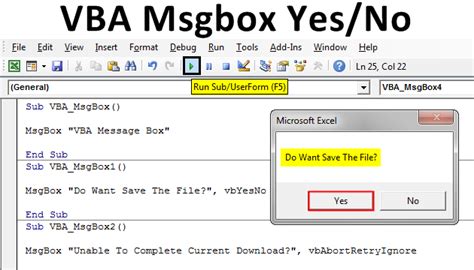
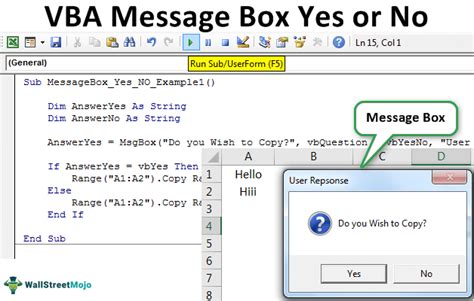
Conclusion
In this article, we have explored how to create and use yes/no message boxes in Excel VBA. We have covered the basics of the MsgBox
function, including how to create a simple yes/no message box and how to get the user's response. We have also discussed how to add more functionality to your message box, including embedding images and specifying default buttons.
By following the examples and tips in this article, you should be able to create effective yes/no message boxes in your own Excel VBA projects. Remember to keep your message boxes clear and concise, and to use them sparingly to avoid overwhelming your users.
We hope this article has been helpful! If you have any questions or need further assistance, please don't hesitate to ask.