Intro
Discover how to automate PDF printing in Excel using VBA. Learn 5 efficient methods to print to PDF using Excel VBA, including late binding, early binding, and shell execution. Master VBA programming and create custom PDF printing solutions with Excel. Easily convert, export, and save your worksheets as PDF files with these expert tips.
Printing to PDF using Excel VBA is a valuable skill, especially for those who need to automate tasks or create reports in a specific format. In this article, we will explore five ways to print to PDF using Excel VBA, including using the Adobe Acrobat library, the PDFCreator library, and other methods.
Why Print to PDF?
Before we dive into the methods, let's discuss why printing to PDF is useful. PDF (Portable Document Format) files are widely supported and can be easily shared, making them ideal for reports, invoices, and other documents that need to be distributed electronically. Additionally, PDFs can be password-protected and signed digitally, ensuring security and authenticity.
Method 1: Using the Adobe Acrobat Library
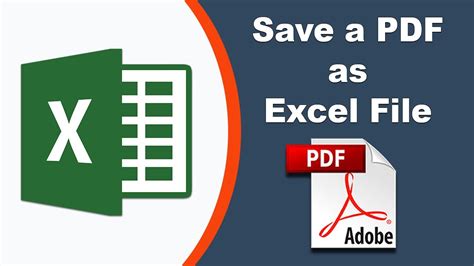
One way to print to PDF using Excel VBA is to use the Adobe Acrobat library. To do this, you will need to have Adobe Acrobat installed on your system. Once installed, you can add the Adobe Acrobat library to your VBA project by following these steps:
- Open the Visual Basic Editor in Excel
- Click Tools > References
- Check the box next to "Adobe Acrobat" and click OK
With the library added, you can use the following code to print a worksheet to PDF:
Sub PrintToPDF()
Dim acrApp As New Acrobat.AcroApp
Dim acrAVDoc As New Acrobat.AcroAVDoc
Dim pdfFile As String
' Set the file path and name for the PDF file
pdfFile = "C:\path\to\file.pdf"
' Open the worksheet to print
Worksheets("Sheet1").Activate
' Print the worksheet to PDF
acrAVDoc.PrintToPDF pdfFile, Worksheets("Sheet1")
' Clean up
Set acrAVDoc = Nothing
Set acrApp = Nothing
End Sub
Method 2: Using the PDFCreator Library
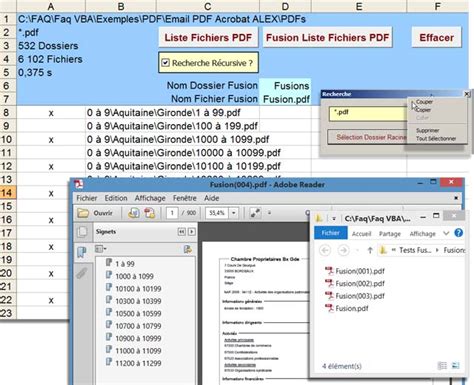
Another way to print to PDF using Excel VBA is to use the PDFCreator library. PDFCreator is a free tool that allows you to create PDF files from any application that can print. To use the PDFCreator library, you will need to download and install PDFCreator on your system.
Once installed, you can add the PDFCreator library to your VBA project by following these steps:
- Open the Visual Basic Editor in Excel
- Click Tools > References
- Check the box next to "PDFCreator" and click OK
With the library added, you can use the following code to print a worksheet to PDF:
Sub PrintToPDF()
Dim pdfCreator As New PDFCreator.PDFCreator
' Set the file path and name for the PDF file
pdfFile = "C:\path\to\file.pdf"
' Open the worksheet to print
Worksheets("Sheet1").Activate
' Print the worksheet to PDF
pdfCreator.PrintToPDF pdfFile, Worksheets("Sheet1")
' Clean up
Set pdfCreator = Nothing
End Sub
Method 3: Using the Excel VBA PrintOut Method
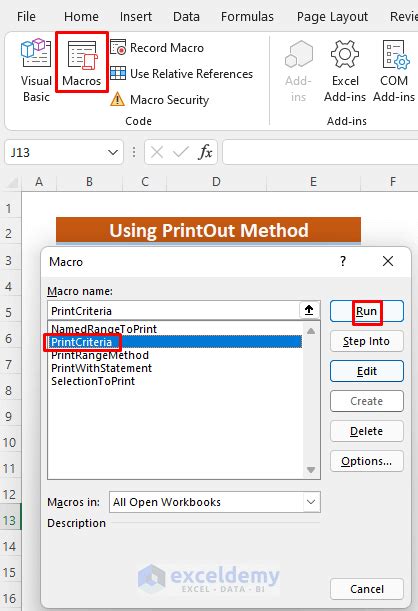
A third way to print to PDF using Excel VBA is to use the PrintOut method. This method allows you to print a worksheet to a file, including PDF files. To use the PrintOut method, you can use the following code:
Sub PrintToPDF()
' Set the file path and name for the PDF file
pdfFile = "C:\path\to\file.pdf"
' Open the worksheet to print
Worksheets("Sheet1").Activate
' Print the worksheet to PDF
Worksheets("Sheet1").PrintOut _
FileName:=pdfFile, _
PrintToFile:=True, _
ActivePrinter:="Adobe PDF"
' Clean up
End Sub
Method 4: Using the Windows API
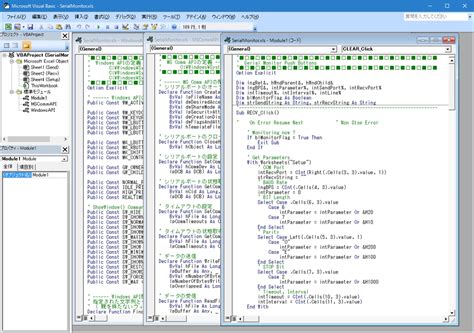
A fourth way to print to PDF using Excel VBA is to use the Windows API. This method requires you to declare the Windows API functions and use them to print the worksheet to a PDF file. To use the Windows API, you can use the following code:
Declare PtrSafe Function ShellExecute _
Lib "shell32.dll" Alias "ShellExecuteA" ( _
ByVal hwnd As Long, _
ByVal lpOperation As String, _
ByVal lpFile As String, _
ByVal lpParameters As String, _
ByVal lpDirectory As String, _
ByVal nShowCmd As Long) As Long
Sub PrintToPDF()
' Set the file path and name for the PDF file
pdfFile = "C:\path\to\file.pdf"
' Open the worksheet to print
Worksheets("Sheet1").Activate
' Print the worksheet to PDF
ShellExecute 0&, "print", pdfFile, "", "", 0&
' Clean up
End Sub
Method 5: Using a Third-Party Add-In
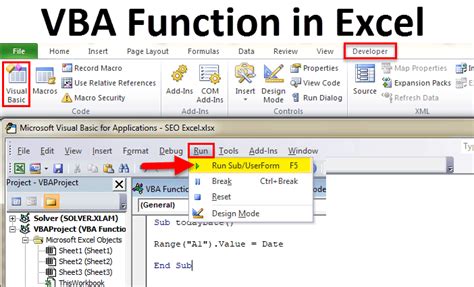
A fifth way to print to PDF using Excel VBA is to use a third-party add-in, such as PDF-XChange or Print Conductor. These add-ins provide a simple way to print worksheets to PDF files, and often offer additional features such as editing and converting PDF files.
To use a third-party add-in, you will need to download and install the add-in on your system. Once installed, you can use the add-in to print worksheets to PDF files.
Conclusion
In this article, we explored five ways to print to PDF using Excel VBA, including using the Adobe Acrobat library, the PDFCreator library, the Excel VBA PrintOut method, the Windows API, and a third-party add-in. Each method has its own advantages and disadvantages, and the best method for you will depend on your specific needs and requirements.
We hope this article has been helpful in providing you with the information you need to print to PDF using Excel VBA. If you have any questions or need further assistance, please don't hesitate to ask.
Gallery of Excel VBA PDF Printing
Excel VBA PDF Printing Image Gallery
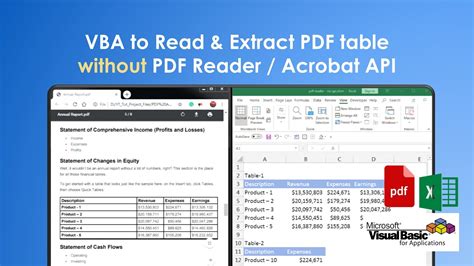
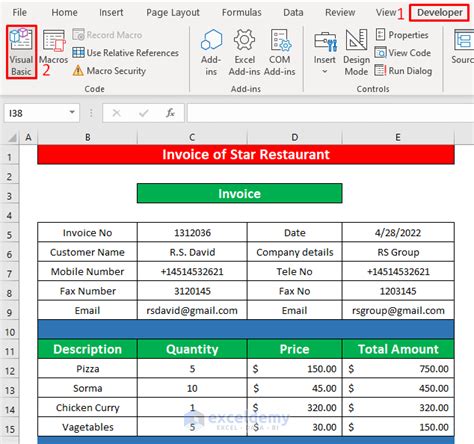
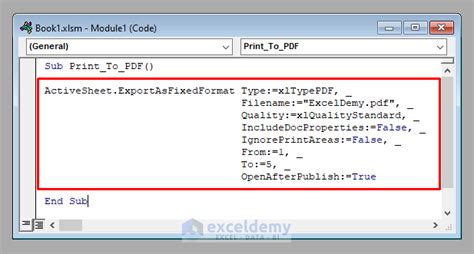
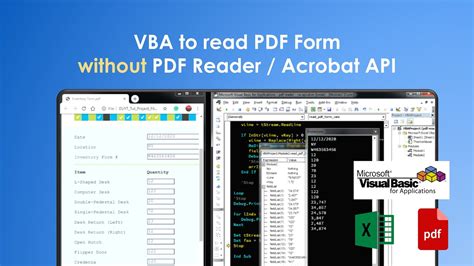
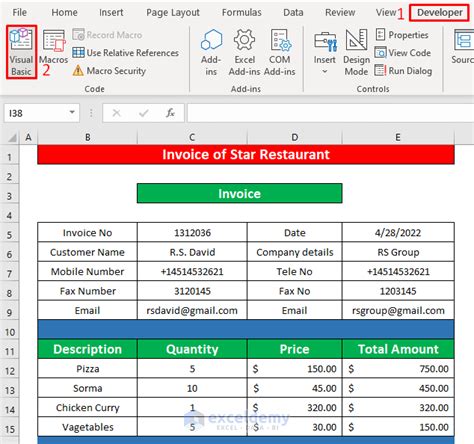
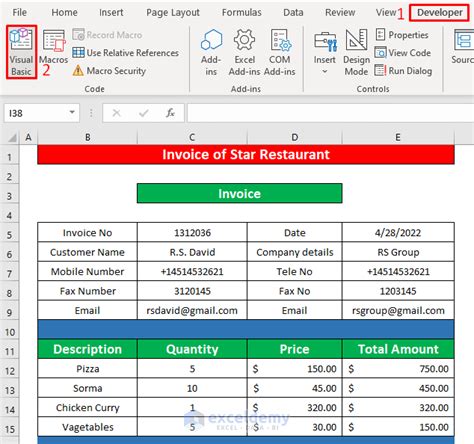
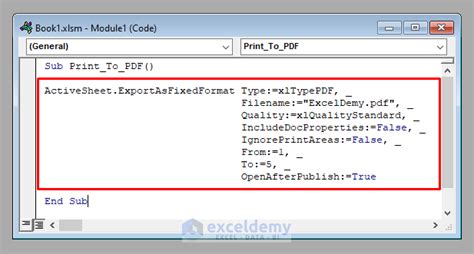
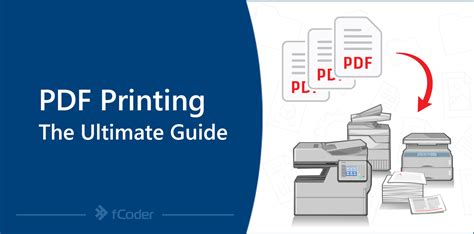
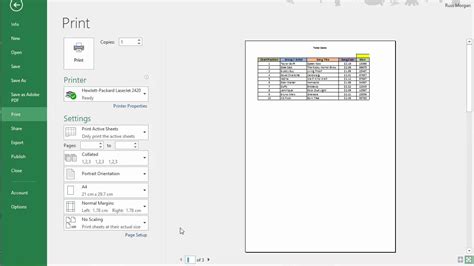
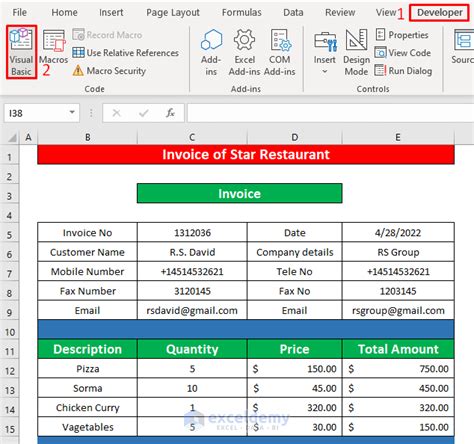