Intro
Discover how to streamline your Excel workflow by mastering VBA sheet removal techniques. Learn 5 efficient methods to delete sheets in Excel VBA, including using loops, specifying sheet names, and more. Improve your coding skills and optimize your spreadsheets with these expert-approved tips and tricks.
Working with Excel can be a cumbersome task, especially when dealing with multiple sheets. Managing these sheets is crucial for data organization and analysis. One of the most efficient ways to automate tasks in Excel is by using Visual Basic for Applications (VBA). This programming language allows users to create macros that can perform a variety of tasks, including removing unwanted sheets.
Removing sheets in Excel VBA is a common requirement for many users. Whether you're looking to declutter your workbook, remove sensitive data, or reorganize your sheets, Excel VBA provides a straightforward solution. In this article, we'll explore five ways to remove sheets in Excel VBA.
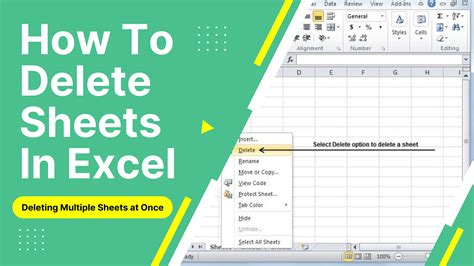
Why Remove Sheets in Excel VBA?
Before diving into the methods, let's discuss why removing sheets in Excel VBA is useful. Removing unnecessary sheets can help:
- Improve workbook performance
- Reduce file size
- Enhance data organization
- Remove sensitive information
- Automate repetitive tasks
Method 1: Delete a Single Sheet Using VBA
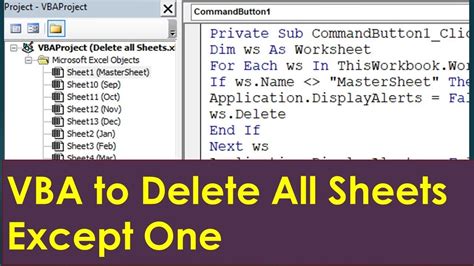
The simplest way to remove a sheet in Excel VBA is by using the Delete
method. Here's an example code snippet:
Sub DeleteSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
ws.Delete
End Sub
In this code, replace "Sheet1"
with the name of the sheet you want to delete.
Step-by-Step Instructions
- Open the Visual Basic Editor by pressing
Alt + F11
or navigating to Developer > Visual Basic. - In the Editor, click
Insert
>Module
to create a new module. - Paste the code snippet into the module.
- Replace
"Sheet1"
with the name of the sheet you want to delete. - Press
F5
to run the code.
Method 2: Delete Multiple Sheets Using VBA
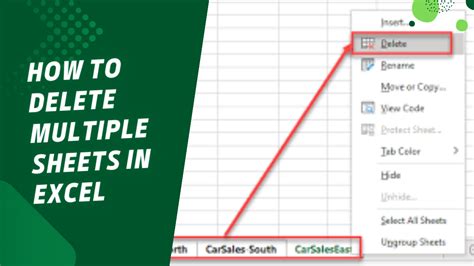
To remove multiple sheets, you can modify the previous code to loop through an array of sheet names.
Sub DeleteMultipleSheets()
Dim ws As Worksheet
Dim sheetNames As Variant
sheetNames = Array("Sheet1", "Sheet2", "Sheet3")
For Each ws In ThisWorkbook.Worksheets
If IsInArray(ws.Name, sheetNames) Then
ws.Delete
End If
Next ws
End Sub
Function IsInArray(val As String, arr As Variant) As Boolean
Dim i As Long
For i = LBound(arr) To UBound(arr)
If arr(i) = val Then
IsInArray = True
Exit Function
End If
Next i
IsInArray = False
End Function
In this code, replace the sheetNames
array with the names of the sheets you want to delete.
Step-by-Step Instructions
- Open the Visual Basic Editor by pressing
Alt + F11
or navigating to Developer > Visual Basic. - In the Editor, click
Insert
>Module
to create a new module. - Paste the code snippet into the module.
- Replace the
sheetNames
array with the names of the sheets you want to delete. - Press
F5
to run the code.
Method 3: Delete All Sheets Except One Using VBA
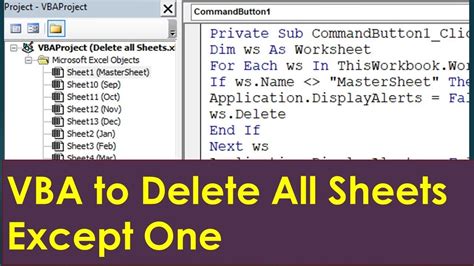
To remove all sheets except one, you can loop through the worksheets and delete each sheet that doesn't match the name of the sheet you want to keep.
Sub DeleteAllSheetsExceptOne()
Dim ws As Worksheet
Dim keepSheet As String
keepSheet = "Sheet1"
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> keepSheet Then
ws.Delete
End If
Next ws
End Sub
In this code, replace "Sheet1"
with the name of the sheet you want to keep.
Step-by-Step Instructions
- Open the Visual Basic Editor by pressing
Alt + F11
or navigating to Developer > Visual Basic. - In the Editor, click
Insert
>Module
to create a new module. - Paste the code snippet into the module.
- Replace
"Sheet1"
with the name of the sheet you want to keep. - Press
F5
to run the code.
Method 4: Delete Sheets Based on a Condition Using VBA
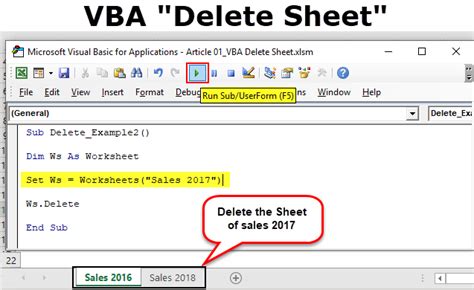
You can also delete sheets based on a specific condition, such as the sheet name containing a certain string.
Sub DeleteSheetsBasedOnCondition()
Dim ws As Worksheet
Dim condition As String
condition = "temp"
For Each ws In ThisWorkbook.Worksheets
If InStr(ws.Name, condition) > 0 Then
ws.Delete
End If
Next ws
End Sub
In this code, replace "temp"
with the condition you want to use.
Step-by-Step Instructions
- Open the Visual Basic Editor by pressing
Alt + F11
or navigating to Developer > Visual Basic. - In the Editor, click
Insert
>Module
to create a new module. - Paste the code snippet into the module.
- Replace
"temp"
with the condition you want to use. - Press
F5
to run the code.
Method 5: Delete Sheets Using a UserForm in VBA
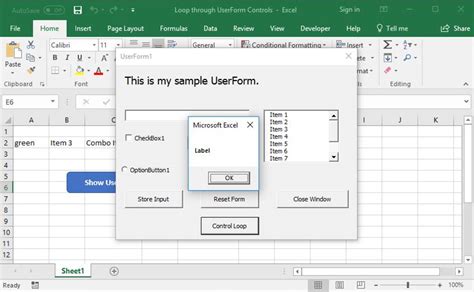
You can also create a UserForm to delete sheets interactively.
Sub DeleteSheetsUsingUserForm()
Dim ws As Worksheet
Dim userForm As UserForm
Set userForm = New UserForm1
userForm.Show
For Each ws In ThisWorkbook.Worksheets
If userForm.chkDelete.Value = True Then
ws.Delete
End If
Next ws
End Sub
In this code, you need to create a UserForm with a checkbox control.
Step-by-Step Instructions
- Open the Visual Basic Editor by pressing
Alt + F11
or navigating to Developer > Visual Basic. - In the Editor, click
Insert
>User Form
to create a new UserForm. - Add a checkbox control to the UserForm.
- Paste the code snippet into the module.
- Press
F5
to run the code.
Remove Sheets in Excel VBA Image Gallery
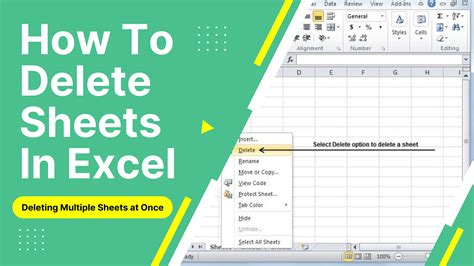
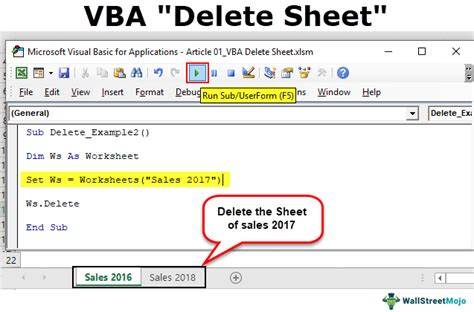
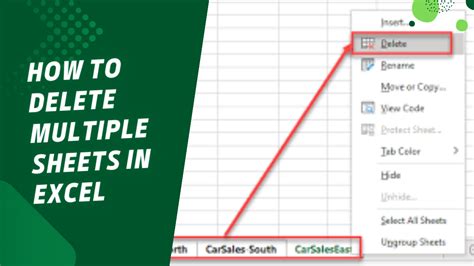
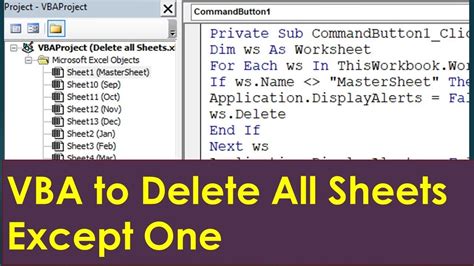
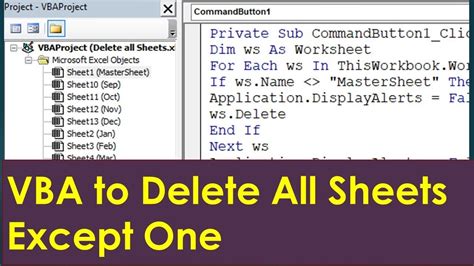
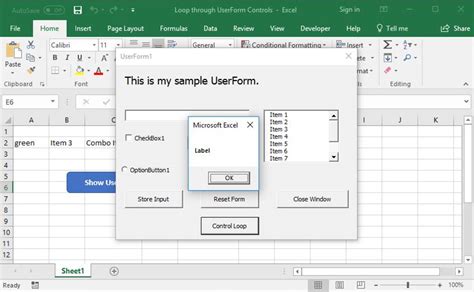
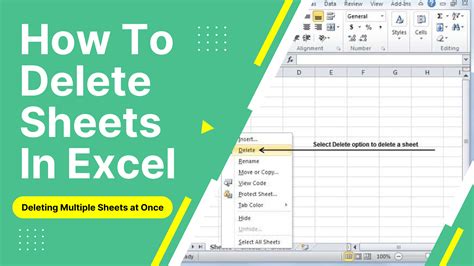
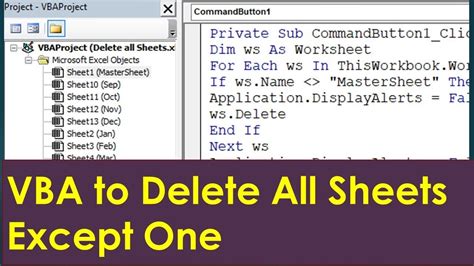
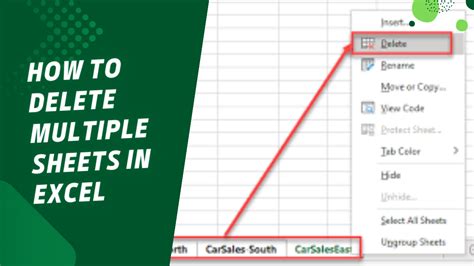
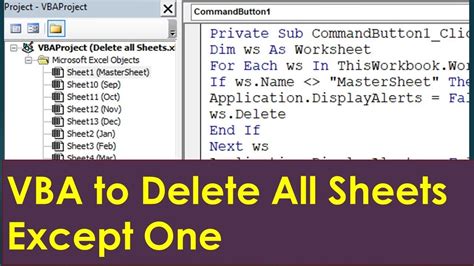
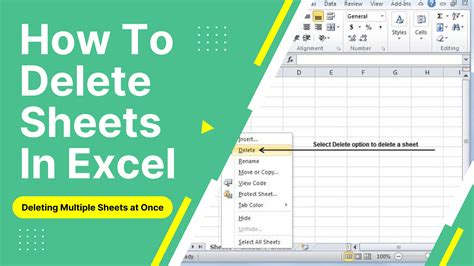
We hope this article has helped you learn how to remove sheets in Excel VBA. Whether you're looking to delete a single sheet or multiple sheets, these methods will help you streamline your workflow and improve your productivity.